Struggling with GlassFish 4 Embedded Setup in Gradle?
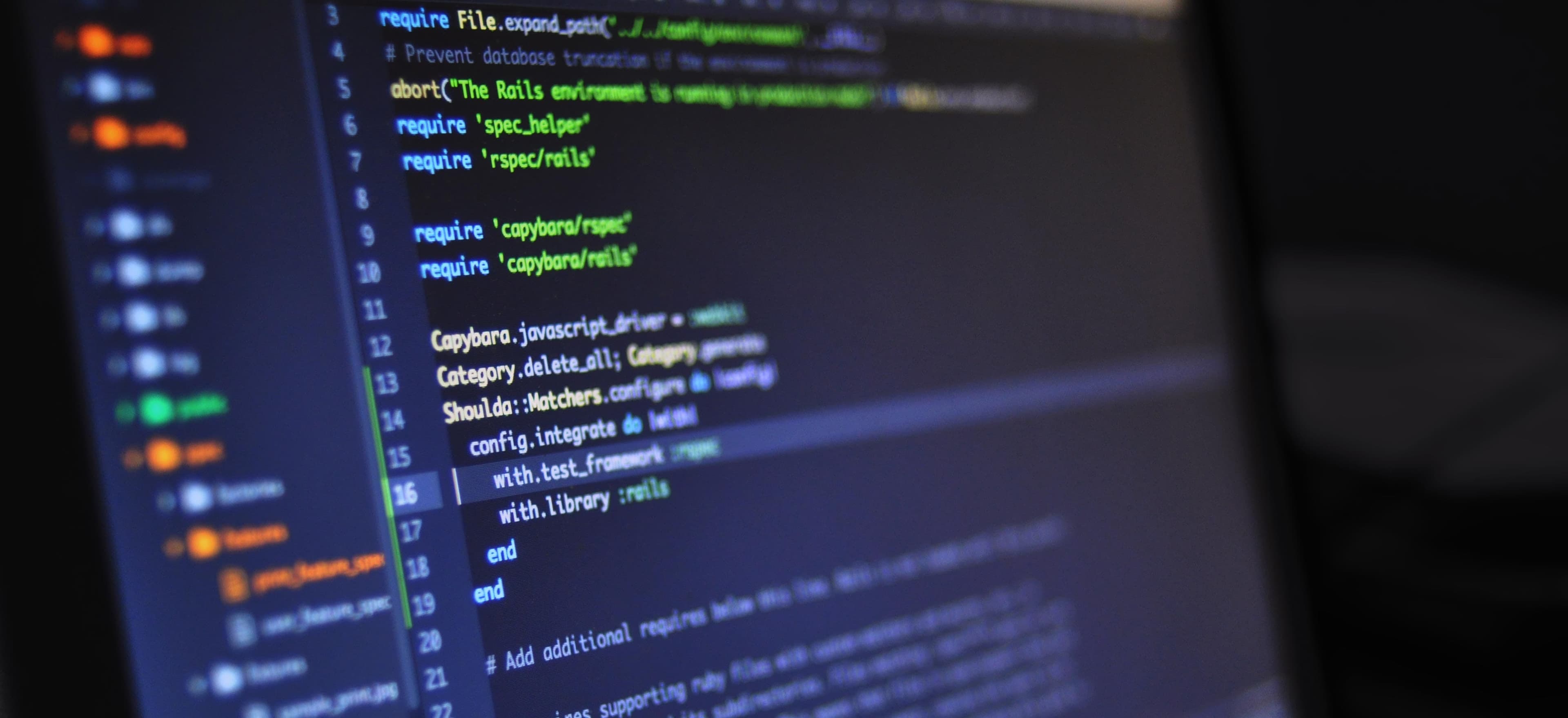
- Published on
Struggling with GlassFish 4 Embedded Setup in Gradle?
Setting up a Jakarta EE application server such as GlassFish in a Gradle project can sometimes feel overwhelming, especially when it comes to using the embedded version. The embedded GlassFish makes development and testing smoother, reducing the setup time required for your applications. In this blog post, I'll guide you through the process of setting up GlassFish 4 embedded in a Gradle project, addressing common pitfalls and ensuring that you have a smooth experience.
Table of Contents
- What is GlassFish Embedded?
- Setting Up Your Gradle Project
- Adding GlassFish Embedded Dependency
- Configuring Your Dockerfile
- Creating a Sample Application
- Running Your Application
- Conclusion
What is GlassFish Embedded?
GlassFish Embedded is a lightweight version of the GlassFish application server that can be integrated directly into your Java application. It allows developers to run a server instance within their applications during development and testing phases. This is particularly useful because it streamlines the process without the need for a dedicated server process to be running in the background.
Benefits of Using GlassFish Embedded
- Reduced setup and configuration time.
- Modular and flexible application development.
- Easy integration with testing frameworks.
Setting Up Your Gradle Project
Let’s begin by creating a new Gradle project for your Jakarta EE application. Open your terminal and execute the following commands:
mkdir glassfish-embedded-gradle
cd glassfish-embedded-gradle
gradle init
This will initialize a new Gradle project with a basic structure. In the build.gradle
file, ensure that you have the Java plugin applied:
plugins {
id 'java'
}
Adding GlassFish Embedded Dependency
To use GlassFish embedded, you must add the necessary dependencies. Edit your build.gradle
file to include the GlassFish Embedded dependency:
repositories {
mavenCentral()
}
dependencies {
implementation 'org.glassfish.main:glassfish-embedded-all:4.1.0'
implementation 'javax:javaee-api:7.0'
testImplementation 'junit:junit:4.13.2'
}
Here, we include the GlassFish embedded library with glassfish-embedded-all
version 4.1.0
. The Java EE API is also included to ensure that we have all the Jakarta EE features we need.
Why Use glassfish-embedded-all
?
The glassfish-embedded-all
package prevents the need to include multiple individual dependencies. It consolidates everything necessary to run a GlassFish server.
Configuring Your Dockerfile
Once you have your Gradle project configured, you might want to think about how this will run in different environments, including Docker. Here’s an example of how you could configure a Dockerfile if you wish to package your application with the embedded server:
FROM openjdk:11-jdk
COPY build/libs/glassfish-embedded-gradle.jar /app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Why Use a Dockerfile?
Using a Dockerfile allows you to deploy your application consistently across different environments, eliminating the "it works on my machine" problems.
Creating a Sample Application
Next, let’s create a simple RESTful application to test our embedded GlassFish server. You can add a new Java class within the src/main/java
directory:
package com.example;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Application;
import javax.ws.rs.core.Response;
@Path("/")
public class HelloWorld {
@GET
public Response hello() {
return Response.ok("Hello World!").build();
}
}
Explanation of the Code
In the above code, we've defined a simple JAX-RS resource. The @Path
annotation indicates that this class will respond to HTTP requests sent to the root URL ("/"). The hello
method handles GET requests and returns a simple "Hello World!" response.
Running Your Application
To launch the embedded GlassFish server, create another class with a main
method to act as the entry point of your application:
package com.example;
import org.glassfish.embedded.*;
import org.glassfish.jersey.server.ResourceConfig;
import java.io.IOException;
public class GlassFishEmbeddedApp {
public static void main(String[] args) {
GlassFish glassfish = GlassFishRuntime.bootstrap();
try {
final HttpServer server = glassfish.getHttpServer();
final ResourceConfig rc = new ResourceConfig(HelloWorld.class);
glassfish.getRest().getHttpServer().start();
System.out.println("Server started at: http://localhost:8080/");
System.in.read(); // Keep the server running
} catch (IOException e) {
e.printStackTrace();
} finally {
glassfish.shutdown();
}
}
}
Why Use GlassFishRuntime.bootstrap()
?
By invoking GlassFishRuntime.bootstrap()
, we initialize the embedded GlassFish server, allowing your application to handle HTTP requests efficiently.
Closing the Chapter
Setting up the GlassFish 4 embedded server in a Gradle project is much easier than it seems, thanks to its lightweight nature and effective integration capabilities. By following the steps outlined in this post, you should be well on your way to embedding GlassFish within your applications seamlessly.
If you're interested in further enhancing your Jakarta EE knowledge, consider checking out the Jakarta EE documentation. This offers a wealth of resources to deepen your understanding and take your development skills to the next level.
Feel free to reach out with questions or share your own experiences with GlassFish embedded in Gradle. Happy coding!
Checkout our other articles