Troubleshooting Android Logs: Common Errors Explained
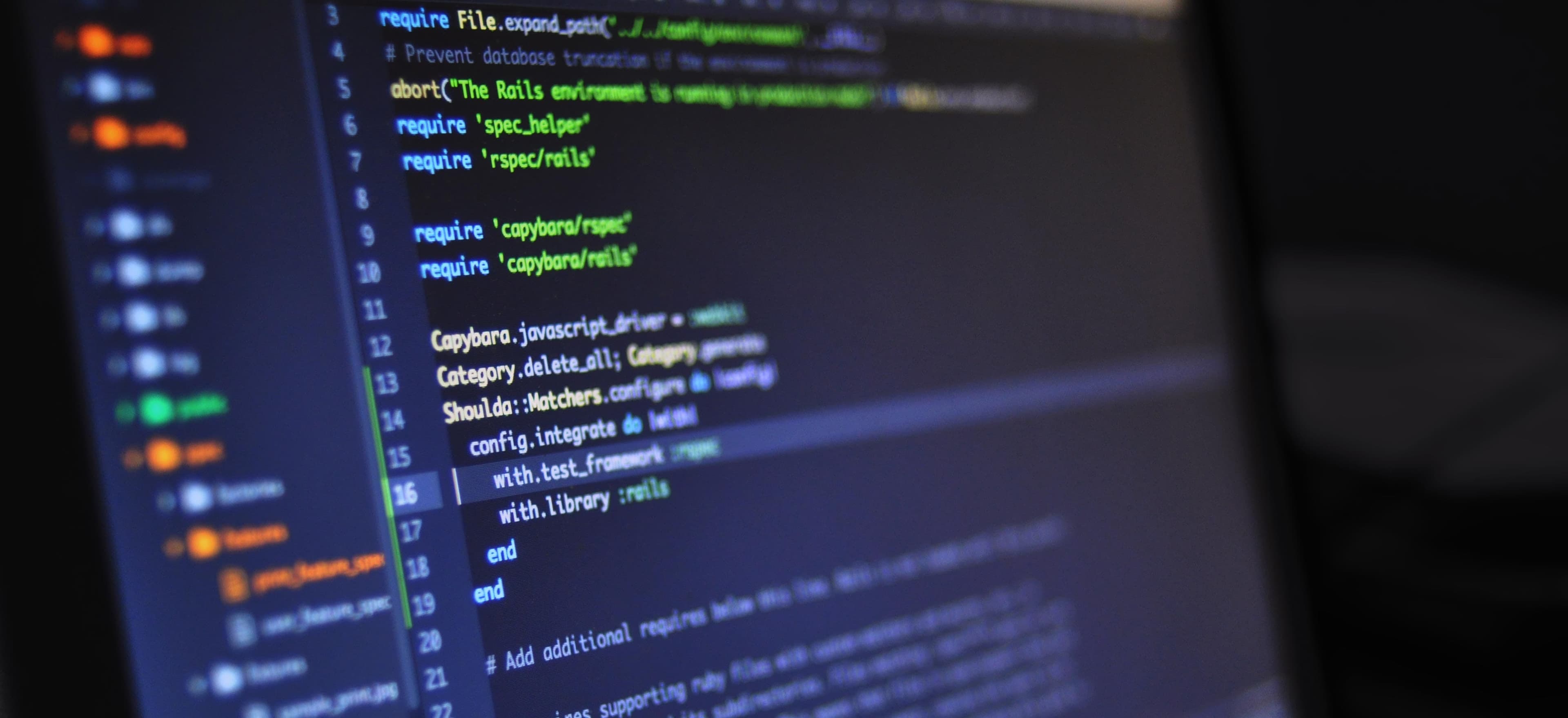
- Published on
Troubleshooting Android Logs: Common Errors Explained
When developing Android applications, one of the most crucial skills for a developer is the ability to effectively troubleshoot issues using logs. Logs provide a window into the application’s behavior and help debug problems occurring on the device. In this article, we will explore common errors that appear in Android logs, understand their implications, and learn how to address them.
Understanding Android Logcat
Before diving into the errors, let’s take a moment to understand what Logcat is and why it is important. Logcat is a command-line tool that displays logs from your Android device or emulator. It can show system messages, stack traces when the device throws an error, and log messages from your applications.
You can access Logcat through Android Studio or use the command line by executing:
adb logcat
This command will output all the logs from the connected device. Understanding these logs can prove invaluable when troubleshooting issues.
Basics of Log Levels
Log messages in Android are categorized into several levels:
- Verbose (V): For detailed messages.
- Debug (D): For debugging messages.
- Info (I): For general informational messages.
- Warning (W): For warning messages.
- Error (E): For error messages.
- Assert (A): For fatal assertions.
Each level indicates the severity of the message, enabling developers to filter logs based on importance.
Common Errors in Android Logs
1. NullPointerException
One of the most common issues developers face in Android is the infamous NullPointerException
. This error typically occurs when the app attempts to access or modify an object or variable that is null
. The log for a NullPointerException
will usually look something like this:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.app, PID: 1234
java.lang.NullPointerException: Attempt to invoke virtual method '...' on a null object reference
How to Fix:
- Check for Null Values: Before accessing any object, ensure it is initialized. Use cautious checks, such as:
if (myObject != null) {
myObject.doSomething();
} else {
Log.e("MyApp", "MyObject is null");
}
- Use Optional: If applicable, consider using Java’s
Optional
class to handle possiblenull
values gracefully.
2. OutOfMemoryError
As apps grow more complex, memory management becomes crucial. An OutOfMemoryError
indicates that your app has exhausted the available memory while trying to allocate a new object. This error may show up as:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.app, PID: 1234
java.lang.OutOfMemoryError: Failed to allocate a 1048576 byte allocation with ...
How to Fix:
- Optimize Bitmap Usage: Bitmaps can consume significant memory. Instead of loading a large bitmap directly, use:
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeResource(getResources(), R.drawable.large_image, options);
options.inSampleSize = calculateInSampleSize(options, reqWidth, reqHeight); // Resample the bitmap
options.inJustDecodeBounds = false;
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.large_image, options);
This code reduces memory usage by loading a scaled-down version of the bitmap.
- Garbage Collection: Manually nullify large objects when they are no longer needed. Calling
System.gc()
triggers garbage collection, though it is not guaranteed to free up memory immediately.
3. NetworkOnMainThreadException
Android does not allow network operations on the main thread. Attempting to make a network call in this thread results in a NetworkOnMainThreadException
. The log entry will look similar to:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.app, PID: 1234
android.os.NetworkOnMainThreadException
How to Fix:
To rectify this, move any network calls to a background thread using:
- AsyncTask (deprecated),
- Thread,
- ExecutorService,
- RxJava, or
- Kotlin Coroutines.
Here’s how you can do it using AsyncTask
:
private class FetchDataTask extends AsyncTask<Void, Void, String> {
protected String doInBackground(Void... params) {
return fetchDataFromServer();
}
protected void onPostExecute(String result) {
// Update UI with the result
}
}
new FetchDataTask().execute();
4. ClassNotFoundException
This exception is thrown when the Java Runtime cannot find a class that it is trying to load. You might see logs like this:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.app, PID: 1234
java.lang.ClassNotFoundException: Didn't find class "com.example.NonExistentClass" on path: ...
How to Fix:
- Verify Class Name: Check for typos or incorrect package naming in your code.
- ProGuard/R8 Configuration: If you are using ProGuard or R8 for code shrinking, ensure that your configuration does not remove necessary classes. You can exclude classes using:
-keep class com.example.NonExistentClass { *; }
- Dependencies: Ensure that all required libraries or modules are included in your project’s dependencies.
5. SecurityException
A SecurityException
occurs when your app tries to perform an operation that the system doesn’t permit without the appropriate permissions. Logs will report it as:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.app, PID: 1234
java.lang.SecurityException: Permission Denial: ...
How to Fix:
- Declare Permissions: Always declare necessary permissions in the
AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
- Runtime Permissions: Since Android 6.0 (API level 23), you must also request permissions at runtime. Example:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, MY_PERMISSIONS_REQUEST_LOCATION);
}
Closing the Chapter
Effective troubleshooting of Android logs is a vital skill for developers. Understanding common error messages allows you to quickly identify and resolve issues within your applications. Remember to utilize log levels, and consistently review your logs in Logcat.
For further reading on mastering Android development, consider checking out Android Developer’s Guide or Advanced Android Development.
By consistently referencing logs and applying the solutions discussed in this article, you will enhance your debugging skills and lead your projects to success. Happy coding!
Checkout our other articles