Handling Message Failures in Spring JMS Transactions
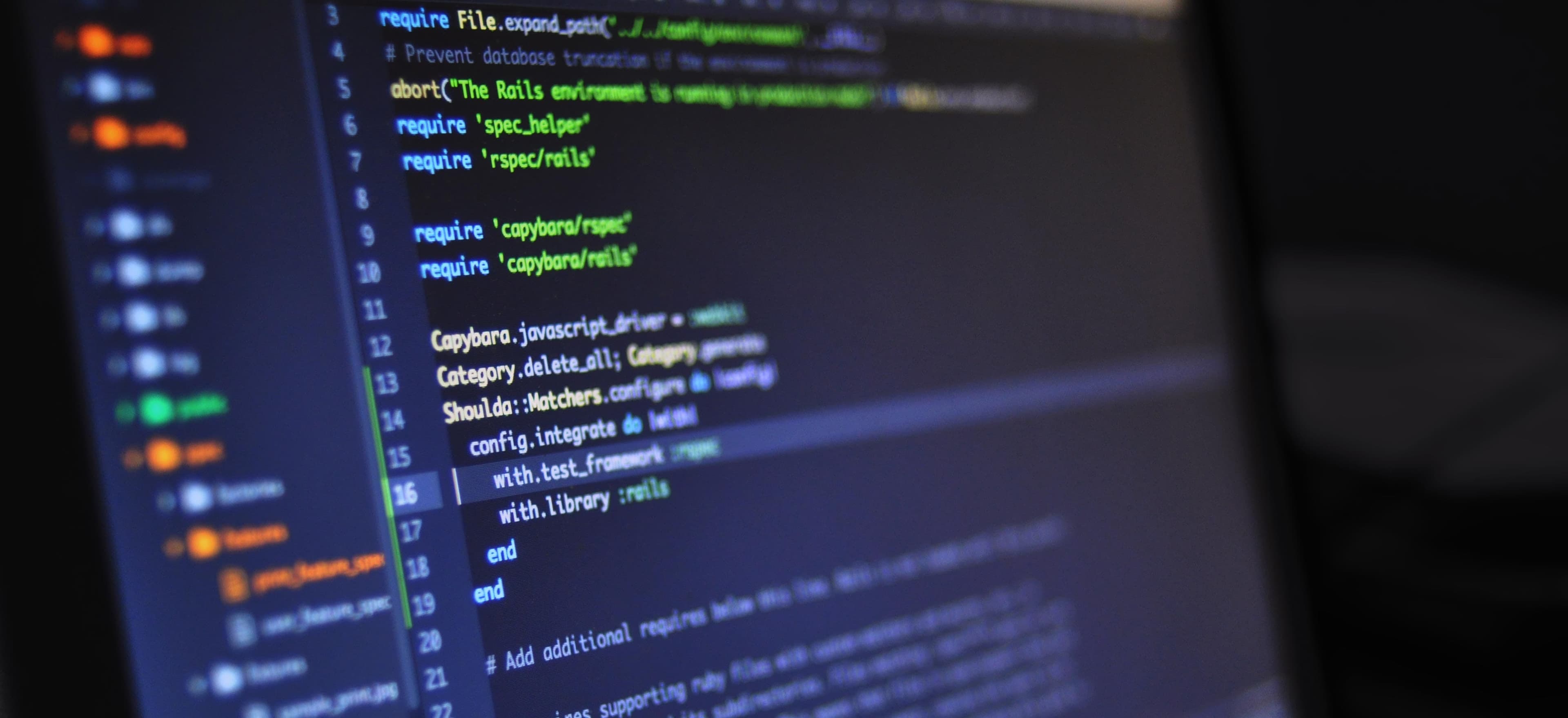
- Published on
Handling Message Failures in Spring JMS Transactions
Java Message Service (JMS) provides a way for applications to create, send, receive, and read messages. When dealing with JMS, especially in a Spring application, it is crucial to manage message failures effectively. This blog post will delve into handling message failures in Spring JMS transactions with an emphasis on best practices, exemplary code snippets, and explanations on why certain approaches yield better reliability and maintainability.
Understanding Spring JMS Transactions
Before we dive into error handling, let's take a moment to understand how Spring handles JMS transactions. Spring’s JMS support leverages transaction management, which allows you to send and receive messages in a transactional scope. This means that if a message fails to process, it won’t affect other successful operations.
Key Components of Spring JMS Transactions
- ConnectionFactory: Used to create connections to the JMS provider.
- JmsTemplate: Simplifies sending and receiving messages.
- MessageListener: This interface helps in consuming messages asynchronously.
Configuring Spring JMS for Transactions
To get started, ensure you have Spring JMS dependencies in your pom.xml
or build.gradle
:
<dependency>
<groupId>org.springframework.jms</groupId>
<artifactId>spring-jms</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.apache.activemq</groupId>
<artifactId>activemq-spring-boot-starter</artifactId>
<version>2.4.0</version>
</dependency>
Example Configuration
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.annotation.EnableJms;
import org.springframework.jms.connection.CachingConnectionFactory;
import org.apache.activemq.ActiveMQConnectionFactory;
import org.springframework.jms.core.JmsTemplate;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.jms.annotation.JmsListener;
@Configuration
@EnableJms
public class JmsConfig {
@Bean
public ActiveMQConnectionFactory connectionFactory() {
return new ActiveMQConnectionFactory("tcp://localhost:61616");
}
@Bean
public CachingConnectionFactory cachingConnectionFactory() {
return new CachingConnectionFactory(connectionFactory());
}
@Bean
public JmsTemplate jmsTemplate() {
return new JmsTemplate(cachingConnectionFactory());
}
@Bean
public PlatformTransactionManager transactionManager() {
// Configure your transaction manager
}
}
In this configuration, we set up a CachingConnectionFactory
that improves performance by buffering connections to queues.
Handling Message Failures
Handling failures in message processing can take various forms, from retries to logging and alerting. Below are common strategies for managing message failures in Spring JMS transactions.
1. Retry Mechanism
A simple but essential strategy involves retrying the message processing when an error occurs. This can be handled either programmatically or through configuration with the help of a RetryTemplate
.
Programmatic Retry Example
import org.springframework.jms.annotation.JmsListener;
import org.springframework.jms.core.JmsTemplate;
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.EnableRetry;
import org.springframework.retry.annotation.Retryable;
import org.springframework.stereotype.Component;
@Component
@EnableRetry
public class MessageListener {
private final JmsTemplate jmsTemplate;
public MessageListener(JmsTemplate jmsTemplate) {
this.jmsTemplate = jmsTemplate;
}
@JmsListener(destination = "exampleQueue")
@Retryable(value = { Exception.class }, maxAttempts = 5, backoff = @Backoff(delay = 2000))
public void listen(String message) {
try {
processMessage(message);
} catch (Exception e) {
// Log the error
throw e; // Rethrow to trigger retry
}
}
private void processMessage(String message) {
// Simulating potential failure
if (Math.random() < 0.5) {
throw new RuntimeException("Simulated processing error");
}
System.out.println("Processed message: " + message);
}
}
In this example, a @Retryable
annotation is applied to the listen
method, which automatically retries the processing logic up to five attempts in case of an exception. The Backoff
parameter ensures that we wait 2 seconds between each retry.
2. Message Acknowledgment
Proper acknowledgment is crucial to ensure that messages are processed only once. In Spring, you have the Session.TRANSACTED
acknowledgment mode which ensures that messages are only acknowledged when the transaction is committed.
3. Dead Letter Queues
If a message cannot be processed after several retries, you may want to consider sending it to a Dead Letter Queue (DLQ). This allows you to handle problematic messages separately without losing them.
Example of DLQ Handling
In your configuration, you can set a DLQ using ActiveMQ settings:
# application.properties
spring.activemq.broker-url=tcp://localhost:61616
spring.activemq.packages.trust-all=true
If a message is deemed unprocessable, you can direct it to a DLQ by configuring your target queue:
@Component
public class MessageFailureHandler {
private final JmsTemplate jmsTemplate;
public MessageFailureHandler(JmsTemplate jmsTemplate) {
this.jmsTemplate = jmsTemplate;
}
public void handleFailure(String message) {
jmsTemplate.convertAndSend("DLQ", message);
System.out.println("Message sent to DLQ: " + message);
}
}
In this case, when message processing fails after retries, the message is sent to a DLQ named DLQ
. Any further manual analysis can be performed on messages in this queue.
Logging and Monitoring
It's essential to log message failures for monitoring purposes. Use tools like Spring Boot Actuator to track the application's health metrics and message processing efficiency.
Wrapping Up
Handling message failures in Spring JMS transactions is crucial for building robust, scalable applications. By employing retries, acknowledging messages properly, and utilizing Dead Letter Queues, you can create a resilient architecture against message processing failures.
Remember that clearer insights into how your application handles failures will improve your message-driven architecture significantly. Implementing logging and monitoring practices will also ensure operational transparency.
For more detailed information about Spring JMS, refer to the Spring JMS Documentation.
Implement these strategies for message failure handling, and ensure your Spring applications are resilient and maintainable. If you have any questions or other approaches not covered in this article, feel free to share your thoughts in the comments below!
Checkout our other articles