Troubleshooting Remote Actor Communication in Akka
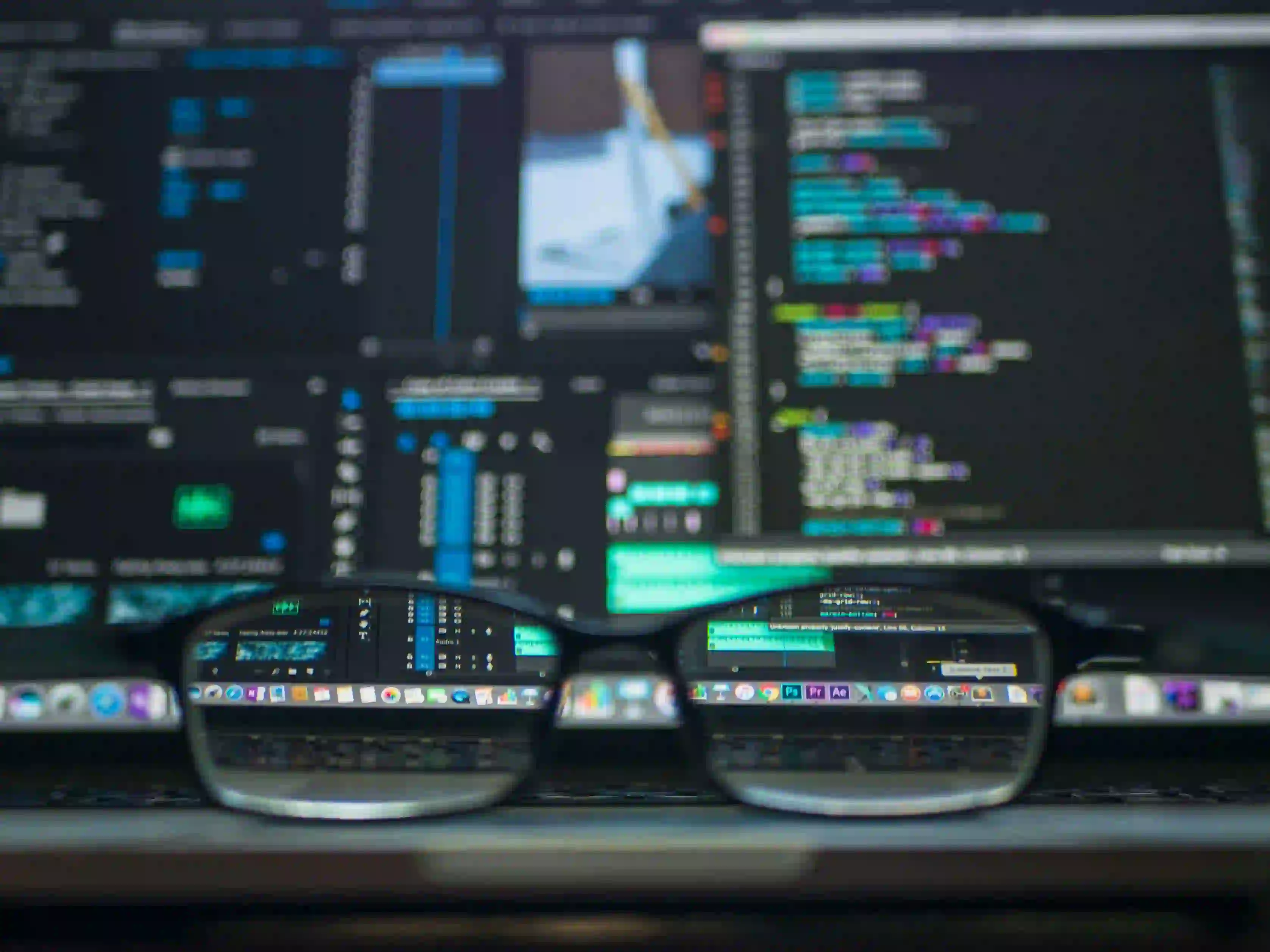
Troubleshooting Remote Actor Communication in Akka
In today's landscape of distributed systems, Akka stands out as a powerful toolkit for building concurrent, resilient applications using the Actor model. However, as with any complex system, remote actor communication can lead to various challenges. This blog post will guide you through common issues seen in Akka's remote communication and how to troubleshoot them effectively.
Understanding Akka's Remote Communication
Akka actors communicate through a well-defined protocol that is surprisingly resilient. Each actor uses its own mailbox, allowing for asynchronous message passing. When actors reside on different nodes in a cluster, they communicate over the network, which introduces complexity.
The significant advantage of Akka's architecture lies in its ability to abstract these interactions. However, network issues, configuration errors, and cluster state management can lead to complications. The most common challenges include:
- Network Connectivity Issues
- Serialization Problems
- Configuration Errors
- Cluster Membership Issues
Let’s delve deeper into each of these areas and how to troubleshoot them.
1. Network Connectivity Issues
Symptoms
- Actors not responding
- Timeouts or delays in message processing
- Unreachable actors
Troubleshooting Steps
-
Ping the Remote Node: Ensure that the network layer is functional by using basic tools like
ping
ortelnet
. -
Check Firewall Rules: Make sure that the ports used by Akka for remote communications are open. Akka typically uses TCP and requires certain ports to be accessible.
-
Look at Akka Logs: Enable logging in Akka to gather insights about failed communication attempts. Adjust your
application.conf
to include a more verbose logging level.
Code Snippet Example: Configure Akka Logging
akka {
loglevel = "DEBUG"
actor {
debug {
receive = on
lifespan = on
autoreceive = on
}
}
}
This configuration will help you catch more details about what is happening with your actors and could pinpoint network-related problems.
2. Serialization Problems
Symptoms
SerializationException
- Unable to send custom messages
- Silent failures
Troubleshooting Steps
-
Ensure Proper Serialization: Check that the messages being passed between actors are serializable. Akka requires that all messages sent over the network implement the
Serializable
interface. -
Use Akka’s Serializer: Utilize Akka’s built-in serializers or configure your own. If you have custom objects, ensure they implement
Serializable
.
Code Snippet Example: Configure a Custom Serializer
akka {
actor {
serializers {
myCustom = "com.example.MyCustomSerializer"
}
serialization-bindings {
"com.example.MyMessage" = myCustom
}
}
}
By specifying your serializer in this manner, you ensure that messages of type MyMessage
are serialized correctly.
3. Configuration Errors
Symptoms
- Connection refused
- Hostname not found
- Actors failing to resolve
Troubleshooting Steps
-
Review Configuration: Go through your
application.conf
to ensure the remote configuration is set correctly. It should include the hostname, port, and protocol settings. -
Check Cluster DNS: If you’re using a clustered setup, ensure that DNS resolution is correctly functioning for all nodes.
Code Snippet Example: Setting Up Basic Remote Configuration
akka.remote.netty.tcp {
hostname = "127.0.0.1"
port = 2552
}
This configuration ensures that the remote actor system can listen on the specified IP and port.
4. Cluster Membership Issues
Symptoms
- Actors not being discovered
- Split-brain scenarios
- Unreachable nodes
Troubleshooting Steps
- Check Cluster Health: Use Akka's
Cluster
API to verify the health status of your nodes.
import akka.cluster.Cluster
import akka.actor.ActorSystem
val system = ActorSystem("MyClusterSystem")
val cluster = Cluster(system)
println(s"Cluster members: ${cluster.state.members}")
This simple Scala snippet will print the current members of the cluster, allowing you to identify missing or unreachable nodes.
- Metrics and Monitoring: Utilize tools like Lightbend Telemetry to visualize and monitor your cluster's health over time.
The Closing Argument
Successful remote communication in Akka is contingent upon a properly configured environment and understanding the complexities of distributed systems. When issues arise, troubleshooting effectively and promptly can make a significant difference in system performance and reliability.
By adhering to the steps outlined in this post, you can address the most common pitfalls in remote actor communication. Remember that remote communication in Akka can be intricate, yet utilizing the right tools and methodologies can pave the way for smooth, efficient actor interactions—regardless of their physical locations.
For further reading on Akka, consider exploring the Akka Documentation and Akka Cluster documentation for in-depth information.
Stay tuned for our next post, where we will explore actor supervision strategies and how to enhance fault tolerance in your Akka applications!