Streamline Java Web Development: Migrate Legacy Content Effortlessly
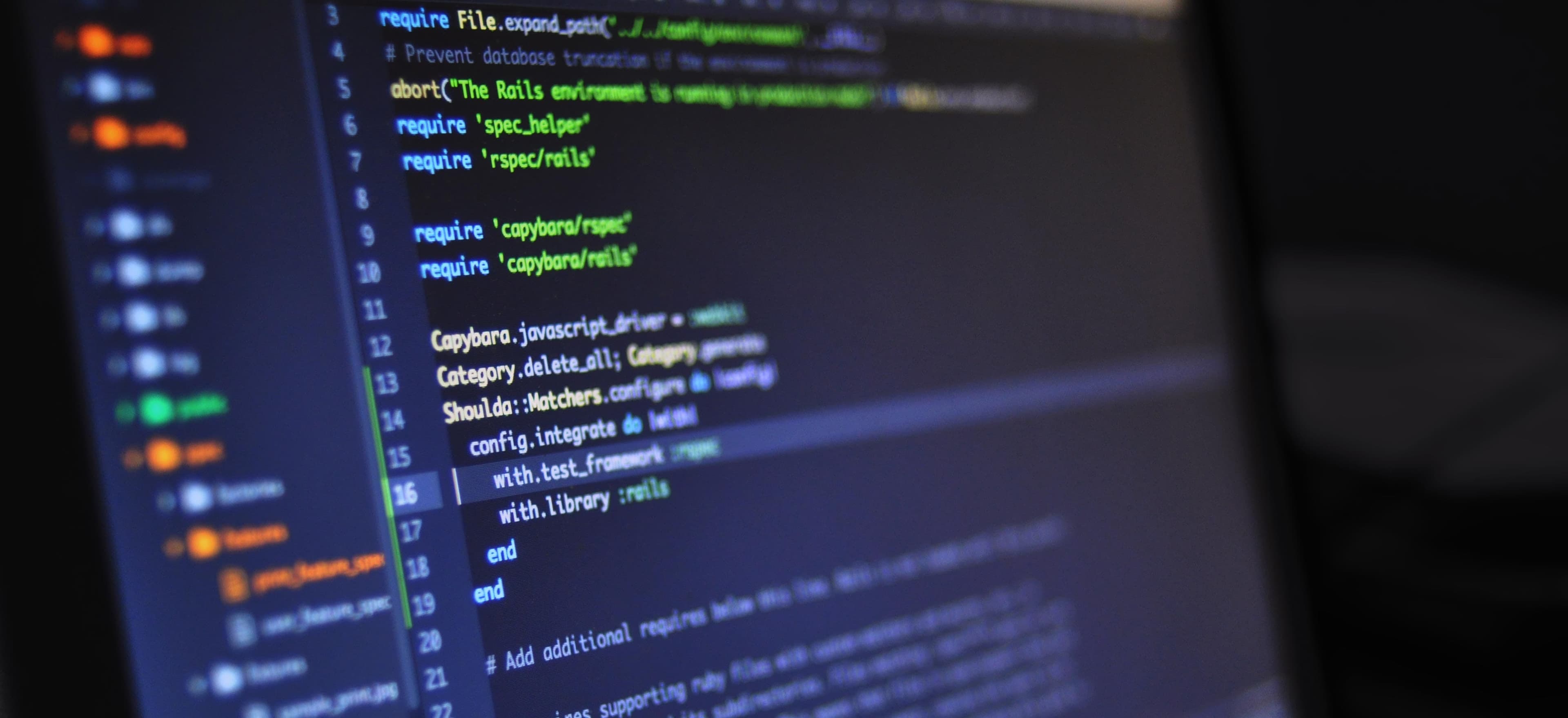
- Published on
Streamline Java Web Development: Migrate Legacy Content Effortlessly
In today's fast-paced digital environment, maintaining a robust web application can involve navigating a labyrinth of legacy content. Many businesses leverage Java for its robust features but face the challenge of integrating older content smoothly. If you're one of those developers looking to revamp your application, this blog post will guide you through strategies for efficient legacy content migration.
Understanding the Challenge
Legacy content can be a double-edged sword. It embodies the historical data and information that makes your application valuable, yet it often resides in outdated systems or formats that can complicate modernization efforts. Migrating this content may get daunting, but leveraging Java's powerful frameworks can make it a straightforward process.
Let's dive into the key strategies and code snippets that can help you migrate legacy content seamlessly.
Key Strategies for Migrating Legacy Content
-
Assess Your Legacy Content: Before anything else, a process evaluation is essential. Identify what still holds value. This might include databases, files, APIs, or services that are still in use.
-
Choose the Right Framework: Java has a plethora of frameworks that can facilitate migration. Frameworks like Spring Boot or Hibernate can simplify fetching, transforming, and loading (ETL) legacy content.
-
Use Design Patterns: Implementing design patterns—such as the Repository Pattern—can isolate your data access layer and make testing easier.
-
Automate the Migration Process: Embrace automation tools and scripts to handle repetitive tasks involved in migration. This minimizes human error and accelerates the entire process.
Implementing the Migration
Now that you have a strategy, let's take a look at how to leverage Java code for this process. We will focus on using Spring Boot for its simplification of configuration and dependency management.
Setting Up Your Spring Boot Project
Make sure to create a new Spring Boot application. You can do this via Spring Initializr. Select dependencies like Spring Web, Spring Data JPA, and your preferred Database Driver (like MySQL if you're migrating from an old database).
Retrieving Data from Legacy Systems
Often, legacy content comes from outdated databases. Here’s a code snippet to create a simple repository to fetch data.
import org.springframework.data.jpa.repository.JpaRepository;
public interface LegacyContentRepository extends JpaRepository<LegacyContent, Long> {
List<LegacyContent> findAll();
}
Why: By extending JpaRepository
, you gain basic CRUD operations without manually writing boilerplate code. This makes content extraction seamless.
Transforming Data
Once you retrieve the data, it might require some transformation to fit into your new model. For this, you can create a service that handles the conversion.
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.stream.Collectors;
@Service
public class LegacyContentService {
private final LegacyContentRepository legacyContentRepository;
public LegacyContentService(LegacyContentRepository legacyContentRepository) {
this.legacyContentRepository = legacyContentRepository;
}
public List<NewContentModel> migrateContent() {
List<LegacyContent> legacyContents = legacyContentRepository.findAll();
return legacyContents.stream().map(this::transform).collect(Collectors.toList());
}
private NewContentModel transform(LegacyContent legacyContent) {
NewContentModel newContent = new NewContentModel();
newContent.setTitle(legacyContent.getTitle());
newContent.setDescription(legacyContent.getDescription());
newContent.setPublishedDate(legacyContent.getCreationDate());
return newContent;
}
}
Why: The migrateContent()
method retrieves all legacy contents and transforms them into a new model setup using Java Streams for a modern, concise approach.
Persisting Data into New System
After transforming the data, the next step is to save it to the new system. Here’s how you could implement this:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class NewContentService {
private final NewContentRepository newContentRepository;
private final LegacyContentService legacyContentService;
public NewContentService(NewContentRepository newContentRepository, LegacyContentService legacyContentService) {
this.newContentRepository = newContentRepository;
this.legacyContentService = legacyContentService;
}
@Transactional
public void saveMigratedContent() {
List<NewContentModel> contentToMigrate = legacyContentService.migrateContent();
newContentRepository.saveAll(contentToMigrate);
}
}
Why: Using the @Transactional
annotation ensures that the entire operation is carried out reliably—either all data is saved, or it's rolled back in case of an error, maintaining data integrity.
Key Takeaways: Streamlining Your Process
Migrating legacy content doesn’t have to be an arduous task if approached correctly. By utilizing frameworks like Spring Boot, employing well-structured repositories, and keeping design principles in mind, you can streamline the entire process.
For additional context on how to integrate legacy content into modern systems using WordPress, refer to the article Revamp Your Site: Integrate Old Content into WordPress.
In summary, whether it's through improved data handling via Spring or meticulous data transformation strategies, focusing on your migration process will yield significant benefits in the long run. Always remember to test your solutions thoroughly and update documentation to support future developers maintaining your newly modernized application.
As you consider your migration plan, remember: well-managed transitions pave the way for innovation and enhanced user experiences. Happy coding!
Checkout our other articles