Common Maven Dependency Conflicts and How to Resolve Them
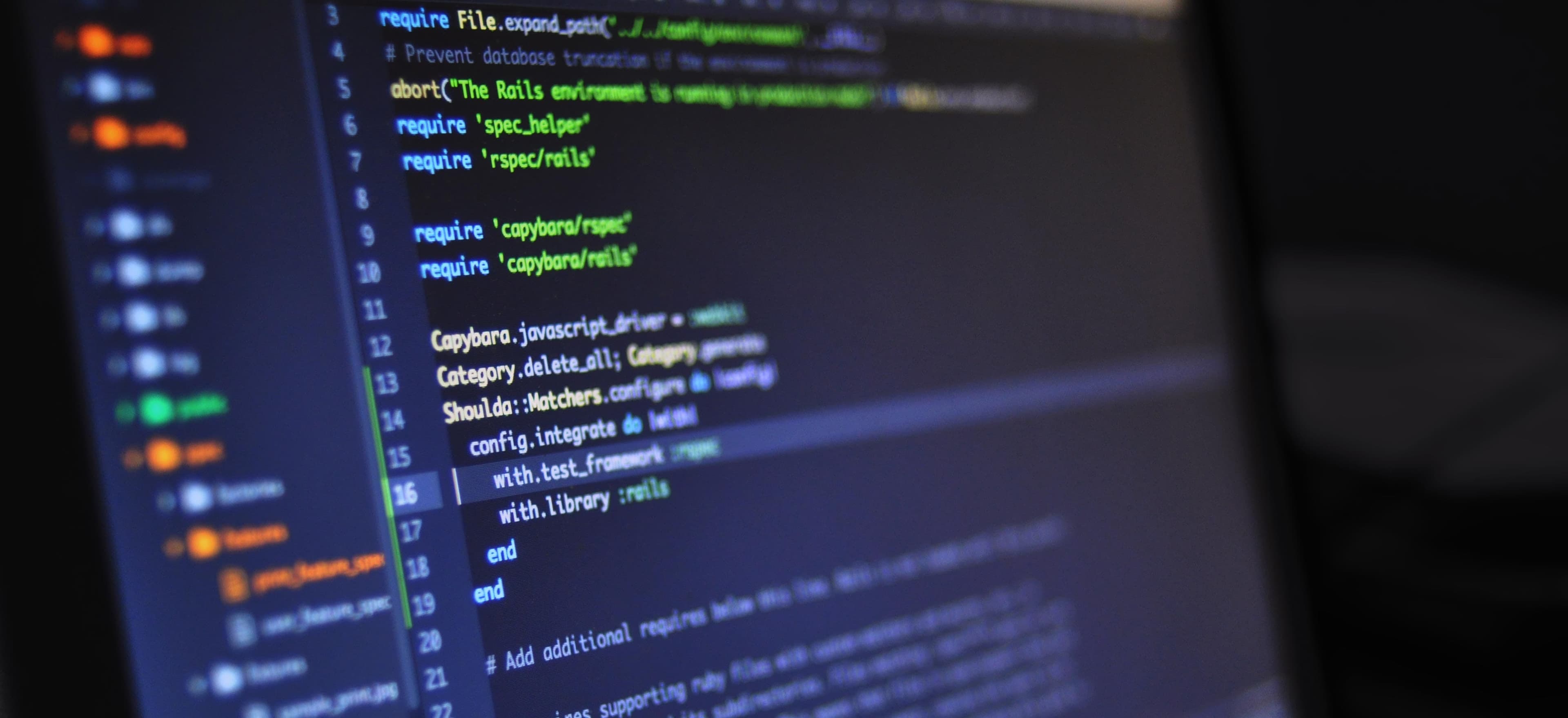
- Published on
Common Maven Dependency Conflicts and How to Resolve Them
Setting the Stage
Maven is a powerful build automation tool used primarily for Java projects. One of the main advantages of using Maven is its dependency management feature, allowing developers to easily include external libraries in their projects. However, Maven dependency conflicts can lead to unexpected behavior, runtime errors, and overall headaches. This article delves into common Maven dependency conflicts and provides clear, actionable solutions to resolve them.
What is a Maven Dependency Conflict?
A Maven dependency conflict occurs when multiple dependencies in your project require different versions of the same library. This can create confusion about which version of the library will be used at runtime. The result? A plethora of potential issues including NoSuchMethodError
, ClassNotFoundException
, or simply unexpected behavior from your application.
Common Causes of Dependency Conflicts
-
Transitive Dependencies: These are dependencies that are not declared directly in your
pom.xml
but are brought in by your direct dependencies. -
Version Range Conflicts: When a dependency specifies a range of versions, and different dependencies of your project specify conflicting ranges.
-
Dependency Exclusions: Sometimes dependencies must be explicitly excluded from transitive dependencies, leading to conflicts if not managed properly.
Identifying Dependency Conflicts
Before resolving conflicts, it's crucial to identify them. Maven provides a helpful command to visualize your project's dependency tree:
mvn dependency:tree
This will output all the dependencies and their respective versions. Pay attention to any discrepancies that might suggest a conflict.
Example Output
Here’s an example of what the output may look like:
[INFO] +- com.example:my-app:jar:1.0-SNAPSHOT:compile
[INFO] | +- org.springframework:spring-core:jar:5.2.0.RELEASE:compile
[INFO] | +- org.hibernate:hibernate-core:jar:5.4.0.Final:compile
[INFO] | | \- org.slf4j:slf4j-api:jar:1.7.25:compile
[INFO] | +--- org.slf4j:slf4j-api:jar:1.7.30:compile
In this example, you can see two conflicting versions of slf4j-api
: 1.7.30
and 1.7.25
.
Resolving Dependency Conflicts
Now that we've identified the conflicts, how do we resolve them? Here’s a step-by-step guide.
1. Specify Dependency Versions Explicitly
One of the most straightforward ways to resolve conflicts is to explicitly define the version of a transitive dependency in your pom.xml
.
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
2. Use Dependency Exclusions
Sometimes, you may want to exclude a specific version of a transitive dependency that is causing conflict. You can do this using the <exclusions>
tag in your pom.xml
.
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.0.Final</version>
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
</exclusion>
</exclusions>
</dependency>
In this example, we’re excluding the transitive slf4j-api
dependency from hibernate-core
, which allows other versions to be used without conflict.
3. Use Maven Dependency Plugin for Debugging
The Maven Dependency Plugin is invaluable for diagnosing issues.
You can use the dependency:analyze
goal, which will analyze your project's dependencies and provide feedback about unused and undeclared dependencies.
mvn dependency:analyze
This command helps you tighten up your dependency declarations, which can often resolve conflicting versions.
4. Set a Dependency Version in the Parent POM
If you’re working with multiple modules in a multi-module Maven project, consider defining dependency versions in the parent POM to ensure consistency across all modules.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
</dependencies>
</dependencyManagement>
This approach standardizes the version used throughout child modules, helping you avoid discrepancies.
Additional Resources
Further reading on managing dependencies in Maven can be found in the official Maven documentation. Understanding the underlying concepts helps streamline your development process.
The Last Word
Maven dependency conflicts can be a significant roadblock in Java development if not handled properly. By identifying, analyzing, and resolving these conflicts using explicit dependencies, exclusions, and dependency management techniques, you can maintain a stable and functional codebase.
Keep this guide handy for future projects and refer to the Maven Dependency Plugin documentation when needed. Happy coding, and may your dependency trees always stay healthy!
Checkout our other articles