Prevent Java Memory Leaks: Key Strategies for Developers
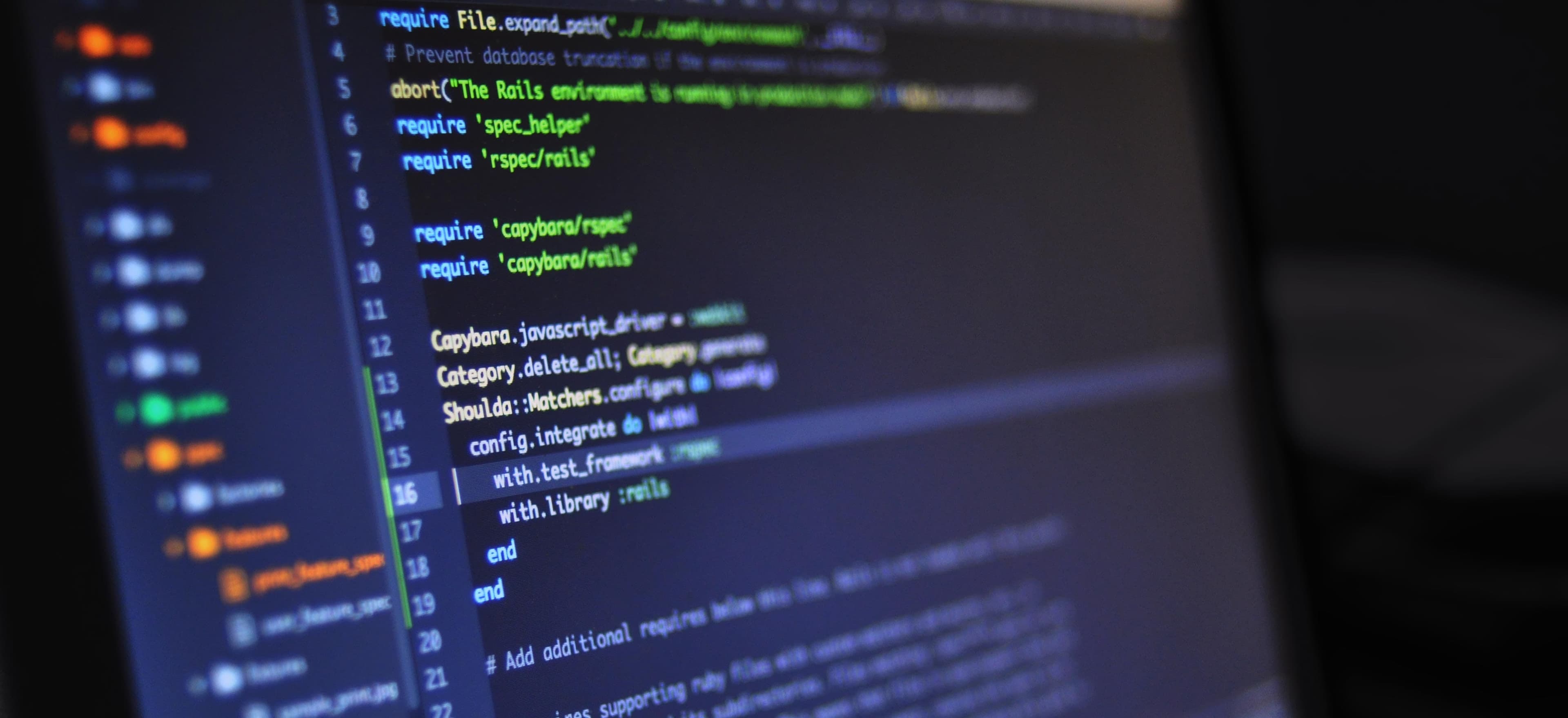
- Published on
Prevent Java Memory Leaks: Key Strategies for Developers
Memory management in Java is often considered a blessing and a curse. While Java developers enjoy the convenience of automatic garbage collection, it does not completely absolve them from the responsibility of managing memory effectively. When memory is not handled properly, it can lead to memory leaks, which can degrade application performance and lead to unexpected crashes. In this article, we will explore key strategies for preventing memory leaks in Java applications, providing you with insights and practical examples.
Understanding Memory Leaks in Java
A memory leak in Java occurs when objects are no longer needed but remain in memory due to lingering references. The Java Virtual Machine (JVM) cannot reclaim this memory, leading to increased memory usage over time. This can be particularly problematic in long-running applications, such as web servers or enterprise applications.
It's crucial to understand that while the garbage collector automatically reclaims memory for unused objects, it only does so for objects that are no longer reachable from the root set (the set of references that the JVM considers active). Therefore, if references to a no longer needed object persist, those objects will not be collected, resulting in a leak.
Common Causes of Memory Leaks
Before we dive into the strategies for preventing memory leaks, let's identify the common causes:
- Static Fields: Objects referenced by static fields persist as long as the class is loaded.
- Unintentional Listener Registrations: Event listeners that are not deregistered can hold references to objects and prevent them from being garbage collected.
- Collections: Improper use of collections can lead to unbounded growth.
- Thread Local Variables: These can lead to leaks if not removed correctly.
- Long-Lived Objects: Objects that live longer than intended can carry references to other objects.
Key Strategies for Preventing Memory Leaks
1. Avoid Unintentional Static References
Static fields can lead to memory leaks if you unintentionally hold onto references that should otherwise be discarded. For example:
public class MemoryLeak {
private static List<Object> leaks = new ArrayList<>();
public static void addLeak(Object obj) {
leaks.add(obj); // This will prevent obj from being garbage collected
}
}
In this code snippet, any object added to the static list leaks
will never be collected, even if it is no longer needed.
Solution: Use instance variables instead and ensure to nullify references when they are no longer needed.
2. Properly Deregister Listeners
Consider the following scenario with an event listener:
public class EventSource {
private List<EventListener> listeners = new ArrayList<>();
public void registerListener(EventListener listener) {
listeners.add(listener);
}
public void fireEvent() {
for (EventListener listener : listeners) {
listener.onEvent();
}
}
}
public class DataProcessor implements EventListener {
public void onEvent() {
// Handle event
}
}
In this example, if DataProcessor
objects are registered as listeners but never deregistered, they remain in memory.
Solution: Implement an appropriate deregistration mechanism such as:
public void unregisterListener(EventListener listener) {
listeners.remove(listener);
}
3. Use Weak References
For situations where you need to hold a reference to an object without preventing its garbage collection, consider using weak references. The java.lang.ref
package provides the WeakReference
class, which allows the referenced object to be collected by the garbage collector when no other strong references exist.
import java.lang.ref.WeakReference;
public class Cache {
private WeakReference<Object> cacheEntry;
public void cacheObject(Object obj) {
cacheEntry = new WeakReference<>(obj);
}
public Object getCachedObject() {
return cacheEntry.get(); // Returns null if the object has been GC'd
}
}
In this case, if cacheEntry
is the only reference to the cached object, it will be garbage collected when needed.
4. Monitor the Use of Collections
Collections can grow indefinitely if elements are continuously added and never removed. For example:
List<String> strings = new ArrayList<>();
public void addString(String str) {
strings.add(str); // This can lead to memory leaks if not managed properly
}
Solution: Either clear the list when done, or limit its size.
A common pattern with limiting size is as follows:
public void addString(String str) {
if (strings.size() >= MAX_SIZE) {
strings.remove(0); // Remove oldest entry
}
strings.add(str);
}
5. Be Cautious with Thread Local Variables
Thread local variables can inadvertently lead to memory leaks if they are not cleaned up properly. Always remember to remove values from ThreadLocal
when they are no longer needed.
public class ContextHolder {
private static ThreadLocal<Object> context = ThreadLocal.withInitial(() -> new Object());
public static void clear() {
context.remove(); // Ensure you clear the thread-local variable
}
}
Failure to invoke clear()
can lead to memory leaks in the context of long-lived threads.
Profiling and Monitoring
No preventive measure can save you if you don’t know there’s a problem. Profiling tools like VisualVM and Eclipse Memory Analyzer can help identify memory leaks. They analyze heap dumps and can point to the suspected objects that are being retained in memory unexpectedly.
For more details on related memory management concerns, check out the article "Conquer NodeJS Memory Leaks: Strategies and Solutions". While it focuses on NodeJS, many concepts can be adapted to Java, showing the universal importance of understanding memory handling in programming.
To Wrap Things Up
Preventing memory leaks in Java requires a proactive approach. By understanding the behaviors of object references in Java and how they interact with garbage collection, developers can effectively manage memory usage. Implementing strategies like avoiding static references, properly deregistering listeners, using weak references, monitoring collections, and managing thread local variables can significantly reduce the chance of memory leaks. Always remember to profile and monitor your applications to catch potential leaks before they become problematic.
These practices will not only bolster your application’s performance but will also enhance the overall user experience by ensuring that your Java applications are responsive and efficient. Stay vigilant, and happy coding!
Checkout our other articles