Java Solutions for Seamlessly Migrating Content to WordPress
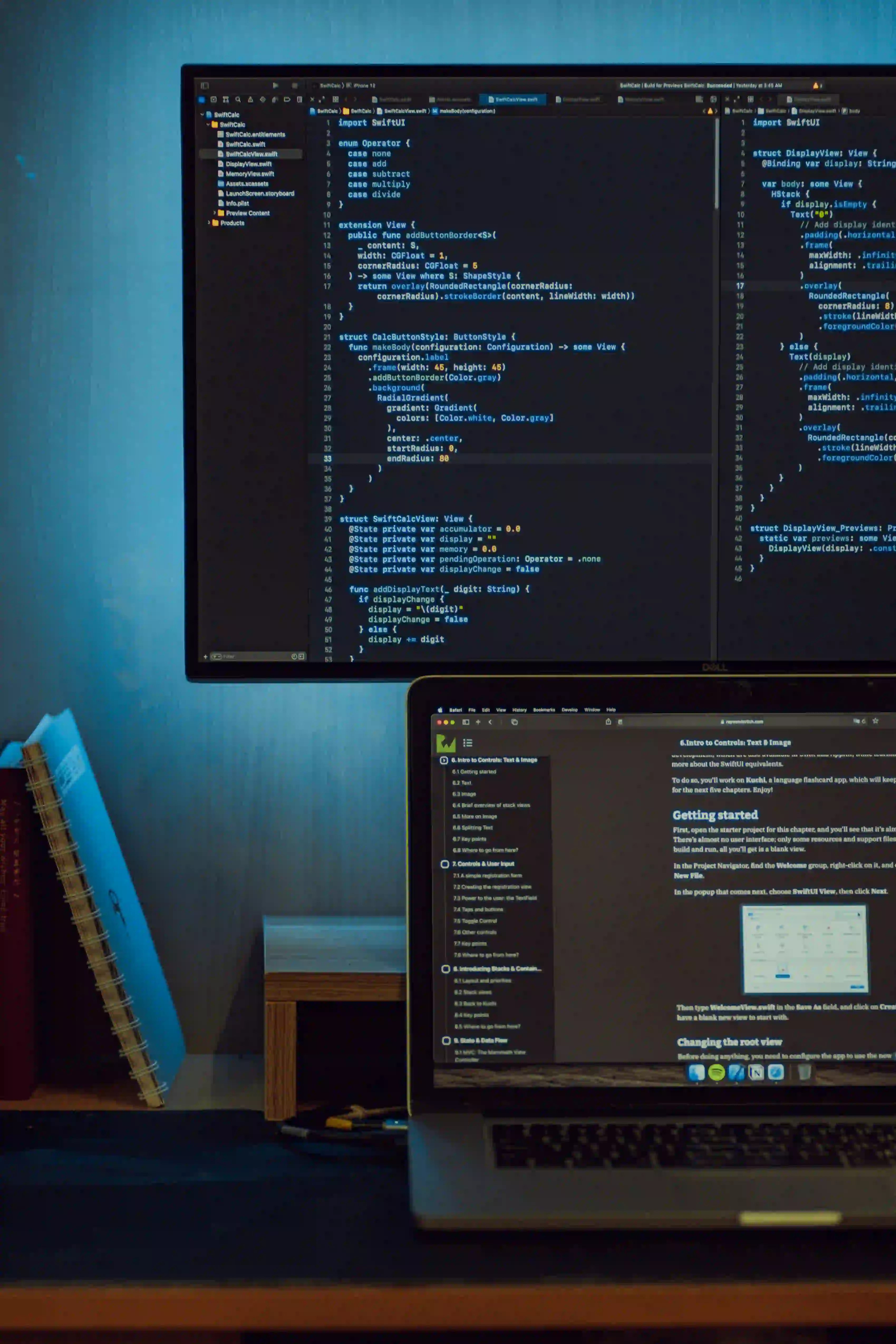
Java Solutions for Seamlessly Migrating Content to WordPress
Migrating to WordPress can often feel like an uphill battle. It’s not just about moving content; it involves ensuring that everything remains intact and performs optimally on the new platform. Thankfully, if you are a Java developer, you have a robust toolkit at your disposal to facilitate this transition. In this blog post, we'll explore how Java can be leveraged to migrate content seamlessly, focusing on a practical approach that balances complexity with clarity.
Understanding the Migration Process
Before diving into the Java solutions, it’s essential to understand the stages of migration. It typically involves:
- Exporting Content: Extracting existing content from the source system.
- Transforming Data: Modifying the extracted data to match WordPress' structure.
- Importing Data: Loading the transformed data into WordPress.
Each of these steps can be handled in Java, utilizing libraries and frameworks to streamline operations.
Step 1: Exporting Content
Database Connectivity
To get your content out of the old system, you first need a database connection. Let’s use JDBC (Java Database Connectivity), a standard Java API for connecting to databases.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class ContentExporter {
private Connection connect() {
String url = "jdbc:mysql://localhost:3306/old_system_db";
String user = "root";
String password = "password";
Connection conn = null;
try {
conn = DriverManager.getConnection(url, user, password);
} catch (SQLException e) {
System.out.println(e.getMessage());
}
return conn;
}
public void fetchContent() {
String sql = "SELECT title, content, date FROM articles";
try (Connection conn = this.connect();
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql)) {
while (rs.next()) {
// Extract content
String title = rs.getString("title");
String content = rs.getString("content");
String date = rs.getString("date");
// Process content as needed
System.out.printf("Title: %s, Content: %s, Date: %s%n", title, content, date);
}
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
Explanation
- Database Connection: Establishes a connection using JDBC.
- SQL Execution: Executes a SQL query to obtain the necessary content.
- Data Extraction: Iterates through the result set, printing each article's details.
Why use JDBC? It provides a standardized method to interact with various databases, making it versatile for different systems.
Step 2: Transforming Data
With the content extracted, the next phase is transforming it into a format WordPress can consume. WordPress utilizes a specific data structure, so we often need to convert our old content accordingly.
Data Transformation Example
Here’s a basic example of transforming data into an XML format compatible with WordPress import plugins.
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import java.io.File;
public class DataTransformer {
public void createXMLFile(String title, String content, String date) {
try {
DocumentBuilderFactory docFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder docBuilder = docFactory.newDocumentBuilder();
// Create document
Document doc = docBuilder.newDocument();
Element rootElement = doc.createElement("wordpress");
doc.appendChild(rootElement);
Element post = doc.createElement("post");
rootElement.appendChild(post);
Element postTitle = doc.createElement("title");
postTitle.appendChild(doc.createTextNode(title));
post.appendChild(postTitle);
Element postContent = doc.createElement("content");
postContent.appendChild(doc.createTextNode(content));
post.appendChild(postContent);
Element postDate = doc.createElement("date");
postDate.appendChild(doc.createTextNode(date));
post.appendChild(postDate);
// Save the XML to a file
// XML writing code goes here (e.g., Transformer to write the Document to a file)
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- XML Document Creation: This snippet shows how to create an XML document with articles formatted appropriately for WordPress.
- Structuring Data: The script organizes each article with its title, content, and date.
Why XML? Many WordPress import tools accept XML data, making it a suitable choice for bulk imports.
Step 3: Importing Data into WordPress
After transforming your content into XML, you need to import it into WordPress. Fortunately, WordPress offers built-in tools to facilitate this.
You can upload your XML file directly through the WordPress admin panel using the "Import" function. However, for larger migrations or automated processes, you might consider using the WordPress REST API for programmatic import.
Example of Using the REST API in Java
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class ContentImporter {
public void importContent(String xmlData) {
try {
URL url = new URL("http://yourwordpresssite.com/wp-json/wp/v2/posts");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.setRequestProperty("Content-Type", "application/xml");
conn.setRequestProperty("Authorization", "Bearer YOUR_ACCESS_TOKEN");
try (OutputStream os = conn.getOutputStream()) {
byte[] input = xmlData.getBytes("utf-8");
os.write(input, 0, input.length);
}
int responseCode = conn.getResponseCode();
System.out.println("Response Code: " + responseCode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- HTTP Connection: Open a connection to the WordPress REST API.
- POST Request: Send the XML data to the specified endpoint.
- Authorization: This example uses Bearer token authentication, which is recommended for security.
Why use the REST API? It allows for dynamic interaction with your WordPress site, enabling automated content migrations without manual uploads.
To Wrap Things Up
Migrating to WordPress doesn’t have to be a daunting task. With Java, you can effortlessly extract, transform, and import your content with precision. Implementing these solutions allows for a smoother transition, ensuring that your blog retains its identity.
For additional context on content migration, check out the article titled "Revamp Your Site: Integrate Old Content into WordPress" at tech-snags.com/articles/revamp-site-integrate-old-content-wordpress.
This will help you grasp how to make the most of existing resources and information while enhancing your site’s performance in WordPress.
In conclusion, whether you're dealing with simple text or complex databases, Java stands as a powerful ally in your content migration journey. Embrace its potential, optimize your workflows, and watch your content thrive in its new home.