Boost Java Performance: Fixing Common Code Inefficiencies
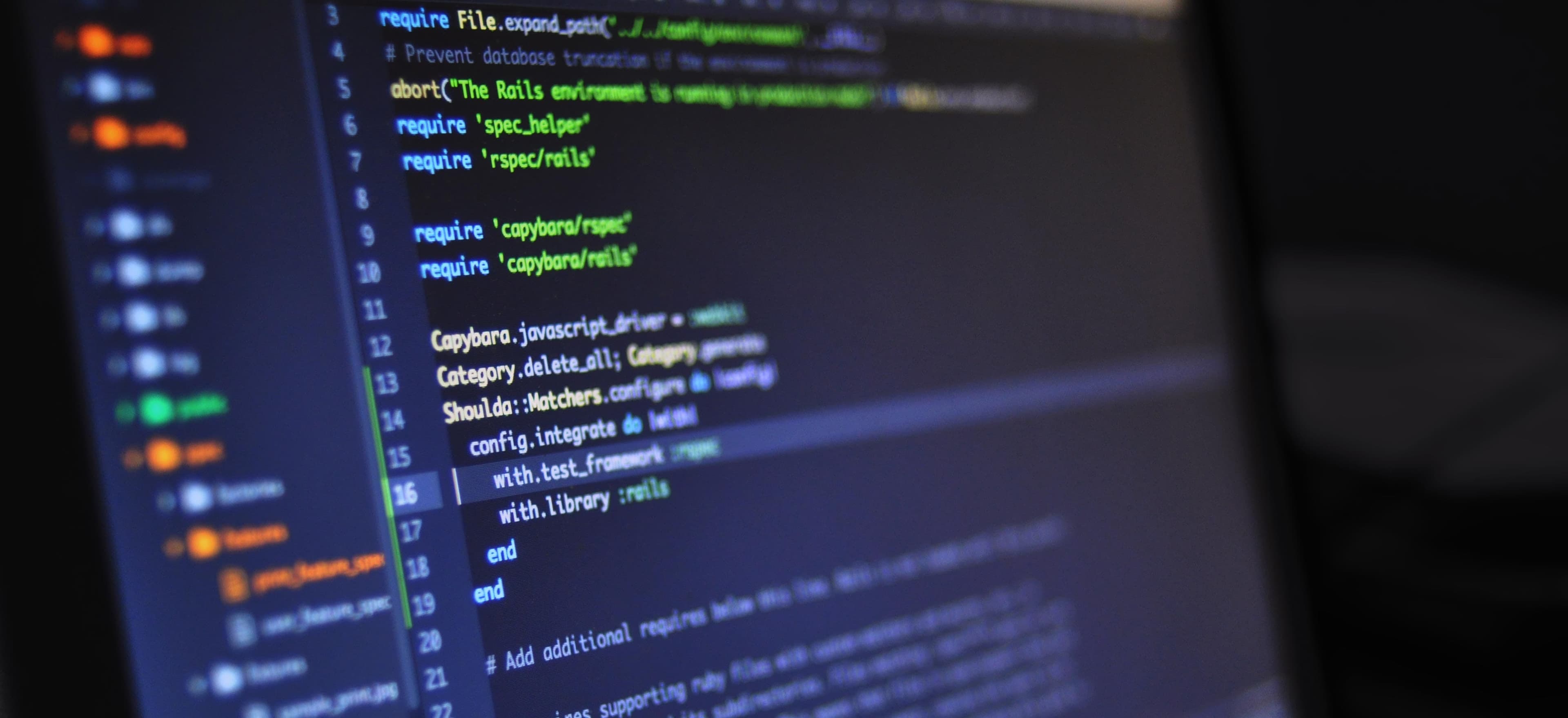
- Published on
Boost Java Performance: Fixing Common Code Inefficiencies
Java is a powerful programming language that is widely used for building robust applications across various platforms. However, like any other programming language, Java code can become inefficient, leading to performance issues. This blog post highlights common code inefficiencies in Java and provides actionable fixes that can enhance performance. By addressing these inefficiencies, developers can ensure their applications run faster and more smoothly.
Table of Contents
- Understanding Java Performance
- Identifying Common Code Inefficiencies
- Profiling and Optimization Techniques
- Conclusion
Understanding Java Performance
In Java, performance can significantly impact user experience. Slow applications can frustrate users, lead to lost revenue, and damage a company's reputation. Therefore, identifying and resolving performance issues is crucial for any developer. Before diving into common code inefficiencies, it’s essential to comprehend the Key Performance Indicators (KPIs) that matter, such as:
- Response Time: The time it takes for a system to respond to a user's request.
- Throughput: The number of requests that a system can handle in a given time period.
Identifying Common Code Inefficiencies
1. Unused Objects and Memory Leaks
Java uses garbage collection to manage memory automatically. However, applications can still suffer from memory leaks if references to objects aren't cleared. An increased memory footprint can cause performance degradation due to extended garbage collection pauses.
Fixing Memory Leaks:
- Weak References: Use
WeakReference
for objects that can be collected if needed.
import java.lang.ref.WeakReference;
// Using WeakReference to avoid memory leaks
class Cache {
private WeakReference<HeavyObject> weakObj;
public void setHeavyObj(HeavyObject obj) {
this.weakObj = new WeakReference<>(obj);
}
public HeavyObject getHeavyObj() {
return weakObj.get();
}
}
Using WeakReference
, we avoid keeping objects alive longer than necessary, allowing the garbage collector to reclaim memory.
2. Inefficient Collections Usage
The choice of collection in Java can greatly impact performance. For instance, using an ArrayList
where a HashSet
is more appropriate can lead to inefficient lookups.
Using the Right Collection:
HashMap
for key-value pairs with fast lookups.ArrayList
for ordered lists with indexed access.HashSet
for storing unique values and checking for their existence quickly.
Example of Efficient Collection Implementation:
import java.util.HashSet;
public class UniqueNames {
private HashSet<String> names;
public UniqueNames() {
names = new HashSet<>();
}
public void addName(String name) {
// Adding a name with O(1) complexity on average
names.add(name);
}
public boolean hasName(String name) {
// Checking existence with O(1) complexity
return names.contains(name);
}
}
This code snippet demonstrates a HashSet
that provides fast lookups and efficient storage of unique names.
3. String Operations Pitfalls
Java Strings are immutable. Every time a string is altered, a new string is created, which can lead to performance issues during heavy string manipulations.
Optimizing String Operations:
- Use
StringBuilder
for concatenation operations.
public class NameList {
public String buildNamesList(String[] names) {
StringBuilder sb = new StringBuilder();
for (String name : names) {
sb.append(name).append(", "); // Efficiently appending strings
}
// Remove the last comma
if (sb.length() > 0) {
sb.setLength(sb.length() - 2); // Trimming the last comma
}
return sb.toString();
}
}
By utilizing StringBuilder
, we reduce the overhead of mutating strings and improve performance during concatenation tasks.
4. Avoiding Premature Optimization
While optimizing is crucial, prioritizing code readability and maintainability is equally important. Sometimes, what seems inefficient at first glance may not significantly affect performance in practical cases, or might even lead to more complex code.
Best Practices:
- Optimize based on profiling results. Identify bottlenecks through tools like Java VisualVM before making changes.
- Always prioritize comprehensibility. Code that’s difficult to read can become a maintenance burden.
Profiling and Optimization Techniques
Before applying any optimization techniques, it's wise first to profile the application. Tools like:
- Java Mission Control
- YourKit
These tools help identify memory usage, CPU load, and method execution times, enabling precise optimizations.
General Optimization Techniques
- Batch Processing: Group database updates to minimize transaction overhead.
- Database Connection Pooling: Optimizing connections can significantly enhance response time.
- Caching: Store frequently accessed data in memory to reduce retrieval times.
To Wrap Things Up
Performance in Java applications is crucial and can significantly influence user satisfaction. Understanding and addressing common code inefficiencies such as memory leaks, inefficient collections, string operations, and avoiding premature optimizations can lead to a remarkable improvement in your application’s performance.
Being methodical—profiling your code before optimization, using appropriate collections, and leveraging powerful tools—will streamline the detecting processes, ensuring a smoother experience for both developers and users.
By embedding these practices into your development routine, you not only boost performance but also contribute to the creation of maintainable and efficient applications. Remember, the goal is not just to write code that works, but to write code that works well.
Checkout our other articles