Overcoming Jank in Android Activity Animations
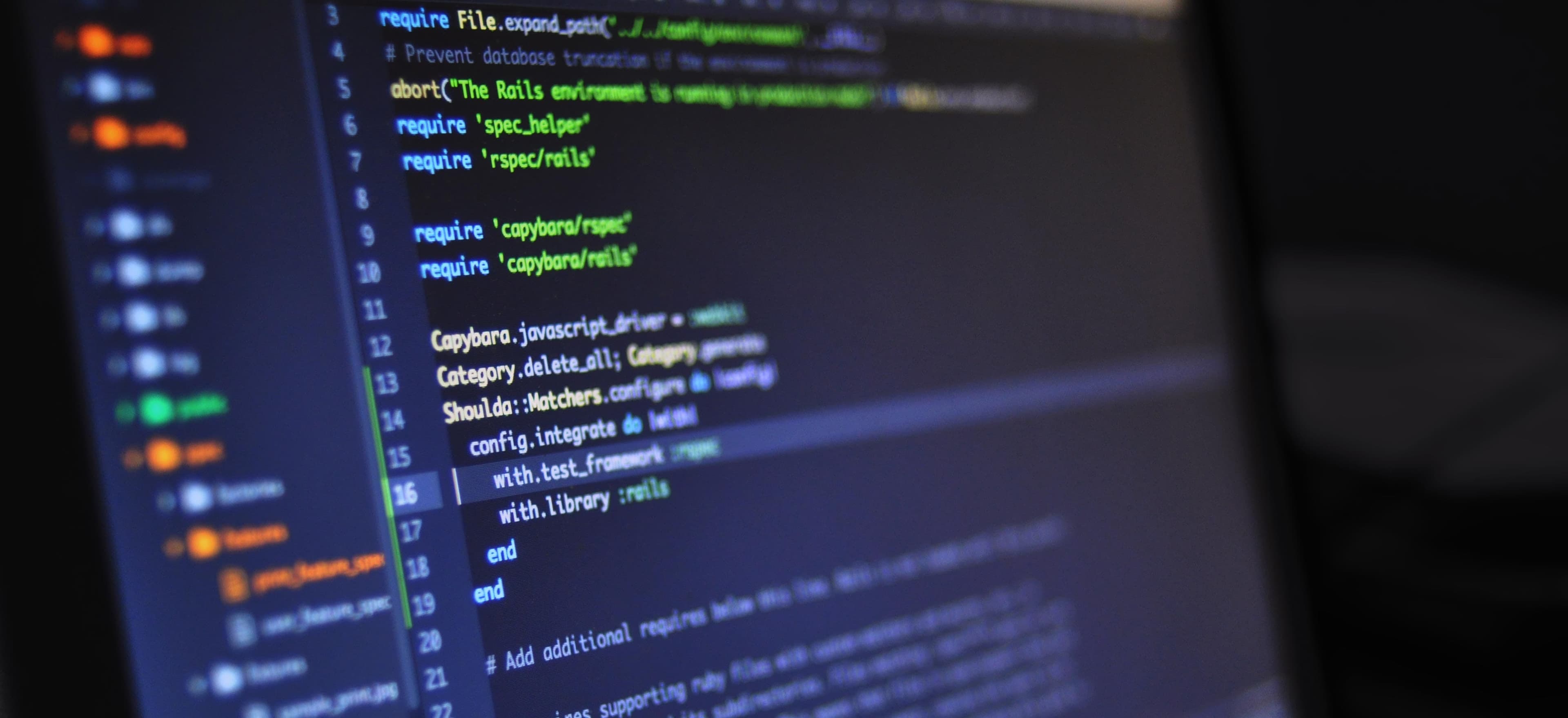
- Published on
Overcoming Jank in Android Activity Animations
Animations play a vital role in enhancing user experience on Android apps. They can make transitions smooth and give users feedback on their interactions. However, experiencing jank—those pesky stutters and slowdowns—during animations can significantly degrade the feel of an application. In this post, we will explore the common causes of jank during Android Activity animations and provide practical solutions to ensure smoother, more responsive transitions.
Understanding Jank in Animations
Jank occurs when frames are dropped during rendering, leading to choppy animations and an overall sluggish feel. It is critical to strive for a steady frame rate, usually at 60 frames per second (fps), to keep animations looking fluid. Anything below this threshold results in noticeable lag.
Common Causes of Jank
- Heavy UI operations: Performing intensive computations on the main thread can block the UI thread, causing frames to drop.
- Overdraw: Drawing too many pixels can slow down rendering. Overdraw occurs when multiple views are drawn in the same position.
- Inefficient Layouts: Using deep view hierarchies can add unnecessary complexity, leading to longer layout times.
- Inadequate Hardware Acceleration: Failing to take advantage of hardware acceleration can hinder performance, as animations won't leverage the GPU.
Strategies to Overcome Jank
Below are several effective strategies to mitigate jank during Activity animations.
1. Use the Choreographer
The Choreographer is an Android component designed to help manage animation callbacks in a more efficient manner. By using it, you can synchronize your animations with the display refresh rate, reducing jank significantly.
Here’s how to implement it:
Choreographer.getInstance().postFrameCallback(new Choreographer.FrameCallback() {
@Override
public void doFrame(long frameTimeNanos) {
// Code to execute on each frame
performAnimation();
Choreographer.getInstance().postFrameCallback(this);
}
});
Why use Choreographer?
Choreographer allows you to execute drawing operations at the precise moment before a new frame is drawn, ensuring better synchronization with the frame display rate.
2. Overhaul Layouts
To avoid deep view hierarchies that can cause lag, ensure your layouts are as flat as possible. Use the following techniques:
- Merge Tags: Use
<merge>
to flatten layouts. - ConstraintLayout: Embrace the use of
ConstraintLayout
for building complex layouts with fewer nested views.
Example of using ConstraintLayout
:
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Why this matters?
By simplifying the layout structure and reducing the number of ViewGroups, you reduce the time Android needs to measure and layout views, leading to more efficient rendering.
3. Optimize Draw Calls
Reducing the number of draw calls can significantly impact performance. Here are techniques to achieve that:
- Use Vector Drawables: These are resolution-independent and provide higher quality at lower memory costs.
- Limit the Use of Transparency: Transparent views require additional rendering and can lead to overdraw.
Vector Drawable Example:
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="24dp"
android:height="24dp"
android:viewportWidth="24"
android:viewportHeight="24">
<path
android:fillColor="#FF000000"
android:pathData="M12,2C6.48,2 2,6.48 2,12s4.48,10 10,10 10,-4.48 10,-10S17.52,2 12,2zM12,17.27L10.24,15.91 *..." />
</vector>
Why optimize draw calls?
By minimizing the graphics the system needs to render on-screen, you decrease the load on the GPU, which can translate into smoother animations.
4. Use view.setLayerType()
For certain animations, setting the layer type to use hardware acceleration can result in smoother transitions.
view.setLayerType(View.LAYER_TYPE_HARDWARE, null);
Why this works?
By utilizing hardware layers, you offload rendering from the main thread to the GPU, allowing for more fluid animations (especially in cases involving scrolling or complex movements).
5. Profile Your Application
Use tools like Android Profiler available in Android Studio. It helps in identifying bottlenecks that lead to jank. Pay attention to:
- CPU Usage: Monitor your CPU cycles to detect slow operations.
- Memory Utilization: Excessive memory consumption can hinder performance.
- GPU Rendering: Keep an eye on frame rendering times.
For detailed steps on profiling, check Android Developers’ profiling guide.
6. Avoid Long-Running Operations
Long-running tasks such as network calls or heavy computations should be offloaded from the UI thread. Use background threads or services to handle these tasks efficiently.
Example using AsyncTask (deprecated, consider using Executors or coroutines instead):
new AsyncTask<Void, Void, Void>() {
@Override
protected Void doInBackground(Void... voids) {
// Perform long-running operation here
return null;
}
@Override
protected void onPostExecute(Void result) {
// Update UI on main thread
}
}.execute();
Why is this crucial?
By keeping the UI thread free from heavy processing, you guarantee that the animations can run smoothly without being interrupted by other processes.
The Last Word
In conclusion, tackling jank in Android Activity animations involves a multifaceted approach. From utilizing techniques like the Choreographer to optimizing layouts and reducing heavy operations on the main thread, developers can create seamless user experiences.
By implementing the strategies outlined in this post, you can significantly improve the performance of your Android apps. For further reading, you might find Android's official animations documentation and material design guidelines useful.
By adhering to these principles, you’ll not only enhance performance but also create a more enjoyable experience for your users. Smooth transitions aren't just a visual luxury—they are crucial to keeping users engaged and happy with your application.
Checkout our other articles