Common Pitfalls in Spring MVC 3 with Apache Tiles
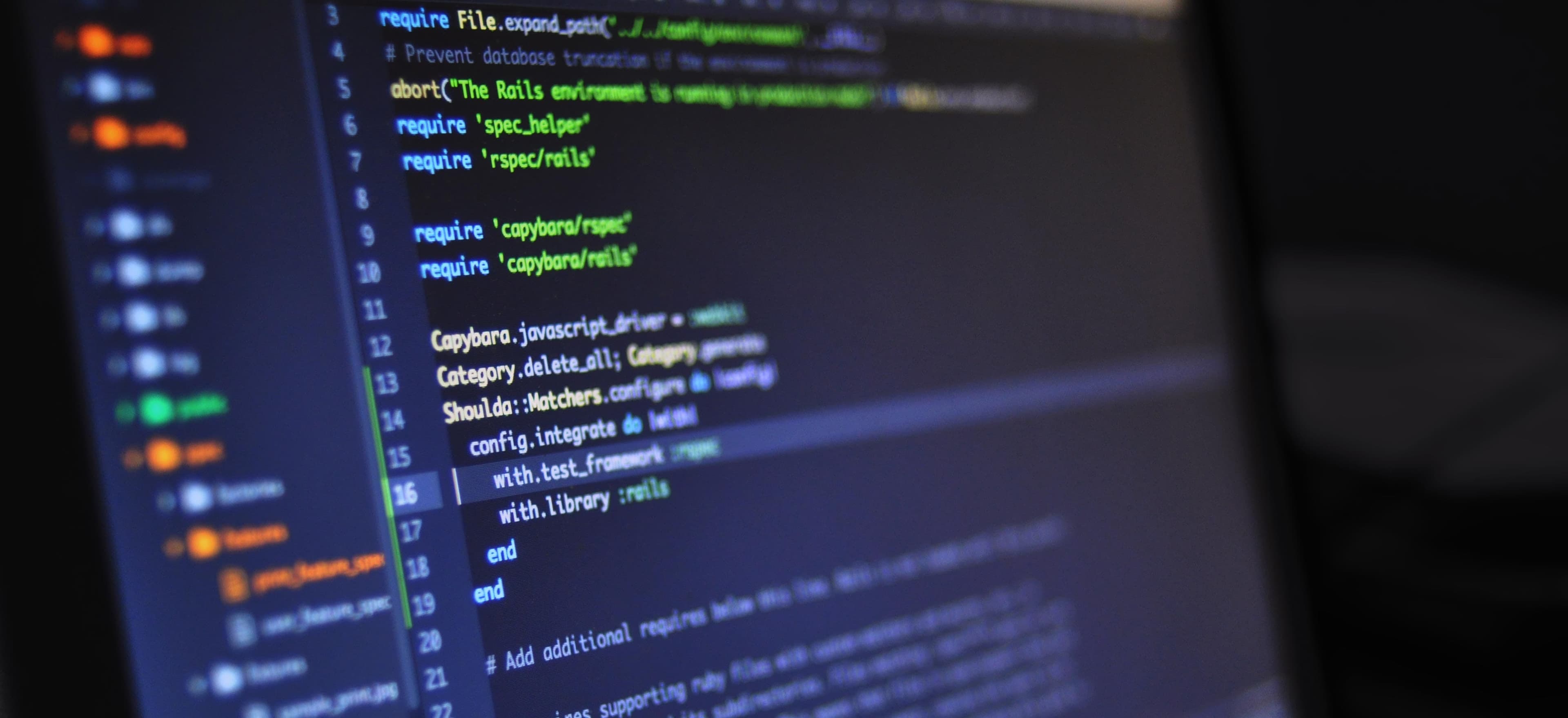
- Published on
Common Pitfalls in Spring MVC 3 with Apache Tiles
Spring MVC, a popular framework for building Java web applications, has established itself as a versatile tool in the developer's toolkit. When combined with Apache Tiles for view management, the potential for creating reusable and consistently styled web pages is enhanced dramatically. However, navigating the complexities of these technologies can lead to several pitfalls. This blog post will discuss common challenges developers face when using Spring MVC 3 with Apache Tiles and provide strategies to overcome them.
Understanding Spring MVC and Apache Tiles
Before delving into common pitfalls, it is essential to grasp the fundamentals of both technologies.
Spring MVC
Spring MVC allows developers to create web applications using the Model-View-Controller pattern, promoting separation of concerns and enhancing testability. Its primary components include:
- Controllers: Handle incoming requests and return models to be rendered by the view.
- Models: Represent the data and business logic of the application.
- Views: Render model data, usually in the form of HTML.
Apache Tiles
Apache Tiles is a templating framework that helps in building composite views. It promotes reuse by managing page layouts and defining views in a modular way. By utilizing Tiles with Spring MVC, developers can achieve a consistent page structure, reducing redundancy.
Common Pitfalls
1. Misconfiguration of View Resolvers
Issue:
A frequent issue among developers is improper configuration of the view resolvers in the web.xml
or Spring configuration files. If the Tiles view resolver isn't set up correctly, the application may fail to render views as intended.
Solution:
Ensure that you configure the Tiles view resolver along with the appropriate prefix and suffix.
<bean class="org.springframework.web.servlet.view.UrlBasedViewResolver">
<property name="viewClass" value="org.springframework.web.servlet.view.tiles3.TilesView"/>
</bean>
<bean class="org.apache.tiles.impl.basic.BasicTilesConfigurer">
<property name="definitionsConfig" value="/WEB-INF/tiles-defs.xml"/>
</bean>
Why?
Correct configuration ensures that your views are resolved according to the Tiles definitions you've established, rendering them accurately without causing 404 errors.
2. Incorrect Tiles Definitions
Issue:
Another common challenge arises from errors in the Tiles definitions file. This could include wrong view names, missing templates, or incorrect paths.
Solution:
Here's an example of a correctly structured tiles-defs.xml
file:
<tiles-definitions>
<definition name="home" template="/WEB-INF/layouts/default.jsp">
<put-attribute name="title" value="Welcome Page"/>
<put-attribute name="content" value="/WEB-INF/views/home.jsp"/>
</definition>
</tiles-definitions>
Why?
Each view needs to correctly reference the template and attributes. Any typo could lead to a view not being rendered, displaying an empty page or a server error.
3. Lack of Proper Error Handling
Issue:
Failing to implement proper error handling can lead to the application crashing or displaying unfriendly error messages to users.
Solution:
Make use of Spring’s exception handling features to provide a better user experience. For instance, using an @ControllerAdvice
can centralize error handling.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public String handleException(Exception ex, Model model) {
model.addAttribute("errorMessage", ex.getMessage());
return "error"; // References an error.jsp view
}
}
Why?
This setup allows you to manage exceptions gracefully without exposing sensitive technical details to the end-user.
4. Overcomplicating Tiles Definitions
Issue:
Sometimes developers complicate Tiles definitions unnecessarily by nesting definitions deeply or relying on too many composite templates, leading to hard-to-troubleshoot issues and a lack of performance.
Solution:
Maintain a balance in your Tiles design. Prefer simple, flat definitions that are easy to manage. Use composition only when truly necessary.
For example, instead of creating multiple layers, opt for straightforward definitions that cover all required attributes directly related to the page.
Why?
Simplified Tiles definitions are more performant and easier to debug. They also reduce the potential for circular references, which may cause the application to crash.
5. Ignoring Spring’s Resource Handling
Issue:
Developers often overlook Spring’s resource handling features when working with stylesheets, JavaScript, or images. This may lead to broken links or failed asset loading, impacting the user interface.
Solution:
Make use of the mvc:resources
tag in your XML configuration or the @EnableWebMvc
annotation, specifying location with proper resource handlers.
<mvc:resources mapping="/static/**" location="/static/"/>
Why?
Proper resource handling ensures that all static files are served correctly, improving overall performance and user experience.
6. Caching Issues with Apache Tiles
Issue:
Caching can be both a boon and a bane. If caching is not configured correctly, it may lead to the display of outdated views.
Solution:
Configure Tiles' caching settings appropriately to serve dynamic content without holding onto old data.
<bean class="org.apache.tiles.impl.basic.BasicTilesConfigurer">
<property name="checkRefresh" value="true"/>
</bean>
Why?
Setting checkRefresh
to true ensures that Tiles evaluates whether to refresh cached views on each request. This is particularly useful for dynamic content.
Closing the Chapter
Integrating Spring MVC with Apache Tiles offers a powerful approach to building modern web applications. However, the potential for problems can arise from configuration missteps, complex Tiles definitions, error handling lapses, and resource management oversight.
By recognizing and addressing these common pitfalls, developers can enhance their application’s performance, user experience, and maintainability. For further reading on Spring MVC best practices, you might want to check out the Spring Documentation and Apache Tiles Official Documentation.
By following the guidance outlined in this blog post, you can navigate confidently in the Spring MVC and Apache Tiles ecosystem, building robust and flexible Java web applications. Happy coding!
Checkout our other articles