Why Java 8 Streams Can Slow Down Your Code Performance
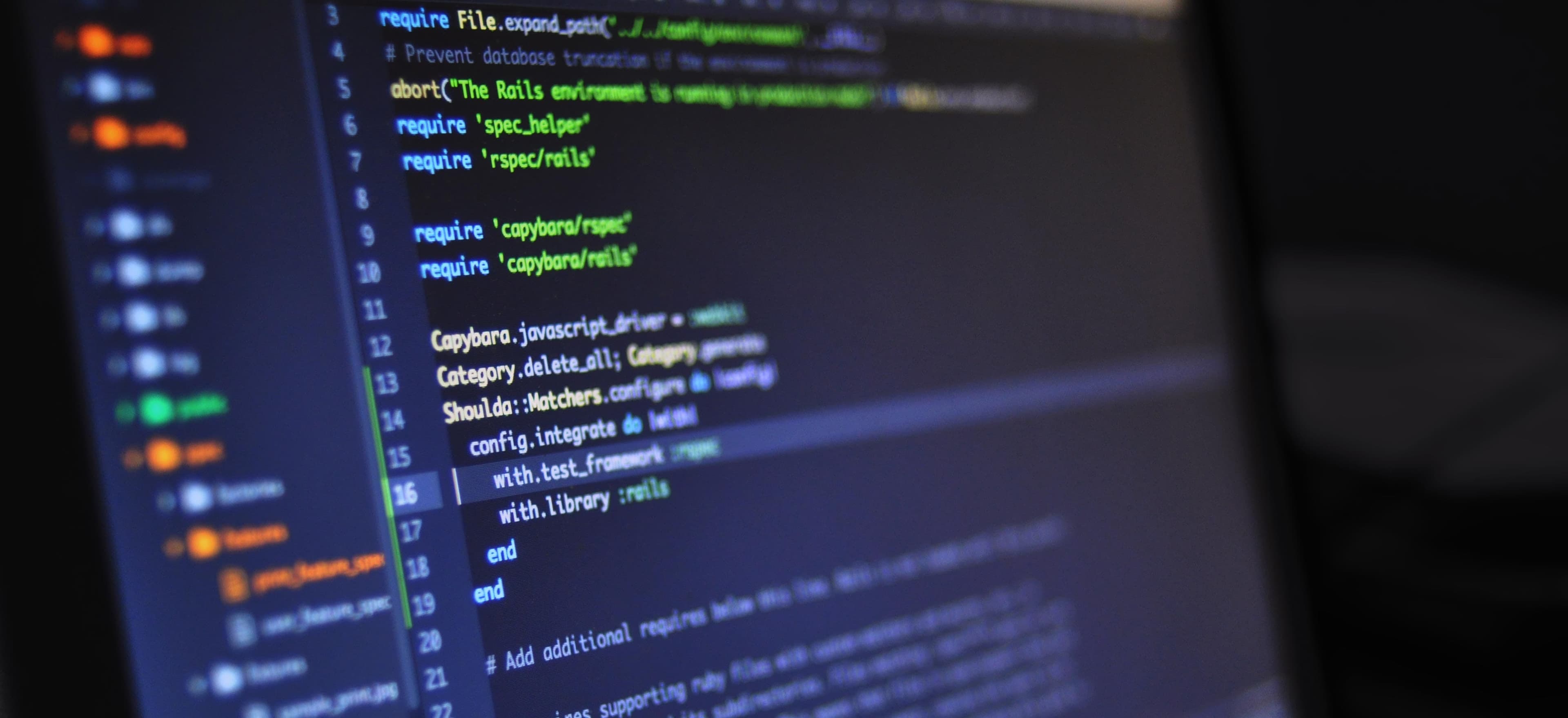
- Published on
Why Java 8 Streams Can Slow Down Your Code Performance
Java 8 marked a significant evolution in the Java programming language with the introduction of Streams, allowing developers to handle sequences of elements in a functional style. While Streams offer several advantages in terms of readability and expressiveness, they can also introduce performance drawbacks if not used judiciously, particularly for large data sets or critical performance applications. In this blog post, we'll explore the common pitfalls associated with Java 8 Streams and provide alternatives for optimizing your code's performance.
The Promise of Streams
Before diving into the potential performance issues, let's highlight what makes Java 8 Streams appealing:
- Conciseness: Streams enable writing less boilerplate code.
- Parallel Processing: Easily switch to parallel executions for collections.
- Lazy Evaluation: Operations are not executed until results are needed.
These advantages encourage developers to embrace Streams for a cleaner codebase.
Here’s a basic example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squares = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
This code snippet is compact and conveys the intent clearly. But the beauty of Streams can quickly turn into a source of performance degradation if you’re not careful.
Common Performance Pitfalls of Java 8 Streams
1. Overhead of Stream Creation
Creating a Stream has its costs. Every time you call .stream()
or .parallelStream()
, you allocate additional objects. For small collections, this overhead can be negligible, but for large data sets repeatedly transformed into Streams, the overhead accumulates.
Optimization Tip: If you are repeatedly processing a collection, consider caching the Stream results or utilizing traditional loops if performance is a concern.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Create a stream and cache results.
List<Integer> squares = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
2. Serialization Overhead
When using Streams over distributed systems or multiple threads where data must be sent over network lines, serialization can introduce latency.
Although Streams can allow parallel processing, if your operation involves complex items or large data sets, the serialization overhead can lead to performance bottlenecks.
Optimization Tip: When possible, keep the processing local to avoid the cost of serialization.
3. Boxing and Unboxing
Using primitive types in Streams incurs additional performance costs due to boxing and unboxing necessary for objects.
For instance, when summing integers using a Stream, beans would need to be converted back and forth between primitive int and Integer object.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream().reduce(0, Integer::sum); // Boxing occurs
Optimization Tip: Use IntStream
, LongStream
, or DoubleStream
for primitive types to avoid this overhead.
int sum = IntStream.range(1, 6).sum(); // No boxing, direct performance
4. Intermediate Operations
Not all Stream operations are created equal. Several intermediate operations can cause multiple passes over the data, negating the performance benefits of Streams.
For example, a chain of filter and map operations can lead to multiple traversals instead of a single pass.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.collect(Collectors.toList()); // Could iterate multiple times
Optimization Tip: Combine operations when possible. Use the peek
method for debugging intermediate values to ensure that you maintain one pass.
5. Performance of Parallel Streams
While Streams can be run in parallel for improved performance, they are not a catch-all solution. The performance gain from parallel processing is realized when the tasks are computationally intensive. For lightweight operations, the overhead of managing tasks in parallel can lead to reduced performance.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> results = numbers.parallelStream()
.map(n -> n * n) // May not benefit from parallel processing
.collect(Collectors.toList());
Optimization Tip: Benchmark performance under various conditions. Use parallel streams judiciously and only when the operations themselves are CPU-bound.
Additional Performance Considerations
-
Avoid Side Effects: Streams should ideally be stateless and no external variables should be modified. Side effects lead to unpredictable performance.
-
Stream Reusability: Streams cannot be reused after a terminal operation has been performed. Always create a new Stream when needed.
-
Sufficient Data Volume: For smaller datasets, traditional loop constructs (for-each loops) might outperform Streams due to the overhead associated with creating Stream objects and executing lambdas.
Wrapping Up
Java 8 Streams provide a robust framework for processing collections with determination and elegance. However, like any powerful feature, they come with potential pitfalls that can negatively impact performance if not managed properly. It is crucial to be mindful about how you leverage Streams in your applications, especially when processing large datasets or in performance-critical sections of your code.
Best Practices to Follow
- Use Streams when working with larger datasets.
- Embrace primitive Streams to avoid boxing costs.
- Measure performance through testing.
- Combine intermediate operations to minimize overhead.
- Use parallel streams cautiously.
- When in doubt, stick to traditional loops for smaller data collections.
By being aware of these performance factors, you can use Java 8 Streams effectively without compromising the efficiency of your code.
Further Reading
By practicing thoughtful implementation and understanding the mechanics behind Java Streams, you can harness their power while minimizing performance drawbacks. Happy Coding!