Troubleshooting Common Issues with JSR 356 on Jetty 9.1
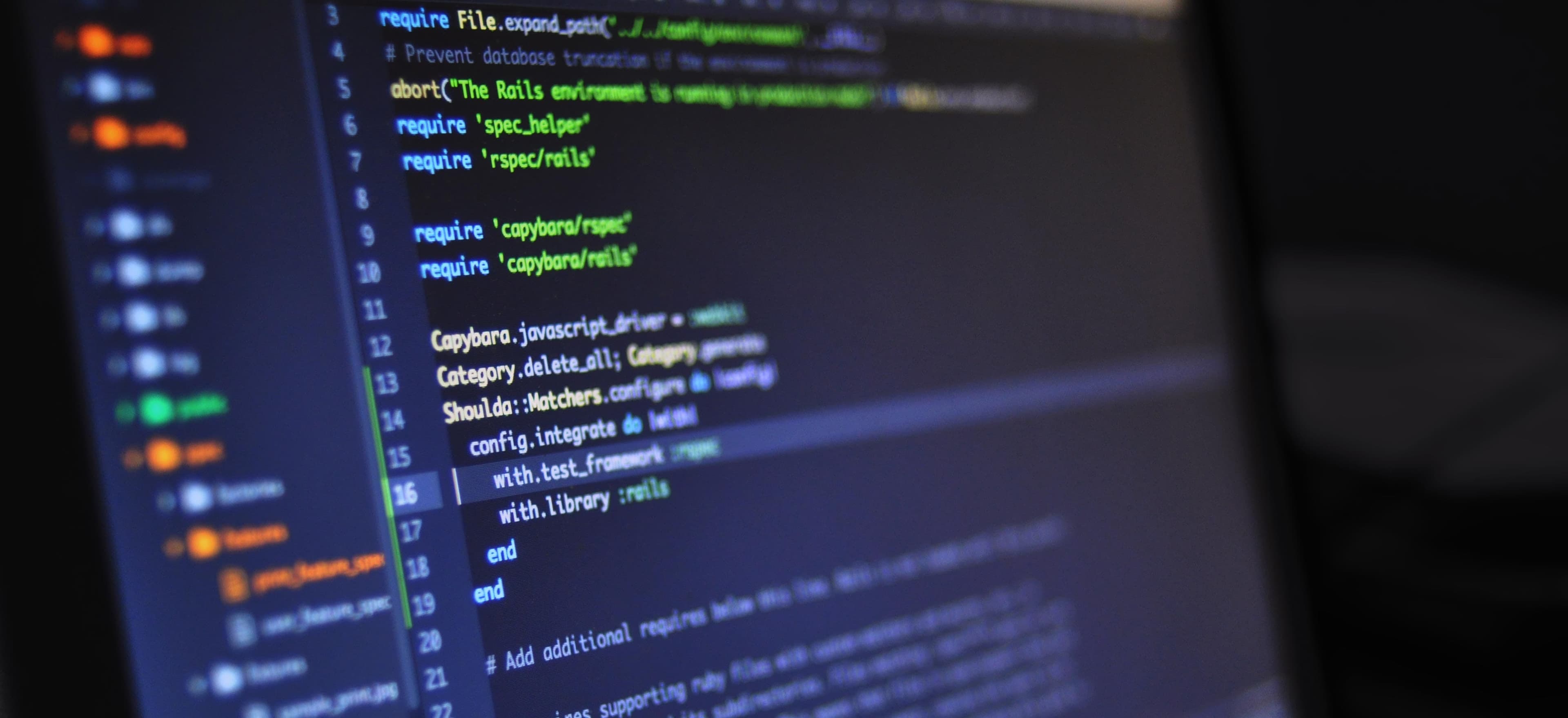
- Published on
Troubleshooting Common Issues with JSR 356 on Jetty 9.1
Java is a powerful language that is constantly evolving, and one of its frameworks, Jetty, provides a lightweight and flexible approach to server-side Java applications. JSR 356, the Java API for WebSocket, is a critical piece for real-time web applications. However, it comes with its quirks. In this blog post, we will explore common issues developers encounter when using JSR 356 on Jetty 9.1 and how to resolve them.
Understanding JSR 356 and Jetty 9.1
JSR 356, officially known as the Java API for WebSocket, enables developers to create real-time, event-driven applications. Jetty, an open-source HTTP server and servlet container, supports JSR 356, allowing for easy deployment and management of WebSocket connections. However, incorporating WebSockets effectively can lead to challenges. Let’s dive into the most common issues and their solutions.
1. Class Not Found Exception
One of the frequent pitfalls is encountering a ClassNotFoundException
when trying to set up the WebSocket. This could stem from one of the following issues:
- Missing dependencies
- Incorrect classpath settings
Solution
Ensure you have the correct dependencies set up in your project. If you're using Maven, include the following in your pom.xml
:
<dependency>
<groupId>org.eclipse.jetty.websocket</groupId>
<artifactId>websocket-server</artifactId>
<version>9.1.0.v20121114</version>
</dependency>
By specifying the correct version, you ensure compatibility with Jetty 9.1. The inclusion of this library is crucial since it provides the essential classes and interfaces required for WebSocket functionalities.
2. Deployment Descriptor Issues
Improper configuration in the web.xml
deployment descriptor can also lead to WebSocket problems. Any syntactical errors or incorrect servlet mappings can prevent the WebSocket from functioning correctly.
Solution
Double-check the web.xml
configurations. Here’s how a standard configuration might look:
<servlet>
<servlet-name>WebSocketServlet</servlet-name>
<servlet-class>org.eclipse.jetty.websocket.server.WebSocketServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>WebSocketServlet</servlet-name>
<url-pattern>/ws/*</url-pattern>
</servlet-mapping>
Make sure that the URL pattern matches where you want to handle WebSocket connections. Consistency is key—any mismatch can lead to connection issues.
3. Connection Refused Errors
Another common issue is the "Connection Refused" error, which usually indicates that the WebSocket server is unreachable. This could be due to several reasons such as server not running or incorrect URL used for connection.
Solution
First, confirm that the Jetty server is up and running. You can do this by checking the console logs or trying to access a static resource served by the same application.
Next, ensure you are using the correct WebSocket URL, typically something like ws://localhost:8080/ws
. Here is a simple JavaScript snippet to demonstrate how to establish a connection:
const socket = new WebSocket("ws://localhost:8080/ws");
socket.onopen = function(event) {
console.log("Connected to WebSocket Server!");
};
socket.onerror = function(error) {
console.log("WebSocket error observed:", error);
};
Using this snippet, you can easily debug if the connection is established. Always check your server logs for additional insights.
4. Event Handling Failures
When the connection is established, you may encounter issues with event handling—especially when sending and receiving messages. Errors here may stem from the misuse of WebSocket API.
Solution
Ensure the appropriate WebSocket event listeners are implemented correctly. Here's an example of a basic WebSocket endpoint:
import javax.websocket.OnMessage;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/ws")
public class MyWebSocket {
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Received message: " + message);
try {
session.getBasicRemote().sendText("Echo: " + message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code snippet, the onMessage
method processes incoming messages and sends back an echo. It’s essential to handle exceptions gracefully to prevent crashes during runtime. By properly managing WebSocket events, you ensure that data is exchanged without hitches.
5. Session Management Issues
Managing WebSocket sessions can become tricky, especially in applications with many concurrent users. Failure to manage sessions could lead to wasted resources or memory leaks.
Solution
You can manage WebSocket sessions effectively by storing connections and handling them properly. Here’s an approach using a concurrent map to store sessions:
import javax.websocket.Session;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
@ServerEndpoint("/ws")
public class MyWebSocket {
private static Map<String, Session> userSessions = new ConcurrentHashMap<>();
@OnOpen
public void onOpen(Session session) {
userSessions.put(session.getId(), session);
System.out.println("Session opened: " + session.getId());
}
@OnClose
public void onClose(Session session) {
userSessions.remove(session.getId());
System.out.println("Session closed: " + session.getId());
}
}
With this setup, every time a user connects, their session is stored, and when they disconnect, their session is removed. This approach helps maintain a clean and efficient server environment.
A Final Look
Troubleshooting JSR 356 on Jetty 9.1 involves understanding the underlying architecture and being able to identify, isolate, and resolve issues. From ensuring dependencies are correctly configured to handling sessions elegantly, each aspect contributes to a robust WebSocket implementation.
If you adhere to proper coding practices and leverage the provided snippets, you can efficiently manage WebSocket communications. For further reading, check out the official Jetty documentation and JSR 356 official specification for additional details on best practices.
Are you encountering a specific JSR 356 issue that wasn’t covered here? Share your experience in the comments, and let’s solve it together!