Mastering Test Double Patterns for Flawless Unit Testing
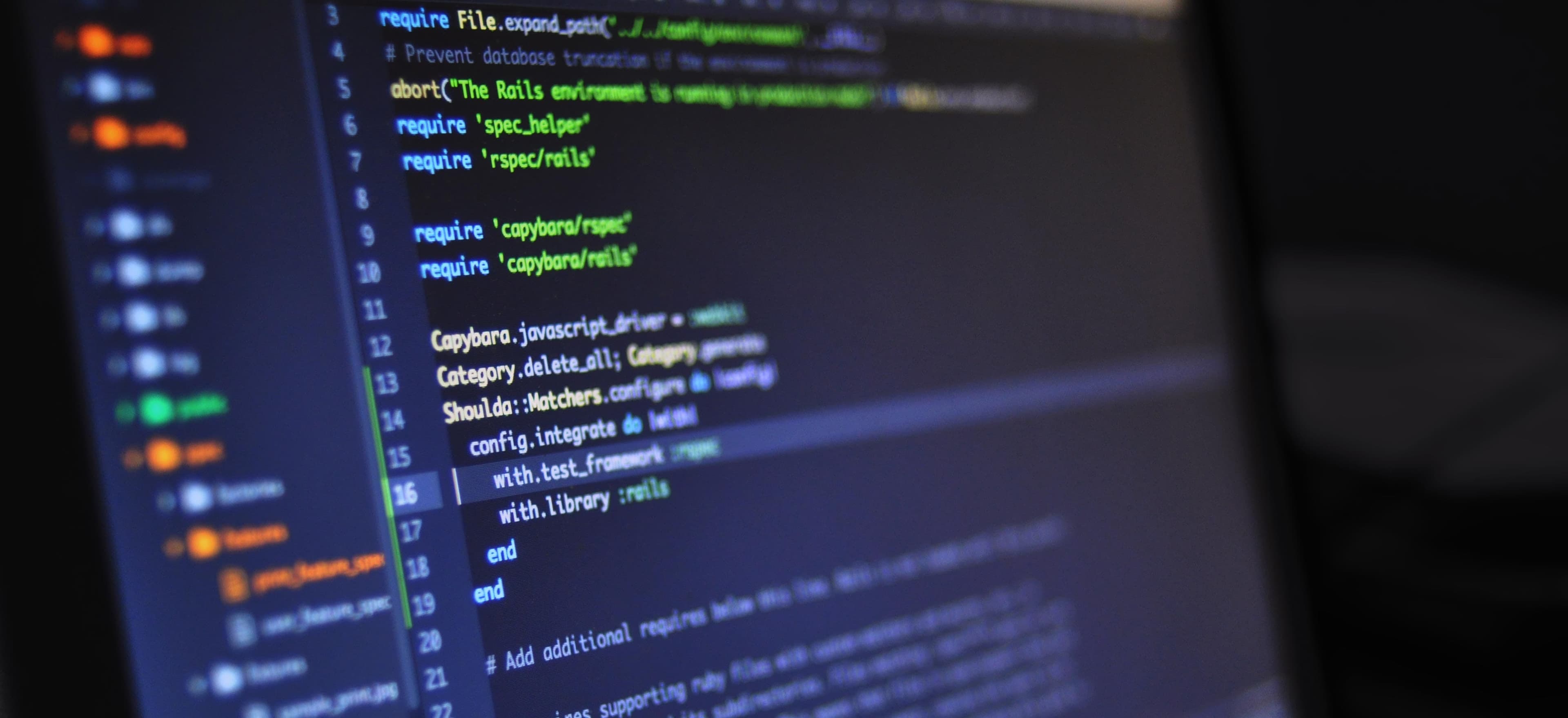
- Published on
Mastering Test Double Patterns for Flawless Unit Testing
Unit testing is an essential part of software development. It ensures that individual components of your code work as expected. However, writing effective unit tests can be tricky, especially when dealing with dependencies. This is where Test Doubles come into play. In this blog post, we will explore the concept of Test Doubles, discuss different patterns, and showcase how they contribute to more robust unit testing in Java.
Table of Contents
- What is a Test Double?
- Types of Test Doubles
- Dummy
- Stub
- Spy
- Mock
- Applying Test Doubles in Java
- Example with Stubs
- Example with Mocks
- Best Practices for Using Test Doubles
- Conclusion
What is a Test Double?
A Test Double is a generic term used to describe any case where you replace a production class with a lightweight version for testing purposes. This replacement helps in isolating the unit under test by controlling the environment and its dependencies.
When a unit test references other collaborating classes, you would typically want to avoid executing these collaborators' real code for the following reasons:
- Performance: Real dependencies might be slow, causing tests to run longer than necessary.
- Isolation: The behavior of a unit test can be affected by external factors.
- Determinism: You want tests that can consistently pass or fail based on the code changes.
Types of Test Doubles
There are four primary types of Test Doubles, each serving a different purpose:
Dummy
Dummies are simple placeholders that are passed into the unit under test but are not used. They are only there to satisfy parameter requirements.
public class DummyExample {
public void processUser(User dummyUser) {
// This method does not use the dummyUser.
// Some processing logic...
}
}
Stub
Stubs are used to provide predetermined responses to method calls. They allow you to simulate different scenarios without requiring real dependencies.
public interface UserService {
String getUserInfo(String userId);
}
public class UserServiceStub implements UserService {
public String getUserInfo(String userId) {
return "Test User Info for " + userId; // Predefined response
}
}
Why Stubs?
Using stubs allows you to simulate different environments and scenarios without the overhead of a real implementation. This is crucial for testing error paths or exceptional scenarios.
Spy
Spies are like partial mocks; they record information about how a method was called. You can use them to verify that certain interactions occurred while still maintaining the real functionality of the methods.
public class UserService {
public void saveUser(User user) {
// Implementation to save a user...
}
}
public class UserServiceSpy extends UserService {
private User savedUser;
@Override
public void saveUser(User user) {
savedUser = user; // Record the user being saved
super.saveUser(user); // Call the real method
}
public User getSavedUser() {
return savedUser;
}
}
Mock
Mocks are the most powerful type of Test Double. They not only simulate the behavior of the dependency but also record interactions. It is common to use libraries like Mockito to create mocks easily.
import static org.mockito.Mockito.*;
public class UserManagerTest {
@Test
public void testUserManagement() {
UserService userService = mock(UserService.class);
when(userService.getUserInfo("1")).thenReturn("Mocked User");
UserManager userManager = new UserManager(userService);
String userInfo = userManager.retrieveUserInfo("1");
verify(userService).getUserInfo("1"); // Verify method call
assertEquals("Mocked User", userInfo); // Confirm behavior
}
}
Why Mocks?
Mocks provide powerful verification capabilities. They allow you to verify that a method was called with certain parameters, providing additional guarantees about your code's behavior.
Applying Test Doubles in Java
Integrating Test Doubles into your unit tests is straightforward. Let's see how this works in practice.
Example with Stubs
Suppose you are testing a UserManager
class that relies on an external UserService
:
public class UserManager {
private final UserService userService;
public UserManager(UserService userService) {
this.userService = userService;
}
public String retrieveUserInfo(String userId) {
return userService.getUserInfo(userId);
}
}
// Test using Stub
public class UserManagerTest {
@Test
public void testRetrieveUserInfoWithStub() {
UserService userServiceStub = new UserServiceStub();
UserManager userManager = new UserManager(userServiceStub);
String userInfo = userManager.retrieveUserInfo("123");
assertEquals("Test User Info for 123", userInfo);
}
}
Example with Mocks
Using a Mock for the same scenario:
import static org.mockito.Mockito.*;
public class UserManagerMockTest {
@Test
public void testRetrieveUserInfoWithMock() {
UserService userServiceMock = mock(UserService.class);
when(userServiceMock.getUserInfo("123")).thenReturn("Mocked User Info");
UserManager userManager = new UserManager(userServiceMock);
String userInfo = userManager.retrieveUserInfo("123");
verify(userServiceMock).getUserInfo("123");
assertEquals("Mocked User Info", userInfo);
}
}
Best Practices for Using Test Doubles
-
Keep It Simple: Don’t overcomplicate your tests with unnecessary doubles. Use only what you need.
-
Clear Intent: The purpose of each Test Double should be clear from the context of the test.
-
Limit Scope: Use mocks only when you need to verify interactions. For simple data returns, prefer using stubs.
-
Use Libraries Wisely: While libraries can provide extensive functionality, you should understand their behavior and limitations. Mockito is a popular choice, and you can find more about it at Mockito Documentation.
-
Refactor as Needed: Code changes may require adjustments in your test doubles. Regularly review and refactor your tests to ensure they align with the current codebase.
Closing Remarks
Mastering Test Double patterns is essential for writing effective unit tests in Java. Dummies, Stubs, Spies, and Mocks each serve distinct purposes and, when used appropriately, can significantly enhance your test structure and reliability. Through careful application, you can ensure your tests are both maintainable and communicate their intent clearly. Happy testing!
For further exploration into effective unit testing practices, consider checking out JUnit 5 User Guide for additional insights into creating robust tests.
Checkout our other articles