Mastering Java: Overcoming Your First Spring Boot Setup Hurdles
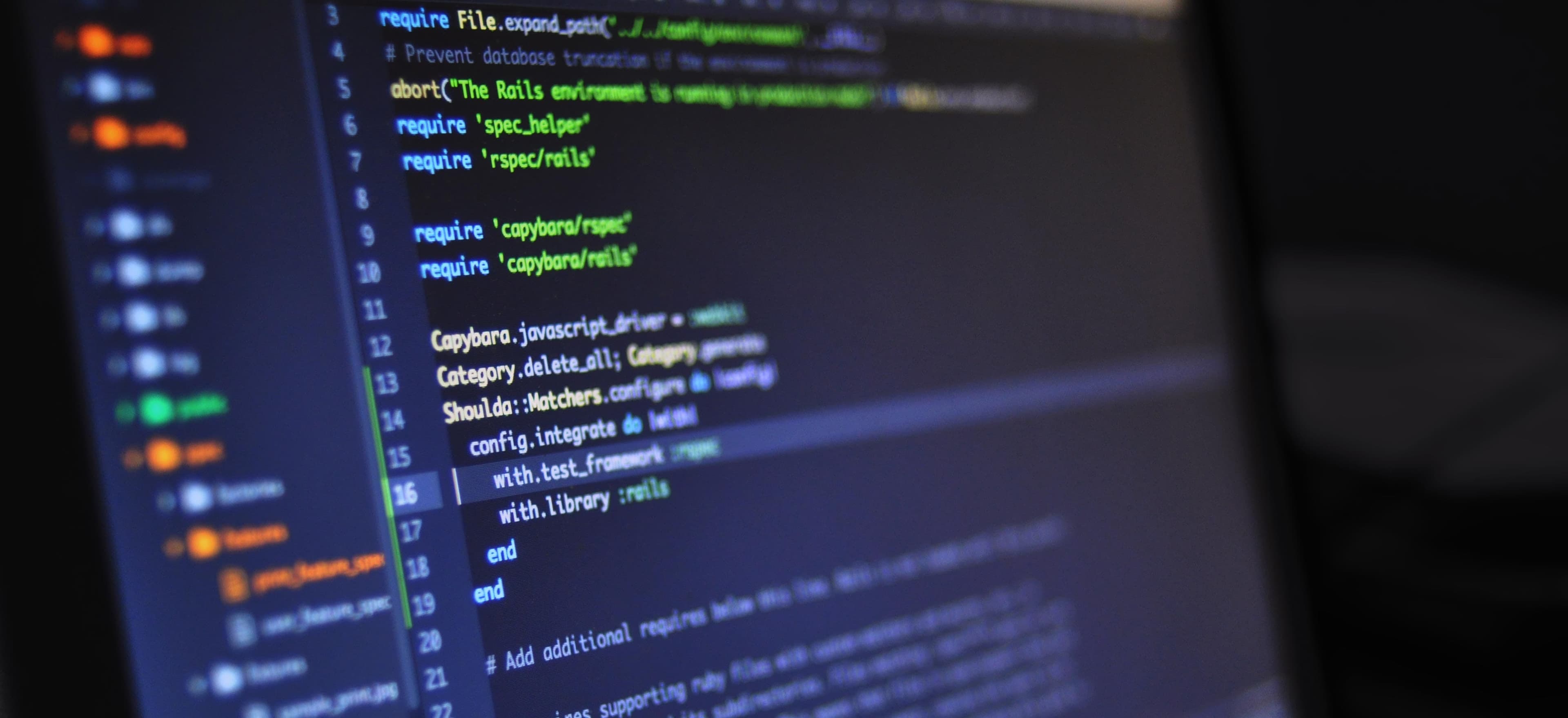
- Published on
Mastering Java: Overcoming Your First Spring Boot Setup Hurdles
Spring Boot has transformed the way developers build Java applications. Its ease of use and extensive feature set make it a go-to framework for creating microservices and web applications. However, as with any technology, the initial setup can sometimes feel daunting. In this guide, we'll walk you through overcoming common hurdles when setting up Spring Boot.
Understanding Spring Boot
Spring Boot simplifies the process of setting up and developing new Spring applications. It eliminates the need for extensive configuration, allowing you to focus on writing business logic. With Spring Boot, you can create stand-alone applications and run them directly. This framework is built on the Spring Framework and provides an opinionated configuration style, which helps you make decisions about the applications you build.
Key Features of Spring Boot
- Auto-configuration: Spring Boot automatically configures necessary libraries based on the classes on your classpath, reducing the need for complex setup.
- Embedded servers: With support for embedded servers like Tomcat and Jetty, you can run your application as a simple .jar file.
- Production-ready features: It includes features like health checks and metrics, making it suitable for production environments.
Prerequisites for Setting Up Spring Boot
Before diving into the setup process, you need to ensure that you have certain prerequisites installed:
- Java Development Kit (JDK): You need JDK 8 or above installed on your machine. You can download the latest version from the Oracle website.
- Maven or Gradle: While Maven is the more commonly used build tool with Spring Boot, Gradle is also a popular alternative.
- Integration with IDE: An Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse can significantly enhance your development experience.
Step 1: Creating a Spring Boot Project
You have multiple options to create a Spring Boot project. The most straightforward way is to use the Spring Initializr, a web-based project generator.
- Go to the Spring Initializr: Visit start.spring.io.
- Project Metadata Configuration:
- Select Maven Project.
- Choose your preferred Java Version.
- Fill in details like Group (for example,
com.example
) and Artifact (for example,demo
).
- Dependencies: Choose the required dependencies. For our example, you can select:
- Spring Web
- Spring Boot DevTools (for easy debugging)
!Spring Initializr Configuration
- Generate the Project: Click on the "Generate" button to download your project as a zip file. Unzip it to your preferred location.
Sample Project Structure
After unzipping, you will notice a typical structure like the following:
demo/
|-- src/
| |-- main/
| | |-- java/
| | | `-- com/
| | | `-- example/
| | | `-- demo/
| | | `-- DemoApplication.java
| | `-- resources/
| | |-- application.properties
|-- pom.xml
- DemoApplication.java is the entry point of your Spring Boot application.
- application.properties allows you to configure various aspects of your application.
Step 2: Importing the Project into Your IDE
Now that you have your Spring Boot project created, it is time to load it into your preferred IDE. Here’s how to do that in IntelliJ IDEA:
- Launch IntelliJ IDEA.
- Select Open and navigate to the directory where you unzipped your project.
- Click OK. The IDE will automatically detect the
pom.xml
file (for Maven projects) and start syncing dependencies.
Sample Code Snippet
The core of any Spring Boot application is its main class. Here’s the DemoApplication.java
code snippet:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Why This Matters: The @SpringBootApplication
annotation signifies that this class serves as the configuration for the Spring Boot application. The SpringApplication.run()
method bootstraps your application, effectively kicking it off.
Step 3: Running Your Application
To run your Spring Boot application:
- Right-click on
DemoApplication.java
in your IDE and select Run 'DemoApplication'. - You should see output indicating that the application is running on
localhost:8080
.
Sample REST Controller
To demonstrate how Spring Boot simplifies building web services, let's create a simple REST controller:
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
Why This Matters: The @RestController
annotation allows you to build a RESTful web service using this class effortlessly. The @GetMapping
annotation maps the /hello
endpoint to the sayHello()
method, which returns a string when accessed.
To test your new endpoint:
- Open your browser and navigate to
http://localhost:8080/hello
. You should see the message "Hello, Spring Boot!".
Step 4: Common Pitfalls and Troubleshooting
Even with a framework as robust as Spring Boot, developers can encounter issues. Here are some common problems and their solutions:
-
Port Already In Use:
-
If you receive an error indicating that port 8080 is already in use, you can change the default port by adding the following line in the
application.properties
file:server.port=8081
-
-
Dependency Issues:
-
Ensure all dependencies are correctly listed in your
pom.xml
. An example of how to add Spring Web dependency is shown below:<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
-
-
404 Error for Endpoints:
- If you get a 404 error when accessing a mapping, confirm that you have mapped the correct URL in your controller.
Closing the Chapter
Setting up Spring Boot for the first time may seem uncomfortable, but this guide should help you navigate the common hurdles. By using Spring Initializr, integrating the project into your IDE, and understanding the core components, you will be set up for success.
For further reading, check out this insightful article titled "Jumpstart Your Node Journey: Solving First-Time Setup Woes" at infinitejs.com/posts/jumpstart-node-journey-solving-setup-woes.
Spring Boot is more than just a framework; it’s an ecosystem that lets you innovate faster and more effectively. Happy coding!
Checkout our other articles