Why Scala's Lambda Syntax Can Confuse Java 8 Devs
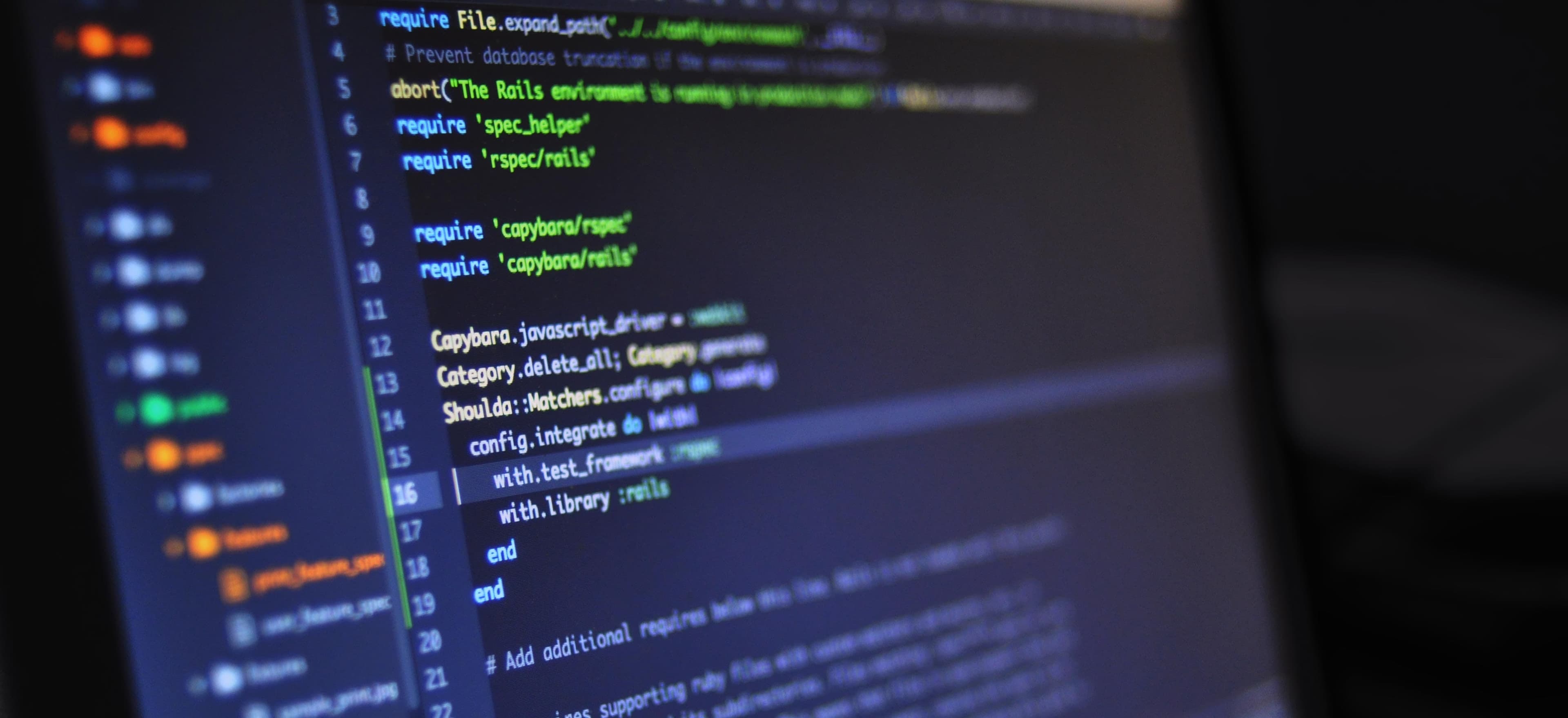
- Published on
Why Scala's Lambda Syntax Can Confuse Java 8 Devs
With the advent of functional programming in Java 8, developers have increasingly adopted a more functional style of coding. However, as they venture beyond Java into other languages that embrace functional programming, such as Scala, they often find themselves facing a different set of syntax rules and conventions. In this blog post, we'll explore the intricacies of Scala's lambda syntax and why it can confuse Java 8 developers.
The Rise of Functional Programming
First, let’s delve briefly into functional programming and its significance. Functional programming emphasizes the use of functions as first-class citizens, allowing them to be passed as arguments, returned from other functions, and assigned to variables. Java 8 introduced several enhancements, such as:
- Lambda Expressions: A concise way to express instances of single-method interfaces (functional interfaces).
- Streams API: A powerful abstraction for processing sequences of elements.
Here’s a simple example of a lambda expression in Java:
// Java 8 Lambda Expression
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
The Scala Approach
Scala also supports lambda expressions, but they come with a unique syntax that may seem foreign to those seasoned in Java. Here’s the equivalent code in Scala:
// Scala Lambda Expression
val names = List("Alice", "Bob", "Charlie")
names.foreach(name => println(name))
Key Differences in Syntax
-
Arrow Functions: In Scala, lambdas are often represented using
=>
, which separates the parameters from the body of the function. While Java uses->
for the same purpose, the shift in the character (from-
to=
) can be jarring for Java developers. -
Type Inference: In Scala, it is common to omit type declarations due to its powerful type inference capabilities. For example:
// Type Inference in Scala
val square = (x: Int) => x * x
In contrast, Java requires explicit type declarations for lambda parameters when they are not inferred from the context.
- Single-Parameter Functions: Scala enables a simpler syntax for single-parameter functions where the parentheses can be omitted. For instance:
// Scala without parentheses
val greet = name => s"Hello, $name!"
In Java, you always need to encapsulate the parameters in parentheses.
- Function Values: Scala treats functions as first-class citizens but also allows you to define functions that return other functions, which can lead to more complex, nested lambda expressions. Here’s a simple example:
// Function that returns another function
def adder(x: Int): Int => Int = {
(y: Int) => x + y
}
val addTwo = adder(2)
println(addTwo(5)) // Outputs: 7
Why This Confusion Occurs
1. Shift in Design Patterns
Java developers are accustomed to an imperative style, which centers around class-based object-oriented programming. Scala, while allowing for OOP, strongly encourages a functional paradigm, leading to a potential mismatch in thinking patterns.
2. Code Readability
While both languages aim for readability, Scala’s more concise syntax can lead to overhead in understanding for Java developers. The reduction in verbosity can sometimes obscure meaning, particularly in complex functions or nested lambdas.
3. Emphasis on Immutability
In Scala, immutability is preferred. Java 8 introduced streams, which are often used with immutable data structures, but developers might not be as accustomed to treating every variable as immutable until explicitly stated.
4. Functional Structure
Java’s introduction of functional programming constructs is layered on top of its existing structure. On the other hand, Scala combines functional programming with a blend of OOP, resulting in diverse styles that may be overwhelming for newcomers.
Examples of Common Confusions
Let’s examine typical scenarios where Java developers may stumble while working with Scala:
1. Higher-Order Functions
Java does support higher-order functions through functional interfaces, but it does not handle them as gracefully as Scala.
// Scala higher-order function
def applyFunction(f: Int => Int, value: Int) = f(value)
val increment = (x: Int) => x + 1
println(applyFunction(increment, 5)) // Outputs: 6
In Java, this would require explicit interfaces, leading to more boilerplate code.
2. Currying
Scala supports currying, a technique of transforming a function with multiple parameters into a series of single-parameter functions. This is less intuitive for Java developers:
// Curried Function in Scala
def curriedAdd(x: Int)(y: Int) = x + y
val addTwo = curriedAdd(2) _ // partially applied
println(addTwo(3)) // Outputs: 5
In Java, achieving a similar effect would require a more complicated structure using class or interface hierarchies.
3. Collections Transformation
Scala’s collections API is more powerful and integrated with functional transformations:
// Map Function in Scala
val numbers = List(1, 2, 3)
val squares = numbers.map(x => x * x)
println(squares) // Outputs: List(1, 4, 9)
In Java, achieving the same would look like this:
// Map Function in Java
List<Integer> numbers = Arrays.asList(1, 2, 3);
List<Integer> squares = numbers.stream()
.map(x -> x * x)
.collect(Collectors.toList());
System.out.println(squares); // Outputs: [1, 4, 9]
4. Implicit Parameters
Scala has the unique feature of implicit parameters, a construct not available in Java.
// Implicit Parameters in Scala
def greet(implicit name: String) = s"Hello, $name"
implicit val myName: String = "Alice"
println(greet) // Outputs: Hello, Alice
In Java, such a feature would require explicit context handling.
Bringing It All Together
Transitioning from Java 8 to Scala can prove to be both rewarding and confusing for developers. The differences in lambda syntax, type inference, immutability, and functional programming paradigms require a paradigm shift in thinking.
To master Scala, Java developers should immerse themselves in Scala's unique features, practice writing Scala code, and understand the underlying principles of functional programming.
If you're looking to gain a deeper understanding of functional programming or Scala’s unique offerings, consider checking out Scala Documentation or Functional Programming in Scala.
By taking the time to explore these differences, Java developers can leverage the full power of Scala and embrace the beauty of functional programming. So, dive in, and happy coding!
Checkout our other articles