Enhance JavaFX Graphics with SVG Gradient Fills
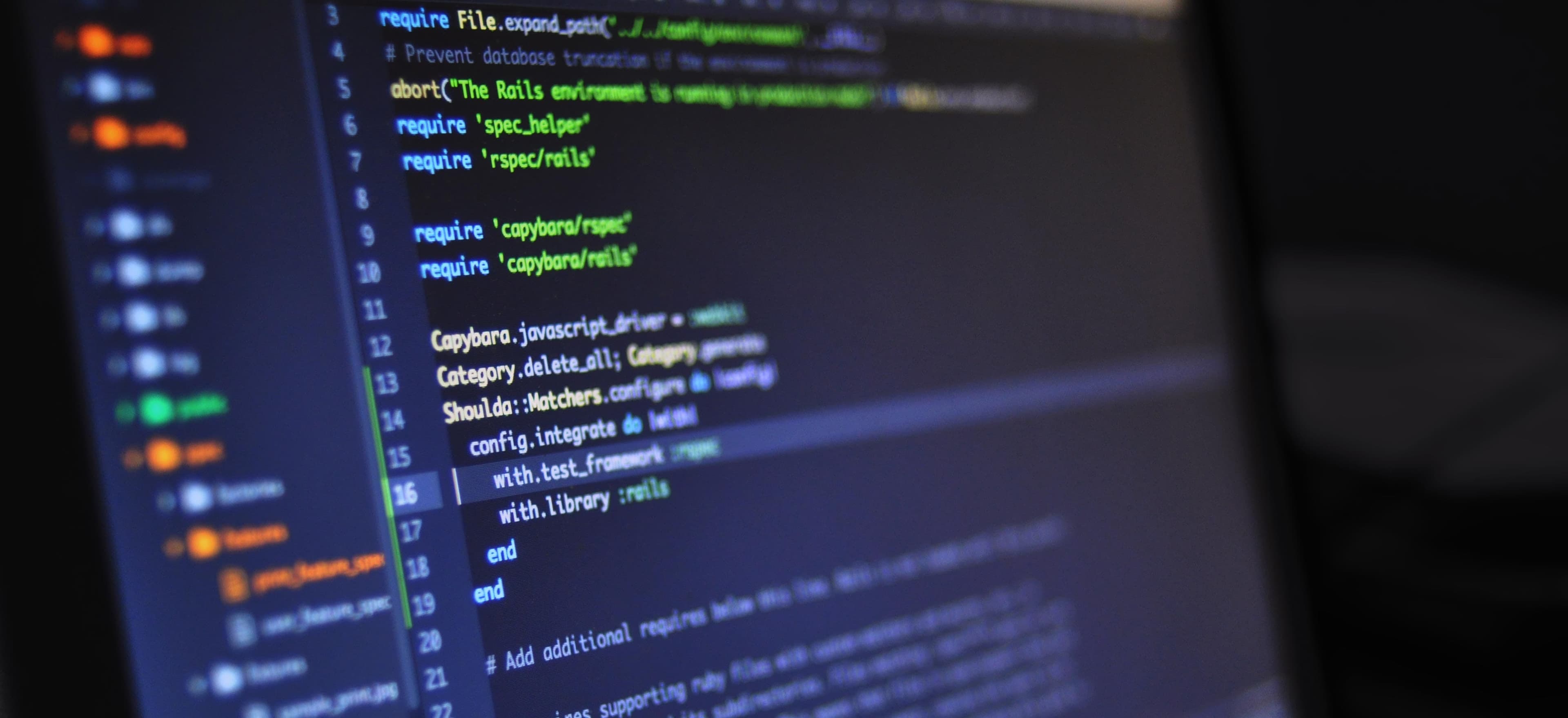
- Published on
Enhance JavaFX Graphics with SVG Gradient Fills
JavaFX has become a popular choice for developing rich desktop applications in Java. One of its powerful features is the ability to create stunning graphics using SVG (Scalable Vector Graphics). In this article, we'll explore how to enhance your JavaFX applications by incorporating SVG gradient fills, adding depth and creativity to your designs.
Why Use SVG Gradient Fills?
SVG gradient fills allow developers to apply smooth transitions between colors, creating visually appealing graphics that can transform an ordinary application into an extraordinary one. This enhancement is particularly useful in modern UI design, where aesthetics play a crucial role in user engagement.
Benefits of SVG
- Scalability: Unlike raster images, SVG graphics scale without losing quality.
- Accessibility: SVG is text-based, making it easier to search and manipulate programmatically.
- Animation: SVG allows for smooth animations and transitions.
Getting Started with JavaFX and SVG
To begin, you'll need to set up your JavaFX development environment. Make sure you have the latest version of Java and JavaFX installed. You can include JavaFX in your project using Maven:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>19.0.0</version>
</dependency>
With your environment ready, we can dive into using gradient fills with SVG. JavaFX supports gradient fills through the LinearGradient
or RadialGradient
classes.
Example: Creating a Linear Gradient Fill
Let’s start with a simple example that applies a linear gradient to a rectangle:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.paint.LinearGradient;
import javafx.scene.paint.Stop;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class GradientFillExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rect = new Rectangle(200, 200);
// Create a linear gradient fill.
LinearGradient linearGradient = new LinearGradient(
0, 0, 1, 1, // startX, startY, endX, endY
true, // proportional
null, // spread, can be null for default
new Stop(0, Color.RED),
new Stop(1, Color.BLUE)
);
rect.setFill(linearGradient);
StackPane root = new StackPane(rect);
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("JavaFX SVG Gradient Fill Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of the Code
- Rectangle Creation: We create a rectangle with specified width and height.
- LinearGradient Object: This object represents a gradient that transitions from color red to blue. The parameters for the
LinearGradient
constructor define the gradient's starting and ending points and whether the gradient is proportional. - Setting Fill: We then apply the gradient to the rectangle.
- Stage Setup: Finally, we set up the stage and scene to display the rectangle.
This example showcases how easy it is to create visually appealing gradient fills in your JavaFX applications.
Exploring Radial Gradients
In addition to linear gradients, Radial Gradients provide a circular transition of colors. Here's how you can implement a radial gradient:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.paint.RadialGradient;
import javafx.scene.paint.Stop;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class RadialGradientExample extends Application {
@Override
public void start(Stage primaryStage) {
Circle circle = new Circle(100);
// Create a radial gradient fill.
RadialGradient radialGradient = new RadialGradient(
0, // focusAngle
0, // focusDistance
0.5, // centerX
0.5, // centerY
1, // radius
false, // proportional
null, // spread
new Stop(0, Color.YELLOW),
new Stop(1, Color.GREEN)
);
circle.setFill(radialGradient);
StackPane root = new StackPane(circle);
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("JavaFX SVG Radial Gradient Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of the Code
- Circle Creation: A circle is created to demonstrate the radial gradient.
- RadialGradient Object: Similar to linear gradients but with focus points and radius.
- Setting Fill: The circle now features a yellow center transitioning to green.
- Stage Setup: The stage is set up to display the circle.
Integrating SVG with JavaFX for Gradient Fills
While we have thus far discussed native JavaFX gradient features, using SVG files can enhance our graphics further. SVG format is versatile, and it allows for complex designs and gradients beyond what's possible with predefined JavaFX gradients.
You can learn more about transforming SVG patterns into gradients by reading the article titled Transforming SVG Patterns into Stunning Gradient Fills.
Loading SVG Files
JavaFX facilitates the use of SVG files through the SVGPath
class. You can load an SVG path and apply a gradient fill similarly to how we did with rectangles and circles.
Here’s a brief example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.paint.LinearGradient;
import javafx.scene.paint.Stop;
import javafx.scene.shape.SVGPath;
import javafx.stage.Stage;
public class SVGGradientExample extends Application {
@Override
public void start(Stage primaryStage) {
SVGPath svgPath = new SVGPath();
svgPath.setContent("M 10 20 L 40 20 L 40 40 L 20 40 Z");
// Create a linear gradient fill.
LinearGradient linearGradient = new LinearGradient(
0, 0, 1, 1, true, null,
new Stop(0, Color.BLUE),
new Stop(1, Color.GREEN)
);
svgPath.setFill(linearGradient);
StackPane root = new StackPane(svgPath);
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("SVG Gradient Fill Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Explanation of the Code
- SVGPath Creation: An SVG path is initialized with SVG content that defines a simple shape.
- Gradient Creation: A linear gradient is applied, transitioning from blue to green.
- Setting Fill: The SVG path is filled using the gradient.
A Final Look
By using SVG gradient fills in your JavaFX applications, you can significantly enhance your graphical designs. Whether you're working with simple shapes or complex SVG paths, the gradients provide a powerful tool for artistic expression and user engagement.
Experiment with different colors and shapes to find the combinations that work best for your application. Don't forget to explore various resources and articles, like Transforming SVG Patterns into Stunning Gradient Fills, to deepen your understanding of SVG integration with JavaFX.
Further Reading
By embracing these techniques, you're not just enhancing visual appeal — you're leveraging the full capabilities of JavaFX to create engaging, modern applications. Happy coding!