Simplifying Subdomain Management with Java Redirects
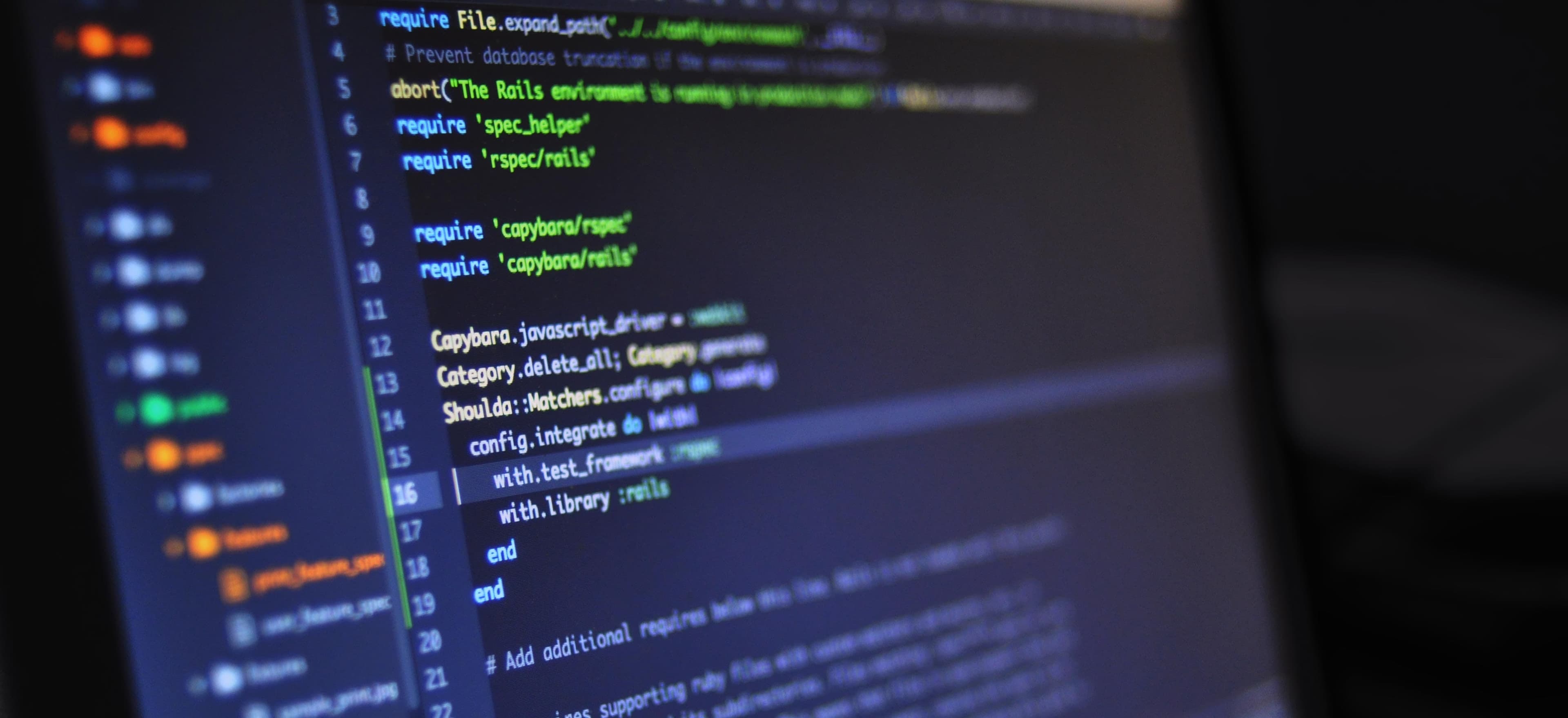
- Published on
Simplifying Subdomain Management with Java Redirects
Managing subdomains within a web application can be a challenging task, especially when trying to maintain a uniform user experience across different domains. To streamline this process, a robust redirect mechanism can be implemented using Java. In this article, we will explore how to implement subdomain redirects effectively to enhance user experience and SEO.
Why Redirect Subdomains?
Redirecting all subdomains to a single subdomain can have several benefits:
-
SEO Optimization: Search engines prefer clean and well-structured URLs. Redirects help in consolidating page authority to a primary subdomain.
-
User Experience: A consistent domain structure improves usability, making it easier for users to bookmark and remember your site.
-
Simplification of Management: Handling multiple subdomains can be cumbersome. A redirect strategy allows central management of content, simplifying deployment and updates.
The Basics of HTTP Redirects
Before diving into the implementation details, it's essential to understand how HTTP redirects work. A redirect is a response sent by a server to tell a client that the requested resource has moved to a different location.
There are several types of redirects, but the two most common are:
-
301 (Moved Permanently): This is the most SEO-friendly option that tells search engines that the content has moved permanently to a new location. This type of redirect transfers page ranking to the new URL.
-
302 (Found): This indicates a temporary redirect, which does not pass the same ranking power to the new URL.
Setting Up a Java Web Application
To illustrate how to perform subdomain redirects using Java, let's set up a basic Java web application. We will use Spring Boot, which simplifies the process of creating standalone, enterprise-grade Spring applications.
Step 1: Create a Spring Boot Application
If you haven't already set up a Spring Boot project, you can use Spring Initializr to bootstrap a new application. Include the Spring Web dependency.
Once you have your project set up, navigate to the main application class.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SubdomainRedirectApplication {
public static void main(String[] args) {
SpringApplication.run(SubdomainRedirectApplication.class, args);
}
}
Step 2: Create a Redirect Controller
Next, create a controller that will handle the redirection of subdomains.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@Controller
public class RedirectController {
@GetMapping("/*")
public void redirect(HttpServletRequest request, HttpServletResponse response) throws IOException {
String host = request.getHeader("Host");
if (host.startsWith("subdomain1.")) {
// Redirect to primary subdomain
response.sendRedirect("https://primarysubdomain.example.com");
} else if (host.startsWith("subdomain2.")) {
// Redirect to primary subdomain
response.sendRedirect("https://primarysubdomain.example.com");
} else {
// Handle other requests
response.sendRedirect("https://primarysubdomain.example.com");
}
}
}
Commentary
-
Handling Requests: The
@GetMapping("/*")
captures all incoming requests regardless of the specific subdomain, enabling centralized control over redirection. -
Checking Subdomains: The
request.getHeader("Host")
retrieves the host information, which allows us to determine which subdomain was accessed. -
Redirecting: Based on the detected subdomain, we call
response.sendRedirect("url")
to redirect the user to the intended location.
Enhancing the Redirect Logic
The current implementation handles only two specific subdomains. To handle more complex scenarios or dynamically manage subdomains, consider creating a mapping using a configuration file or a database.
Example with Mapping
You can use a Map to store multiple subdomains and their corresponding redirection targets.
import java.util.HashMap;
import java.util.Map;
@Controller
public class RedirectController {
private final Map<String, String> subdomainRedirectMap = new HashMap<>();
public RedirectController() {
// Populate subdomain mappings
subdomainRedirectMap.put("subdomain1.example.com", "https://primarysubdomain.example.com");
subdomainRedirectMap.put("subdomain2.example.com", "https://primarysubdomain.example.com");
// Add more subdomains as needed
}
@GetMapping("/*")
public void redirect(HttpServletRequest request, HttpServletResponse response) throws IOException {
String host = request.getHeader("Host");
// Check if the host is in the redirect map
String redirectUrl = subdomainRedirectMap.get(host);
if (redirectUrl != null) {
response.sendRedirect(redirectUrl);
} else {
// Fallback or handle other requests
response.sendRedirect("https://primarysubdomain.example.com");
}
}
}
Benefits of Mapping
-
Maintainability: Editing redirection rules is easier when subdomain mappings are centralized in one location.
-
Scalability: Adding new subdomains requires minimal changes; simply update the map.
Important Considerations
Before deploying your redirect implementation, keep in mind these critical factors:
-
Testing: Always test your redirects to ensure they work as intended. Use tools like Redirect Checker to verify the redirect chain.
-
Caching: Be aware of browser caching; a browser might cache a 301 redirect. Use cache-busting techniques if necessary.
-
Performance: Too many redirects can slow down your application. Aim for simplicity and reduce unnecessary redirects.
Wrapping Up
Redirecting all subdomains to a single subdomain is an effective way to streamline user management, improve SEO, and provide a consistent user experience. By implementing a redirection strategy using Java, specifically with a Spring Boot framework, you can easily manage subdomain requests and enhance your web application.
For further reading on this topic, consider checking out Redirecting All Subdomains to One Subdomain. This article offers insights and strategies for managing subdomains effectively.
Feel free to comment below with any questions or share your experiences with managing subdomains. Happy coding!
Checkout our other articles