Overcoming Batching Challenges in Neo4j Java REST API
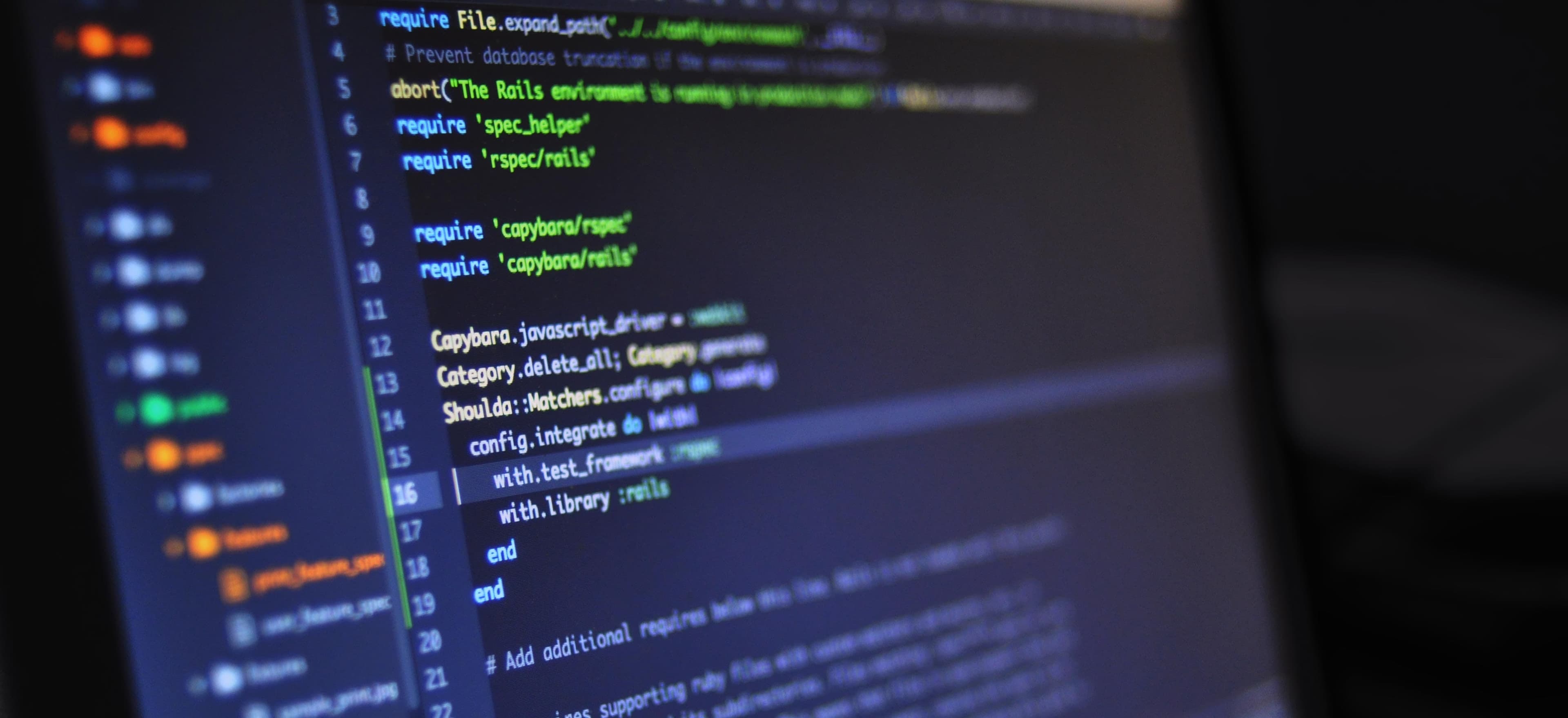
- Published on
Overcoming Batching Challenges in Neo4j Java REST API
The Neo4j database is an open-source graph database known for its robust graph processing capabilities. It allows developers to leverage relationships among data points, making it an excellent choice for applications relying on complex data interactions. However, when you're working with large datasets or multiple transactions, you may face "batching" challenges. This blog post aims to address those challenges within the context of the Neo4j Java REST API.
By overcoming these batching hurdles, you’ll optimize database operations, reduce transaction overhead, and ensure smoother interactions with your Neo4j database.
Understanding Batching in Neo4j
Batching involves grouping multiple operations into single transactions for efficiency. When working with large datasets, executing individual operations can lead to significant performance degradation. Batching minimizes communication overhead between your application and database, leading to:
- Reduced Network Latency: Fewer separate requests mean less round-trip time to the server.
- Improved Throughput: Instead of executing operations one by one, multiple can be executed in a single call.
- Resource Management: Batching helps manage resources like memory and CPU more effectively.
Setting Up Neo4j with Java
Before diving into batching, let’s set up the Neo4j database with a Java application using the REST API. If you haven’t already, you will need to include the following dependencies in your Maven pom.xml
:
<dependency>
<groupId>org.neo4j</groupId>
<artifactId>neo4j-java-driver</artifactId>
<version>4.4.6</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
Basic Connection Setup
Here is a simple setup for connecting to your Neo4j database:
import org.neo4j.driver.AuthTokens;
import org.neo4j.driver.GraphDatabase;
import org.neo4j.driver.Session;
public class Neo4jConnection {
private final String uri = "bolt://localhost:7687";
private final String user = "neo4j";
private final String password = "password";
public Session createSession() {
return GraphDatabase.driver(uri, AuthTokens.basic(user, password)).session();
}
}
Why This Code?
- Encapsulation: Wrapping the connection in a class promotes better organization and encapsulation.
- Security: The use of
AuthTokens.basic
secures your credentials while establishing a connection. - Flexibility: Changing connection parameters requires minimal changes in the codebase.
Batching Operations
Now that we have a connection set up, let’s address how to implement batching effectively.
A Basic Batching Example
Consider that you want to create multiple nodes in the Neo4j database. Instead of executing each node creation individually, you can batch the requests:
import org.neo4j.driver.Session;
import org.neo4j.driver.TransactionWork;
import java.util.List;
public class BatchInsert {
private Neo4jConnection neo4jConnection;
public BatchInsert() {
this.neo4jConnection = new Neo4jConnection();
}
public void batchInsertNodes(List<String> nodeNames) {
try (Session session = neo4jConnection.createSession()) {
session.writeTransaction(new TransactionWork<Void>() {
@Override
public Void execute(org.neo4j.driver.Transaction tx) {
for (String nodeName : nodeNames) {
tx.run("CREATE (n:Node {name: $name})",
org.neo4j.driver.Values.parameters("name", nodeName));
}
return null;
}
});
}
}
}
Why This Approach?
- Transaction Management: Utilizing a transaction to group operations allows the operation to be atomic. If one operation fails, all operations can be rolled back.
- Streamlining: By iterating through the collection of node names, we keep the code clean and efficient.
- Error Handling: The
try-with-resources
statement ensures that resources are freed properly after usage.
Advanced Batching Techniques
While the above example illustrates basic batching, Neo4j allows for even more sophisticated batch processing strategies.
Using APOC Procedures for Custom Batching
Neo4j's APOC library (Awesome Procedures on Cypher) allows you to perform complex tasks that the standard Cypher language may not easily support. This library includes procedures for batching data imports, which could significantly improve your processing strategy.
To use APOC for batching, you'd follow these steps:
- Ensure that the APOC library is installed in your Neo4j instance.
- Modify your Java code to utilize APOC procedures for batch creation.
public void batchInsertUsingAPOC(List<String> nodeNames) {
try (Session session = neo4jConnection.createSession()) {
StringBuilder cypher = new StringBuilder("CALL apoc.create.node(['Node'], $props) ");
for (String nodeName : nodeNames) {
cypher.append(" YIELD node WITH node ");
cypher.append(" SET node.name = $name ");
}
session.writeTransaction(tx ->
tx.run(cypher.toString(),
org.neo4j.driver.Values.parameters("name", nodeNames.get(0))));
}
}
Benefits of Using APOC
- Efficiency: APOC procedures are expressly designed for high performance, given that they can process large datasets in even fewer queries.
- Flexibility: With APOC, adding complex logic to your batch processes becomes much easier and maintains readability.
- Advanced Features: Beyond batching, APOC provides additional capabilities, such as data import/export, which can be vital in data migrations.
Monitoring and Testing
When implementing batching strategies, it is crucial to monitor your transactions. Excessive batching can lead to transaction timeouts or memory issues. Implementing logging can help identify bottlenecks in your operations:
import java.util.logging.Logger;
public static void logBatchStatus(String status) {
Logger logger = Logger.getLogger("Neo4jBatchLogger");
logger.info("Batch Operation Status: " + status);
}
A Final Look
Optimizing batching in Neo4j using the Java REST API requires a mix of strategic design, smart code organization, and possibly even advanced third-party procedures like APOC. You should continuously monitor and analyze your application’s performance to ensure efficient batch operations.
By following the strategies outlined in this blog post, you can mitigate problems related to batching, streamline your database interactions, and ultimately enhance the performance of your graph database applications.
Feel free to explore the documentation for further insights:
- Neo4j Java Driver Documentation
- APOC Documentation
When batching effectively, you're not just improving application performance; you’re ensuring a better experience for your end-users. Taking the time to refine these processes will pay off significantly in both the short and long term. Happy coding!
Checkout our other articles