Java Memory Management: Tackle Memory Leaks in Your Apps
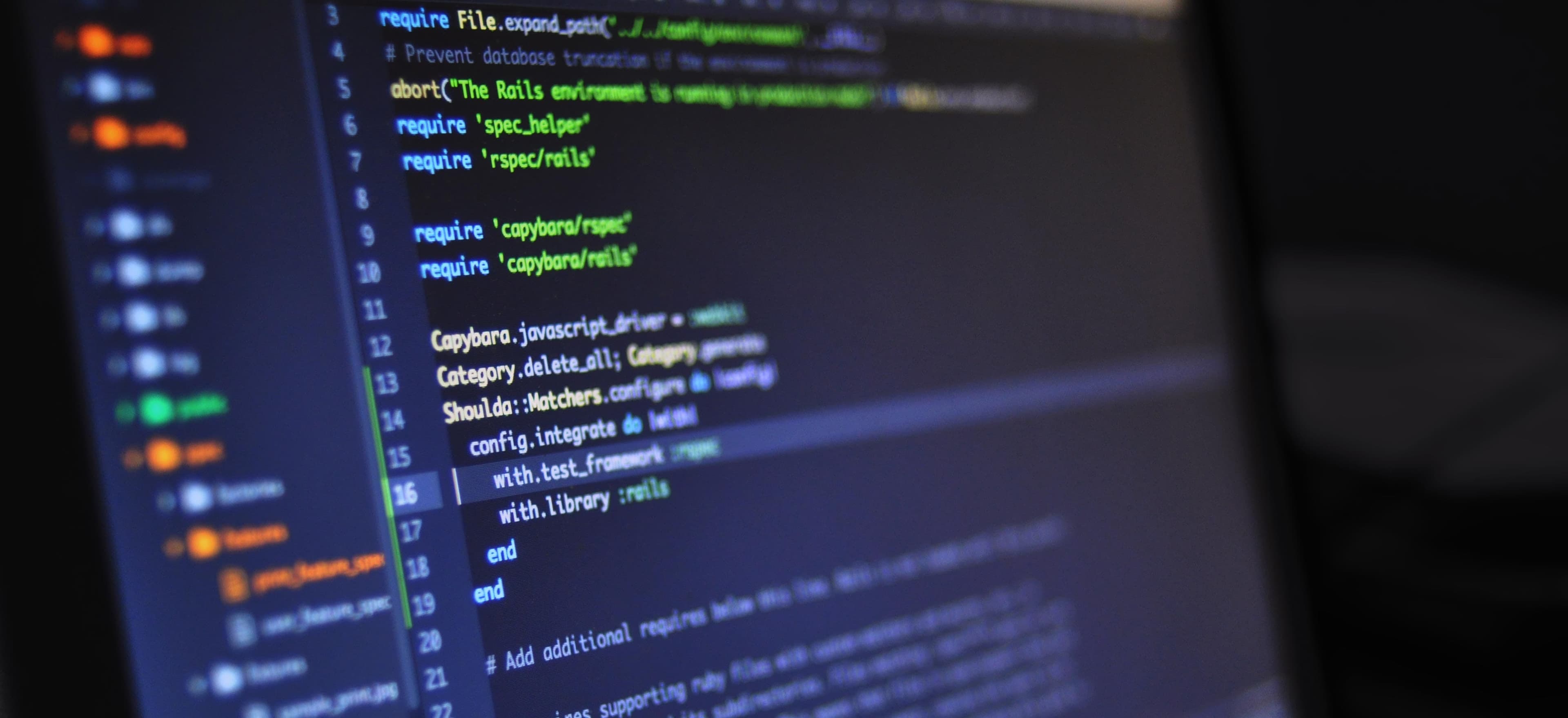
- Published on
Java Memory Management: Tackle Memory Leaks in Your Apps
Java is a powerful language, widely utilized for building robust applications. However, like any other programming language, Java is not immune to memory management issues, particularly memory leaks. This article aims to provide insights into Java's memory management practices and detailed strategies to identify and tackle memory leaks, ensuring your application runs smoothly and efficiently.
Understanding Memory Management in Java
Java employs an automatic memory management system known as the Java Garbage Collector (GC). This system is responsible for reclaiming memory taken up by objects that are no longer in use. The major memory areas in Java include:
- Heap Memory: This is where Java objects are stored. As developers create new objects, space in the heap is allocated dynamically.
- Stack Memory: This memory area handles primitive types and references to objects in the heap. Each thread has its own stack, which stores local variables and function call information.
- Method Area: This is where class-level data is stored, including metadata, static variables, and constants.
The Role of Garbage Collection
Garbage Collection is pivotal to Java's memory management. It relieves developers from the burden of manual memory management, minimizing the chances of memory leaks. However, GC is not flawless, and it can sometimes leave certain objects referenced unintentionally, leading to memory leaks.
What is a Memory Leak?
A memory leak occurs when an application retains a reference to an object that is no longer needed. The object cannot be reclaimed by the garbage collector, consuming valuable memory resources. Memory leaks lead to increased memory consumption, ultimately resulting in performance degradation and application crashes.
Common Causes of Memory Leaks in Java
Identifying potential causes of memory leaks is crucial for maintaining application performance. Here are some common culprits:
- Unclosed Resources: Failing to close connections, files, or streams can prevent the garbage collector from reclaiming memory.
- Static Collections: Using static collections to store objects without clearing them can lead to prolonged memory retention.
- Event Listeners: If event listeners are not removed when they are no longer needed, the objects they reference can remain in memory.
- Inner Classes: Non-static inner classes implicitly hold a reference to their outer class, which can lead to leaks if not handled properly.
Detecting Memory Leaks
Detecting memory leaks can be tricky, especially in large applications. Here are some techniques you can use to identify leaks:
- Profiling Tools: Java Profilers, such as VisualVM, YourKit, and Eclipse Memory Analyzer (MAT), can help visualize memory usage and identify leaks.
- Garbage Collection Logs: Enabling GC logging can provide insights into how memory is allocated and released over time.
- Heap Dumps: Analyzing heap dumps can help locate objects consuming excessive memory and their references.
Example: Using Java Profilers
Java Profilers provide valuable insights into your application's memory consumption. Here's how you can utilize VisualVM to detect memory leaks:
-
Start your Java application with the following options to enable profiling:
java -Dcom.sun.management.jmxremote -jar yourapp.jar
-
Launch VisualVM and connect to your application.
-
Monitor the heap memory usage in the VisualVM window. Look for signs of unexpected growth over time.
-
Use the "Heap Dump" feature to analyze the objects in memory. You can find the path to the unwanted memory link.
By employing profiling tools, developers can identify the specific objects that are not being garbage collected, allowing for efficient debugging.
Strategies to Prevent Memory Leaks
Creating a memory leak-free Java application involves adopting best practices. Here are several strategies to prevent memory leaks:
1. Close Resources
Always close resources when they are no longer needed. This includes database connections, input/output streams, and network connections. Use try-with-resources
where applicable:
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
// The reader is automatically closed, preventing potential memory leaks.
2. Use Weak References
When caching objects, consider using WeakReference
. This allows the garbage collector to reclaim memory from objects when memory is needed:
import java.lang.ref.WeakReference;
class Cache {
private WeakReference<Object> cachedObject;
public void cacheObject(Object obj) {
cachedObject = new WeakReference<>(obj);
}
public Object getCachedObject() {
return cachedObject.get();
}
}
3. Remove Event Listeners
Always unregister event listeners when they are no longer needed. For example:
button.addActionListener(e -> System.out.println("Clicked!"));
// When the button is no longer in use
button.removeActionListener();
4. Be Mindful of Inner Classes
Consider using static inner classes or external classes to avoid implicit references to the outer class:
class OuterClass {
private String data = "Outer Data";
static class StaticInnerClass {
void display() {
System.out.println("Static Inner Class");
}
}
}
By using static inner classes, we avoid holding unnecessary references to the outer class, reducing the risk of memory leaks.
Learning from NodeJS
Just as Java developers need to manage memory leaks diligently, NodeJS developers encounter similar challenges, particularly with asynchronous programming. The article "Conquer NodeJS Memory Leaks: Strategies and Solutions" elaborates on strategies applicable within NodeJS applications and can offer useful insights for Java developers seeking to enhance their understanding of memory management. You can read it here.
The Closing Argument
Effective memory management is crucial to ensuring the smooth operation and performance of Java applications. By understanding how Java handles memory and adopting best practices to prevent memory leaks, developers can create more efficient and reliable applications. Always remember to monitor memory usage, implement strategic caching, and manage event listeners diligently.
As you build and manage Java applications, reference these practices and insights for a cleaner, more reliable memory management experience. Happy coding!
Checkout our other articles