Unlocking Java: A Developer's Guide to Intuitive Programming
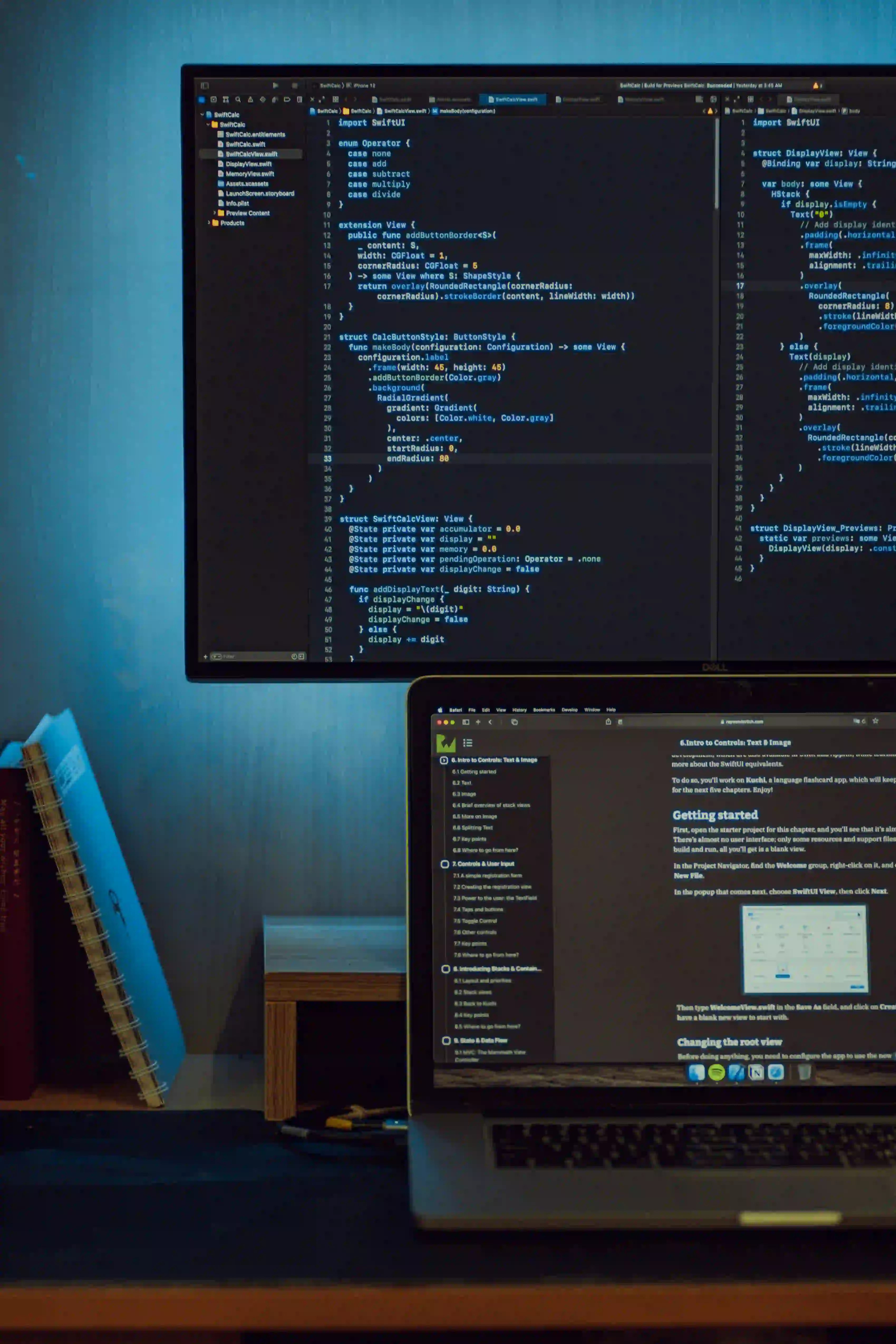
Unlocking Java: A Developer's Guide to Intuitive Programming
Java has long been considered one of the most versatile and powerful programming languages on the market. Its application spans from enterprise software development to mobile app creation. In this blog post, we will explore various concepts in Java, focusing on how to develop a more intuitive understanding of the programming paradigm it embodies.
The Essence of Java
At its core, Java is an object-oriented programming (OOP) language. This style of programming centers around objects, classes, and the principles of encapsulation, inheritance, and polymorphism. Understanding these principles will not only enhance your programming skills but also make your code much cleaner and easier to maintain.
Key Java Concepts Explained
-
Classes and Objects
Objects are instances of classes. They're the blueprints that contain both data (attributes) and methods (functions) that manipulate that data.
☕snippet.javapublic class Car { // Attributes private String model; private int year; // Constructor public Car(String model, int year) { this.model = model; this.year = year; } // Method public void displayInfo() { System.out.println("Model: " + model + ", Year: " + year); } }
Why this matters: Understanding classes and objects is crucial for structuring your code effectively. You're building components that are easy to manage and reuse.
-
Encapsulation
Encapsulation is about controlling access to the data in your classes. You expose only what's necessary, which helps to maintain the integrity of the data.
☕snippet.javapublic class BankAccount { private double balance; // Constructor public BankAccount(double initialBalance) { balance = initialBalance; } // Accessor method public double getBalance() { return balance; } // Method to deposit money public void deposit(double amount) { if (amount > 0) { balance += amount; } } }
Why this matters: Encapsulation provides security. By restricting how data in your objects is accessed, you protect the internal state of your objects from corrupt modifications.
The Power of Inheritance
Inheritance allows you to create new classes based on existing ones. This promotes code reusability and creates a natural hierarchy in your application.
public class ElectricCar extends Car {
private int batteryCapacity;
public ElectricCar(String model, int year, int batteryCapacity) {
super(model, year);
this.batteryCapacity = batteryCapacity;
}
@Override
public void displayInfo() {
super.displayInfo();
System.out.println("Battery Capacity: " + batteryCapacity + " kWh");
}
}
Why this matters: Inheritance encourages a versatile codebase where changes in the parent class propagate to child classes. This minimizes redundancy and accelerates the development process.
Polymorphism Unleashed
Polymorphism allows methods to do different things based on the object that's calling them, which can be incredibly powerful.
public class Vehicle {
public void move() {
System.out.println("The vehicle is moving");
}
}
public class Bicycle extends Vehicle {
@Override
public void move() {
System.out.println("The bicycle is pedaled forward");
}
}
Why this matters: With polymorphism, you can invoke the same method on different objects, each responding in their own way. This makes your code more flexible and enhances its scalability.
Best Practices in Java Programming
-
Use Meaningful Names Always strive for clarity. Use descriptive names for classes, methods, and variables, making it easier for others (and your future self) to understand your code.
-
Comment Your Code Wisely Comments should clarify the 'why' behind complex logic. Don't overdo it; the code itself should be self-explanatory.
-
Follow a Consistent Coding Style Adhere to naming conventions and spacing styles. Consistency allows you and others to read the code easily.
-
Optimize Performance Understand the nuances of Java, like garbage collection and memory management. This will help avoid performance bottlenecks.
Creating Intuitive Applications
Creating intuitive applications with Java relies heavily on user experience. Here are some ways to ensure your application is intuitive:
-
Design first Create prototypes and gather user feedback before diving into coding. Tools like Figma can help with UI design.
-
Focus on the User Journey Anticipate user actions. The flow of your application should be logical.
-
Incorporate Feedback Loops Encourage user feedback after release to fix bugs and improve features.
By integrating these principles into your development process, you can create applications that not only function well but also resonate with users.
Exploring Related Fields: A Nuanced Approach
In programming, just like in art, it's essential to grasp the philosophical elements that underpin your work. As discussed in the fascinating article "Die Geheimnisse des Tarot: Kunst, Intuition und Magie" on auraglyphs.com (https://auraglyphs.com/post/die-geheimnisse-des-tarot-kunst-intuition-und-magie), intuition plays a paramount role in creative processes, including programming. While algorithms and structure are vital in coding, tapping into your intuitive senses can lead to innovative solutions.
Lessons Learned
Your journey with Java doesn't end with mastering its mechanics. It's crucial to foster an intuitive understanding of programming concepts. By leveraging OOP principles, best practices, and focusing on the user experience, you'll find yourself not just writing code, but crafting software that is both powerful and user-friendly.
Continue exploring and practicing, and remember: the more intuitive your programming becomes, the more seamless your development experience will be. Happy coding!