Mastering Performance: Troubleshooting Java Microbenchmarking
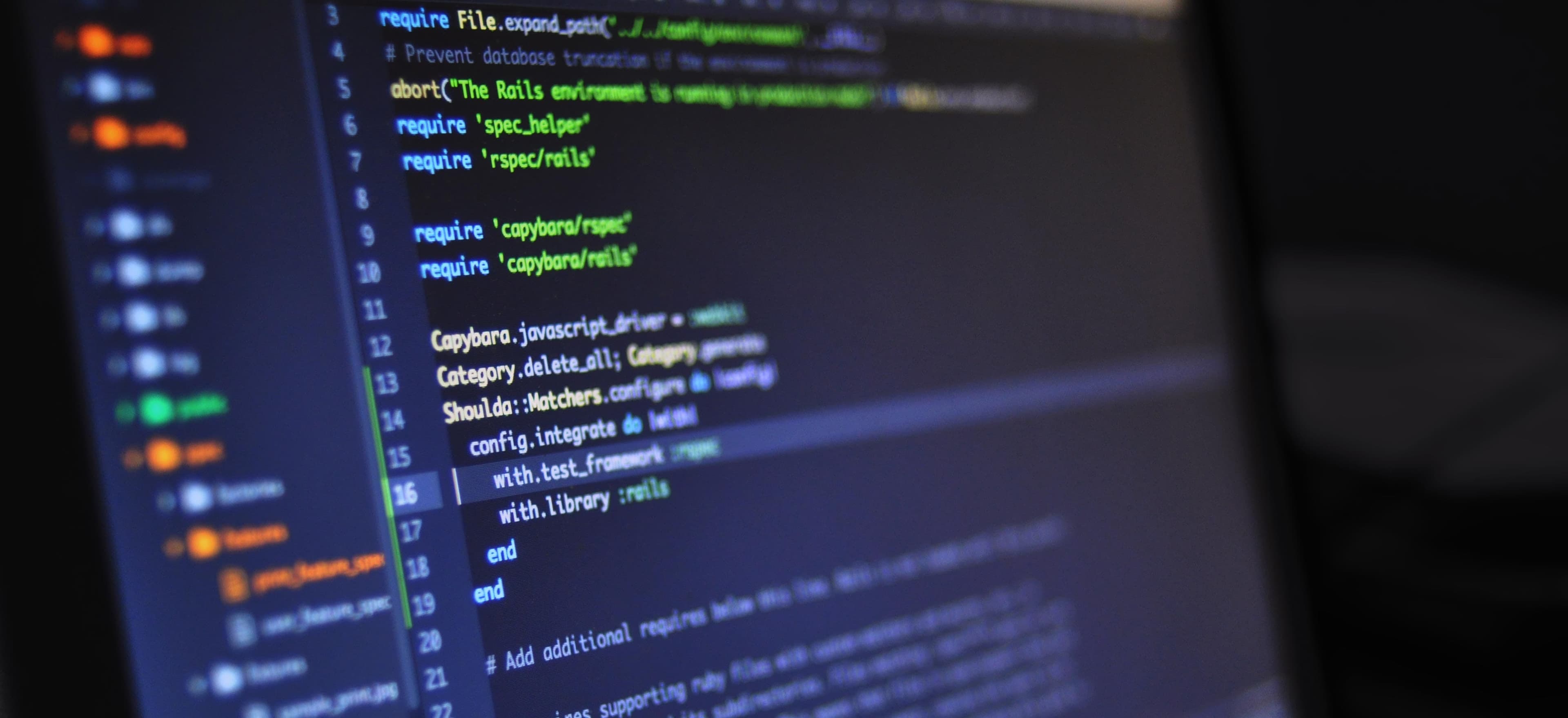
- Published on
Mastering Performance: Troubleshooting Java Microbenchmarking
Microbenchmarking is an essential skill for Java developers aiming to optimize their applications. Accurate performance measurements can lead to improved system efficiency and better resource utilization. However, microbenchmarking is fraught with challenges. In this blog post, we will walk through the intricacies of Java microbenchmarking, the common pitfalls, and how to troubleshoot your benchmarks effectively.
What is Microbenchmarking?
Microbenchmarking refers to the practice of measuring the performance of small sections of code, often a single method or function. The idea is to isolate and measure specific code paths to guide optimization efforts.
Why Is Microbenchmarking Important?
- Performance Validation: Microbenchmarking can help confirm that your optimizations yield the desired performance improvement.
- Informed Decision Making: By measuring benchmarks, data-driven decisions can be made.
- Identify Bottlenecks: It helps in determining if any particular function or operation degrades performance.
Challenges in Java Microbenchmarking
Microbenchmarking might seem straightforward, but several factors can lead to misleading results. Here are some challenges:
- JIT Compilation: The Just-In-Time (JIT) compiler can optimize performance during runtime, altering your benchmarked times.
- Garbage Collection: GC can introduce latencies during method execution affecting timing results.
- Warm-up Time: Code may need to run several times to allow the JVM to optimize.
- Measurement Overhead: The method of measuring time can introduce its own overhead.
- Optimization by the Compiler: Dead code elimination can skew the results.
Java Microbenchmarking Tools
While many tools exist for benchmarking, one of the most respected is Java Microbenchmark Harness (JMH). JMH is specifically designed for this purpose and accommodates the peculiarities of the JVM, addressing the issues mentioned above.
First Steps with JMH
Let's install JMH and create a simple benchmark.
-
Setting up JMH You can add JMH to your Maven project by including the following dependency in your
pom.xml
.<dependency> <groupId>org.openjdk.jmh</groupId> <artifactId>jmh-core</artifactId> <version>1.35</version> <!-- Check Maven repository for the latest version --> </dependency> <dependency> <groupId>org.openjdk.jmh</groupId> <artifactId>jmh-generator-annprocess</artifactId> <version>1.35</version> </dependency>
-
Creating Your First Benchmark
Here's an example of how to create a simple benchmark with JMH.
import org.openjdk.jmh.annotations.*; import java.util.concurrent.TimeUnit; @BenchmarkMode(Mode.AverageTime) @OutputTimeUnit(TimeUnit.MILLISECONDS) @State(Scope.Benchmark) public class ExampleBenchmark { private int[] array; @Setup(Level.Trial) public void setup() { array = new int[10000]; for (int i = 0; i < array.length; i++) { array[i] = i; } } @Benchmark public int sum() { int sum = 0; for (int number : array) { sum += number; } return sum; } }
Breakdown of the Code
- Annotations: JMH uses annotations to define various aspects of the benchmark.
@BenchmarkMode
: Specifies the type of benchmark. Here, we're measuring the average time taken.@OutputTimeUnit
: Defines the time unit for output.@State
: Represents the state of the benchmark. Here, we initialize the data we'll benchmark.@Setup
: Prepares test data before the benchmark begins.
Running the Benchmark
You can run the benchmark from the command line using the Maven plugin or your IDE setup. Make sure to read the generated output closely.
Interpreting Results
Once the benchmark runs successfully, JMH will produce output similar to:
# Run complete. Total time: 00:00:02
Benchmark Mode Cnt Score Error Units
ExampleBenchmark.sum avgt 10 0.001 ± 0.000 ms/op
What Do the Results Mean?
- Score: This tells you how long, on average, the operation took per invocation.
- Error: This gives insight into the variability in your measurements.
- Units:
ms/op
indicates milliseconds per operation.
Common Issues in Microbenchmarking
-
Insufficient Warm-up: Always increase the warm-up iterations if you see high variability in results.
@Warmup(iterations = 5) @Measurement(iterations = 10)
-
Not Taking into Account GC: If GC causes your measurements to fluctuate, you may want to look into GC logging. This can be configured in your JVM options.
-
Too Short Measurement Duration: Make sure that your operation runs long enough so that JIT compilation has a chance to optimize it.
-
Using the Wrong Benchmark Configuration: Misconfigure benchmarks and you may end up measuring something entirely different from what you intended. Always profile in real-work scenarios.
-
Ignoring Averages: Focus overwhelming on averages can be misleading. Consider looking at percentile distributions too for holistic understanding.
Tips for Accurate Benchmarking
- Keep Your Benchmark Code Simple: Avoid adding unnecessary complexity that may skew results.
- Use Multiple Parameters: Experiment with different parameters to find optimum conditions.
- Review Your Results Critically: Don't just accept numbers at face value. Investigate outliers.
To Wrap Things Up
Microbenchmarking in Java is a complex but necessary task that can significantly improve application performance when executed correctly. Using tools like JMH can mitigate common pitfalls and enhance the accuracy of your measurements. As you dive deeper into performance tuning, you will develop a keen intuition for benchmarking nuances.
For further reading on Java performance tuning, please refer to the articles on Java Performance Tuning and Understanding Java Garbage Collection. Happy benchmarking!
Checkout our other articles