Mastering Java Streams for Cleaner State Management
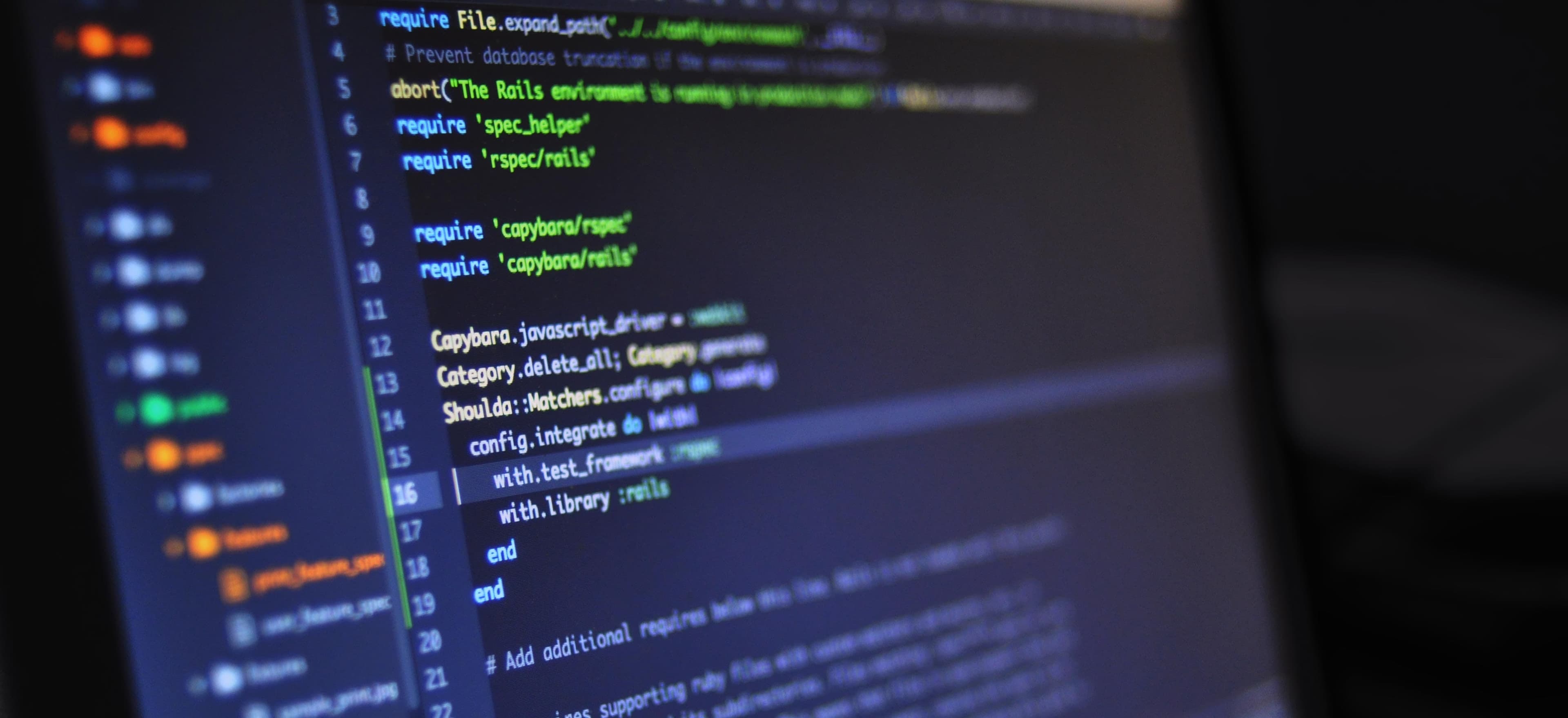
- Published on
Mastering Java Streams for Cleaner State Management
Java has a rich ecosystem, and with its evolution, newer paradigms have inched their way into mainstream programming practices. One such paradigm that has gained popularity is the concept of streams, which allows developers to process data in a functional style. When considering state management in applications, especially when incorporating libraries like Redux, it's crucial to have a clean and efficient way to handle state mutations. In this blog post, we will dive deep into mastering Java Streams for cleaner state management.
Understanding Java Streams
Java Streams were introduced in Java 8 and provide a powerful way to deal with sequences of elements. They allow you to process collections of objects with ease. Think of it as a pipeline where you can filter, map, and process data while maintaining a clean and readable codebase.
Why Use Streams?
- Readability: Streams enable a more functional programming style that often leads to cleaner and more understandable code.
- Conciseness: Code using streams can be significantly shorter than its traditional counterparts.
- Parallelism: Streams can effortlessly switch from sequential to parallel execution, taking advantage of multi-core processors.
Basic Operations on Streams
To illustrate how streams work, let's consider a simple example. Suppose we have a list of integers, and we want to filter out the even numbers and square them.
Here’s how you can create this using streams:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Using Java Streams to filter and square even numbers
List<Integer> squaredEvens = numbers.stream()
.filter(n -> n % 2 == 0) // Filter even numbers
.map(n -> n * n) // Square the numbers
.collect(Collectors.toList()); // Collect results
System.out.println(squaredEvens); // Output: [4, 16, 36]
}
}
Commentary on the Code
- Filter Operation: The
filter
method is used to specify a criterion. Here, we filter only even numbers using a lambda expression. - Map Operation: The
map
method transforms the remaining elements. In our case, we square them. - Collect Operation: Finally, with
collect
, we gather the results into a List.
The reason we can use such methods seamlessly is due to the power of streams, which make it easy to manipulate data without altering the original source.
Leveraging Streams for State Management
As we manage state in applications, particularly those built with patterns similar to Redux (for instance, applications that heavily rely on global state management), it becomes essential to handle state immutably and efficiently. Streams offer a great way to make state updates cleaner and less error-prone.
Immutable Data Structures
While mutating global state can lead to bugs, especially in large applications, using immutable data structures can help maintain clarity. By combining Java Streams with immutable collections, you can manage state changes transparently.
Here's how we might structure state management using streams:
Initial State Representation
import java.util.ArrayList;
import java.util.List;
public class AppState {
private final List<String> items;
public AppState(List<String> items) {
this.items = new ArrayList<>(items); // Creating a new instance for immutability
}
public List<String> getItems() {
return List.copyOf(items); // Return an unmodifiable view of the list
}
public AppState addItem(String item) {
List<String> updatedItems = new ArrayList<>(this.items);
updatedItems.add(item);
return new AppState(updatedItems); // Return a new state instance
}
}
Commentary on State Changes
- Immutable Collections: By returning
List.copyOf(items)
, we ensure the state cannot be altered directly from outside the class. - State Updates: The
addItem
method creates a new instance ofAppState
whenever an item is added. This helps prevent unintended side effects, promoting robustness in state management.
You can find more on effectively managing state with Redux-like patterns in the article titled Taming Redux: Simplify State Management in Apps.
Using Streams to Handle State Updates
Now, let's combine streams with our state management.
Suppose we want to filter our state entries based on some criteria each time we access them.
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
AppState currentState = new AppState(List.of("Item1", "Item2", "Item3"));
// Filter to get items that start with "Item"
List<String> filteredItems = currentState.getItems().stream()
.filter(item -> item.startsWith("Item"))
.collect(Collectors.toList());
System.out.println(filteredItems); // Output: [Item1, Item2, Item3]
}
}
Commentary on the Filtering Process
- Filter for Criteria: We apply a filter to access specific elements from the state.
- Stream Operations: Using the streams approach, we ensure that even while accessing the state, we don’t modify the original data.
- New List Creation: The result is immutable and helps maintain state integrity in the application.
Summary and Conclusion
Java Streams provide a powerful, concise, and readable way to handle data and state management in a functional style. By leveraging streams, you can ensure that your applications are built on solid principles of immutability, fostering maintainability and reducing errors that often come with state management.
For developers looking to build robust applications, integrating Java Streams into your state management strategy can help enhance both performance and code quality. Always remember to treat state as immutable, apply functional programming paradigms like streams, and be intentional about how you manage state changes.
For more information on managing state in applications, explore the best practices discussed in Taming Redux: Simplify State Management in Apps. Happy coding!