Troubleshooting Common Issues with Jersey JAX-RS Streaming JSON
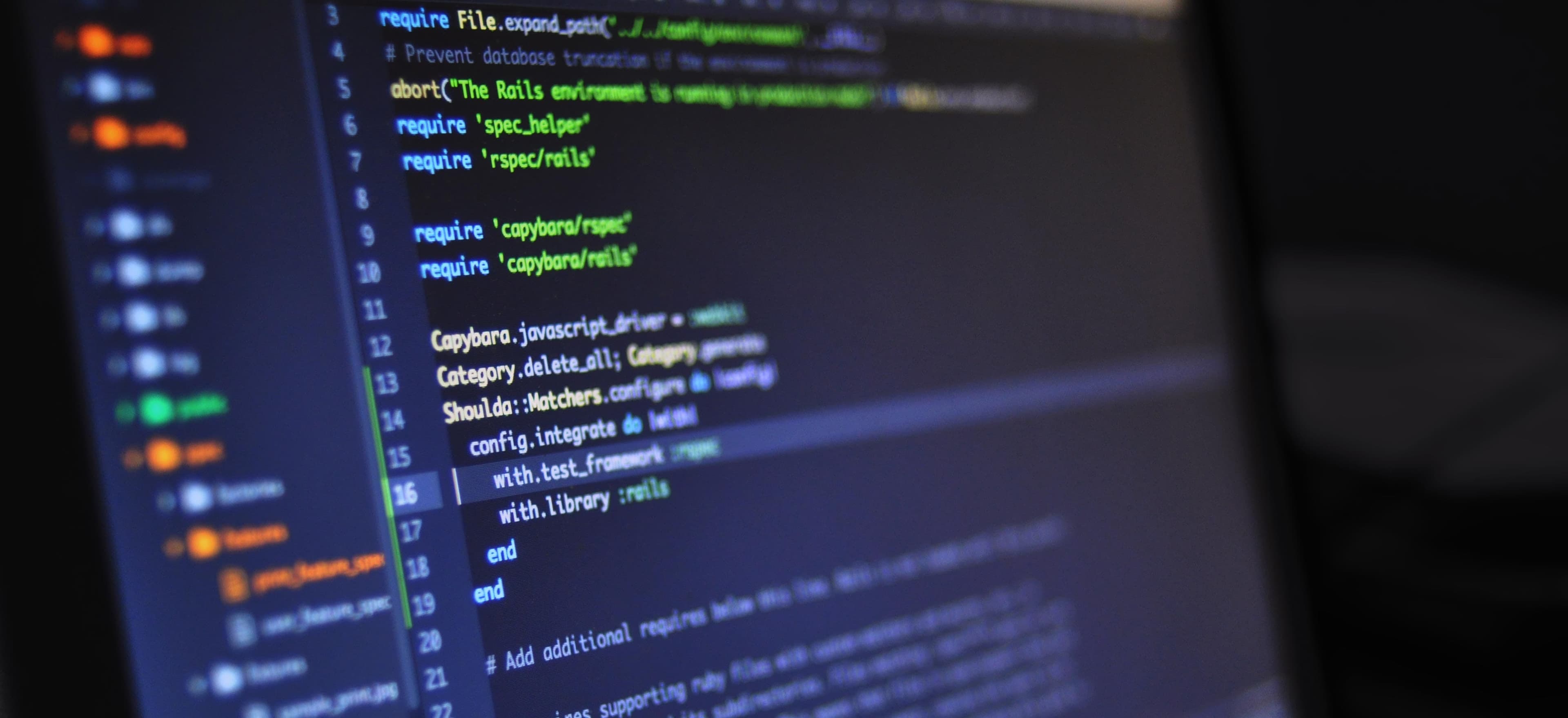
- Published on
Troubleshooting Common Issues with Jersey JAX-RS Streaming JSON
In the realm of Java web services, JAX-RS (Java API for RESTful Web Services) has become the go-to framework for building RESTful APIs. When using Jersey, the reference implementation of JAX-RS, you may often find yourself needing to stream JSON data efficiently. However, like any technology, you may encounter a range of common issues during the implementation or execution of streaming JSON responses.
This blog post outlines some of the most frequent pitfalls and their solutions when working with Jersey's streaming JSON capabilities.
Understanding Streaming JSON with Jersey
Before diving into troubleshooting, let’s quickly understand what streaming JSON means in the context of Jersey.
Streaming JSON allows your application to send data efficiently, in parts, rather than invoking an entire JSON object at once. This is particularly useful for large datasets, as it minimizes memory consumption and reduces latency.
Example of Streaming JSON Response
Here’s a basic example of how you can implement streaming JSON in a Jersey resource:
@Path("/stream")
public class StreamingResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public void streamJson(@Context HttpServletResponse response) throws IOException {
response.setContentType("application/json");
PrintWriter writer = response.getWriter();
writer.write("[");
for (int i = 1; i <= 100; i++) {
writer.write("{\"number\":" + i + "}");
if (i < 100) {
writer.write(",");
}
writer.flush(); // Immediately send the data to the client
}
writer.write("]");
writer.close();
}
}
In this snippet, we set the content type to application/json
and write each object in a loop, flushing it after each write to ensure that the client can start processing the data without waiting for the entire response.
Common Issues and their Solutions
1. Incorrect Content-Type
Problem:
If the client does not receive the correct Content-Type
, the data may not be processed as JSON.
Solution:
Ensure that you specify the correct Content-Type
when sending the response. The example above sets response.setContentType("application/json")
, which is crucial for instructing the client about how to interpret the data received.
2. JSON Not Formatted Properly
Problem: When streaming JSON, it is vital to maintain valid JSON format. Missing commas, brackets, or quotes can lead to malformed JSON.
Solution: Always validate your JSON structure before sending it. Use libraries such as JSON Schema Validator if you're uncertain about your JSON format.
3. Large Data Volumes Consume Excessive Memory
Problem: Streaming large datasets can lead to high memory usage if not managed correctly.
Solution: Instead of buffering large datasets in memory, stream them directly. This is illustrated in the sample above. Flushing the JSON stream after each iteration allows smaller chunks of data to be processed, keeping memory utilization low.
4. Concurrency Issues
Problem: Multiple simultaneous requests to your streaming endpoint can result in unexpected behavior because of shared resources.
Solution: Always ensure that your resource methods are stateless. Each request should handle its response independently, discouraging shared mutable state. Consider using synchronized blocks if necessary, but be cautious about performance impacts.
5. Timeouts on the Client-Side
Problem: Streaming responses that take too long due to large data processing may lead to client timeouts.
Solution: Keep the response streaming smooth and avoid long waits between writes. Implementing data batching—sending data in smaller groups—can help reduce timeouts. Here’s an adaptation of the previous example implemented with batching:
@Path("/batchStream")
public class BatchStreamingResource {
private static final int BATCH_SIZE = 10;
@GET
@Produces(MediaType.APPLICATION_JSON)
public void streamBatchedJson(@Context HttpServletResponse response) throws IOException {
response.setContentType("application/json");
PrintWriter writer = response.getWriter();
writer.write("[");
for (int i = 0; i < 100; i++) {
writer.write("{\"number\":" + i + "}");
if ((i + 1) % BATCH_SIZE == 0) {
writer.write(","); // Add a comma to separate batches
writer.flush(); // Flush after each batch
}
}
writer.write("]");
writer.close();
}
}
6. Error Handling Issues
Problem: Errors occurring during the streaming process can go unnoticed and unreported.
Solution: Implement proper error handling using Java's exception handling mechanisms. Consider returning error details in JSON format to provide the client with more context:
try {
// Streaming logic
} catch (IOException e) {
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
response.getWriter().write("{\"error\":\"" + e.getMessage() + "\"}");
}
7. Streaming Endpoints Not Supported by Some Clients
Problem: Certain clients may not be equipped to handle streamed JSON responses.
Solution: Implement fallbacks for clients that cannot handle streaming. You can provide the same data in a non-streaming format as a separate endpoint. Here's how you can do that:
@GET
@Path("/nonStreaming")
@Produces(MediaType.APPLICATION_JSON)
public List<Data> getNonStreamingJson() {
// Return the entire dataset as a List
return database.fetchAllData();
}
In Conclusion, Here is What Matters
Streaming JSON using Jersey provides significant benefits, particularly when dealing with large datasets. However, you must navigate various pitfalls to ensure reliability and performance. By following the solutions outlined in this post, you can troubleshoot common issues effectively and provide a robust streaming experience.
Additional Resources
With these guidelines, you can enhance your Jersey streaming implementation, making it an efficient and reliable choice for developing RESTful APIs. Happy coding!
Checkout our other articles