Navigating Data Chaos: The Need for Effective Conversion Tools
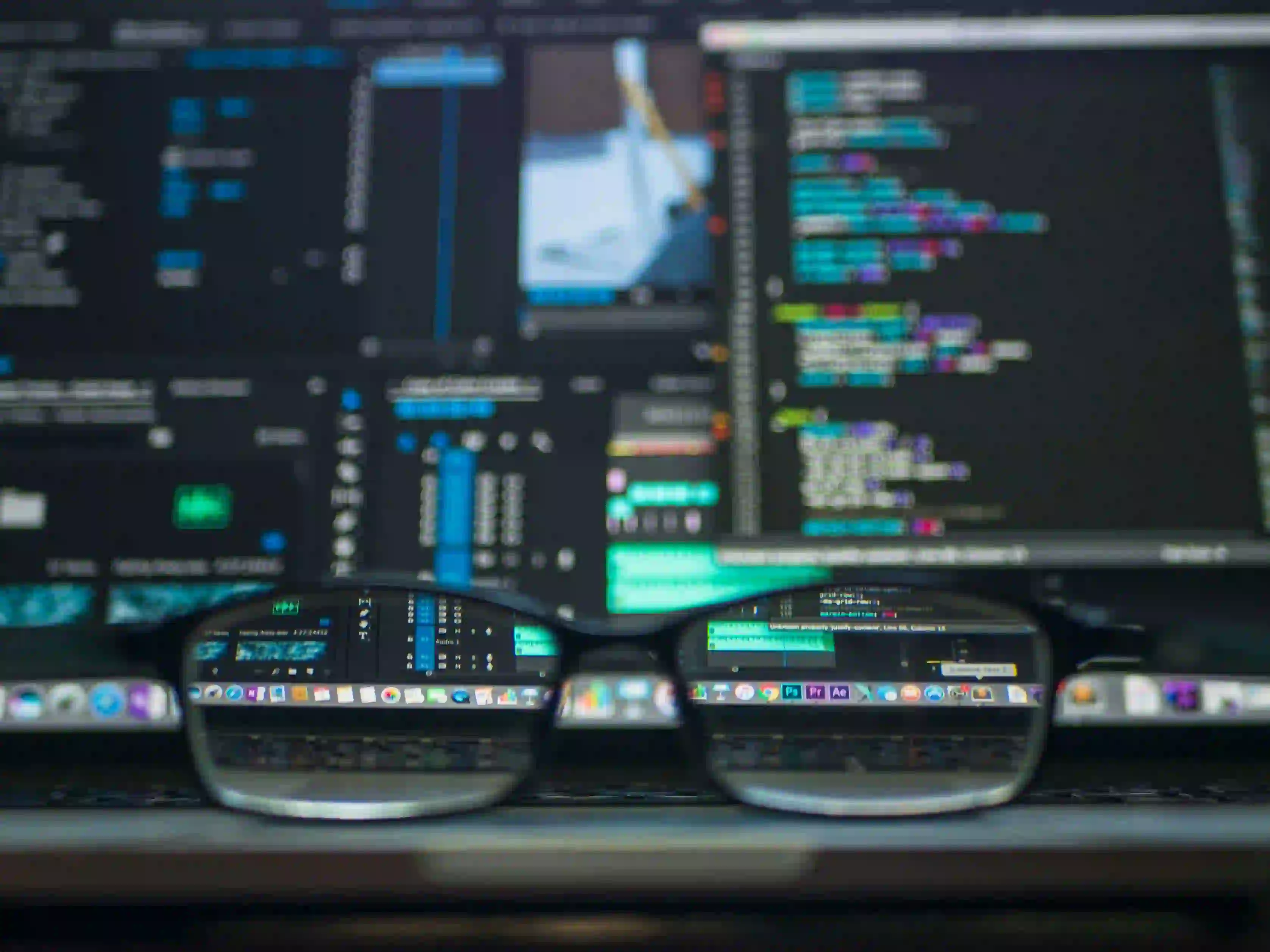
Navigating Data Chaos: The Need for Effective Conversion Tools
The digital age offers unparalleled volumes of data, but with this abundance comes an inherent chaos. Organizations grappling with diverse data formats often find themselves in dire need of effective data conversion tools. This blog post aims to highlight the significance of these tools, how they can simplify processes, and gives you an overview of various strategies to navigate data chaos.
Understanding Data Chaos
Data chaos refers to the disorganized state of information encountered by businesses. This confusion arises from incoming data streams, most often in various formats such as CSV, JSON, XML, and even proprietary spreadsheets. The problems created by data chaos are manifold; they can cause inefficiencies in analytics, increased operational costs, and hindered decision-making processes.
Effective data conversion tools help organizations transform data into formats that are easier to manage and analyze. They enable seamless integration across systems, improving interoperability, and streamlining workflows.
Why Data Conversion Tools are Essential
- Interoperability: Data formats are not always compatible; conversion tools bridge this gap.
- Efficiency: Automating the conversion process saves time and resources.
- Data Quality Improvement: Tools often come with built-in validation that enhances data quality.
- Better Insights: With organized data, businesses can leverage analytics to gain actionable insights.
Popular Data Formats and Conversion Techniques
1. CSV (Comma-Separated Values)
CSV is a widely-used format for storing tabular data. While easy to read, it often surfaces as a problematic format for programs that need to handle complex data types.
Example: Using Java to convert CSV to JSON.
import com.fasterxml.jackson.databind.ObjectMapper;
import com.opencsv.CSVReader;
import com.opencsv.CSVWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class CSVToJsonConverter {
public static void main(String[] args) {
String csvFile = "data.csv";
String jsonFile = "data.json";
try (CSVReader reader = new CSVReader(new FileReader(csvFile));
FileWriter writer = new FileWriter(jsonFile)) {
String[] nextLine;
List<String[]> data = new ArrayList<>();
// Read CSV data
while ((nextLine = reader.readNext()) != null) {
data.add(nextLine);
}
// Convert to JSON
ObjectMapper mapper = new ObjectMapper();
String jsonOutput = mapper.writeValueAsString(data);
writer.write(jsonOutput);
System.out.println("CSV data successfully converted to JSON.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Code?
- We leverage the Jackson library for JSON conversion due to its simplicity and robustness.
- OpenCSV handles the CSV reading, simplifying the approach to file I/O.
2. JSON (JavaScript Object Notation)
JSON has gained immense popularity because of its lightweight and easy-to-read structure. However, converting JSON data back to other formats may be needed based on specific use cases, especially when interfacing with systems that require structured formats.
Example: Converting JSON to XML.
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import java.io.File;
import java.io.IOException;
public class JsonToXmlConverter {
public static void main(String[] args) {
String jsonFile = "data.json";
String xmlFile = "data.xml";
ObjectMapper jsonMapper = new ObjectMapper();
XmlMapper xmlMapper = new XmlMapper();
try {
// Read JSON
Object json = jsonMapper.readValue(new File(jsonFile), Object.class);
// Write to XML
xmlMapper.writeValue(new File(xmlFile), json);
System.out.println("JSON data successfully converted to XML.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Code?
- The code utilizes Jackson libraries for both JSON and XML, ensuring a consistent programming model.
- Using the ObjectMapper to handle JSON allows for versatility when reading Java objects.
3. XML (eXtensible Markup Language)
XML has historically been a standard for structured data. However, its verbosity can create unnecessary overhead. As applications evolve, converting XML data into more compact formats like JSON or CSV becomes imperative.
4. Proprietary Formats
Some formats are strictly tied to proprietary software and may require specialized tools for conversion. This often increases organizational dependency on specific software vendors.
Choosing the Right Tool
Selecting the appropriate data conversion tool involves evaluating several factors:
- Scalability: Will the tool handle evolving data volume and complexity?
- Ease of Use: Is it user-friendly, or does it require extensive learning?
- Cost: What are the licensing costs? Are there hidden fees for additional features?
Online Tools
Many online data conversion tools can simplify tasks without writing code. However, reliance on the internet for sensitive data is risky. Here are some reputable online tools that you may find useful:
Implementation in Business Processes
Integrating conversion tools within your business processes requires careful planning.
- Assess Data Needs: Identify which formats you frequently encounter and need conversion.
- Automate Where Possible: Use scripts or integration with ETL (Extract, Transform, Load) tools.
- Training and Best Practices: Ensure the staff understands the tools and best practices surrounding data handling.
My Closing Thoughts on the Matter
Understanding the tools available for data conversion is critical in a world overwhelmed by data chaos. The right tools help make data manageable, boosting efficiency and decision-making capabilities.
Effective data conversion is not merely a technical requirement—it is a business necessity. Organizations equipped with the right conversion tools will navigate their data chaos effectively, transforming potential disruptions into opportunities for growth.
For further reading, you can explore Effective Data Management Techniques or check out Best Practices in Data Governance.
Adapt your strategies, embrace the right tools, and watch your data workflow flourish.