Understanding Java's Role in Historical Data Analysis
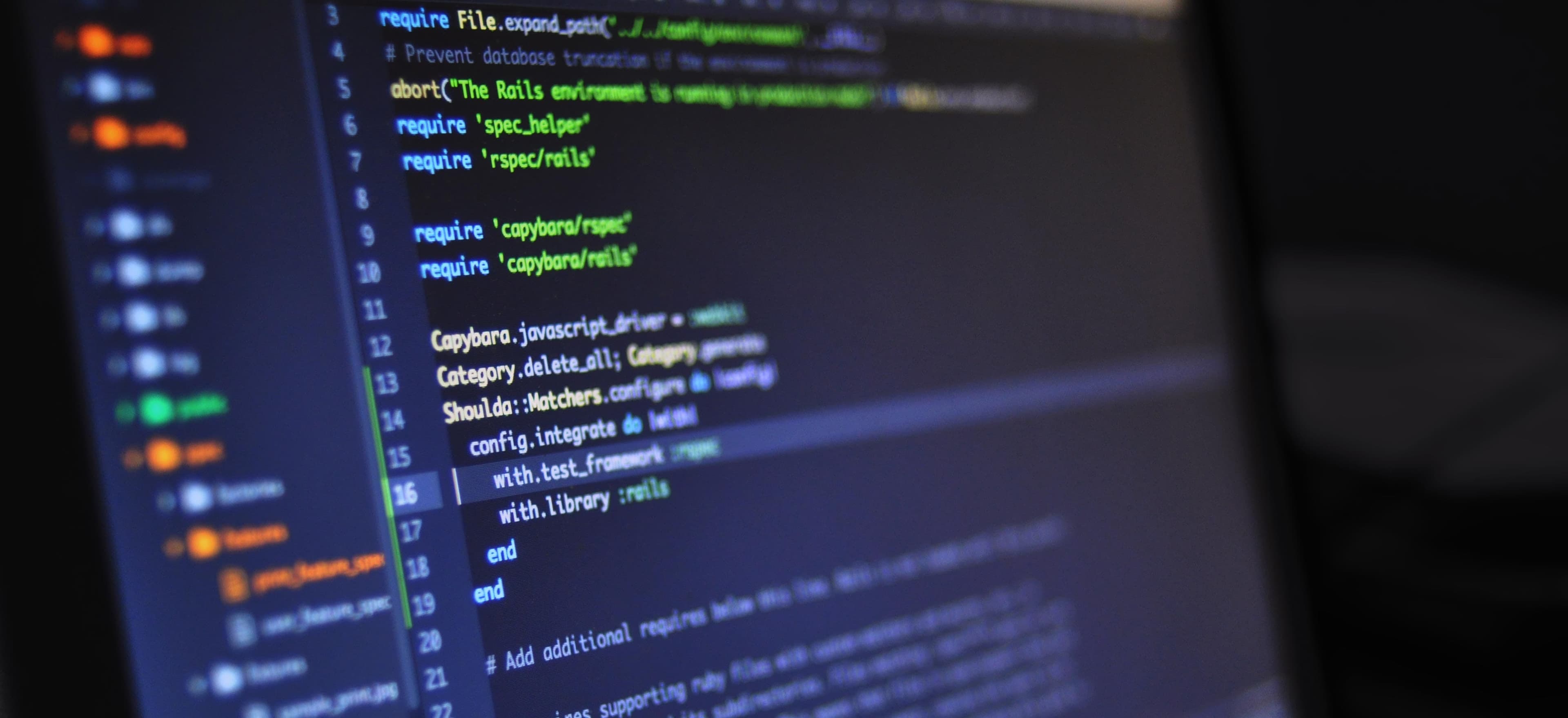
- Published on
Understanding Java's Role in Historical Data Analysis
In the age of big data, historical analysis has become a crucial aspect of various fields, ranging from economics to social sciences. One programming language that has stood the test of time and remains popular among data analysts is Java. This article will explore Java's role in historical data analysis, evaluate its advantages, and showcase some practical examples with code snippets to illustrate its usage.
Why Java for Data Analysis?
Java is a versatile and robust programming language widely used in enterprise-level applications. Its stability and scalability make it a suitable option for handling large datasets, especially when analyzing historical data. Here are some reasons why Java stands out in this area:
-
Performance: Java is known for its high performance, thanks in part to its Just-In-Time (JIT) compiler, which translates bytecode into native machine code for execution.
-
Robust Libraries: Java boasts an extensive collection of libraries and frameworks tailored for data processing and analysis, such as Apache Hadoop for distributed computing and Apache Spark for data processing.
-
Object-Oriented: Java’s object-oriented principles promote code reusability, making it easy to maintain and extend analysis systems.
-
Platform Independence: Java's "write once, run anywhere" (WORA) capability allows your applications to run on any system that has a Java Virtual Machine (JVM), enhancing accessibility.
-
Community Support: With a vast user community, developers can easily find solutions, libraries, and tools.
The Role of Java in Historical Data Analysis
When it comes to historical data, we often deal with datasets that span multiple years or decades. This data can include economic trends, population statistics, and even social phenomena. Analyzing this type of data using Java involves several steps:
Data Collection
The first step is to collect historical data. You can leverage Java's networking capabilities to fetch data from various sources, including APIs.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class DataFetcher {
public String fetchData(String urlString) throws Exception {
URL url = new URL(urlString);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder content = new StringBuilder();
String line;
while ((line = in.readLine()) != null) {
content.append(line).append("\n");
}
in.close();
connection.disconnect();
return content.toString();
}
}
Analyzing the Data
Once the data is collected, the next step is analysis. You can use libraries such as Apache Commons Math or JFreeChart for statistical analysis and visualization.
For example, let's calculate the average value of a dataset. Here is a snippet using the Apache Commons Math library:
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
public class DataAnalyzer {
public double calculateAverage(double[] data) {
DescriptiveStatistics stats = new DescriptiveStatistics();
for (double value : data) {
stats.addValue(value);
}
return stats.getMean();
}
}
Data Visualization
Visual representation of data often provides better insights and easier understanding. In Java, JFreeChart can be employed to create various types of charts. Here's an example of how you can create a simple line chart:
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.xy.XYSeries;
import org.jfree.data.xy.XYSeriesCollection;
import javax.swing.JFrame;
public class LineChart {
public void createChart(String title, double[] xData, double[] yData) {
XYSeries series = new XYSeries("Historical Data");
for (int i = 0; i < xData.length; i++) {
series.add(xData[i], yData[i]);
}
XYSeriesCollection dataset = new XYSeriesCollection(series);
JFreeChart chart = ChartFactory.createXYLineChart(title, "Year", "Value", dataset);
JFrame frame = new JFrame();
frame.setContentPane(new ChartPanel(chart));
frame.pack();
frame.setVisible(true);
}
}
Each of the above segments is crucial in performing a complete historical data analysis, from fetching the data to visualizing it.
A Real-World Example: Analyzing Historical Economic Data
Suppose you want to analyze the economic growth of Germany from 1871 to 1914. You could incorporate data from the article "Aufstieg und Fall: Das Deutsche Reich (1871-1914)" found at lernflux.com/post/aufstieg-und-fall-deutsches-reich-1871-1914. This period is significant for understanding Germany's industrialization and economic expansion.
Step 1: Fetching Data
You might fetch GDP data over the years, perhaps from an API that provides historical economic data.
Step 2: Analyzing and Visualizing Data
You could calculate key metrics using the aforementioned methods, such as calculating the average GDP growth rate or analyzing other economic factors like unemployment rates.
In summary, after you have collected and processed the data, you can generate visuals to present the findings clearly.
My Closing Thoughts on the Matter
Java is more than just a programming language; it’s a powerful tool for historical data analysis. Its performance, extensive library support, and community backing make it an ideal choice for tackling the challenges associated with large datasets. By following the steps outlined in this article, including data collection, analysis, and visualization, you can derive meaningful insights from historical data.
Whether in academic settings or industry applications, understanding and leveraging Java for data analysis can provide significant advantages. As this technology continues to evolve, Java remains a relevant and practical solution for any data analyst looking to make sense of historical data.
By mastering Java's capabilities, you will not only enhance your technical skills but also contribute to a deeper understanding of historical contexts and trends through data analysis. Happy coding!
Checkout our other articles