Mastering the On/Off Toggle: Tips for Android Users
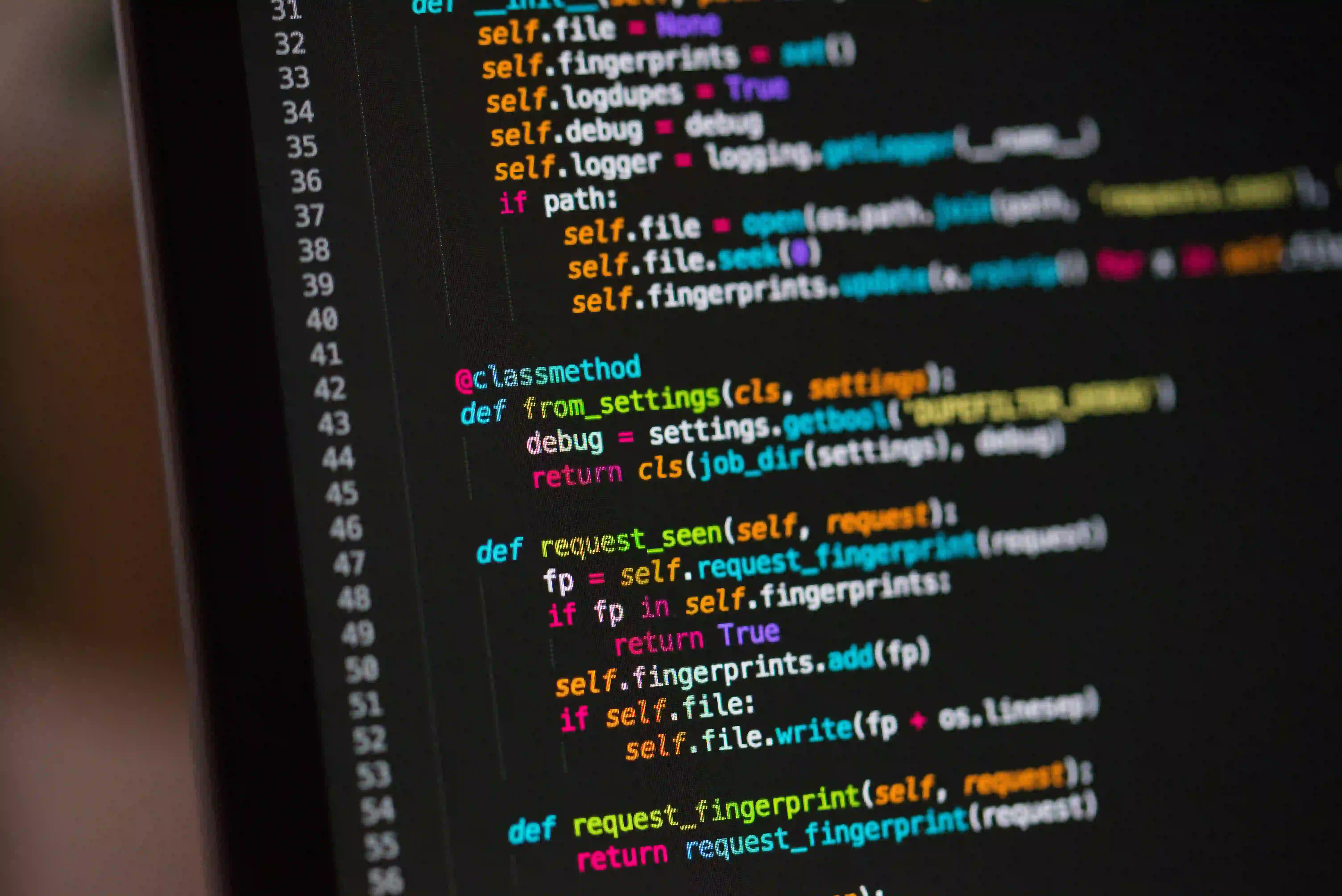
Mastering the On/Off Toggle: Tips for Android Users
In the digital realm, user interface elements play a fundamental role in providing seamless interactions. One such crucial element is the toggle switch. Whether you're controlling haptic feedback, managing notifications, or handling app permissions, mastering the on/off toggle can significantly enhance your Android user experience. In this post, we will explore various aspects of the on/off toggle, offering tips, best practices, and concrete code examples to empower both users and developers.
Understanding the On/Off Toggle
The on/off toggle is a simple yet powerful UI element that allows users to enable or disable a feature with a single touch. This binary state can provide instant feedback, which is essential for user satisfaction. In Android, toggles are adeptly represented by the Switch
widget.
Where are Toggles Used?
- Settings Menu: Adjusting various device features.
- App Permissions: Managing what features an app can access.
- Notifications: Turning on/off notifications for specific applications.
These toggles can streamline user interactions and lead to increased efficiency.
Best Practices for Using Toggle Switches
When implementing toggles in your Android application, consider the following best practices to ensure a smooth user experience.
1. Use Clear Labels
Ensure your toggle switches have clear and concise labels. Users should understand what feature they are enabling or disabling at a glance.
<Switch
android:id="@+id/switch_example"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enable Feature" />
2. Provide Contextual Information
For complex features, provide a brief description or instructions. A tool-tip or an accompanying help icon can aid user comprehension.
3. Visual Feedback
Ensure toggles give immediate visual feedback. When a user toggles a switch, it should reflect its new state instantly.
4. Default States
Carefully consider the default state of your toggles. For features that impact security or data usage, the default should typically be set to "off".
5. Use Animation
A subtle animation can provide a smoother transition when toggling between states. This can enhance user satisfaction.
Implementing Toggle Switches in Android
Let's take a closer look at implementing toggle switches in your Android application. Below is a straightforward example of how to do this using Kotlin's syntax.
Step 1: Build Your Layout
First, create a layout file (e.g., activity_main.xml
) with the toggle switch.
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Notifications" />
<Switch
android:id="@+id/switch_notifications"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enable Notifications" />
</LinearLayout>
Step 2: Add Functionality in Your Activity
Now, let’s wire up our toggle switch so that it performs an action when toggled.
class MainActivity : AppCompatActivity() {
private lateinit var notificationsSwitch: Switch
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
notificationsSwitch = findViewById(R.id.switch_notifications)
// Set an initial state
notificationsSwitch.isChecked = false
notificationsSwitch.setOnCheckedChangeListener { buttonView, isChecked ->
if (isChecked) {
enableNotifications()
} else {
disableNotifications()
}
}
}
private fun enableNotifications() {
// Logic to enable notifications
Toast.makeText(this, "Notifications Enabled", Toast.LENGTH_SHORT).show()
}
private fun disableNotifications() {
// Logic to disable notifications
Toast.makeText(this, "Notifications Disabled", Toast.LENGTH_SHORT).show()
}
}
Commentary on the Code
- User Feedback: The Toast messages give immediate feedback to the user, confirming their actions.
- State Management: The
isChecked
property is used to allow for a default off state, which is an essential aspect of managing user expectations.
Step 3: Testing
It is crucial to test your toggles rigorously. Make sure actions occur as expected, regardless of device type or Android version.
Common Use-Cases for Toggles
-
Dark Mode: Users greatly appreciate the option to switch between dark and light themes. Using a toggle can make this feature easily accessible.
-
Location Services: Users often need to enable or disable location services based on context. A toggle is an ideal solution.
-
Data Saver Options: For users keen on conserving data, a toggle can help to control background data usage from applications.
Accessibility Considerations
Making your toggle switches accessible is a critical step in app development.
- TalkBack: Ensure that toggle switches have content descriptions, so they are readable by screen readers.
- Color Contrast: Make sure the toggle’s colors are distinct enough to be easily recognized by users with visual impairments.
Here’s how to add a content description to the toggle:
<Switch
android:id="@+id/switch_notifications"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enable Notifications"
android:contentDescription="Toggle to enable or disable notifications" />
A Final Look
Mastering the on/off toggle is essential for both Android users and developers. Properly implemented, toggles can significantly enhance user experience by allowing for quick, intuitive changes in application settings.
By following best practices in design and implementation, such as using clear labels, providing contextual information, and ensuring accessibility, you can create an application that caters to a broader audience.
For more insightful articles on Android UI best practices, do check out Android Developers and Material Design Guidelines.
With these tips in hand, you are now well-equipped to leverage the on/off toggle in enhancing user experience for your Android applications. Happy coding!