Fix Java App Crashes Due to Missing Fonts
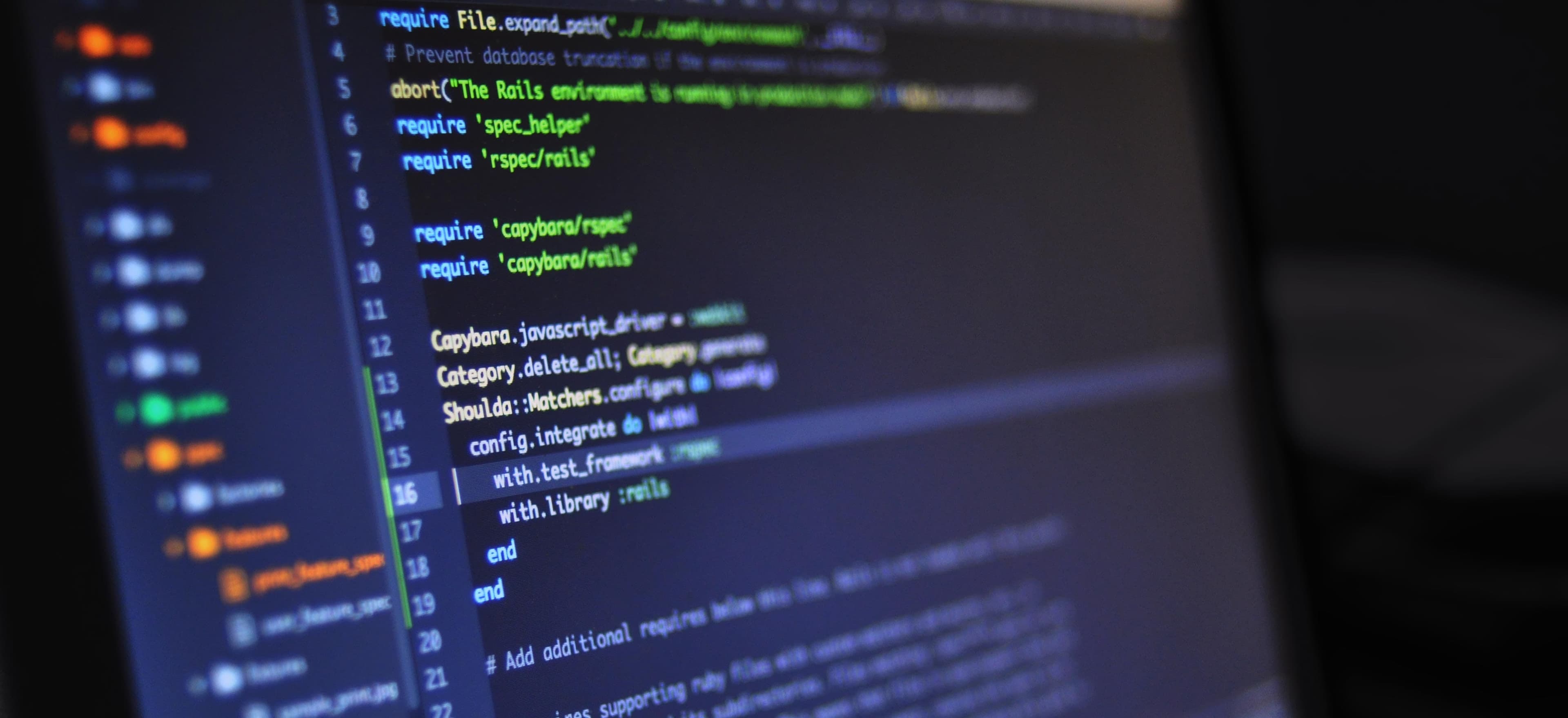
- Published on
Fix Java App Crashes Due to Missing Fonts
Java applications often encounter various issues related to the environment in which they run. One such issue that can lead to unexpected crashes is the absence of necessary font files. This blog post will explore how missing fonts can lead to runtime errors, the importance of handling such failures gracefully, and strategies to fix these problems in your Java applications.
Understanding the Problem
Java uses the Abstract Window Toolkit (AWT) and Swing libraries, which rely on system fonts to render text in graphical user interface (GUI) components. When a required font file is not found, it can lead to exceptions that cause the application to fail. Let's look at some common types of errors you might encounter:
- java.awt.FontFormatException: This occurs when an application attempts to load a non-existent font.
- java.awt.Font: When a font is not available, the default font may not match what your application expects, potentially resulting in visual discrepancies.
Example of a FontException
Consider the following example where an application attempts to load a specific font:
import java.awt.Font;
import java.io.File;
import java.io.IOException;
import javax.swing.*;
public class FontLoader {
public static void main(String[] args) {
try {
Font customFont = Font.createFont(Font.TRUETYPE_FONT, new File("path/to/missing/font.ttf"));
GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment();
ge.registerFont(customFont);
} catch (FontFormatException | IOException e) {
System.err.println("Error: Could not load font. " + e.getMessage());
e.printStackTrace();
}
}
}
In this example, if "font.ttf" is missing, the program will throw a FontFormatException
or IOException
, causing it to crash unless handled properly.
Why Fonts Matter in Your Java Application
Fonts play a crucial role in the user experience:
- Aesthetics: Custom fonts can greatly enhance the visual appeal of your application.
- Readability: Some fonts are more readable than others depending on the context. Missing fonts can lead to unreadable text.
- Brand Identity: Consistent use of specific fonts can strengthen your brand image.
Given these factors, it is crucial to handle font loading gracefully to ensure your application remains robust.
Strategies to Fix Missing Font Crashes
Let's dive into various methods to handle font loading issues effectively.
1. Use a Fallback Font
When your primary font fails to load, it's a good practice to use a fallback. You can layer multiple fonts and set the application to revert to the next available font:
public Font loadFont(String fontPath) {
Font font;
try {
font = Font.createFont(Font.TRUETYPE_FONT, new File(fontPath));
GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment();
ge.registerFont(font);
} catch (FontFormatException | IOException e) {
System.err.println("Error loading font: " + e.getMessage());
font = new Font("Serif", Font.PLAIN, 12); // Fallback font
}
return font;
}
Dry-running this method ensures that if the custom font fails to load, a standard font will be used, reducing the chance of a crash.
2. Check for Font Availability
Before loading a font, check if it’s available in the GraphicsEnvironment
. This proactive check prevents runtime errors:
public boolean isFontAvailable(String fontName) {
String[] fontFamilies = GraphicsEnvironment.getLocalGraphicsEnvironment().getAvailableFontFamilyNames();
for (String family : fontFamilies) {
if (family.equalsIgnoreCase(fontName)) {
return true;
}
}
return false;
}
public void setupFont(String fontName) {
if (isFontAvailable(fontName)) {
try {
// Load custom font
} catch (Exception e) {
// handle error
}
} else {
System.out.println("Font not available. Fallback will be used.");
// Use fallback font
}
}
In this method, you can ensure that your application does not leap blindly into loading a font that may not exist, safeguarding against failures.
3. Bundle Fonts with Your Application
To eliminate dependency on the user’s system fonts, you can bundle required fonts with your application JAR file. Store your font files in a resource folder and access them using a class loader:
public Font loadFontFromResources(String fontResource) {
try (InputStream fontStream = getClass().getResourceAsStream(fontResource)) {
return Font.createFont(Font.TRUETYPE_FONT, fontStream);
} catch (FontFormatException | IOException e) {
System.err.println("Unable to load font: " + e.getMessage());
return new Font("Serif", Font.PLAIN, 12); // Fallback font
}
}
This method ensures that your application always has access to the required fonts, independent of the user's system configuration.
4. Exception Logging and User Feedback
Besides handling errors, providing useful feedback improves user experience. Implement logging and show user-friendly messages when a font can't be loaded.
try {
// attempt to load font
} catch (FontFormatException | IOException e) {
System.err.println("Font loading failed. " + e.getMessage());
JOptionPane.showMessageDialog(null, "We couldn't load the specified font. Please check your installation.");
}
This gives users a clear idea of why something may not look as expected without overwhelming them with technical jargon.
Lessons Learned
Addressing missing fonts in a Java application is crucial for maintaining stability and ensuring a pleasant user experience. By implementing the strategies discussed above, you can prevent crashes and provide meaningful feedback to users.
Here’s a summary of the techniques covered:
- Use fallback fonts.
- Check for font availability.
- Bundle fonts with your application.
- Implement exception logging and provide user feedback.
By following these guidelines, developers can create resilient Java applications that are less prone to issues related to missing fonts and deliver a better overall user experience. For more great Java resources, consider visiting Oracle's Java Documentation or Java Code Geeks.
Additional Resources
With these strategies and resources, you can enhance your Java applications and reduce the chances of crashes due to missing fonts. Happy coding!
Checkout our other articles