Mastering Spring Profiles: Troubleshooting XML Configuration Issues
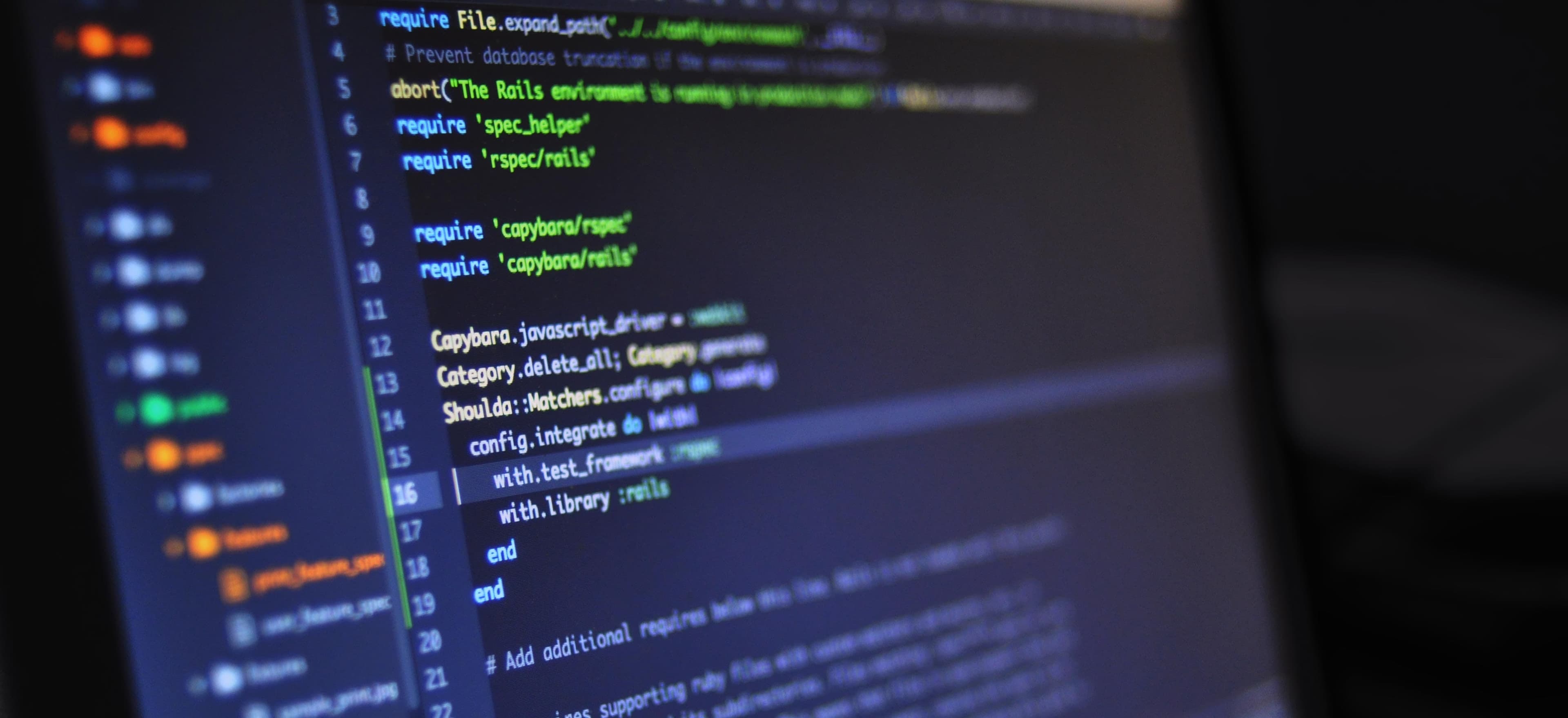
- Published on
Mastering Spring Profiles: Troubleshooting XML Configuration Issues
Spring Framework is a powerful tool for building Java applications. One of its most advantageous features is Spring Profiles, which allows you to segregate parts of your application configuration and make it only available in certain environments. However, when working with XML configuration, you might encounter some challenges. This blog post aims to shed light on common issues you might face and how to troubleshoot them effectively.
What are Spring Profiles?
Spring Profiles is an integral part of the Spring Framework that allows you to define beans that are loaded conditionally based on the active profile. This can be especially useful when you are running your application in different environments—like development, testing, and production.
For example, let's consider a scenario where you might use different databases in different environments. You can define two profiles: dev
for your development environment and prod
for production.
Defining Profiles
You can define profile-specific beans in your XML configuration like this:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<beans profile="dev">
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/dev_db"/>
<property name="username" value="devuser"/>
<property name="password" value="devpass"/>
</bean>
</beans>
<beans profile="prod">
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/prod_db"/>
<property name="username" value="produser"/>
<property name="password" value="prodpass"/>
</bean>
</beans>
</beans>
Activating Profiles
To activate a Spring profile, you can set it in several ways. The most common method is through application properties. For example, you can add the following line to your configuration file:
spring.profiles.active=dev
Common XML Configuration Issues
As you progress with your implementation, you may face issues related to XML configuration for Spring Profiles. Here are a few tips to troubleshoot common problems:
1. Misconfigured Profiles
The first thing to check is whether your profiles are correctly defined and activated. If you mistype a profile name or forget to include the profile
attribute in your XML bean definition, Spring won’t load those beans.
Tip: Always double-check your profile names for consistency across XML configurations and properties.
2. Beans Not Loaded
If the beans specific to a profile are not loaded, you may need to check:
- Ensure that you are activating the correct profile.
- Validate your XML syntax; misplaced tags can lead to sections being ignored.
For example, if you forgot to declare an XML header like this:
<?xml version="1.0" encoding="UTF-8"?>
<beans ... >
It could cause the Spring context to misinterpret your definitions.
3. XML Schema Mismatches
Ensure that the XML schema locations are correct. A mismatch here can lead to parsing errors or result in unexpected behavior. Here’s a reminder of the correct schema location:
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd"
Leveraging Profiles for Testing
Using Spring Profiles isn’t just limited to different environments. They can also be beneficial in unit tests and integration tests. For instance, you can use the @ActiveProfiles
annotation in your test classes to specify which profile should be active while the tests are running.
However, in XML configurations, ensure that your test contexts are correctly set up to read the right profile.
Example: Using Profiles in Tests
Here’s how you might set up a test configuration:
<beans profile="test">
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="org.h2.Driver"/>
<property name="url" value="jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE"/>
<property name="username" value="sa"/>
<property name="password" value=""/>
</bean>
</beans>
In your test class, you then activate it:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:applicationContext.xml")
@ActiveProfiles("test")
public class MyServiceTest {
// Test code here
}
Tips for Effective Troubleshooting
-
Logs and Debugging: Always enable debug-level logging for your Spring application. This will give you insights into which beans are being loaded and from which profiles.
-
Documentation and Community: Don't hesitate to refer to Spring's official documentation for profiles or reach out to community forums for help.
-
Simplify Configuration: If you are facing persistent issues, try simplifying your configuration by isolating problems. Start with a minimal XML file and gradually add components to identify the problematic areas.
In Conclusion, Here is What Matters
Mastering Spring Profiles can greatly enhance the modularity and efficiency of your application’s configuration management. While XML configurations might seem cumbersome compared to Java-based configurations, they offer a clear structure that can be beneficial for certain projects.
By understanding common pitfalls and your way around them, you can leverage Spring Profiles to create robust applications that handle various environments with ease. Remember to keep the basics such as profile activation, bean definition, and XML syntax in check.
Embrace this powerful feature, and you will quickly become adept at managing complex configurations in Spring applications.
For further reading, you might find these resources helpful:
- Spring Profiles Guide
- Spring XML Configuration
By following these principles, you'll not only troubleshoot specific XML issues but also gain a broader understanding of working with Spring Profiles in your applications. Happy coding!
Checkout our other articles