Mastering Dynamic Bean Registration: Common Pitfalls
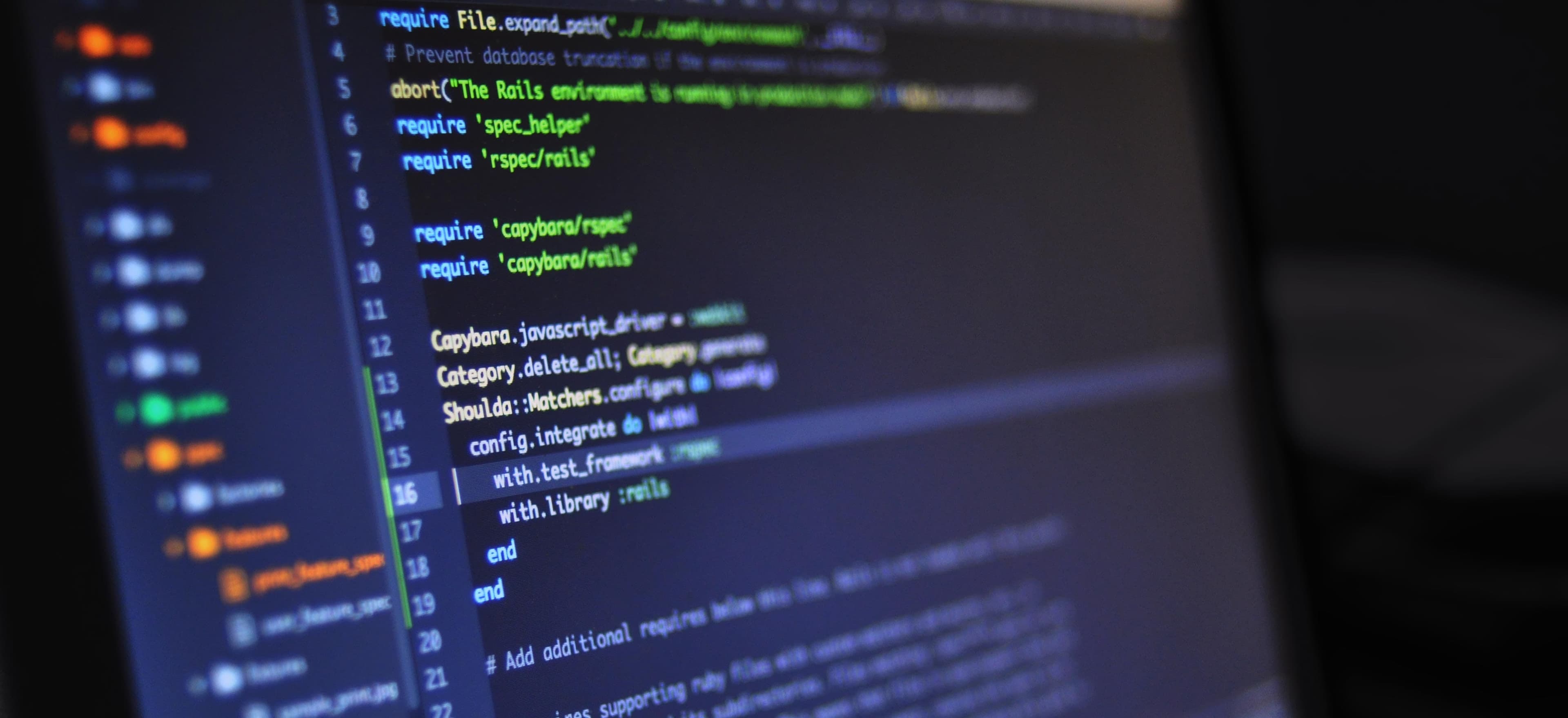
- Published on
Mastering Dynamic Bean Registration: Common Pitfalls
Dynamic bean registration is a powerful feature in the Spring framework, allowing developers to add beans programmatically at runtime. While this capability enhances the flexibility and dynamism of your applications, it also introduces a set of common pitfalls that developers often encounter. This blog post will explore these challenges and offer best practices to help you navigate them more effectively.
What is Dynamic Bean Registration?
Dynamic bean registration allows developers to define and register beans in the Spring application context after the application has started. This approach can be particularly useful for scenarios where the beans to be registered are not known at compile time. Common use cases include:
- Plugin architectures
- Factories
- Custom configurations based on specific conditions
To get started, let's take a look at a basic example of dynamic bean registration.
Example: Simple Dynamic Bean Registration
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Configurable;
import org.springframework.beans.factory.config.ConfigurableBeanFactory;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class DynamicBeanConfig {
@Autowired
private ConfigurableBeanFactory beanFactory;
public void registerDynamicBean(String beanName) {
// Create a new bean definition for a simple String bean
BeanDefinitionBuilder beanDefinitionBuilder = BeanDefinitionBuilder.genericBeanDefinition(String.class);
beanDefinitionBuilder.addConstructorArgValue("Dynamic Bean Value");
// Register the bean definition with the context
beanFactory.registerBeanDefinition(beanName, beanDefinitionBuilder.getBeanDefinition());
}
}
In this example:
- We use
BeanDefinitionBuilder
to define a simple string bean. - The bean is registered with the application context using
beanFactory.registerBeanDefinition
.
This is a straightforward example. However, dynamic bean registration can expose you to various pitfalls.
Common Pitfalls of Dynamic Bean Registration
1. Scope Management Issues
Problem: Managing the lifecycle and scope of dynamically registered beans can become complicated. The default scope for beans is singleton, but this may not always be your intention.
Solution: Clearly define the scope of your bean, especially if you need prototype or request scope.
beanDefinitionBuilder.setScope("prototype"); // For prototype scope
Understanding the scope is crucial. For instance, if you register a prototype bean but treat it like a singleton, you will encounter unexpected behavior.
2. Misconfigured Dependencies
Problem: Dynamically registered beans may have dependencies that are not satisfied because the registration occurs post-context initialization.
Solution: Ensure that your dependencies are registered before you register the dynamic bean or use a @Bean
method that allows for discovery.
3. Assuming Immediate Availability
Problem: Developers sometimes assume that a dynamically added bean will be immediately available in the application context.
Solution: Always ensure that you register the beans at the right time. Using ApplicationContextAware
to access the context might help you manage timings better.
4. Bean Overriding Confusion
Problem: If a bean with the same name already exists in the application context, registering a bean with the same name can lead to confusion and errors.
Solution: Use unique names for dynamically registered beans or set allow-bean-definition-overriding
to true in application.properties
. However, use caution with this setting to avoid unforeseen issues.
5. Testing Challenges
Problem: Testing dynamic beans can be tedious, especially if your tests expect fixed beans.
Solution: Implement tests that mock or stub dynamic bean registration. Consider using @MockBean
for testing your beans within the Spring test context.
How to Avoid Common Pitfalls
Here are some strategies to avoid pitfalls when working with dynamic bean registration.
1. Use FactoryBeans
FactoryBeans can help manage the complexity of dynamic bean registration. They allow you to create objects that can be managed by Spring.
import org.springframework.beans.factory.FactoryBean;
public class MyDynamicBeanFactory implements FactoryBean<MyDynamicBean> {
@Override
public MyDynamicBean getObject() throws Exception {
return new MyDynamicBean("Example Value"); // Dynamic value created on demand
}
@Override
public Class<?> getObjectType() {
return MyDynamicBean.class;
}
@Override
public boolean isSingleton() {
return false; // Indicate if this factory produces singleton beans
}
}
2. Establishing Naming Conventions
Adopting clear naming conventions for your dynamic beans ensures transparency. For example, prefix dynamically registered beans with "dynamic_". This reduces the chance of conflicts or confusion.
3. Documentation
Properly documenting dynamic bean registration practices aids both current and future developers. Clear documentation helps other team members understand the purpose and use cases of dynamic beans in your applications.
4. Leverage Profiles
Using Spring Profiles can help avoid pitfalls associated with conditional bean registration. This allows you to segregate application components based on deployment requirements and avoids unnecessary complexity.
@Profile("dev")
@Bean
public MyBean myDevBean() {
return new MyBean("Development Bean");
}
5. Configuration Classes
Keep dynamic registration logic organized by encapsulating it within specific configuration classes. This makes the registration logic cleaner and facilitates easier maintenance.
Wrapping Up
Dynamic bean registration is a robust tool that provides enhanced flexibility in the Spring framework. However, navigating its common pitfalls requires diligence and best practices. By primarily focusing on scope management, dependency configurations, and bean naming conventions, you can mitigate potential issues effectively.
For more detailed information on Spring Bean Lifecycle and managing beans in a Spring context, consider looking at the Spring Documentation.
As you proceed with dynamic bean registration, ensure to apply these lessons to not only improve your code's performance but maintain its maintainability.
Checkout our other articles