Handling Backpressure in RxJava: Common Pitfalls Explained
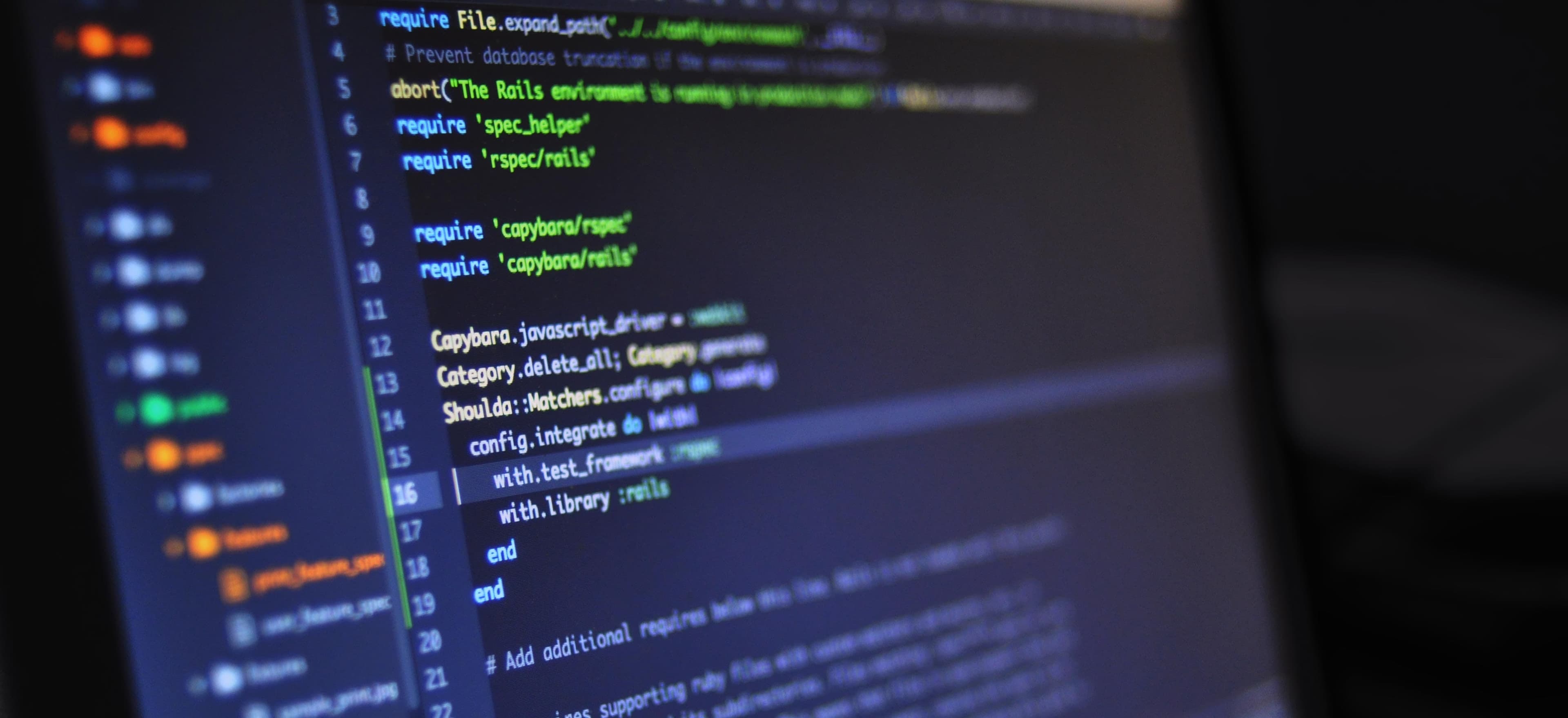
- Published on
Handling Backpressure in RxJava: Common Pitfalls Explained
Dealing with asynchronous data streams is a fundamental challenge for developers using Java. With the introduction of RxJava, reactive programming became a more manageable task, allowing developers to handle streams of data in a not-so-traditional manner. However, as we dive deeper into the reactive world, one critical concept needs emphasis: backpressure.
Backpressure is the mechanism that allows a Subscriber to signal to a Publisher that it cannot handle data at the rate it is receiving it. Handling backpressure correctly is essential to preventing application failures and ensuring a smooth flow of data. In this post, we'll explore common pitfalls experienced while handling backpressure in RxJava, dive into effective implementations, and illustrate practical solutions.
What Is Backpressure?
Before examining the pitfalls of backpressure, let's define the term. Backpressure occurs when a downstream consumer cannot keep up with the rate of data produced by the upstream producer. This mismatch can create a backlog, leading to overwhelming resource consumption and potential application crashes.
For example, if you are consuming large datasets from a database or an external API and processing them in a limited resource environment, failing to handle backpressure might result in memory overflow.
Understanding the Default Behavior
RxJava offers various operators to manage backpressure, notably through the Flowable
type which inherits from Observable
. By default, Observable
does not adequately support backpressure; hence, developers are encouraged to use Flowable
for scenarios where backpressure management is crucial.
Example Code Snippet: Basic Flowable
import io.reactivex.Flowable;
public class BackpressureExample {
public static void main(String[] args) {
Flowable<Integer> flowable = Flowable.range(1, 10)
.onBackpressureBuffer(5); // Buffering a maximum of 5 items
flowable
.observeOn(Schedulers.io())
.subscribe(item -> {
// Simulate a slow consumer
Thread.sleep(1000);
System.out.println("Received: " + item);
});
// Keep the main thread alive for demonstration
try {
Thread.sleep(15000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
In this example, we use the onBackpressureBuffer
operator to allow the Flowable
to hold onto a maximum of 5 items while the consumer takes its time. This can mitigate the risk of overwhelming the subscriber.
Common Pitfall 1: Ignoring Backpressure
Ignoring backpressure is akin to ignoring the check engine light on your car's dashboard. While it may not affect you immediately, in the long run, you are bound to encounter serious issues. Many developers might instinctively use Observable
for their operators—this is an unreliable approach.
Solution
Always evaluate your data sources and choose Flowable
where backpressure might become an issue. Surrounding yourself with the right tools is essential. Read more about RxJava and familiarize yourself with its ecosystem.
Pitfall 2: Misusing Buffering Strategies
Buffering strategies can be deceptive. On the one hand, buffering can temporarily alleviate backpressure problems; on the other hand, it can lead to excessive memory consumption if not used wisely.
Example Code Snippet: Buffers without Limits
Flowable<Integer> flowable = Flowable.range(1, 100)
.onBackpressureBuffer(); // No limit will lead to possible OOM
flowable
.observeOn(Schedulers.io())
.subscribe(item -> {
Thread.sleep(100); // Simulating slow processing
System.out.println("Processed: " + item);
});
In this case, the Flowable
is configured to hold an unlimited number of buffered items. While this might work for small data sets, in a real-world scenario, it can quickly lead to out-of-memory (OOM) exceptions.
Solution
Whenever you use buffering, ensure that you define a maximum buffer size to avoid infinite growth. A common practice is to apply a limit to your buffers, as shown below:
.onBackpressureBuffer(10); // Limit to 10 items
Pitfall 3: Misunderstanding the Flowable's Request Mechanics
Another frequent error is misleading the number of items requested from the upstream publisher. When you subscribe to a Flowable
, it indicates how many items the subscriber can handle at any moment via the request(n)
method.
Example Code Snippet: Requesting Insufficient Items
flowable
.observeOn(Schedulers.io())
.subscribe(item -> {
// Requesting only 1 item at a time
request(1);
System.out.println("Received: " + item);
});
This approach can severely restrict the flow of data, leading to unnecessary delays.
Solution
Tune the request size based on your processing capabilities. A common practice is to request several items at once:
request(5); // Process 5 items at a time
Understand Your Application Load
The nature of your application dictates how much data can be handled effectively. Monitoring and fine-tuning the request size can optimize performance and prevent backpressure-induced bottlenecks.
Pitfall 4: Inadequate Error Handling
Reactive streams are designed to propagate errors. However, inadequate error handling when dealing with backpressure can lead to unexpected crashes or resource leaks in your application.
Example Code Snippet: Unhandled Errors
flowable
.subscribe(item -> {
// Simulate an error
if (item % 3 == 0) {
throw new RuntimeException("Simulated error");
}
System.out.println("Processed: " + item);
});
In this code snippet, unhandled exceptions might terminate the entire flow, resulting in data loss.
Solution
Implement robust error handling in your subscribers:
flowable
.subscribe(item -> System.out.println("Processed: " + item),
throwable -> System.err.println("Error: " + throwable.getMessage()));
A Safety Net
Utilizing operators like retry()
or onErrorResumeNext()
can help create a safety net against such issues, preserving functionality even as challenges arise within the data stream.
Final Thoughts
Effectively managing backpressure in RxJava is crucial for building resilient and efficient applications. By understanding and addressing the common pitfalls outlined in this post, you can significantly improve your reactive systems.
Remember:
- Always prefers
Flowable
when dealing with data that can generate backpressure. - Define limits on buffering strategies to prevent resource exhaustion.
- Tune the request mechanics based on your processing needs.
- Implement adequate error handling to ensure your application remains robust.
Handling backpressure is not just an optimization task; it’s a necessary skill for anyone aiming to build scalable systems in Java. As you enhance your RxJava skills, make sure you're aware of these best practices, and feel confident in your ability to manage backpressure efficiently.
For more in-depth learning, feel free to visit RxJava: Documentation for further insights.