Troubleshooting Spring MockMvc: JSON Response Issues
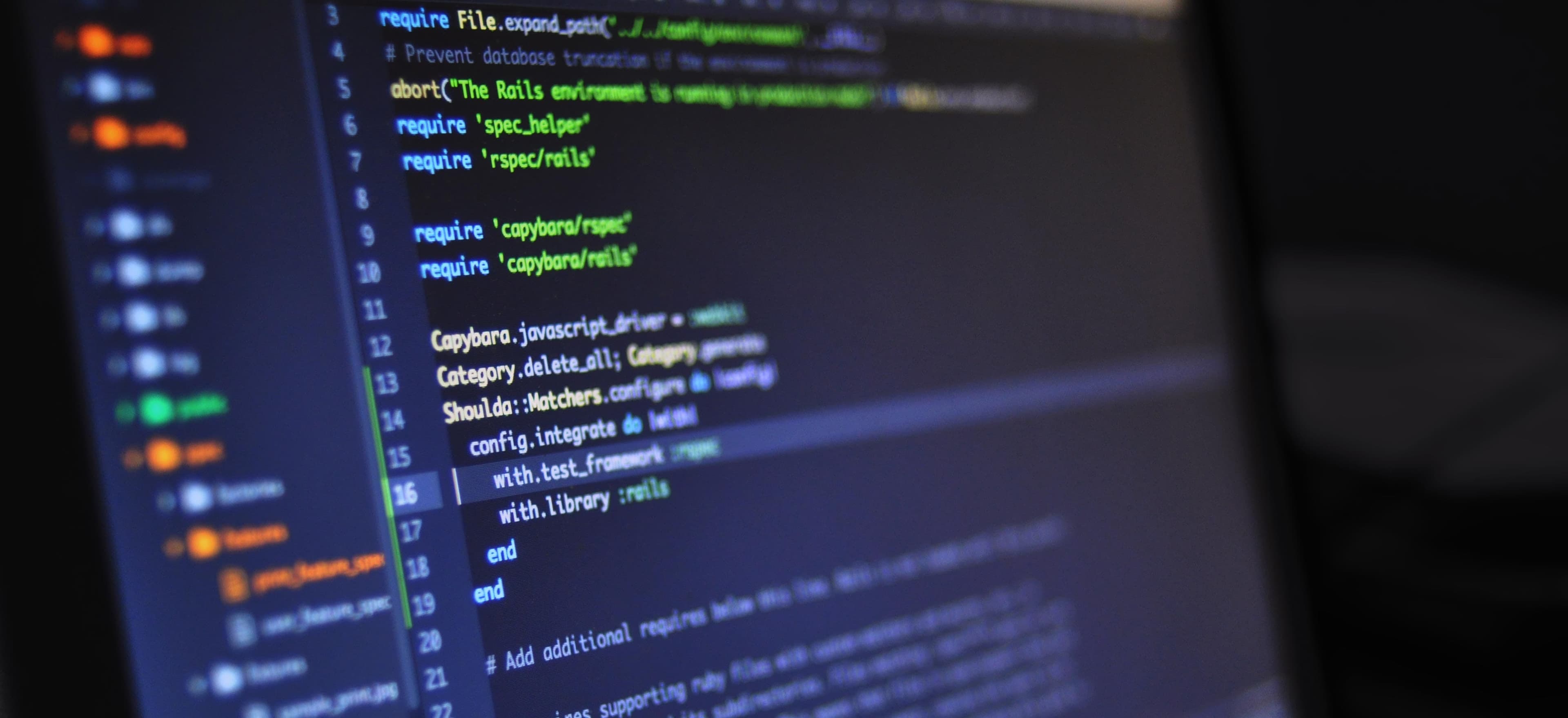
- Published on
Troubleshooting Spring MockMvc: JSON Response Issues
When it comes to testing Spring applications, MockMvc serves as an invaluable tool. It helps simulate HTTP requests to your controllers without the need to spin up an entire server. However, developers often encounter JSON response issues while using MockMvc. This blog post will walk you through common problems, effective troubleshooting techniques, and best practices to ensure your JSON responses behave as expected.
Understanding MockMvc
Before diving into troubleshooting, let's quickly recap what MockMvc is. MockMvc allows you to test your Spring MVC controllers by performing requests and verifying responses in a controlled environment. It seamlessly integrates with the Spring test framework, making it robust and flexible.
Example Setup of MockMvc:
@RunWith(SpringRunner.class)
@SpringBootTest
@AutoConfigureMockMvc
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetUser() throws Exception {
mockMvc.perform(get("/users/{id}", 1))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON))
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
Explanation:
@SpringBootTest
: This annotation tells Spring Boot to look for a configuration class in the package and create an application context.@AutoConfigureMockMvc
: This initializes theMockMvc
instance.mockMvc.perform(...)
: This performs the actual request.andExpect(...)
: These methods define expectations for the response.
Common JSON Response Issues
Despite MockMvc's efficacy, several issues can arise:
- Incorrect Content Type
- Malformed JSON
- Unexpected HTTP Status Codes
- Empty Response Body
- Incorrect JSON Structure
Let's talk about these issues in detail.
1. Incorrect Content Type
When testing, often developers expect a JSON response but end up with a different content type. This discrepancy can lead to misconceived assumptions about the result.
Troubleshooting Steps:
- Make sure your controller is producing the
application/json
content type. Use the@RequestMapping
or@GetMapping
annotation withproduces
specified.
@GetMapping(value = "/users/{id}", produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
2. Malformed JSON
If your response is not well-formed JSON, it might cause issues during testing. This can happen due to missing fields or incorrect serialization of your objects.
Troubleshooting Steps:
- Check your entity classes and ensure they have the correct getters and setters. Use libraries like Jackson that handle JSON serialization effectively. Annotate your POJOs accordingly.
@JsonProperty("name")
private String name;
Why This Matters: Jackson uses these annotations to serialize your objects into JSON properly. If fields are not mapped correctly, your JSON becomes malformed.
3. Unexpected HTTP Status Codes
Receiving an unexpected HTTP status code can be frustrating. MockMvc tests expect a specific status based on your endpoint's logic.
Troubleshooting Steps:
- Ensure your business logic returns the correct status. For instance, if an entity is not found, make sure to return a 404 Not Found status.
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
if (user == null) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).build();
}
return ResponseEntity.ok(user);
}
4. Empty Response Body
An empty JSON response body can lead to confusion. If you're expecting data but getting back {}
, there could be multiple underlying reasons.
Troubleshooting Steps:
- Validate that the service layer is returning an entity. Adding debug logs can help identify where data retrieval fails.
Logging Example:
logger.debug("User found: {}", user);
5. Incorrect JSON Structure
Your test might expect certain fields in specific formats. If the structure does not match, your test will fail.
Troubleshooting Steps:
- Validate the response using tools like JSON Schema Validator. Ensure that your DTOs and entity classes are correctly modeled to match the expectations.
Testing for JSON Structure:
.andExpect(jsonPath("$.id").value(1))
.andExpect(jsonPath("$.email").exists());
Additional Testing Strategies
- JSON Assertions: Using libraries like JsonPath can help assert nested fields in your JSON responses.
- Mock Services: Use Mockito to mock dependencies. This ensures your tests focus on the controller logic only, isolating them from service layer issues.
Best Practices
-
Use DTOs: Always convert your entities to Data Transfer Objects (DTOs) when responding with JSON. This prevents exposing sensitive data and allows you to control the API response structure.
-
Error Handling: Implement a global exception handler. This way, your API will return consistent error responses. Use
@ControllerAdvice
together with@ExceptionHandler
.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<ErrorResponse> handleUserNotFound(UserNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(ex.getMessage(), HttpStatus.NOT_FOUND);
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
}
- Use Spring Profiles: Utilize different profiles during testing to mimic various environments. This allows for better testing coverage across different scenarios.
The Last Word
Spring MockMvc is a robust tool for testing MVC controllers, but developers must be vigilant and thorough in their approach. By understanding and diagnosing common JSON response issues, you can maintain high code quality and ensure that your APIs are functioning as intended.
Resources
- Spring MockMvc Documentation
- Building RESTful Web Services with Spring Boot
- Jackson JSON Documentation
By following the guidelines laid out in this post, you'll be well-equipped to troubleshoot and resolve JSON response issues with Spring MockMvc. Happy coding!
Checkout our other articles