Choosing Between Fundamental and Elaborative Refactoring Strategies
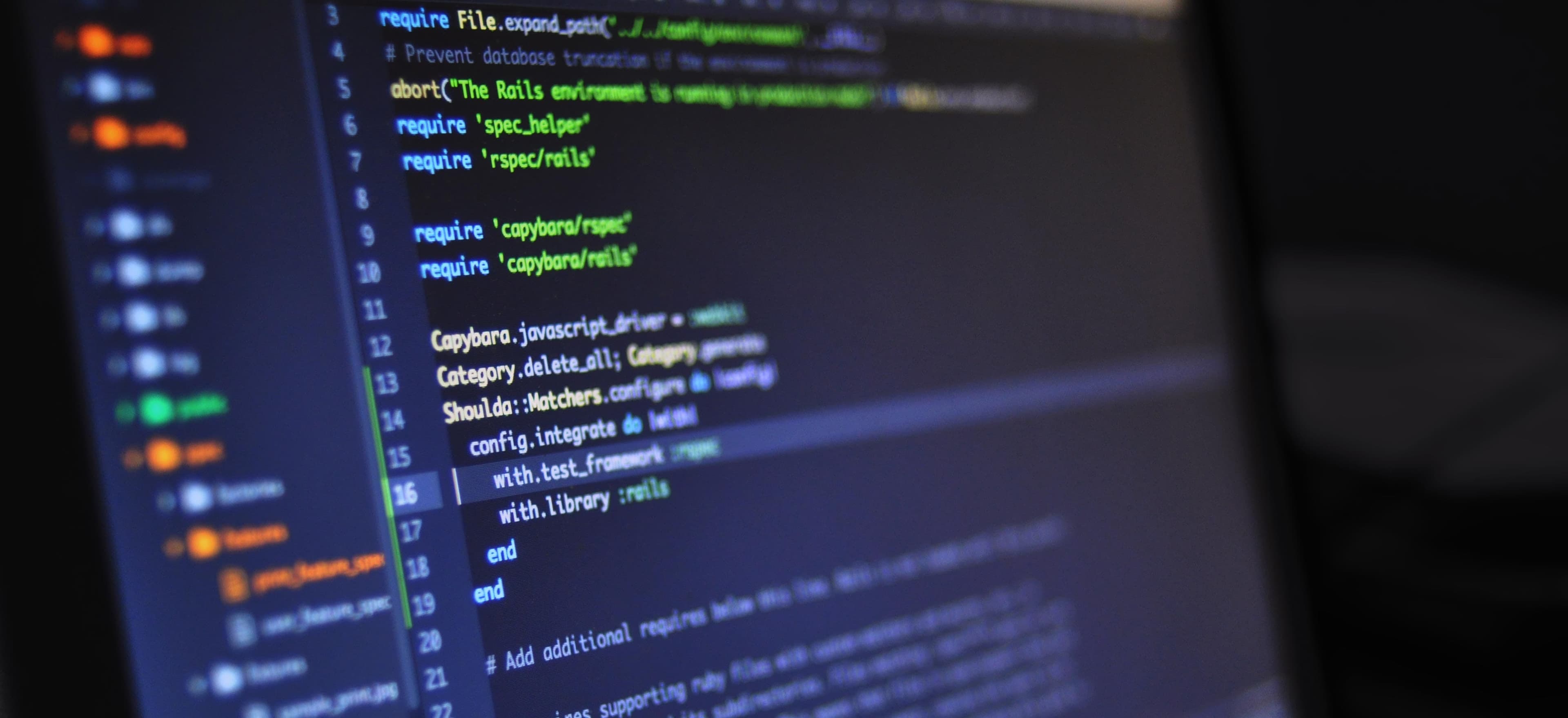
- Published on
Choosing Between Fundamental and Elaborative Refactoring Strategies
Refactoring is a crucial practice in software development that enhances code readability, reduces complexity, and maintains a high level of quality. However, when it comes to refactoring, developers face a significant decision: should they adopt a fundamental or elaborative strategy? In this blog post, we'll explore the differences between these strategies, the scenarios in which each is beneficial, and provide some practical Java code snippets to illustrate the concepts.
Understanding Refactoring
Refactoring involves modifying existing code without changing its external behavior. This process is critical for maintaining a codebase over time as requirements evolve. You can think of refactoring as performing a routine maintenance check on your house: it helps to keep things functioning optimally and looking good.
Why Refactor?
Refactoring offers several advantages:
- Improves Readability: Cleaner code is easier to read and understand.
- Enhances Maintainability: A well-structured codebase is simpler to extend or modify in the future.
- Reduces Technical Debt: Addressing known issues now can avoid larger problems down the line.
Fundamental Refactoring
Fundamental refactoring strategies focus on core or primary problems. These strategies target the most critical sections of the codebase and work to improve fundamental issues causing bugs or performance problems.
Characteristics of Fundamental Refactoring
- Targeted Approach: Concentrates on specific problems.
- Quick Wins: Provides immediate improvements.
- Low Risk: Confined changes reduce the risk of introducing new bugs.
When to Use Fundamental Refactoring
Use fundamental refactoring when you're facing:
- Technical Debt: High-potential areas that are easy to refactor for immediate gains.
- Performance Bottlenecks: Critical paths in the code demanding optimization.
- Complexity Issues: Sections of the code that are overly complicated and creating confusion.
Example of Fundamental Refactoring in Java
Let's examine a simple refactoring scenario that improves a method's readability.
public class Order {
private List<Item> items;
public Order(List<Item> items) {
this.items = items;
}
public double calculateTotalPrice() {
double totalPrice = 0;
for (Item item : items) {
totalPrice += item.getPrice();
}
return totalPrice;
}
}
Why Refactor?
In this case, we want to enhance the method calculateTotalPrice
. The current implementation is acceptable, but it can be improved for readability.
Refactored Code
public class Order {
private List<Item> items;
public Order(List<Item> items) {
this.items = items;
}
public double calculateTotalPrice() {
return items.stream()
.mapToDouble(Item::getPrice)
.sum();
}
}
Commentary
By using Java Streams, we significantly reduced the complexity of the code. This method is more readable and efficiently communicates what it does: it calculates the sum of the prices in items
. This is a prime example of fundamental refactoring — improving readability and maintainability without altering functionality.
Elaborative Refactoring
Elaborative refactoring involves a deep dive into the architecture and design of code. Unlike fundamental strategies, elaborative refactoring addresses the structural aspects of the codebase and seeks long-term improvements.
Characteristics of Elaborative Refactoring
- Comprehensive Approach: A focus on the overall architecture.
- Potentially Extensive Changes: Can lead to a complete overhaul or substantial modifications.
- Higher Risk: Changes can unintentionally affect system behavior.
When to Use Elaborative Refactoring
Choose elaborative refactoring when:
- Poor Design Choices: There’s a need to revise architectural decisions.
- Significant Feature Additions: New features that could benefit from an improved structure.
- Facilitating Scalability: Preparation for a more scalable design framework.
Example of Elaborative Refactoring in Java
Consider a scenario where we want to introduce a new feature in a poorly designed class.
Original Code
public class User {
private String userName;
private String email;
public User(String userName, String email) {
this.userName = userName;
this.email = email;
}
public void sendEmail(String message) {
// Email sending logic here
}
public void logUserActivity(String activity) {
// Logging logic here
}
}
Observations
The User
class is handling responsibilities that go beyond its primary purpose. It mixes user data management with utilities like sending emails and logging activities.
Refactored Code
public class User {
private String userName;
private String email;
public User(String userName, String email) {
this.userName = userName;
this.email = email;
}
// More user-related methods could go here
}
public class EmailService {
public void sendEmail(User user, String message) {
// Email sending logic here
}
}
public class ActivityLogger {
public void logUserActivity(User user, String activity) {
// Logging logic here
}
}
Commentary
We separated responsibilities by creating two new classes: EmailService
and ActivityLogger
. This follows the Single Responsibility Principle, making the code cleaner, more maintainable, and easier to extend. By using elaborative refactoring, we set the class structure for future enhancements efficiently.
Choosing the Right Strategy
Selecting between fundamental and elaborative refactoring depends on your project's specific needs, the current state of the codebase, and long-term goals.
- Fundamental Refactoring is ideal for quick, focused fixes.
- Elaborative Refactoring aligns with a strategic vision for the application architecture.
Checklist for Decision Making
-
Assess the Current Code Quality
- Are there quick victories?
- Are there structural issues needing attention?
-
Understand the Project Goals
- Is this a maintenance task or a feature addition?
- Will this affect future scalability?
-
Team Capacity and Experience
- Is your team prepared to undertake extensive changes?
- Do they have a good grasp of design patterns and principles?
Wrapping Up
Refactoring is an essential procedure for maintaining a healthy codebase, and choosing the right strategy can have lasting effects on the quality and maintainability of your software. Whether you lean towards fundamental refactoring for immediate gains or opt for elaborative refactoring for broader architectural improvements, remember to keep the end goals in focus.
For more insights on refactoring strategies, consider checking the following resources:
Taking the time to assess your choices can significantly impact the future of your code project. Happy coding!
Checkout our other articles