Avoiding Common Pitfalls in Spring Boot Cache Configuration
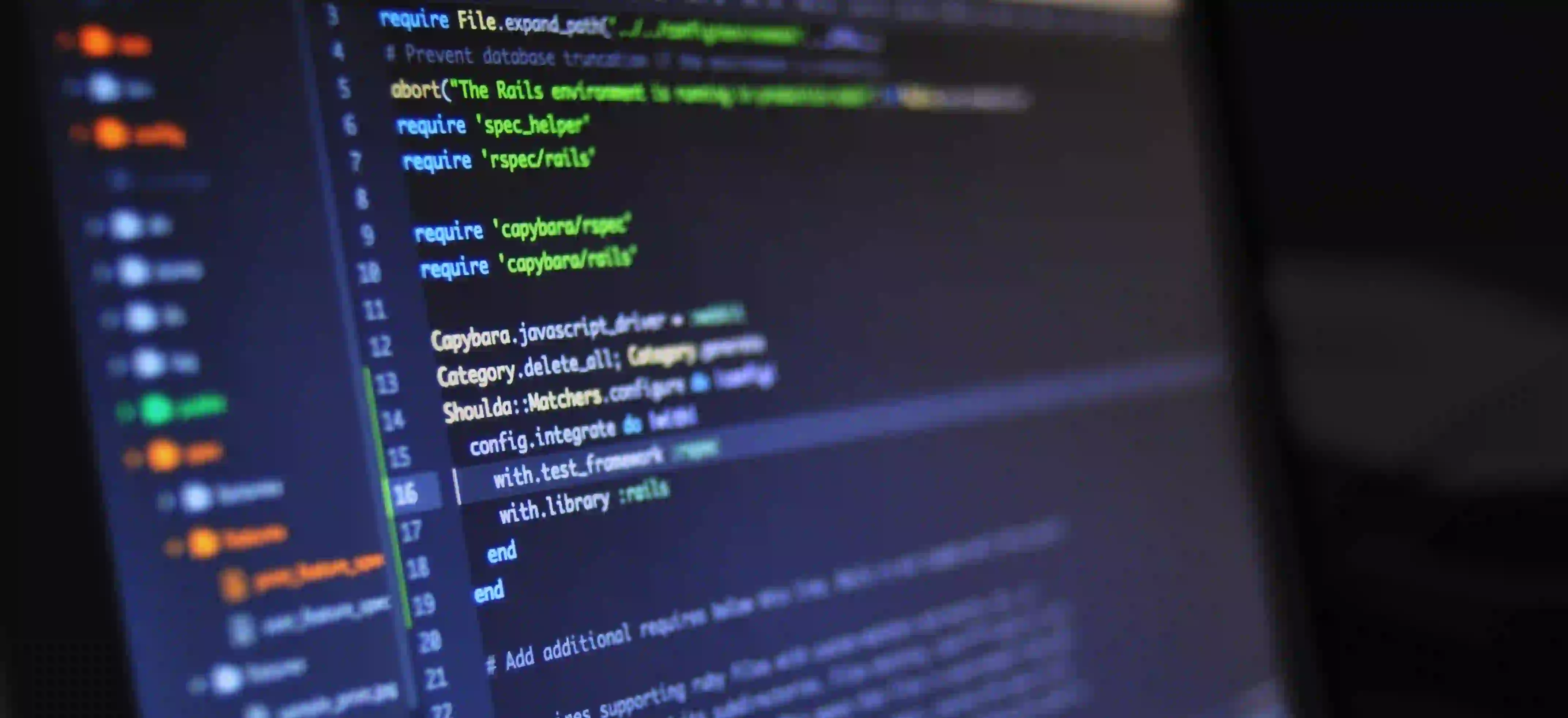
Avoiding Common Pitfalls in Spring Boot Cache Configuration
Spring Boot simplifies application development immensely, especially when it comes to caching. However, with great power comes great responsibility; improper configuration can lead to performance issues, unexpected behavior, or even application failures. This blog post will discuss common pitfalls in Spring Boot cache configuration and how to avoid them.
What is Caching?
Caching is the process of storing frequently accessed data in a temporary storage area to reduce latency and improve performance. In a Spring Boot application, caching can significantly enhance response times and reduce database load, which is crucial in microservices or high-load environments.
Why Use Caching?
- Improved Performance: By saving expensive operations (like database queries), the application can respond faster to user requests.
- Reduced Load: Caching prevents the application from repeatedly querying data sources, reducing overall load on servers.
- Enhancing User Experience: Faster response times lead to better user satisfaction.
Spring Boot Caching Setup
Before diving into pitfalls, let's set up a basic caching environment using Spring Boot.
Basic Configuration
To get started, we can create a simple Spring Boot application. We will use an in-memory cache like ConcurrentHashMap with the @EnableCaching
annotation.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.annotation.EnableCaching;
@SpringBootApplication
@EnableCaching
public class CacheApplication {
public static void main(String[] args) {
SpringApplication.run(CacheApplication.class, args);
}
}
Simple Service with Caching
Here's a simple service that demonstrates caching:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class MathService {
@Cacheable("squareCache")
public int square(int number) {
simulateSlowService(); // Simulate a delay
return number * number;
}
// Simulating a slow service
private void simulateSlowService() {
try {
Thread.sleep(3000); // 3-second delay
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
}
}
In this service, the square
method is annotated with @Cacheable
, which instructs Spring to cache the result of the method. The next time the method is called with the same parameter, the cached result will be returned, significantly reducing the response time.
Common Pitfalls in Cache Configuration
Now that we've set up the basic caching, let's dive into some common pitfalls that developers encounter.
1. Not Defining Cache Regions
One key aspect of cache is the ability to define different cache regions for different types of data. Failing to do this can lead to inefficient memory usage and performance bottlenecks.
Avoid it by defining cache regions:
spring:
cache:
cache-names: squareCache # Define custom cache names
2. Ignoring Cache Evictions
Caching is not just about storing data; it’s also about keeping it relevant. If cached data becomes stale but is not evicted, it can lead to serving incorrect data.
Use @CacheEvict
to address this:
import org.springframework.cache.annotation.CacheEvict;
@CacheEvict(value = "squareCache", allEntries = true)
public void clearCache() {
// Logic to clear cache
}
In this example, calling the clearCache
method will remove all entries in the squareCache
, ensuring the next call to square
fetches fresh data.
3. Not Handling Cache Fill Rate
Not monitoring the fill rate of your caches can lead to memory leaks. If your application has a high cache hit rate but the cache memory is not managed properly, it can consume all available memory.
Monitor Cache Usage:
Using tools such as Spring Actuator can help you monitor cache usage. Add the following dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Then, expose cache metrics via Actuator endpoints:
management:
endpoints:
web:
exposure:
include: "*"
4. Cache Not Invalidating on Changes
When the underlying data changes, the cache must be invalidated. Failing to do this means that users will continue to get stale data.
To invalidate a cache, you'll want to use:
@CachePut(value = "squareCache", key = "#number")
public int updateSquare(int number) {
return square(number); // Cache is updated with fresh data
}
Using @CachePut
allows you to update an existing cache without needing to clear it entirely.
5. Caching Too Much
Another pitfall is over-caching. Caching every method in your application may lead to increased memory usage and can make your application slow if the cache becomes large.
Cache Only What’s Necessary:
Identify frequently accessed data and cache those select methods rather than indiscriminately caching everything. This can be done by scrutinizing logs or metrics to find which methods have high data-access rates.
6. Not Utilizing Caching Annotations Properly
Simple mistakes with caching annotations can lead to unintended consequences. For example, placing @Cacheable
on a non-public
method will not produce the desired caching effect since Spring AOP (Aspect-Oriented Programming) relies on proxies created for public methods.
Correct Usage of Annotations:
Make sure all caching annotations (like @Cacheable
, @CacheEvict
, and @CachePut
) are applied to public methods.
@Override
@Cacheable("squareCache")
public int square(int number) {
// ...
}
7. Poor Cache Key Design
Improper cache key design can lead to cache misses (where data is not found in cache), which negates the performance benefits of caching.
Keep in mind that Spring generates cache keys based on method parameters by default. To customize this, you can use SpEL (Spring Expression Language).
@Cacheable(value = "squareCache", key = "#number")
public int square(int number) {
//...
}
Customizing your cache keys can prevent collisions and improve cache efficiency.
Closing the Chapter
Caching can drastically improve the performance of your Spring Boot applications, but improper configuration can cause a variety of issues. By avoiding common pitfalls like neglecting cache regions, not monitoring cache usage, and over-caching, you’ll be on your way to a more performant application.
For further reading on caching strategies in Spring, check out the Spring Documentation.
By following these best practices, you can maximize the benefits of caching and ensure your application runs like a well-oiled machine. For additional insights on Spring Boot best practices, feel free to explore more articles on Baeldung. Happy coding!