Understanding Hibernate Flush Strategies: Common Pitfalls Explained
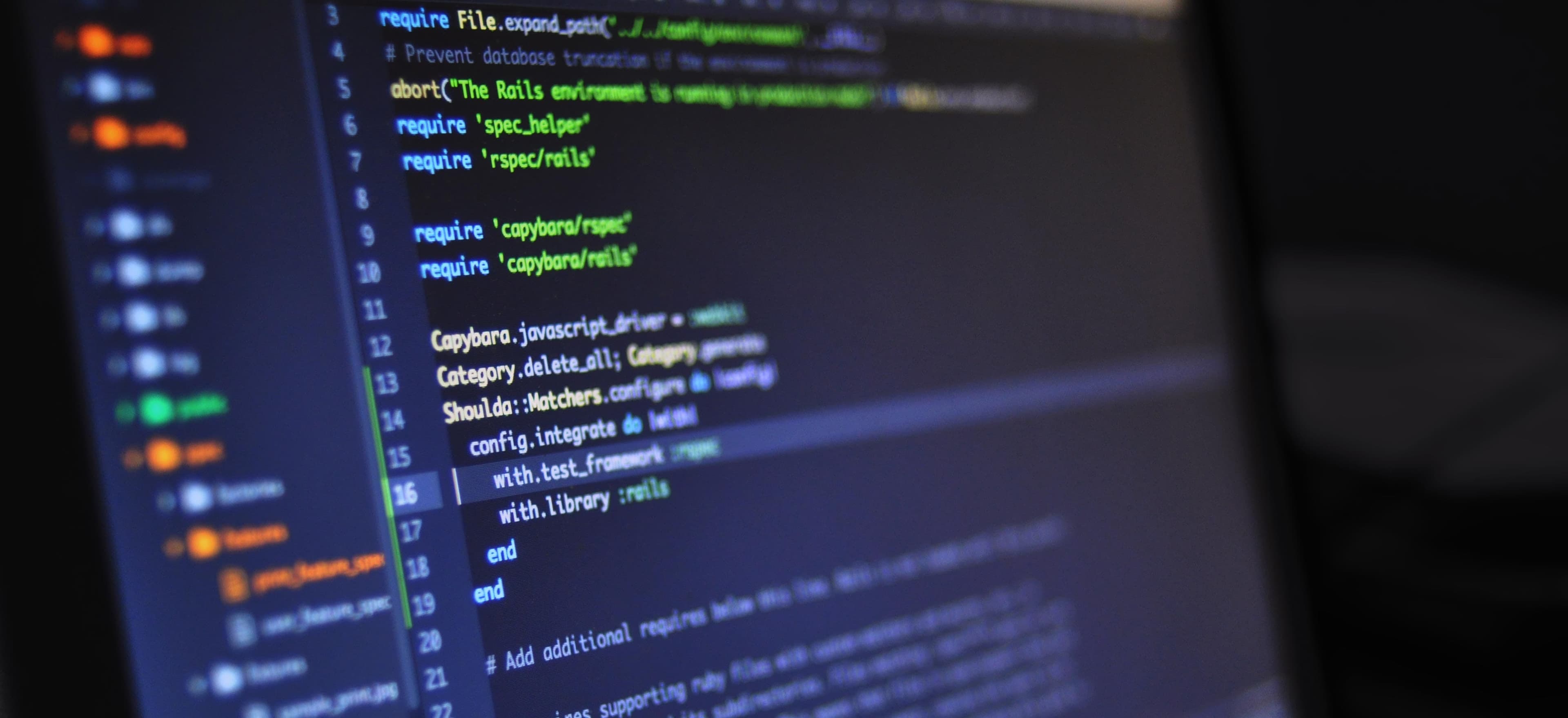
- Published on
Understanding Hibernate Flush Strategies: Common Pitfalls Explained
Hibernate serves as one of the leading frameworks for Object-Relational Mapping (ORM) in Java applications. One of its more nuanced areas, often fraught with confusion, is the concept of flush strategies. In this article, we'll delve into the various flush strategies provided by Hibernate, their common pitfalls, and best practices to avoid those pitfalls.
What is Hibernate Flush?
Before we dive into flush strategies, let’s clarify what flushing actually means in Hibernate. Flushing is the process through which Hibernate synchronizes its in-memory state (the Hibernate Session) with the database. In simpler terms, it ensures that any unsaved changes to your entities are written to the database.
Flushing can be triggered automatically before a query is executed or manually by calling the flush()
method on the Session
object. Understanding how this process works allows for better control over your transaction's performance and integrity.
Hibernate Flush Strategies
Hibernate offers several flush strategies for managing flush behavior:
- AUTO
- COMMIT
- MANUAL
Let’s take a closer look at each of these strategies.
AUTO Flush Mode
In AUTO
flush mode, Hibernate automatically flushes the session before executing a query. This ensures that any changes made in the current transaction are reflected in the database.
Code Example:
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
// Setting flush mode to AUTO (default behavior)
session.setFlushMode(FlushMode.AUTO);
// Making changes to an entity
User user = new User();
user.setName("John Doe");
session.save(user);
// The session will flush automatically before the next query
List<User> users = session.createQuery("from User", User.class).list();
transaction.commit();
session.close();
Pitfall:
Relying solely on AUTO
mode can lead to performance issues, particularly in large transactions. Since it flushes automatically before each query, you might perform unnecessary database writes.
COMMIT Flush Mode
With COMMIT
flush mode, Hibernate flushes the session only when the transaction is committed. This allows you to execute multiple operations without immediately writing to the database.
Code Example:
session.setFlushMode(FlushMode.COMMIT);
// Different operations can be done here before the commit
User user1 = new User();
user1.setName("Alice");
session.save(user1);
User user2 = new User();
user2.setName("Bob");
session.save(user2);
// No flush occurs until commit is called
transaction.commit(); // Flush occurs here
Pitfall:
While this mode is beneficial for performance, developers may forget to manually flush the session when needed. If the session is not flushed before a read operation that requires up-to-date data, you may get stale data.
MANUAL Flush Mode
MANUAL
flush mode requires you to invoke the flush()
method explicitly whenever you need to synchronize the session state with the database.
Code Example:
session.setFlushMode(FlushMode.MANUAL);
// Perform multiple operations
User user3 = new User();
user3.setName("Charlie");
session.save(user3);
// Explicitly flush when needed
session.flush(); // Only flush when it's absolutely necessary
Pitfall:
While MANUAL
mode gives you tight control, it can lead to potential data integrity issues if you forget to flush the session at the right time. Always document when and where you manually call flush to ensure that all team members are aware.
Best Practices for Managing Flush Strategies
-
Evaluate Your Use Case: When designing your application, evaluate whether you actually need automatic flushing or if a manual trigger suffices. For performance-critical applications, consider using COMMIT or MANUAL flush modes.
-
Avoid Frequent Flushes: If your application frequently flushes the session during operations, it may lead to increased load on the database. Consider batching operations to minimize the number of flush calls.
-
Use Session Clear: Sometimes, your session can get filled with many entities. Use
session.clear()
to detach all entities and avoid potential memory issues. -
Testing and Monitoring: Always test your application thoroughly. Use monitoring tools to track how often flush operations occur and their impact on performance.
-
Consult Documentation: The official Hibernate documentation contains valuable information on best practices and deeper explanations of flush strategies. Use it as a reference point.
A Final Look
Understanding Hibernate flush strategies is crucial for achieving optimal database performance and maintaining data integrity. Each flush mode – AUTO, COMMIT, and MANUAL – offers its benefits and potential pitfalls. By being aware of these nuances, developers can choose the right flush strategy that best suits their application's needs.
By applying best practices and being cautious with automatic and manual flushing, you can enhance the efficiency and performance of your Java applications that rely on Hibernate for ORM.
Additional Resources
In summary, whether you’re a seasoned Hibernate user or just starting out, mastering flush strategies will significantly elevate your Java development skills. Be mindful of the implications of each strategy and always strive to write efficient, maintainable code. Happy coding!