Troubleshooting CI Issues in Java 8 with Jenkins and Sonar
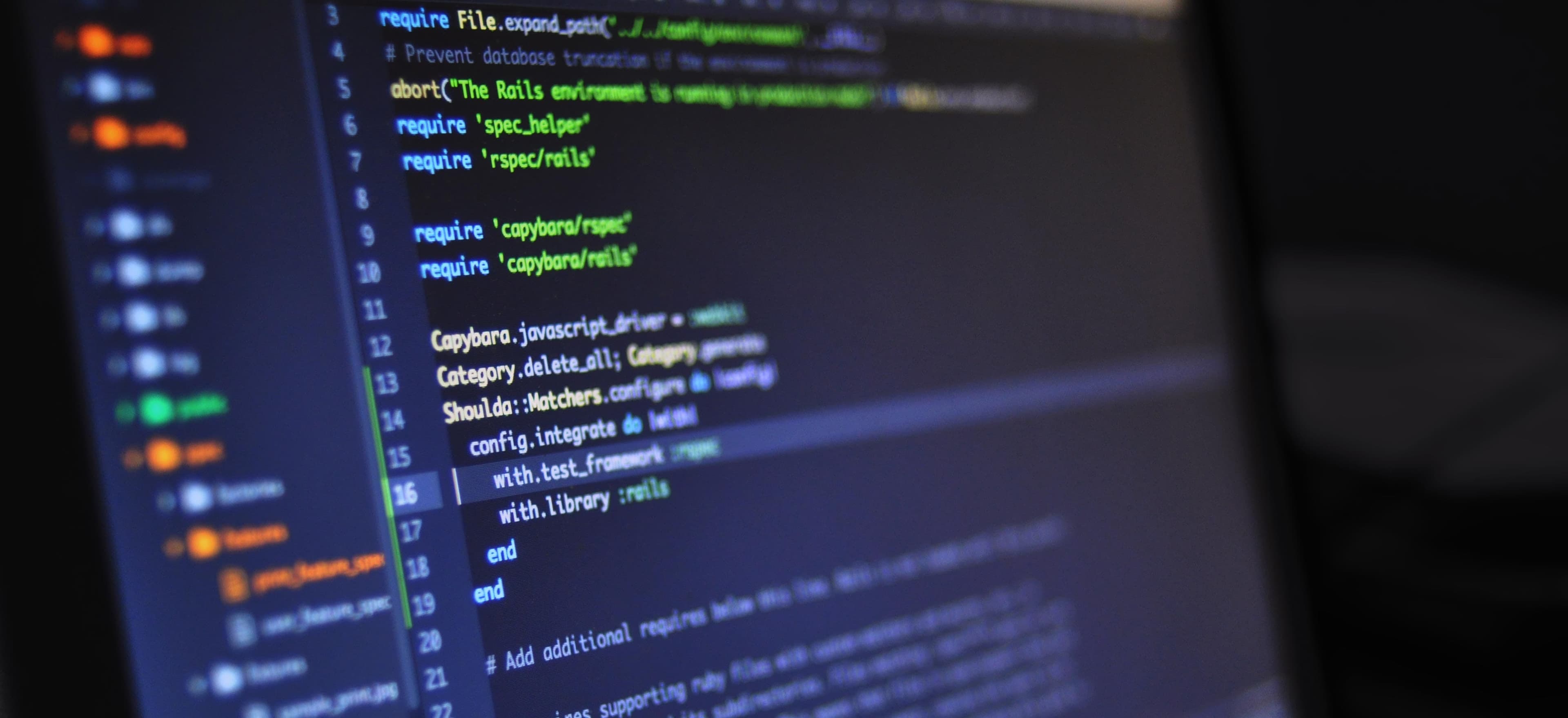
- Published on
Troubleshooting CI Issues in Java 8 with Jenkins and Sonar
Continuous Integration (CI) in software development often involves automated tests, builds, and quality checks to ensure that the code maintains a certain standard before being deployed. Tools such as Jenkins and SonarQube are vital in this process. However, issues can arise during CI pipelines, which can be frustrating. In this post, we'll troubleshoot common CI issues when using Java 8 with Jenkins and SonarQube while providing clear steps and solutions to enhance your development workflow.
Understanding the Setup
Before diving into troubleshooting, let’s briefly review Jenkins and SonarQube.
- Jenkins is an open-source automation server used for automating building, testing, and deploying code. In a Java context, it's typically configured to run Maven or Gradle builds.
- SonarQube is a tool for continuous inspection of code quality. It provides insights into code vulnerabilities, code smells, and duplications that help maintain a healthy codebase.
Prerequisites
- Java 8 installed: Ensure that you are using Java 8, as some dependencies might not work properly with later versions.
- Maven or Gradle: Whichever build tool you prefer for your Java project should be set up.
- Jenkins installed and configured: You should have the Jenkins CI server running.
- SonarQube server installed and running: Make sure SonarQube is accessible.
Common CI Issues and Solutions
Let’s explore some typical issues you might face and how to resolve them.
1. Jenkins Build Fails Due to Missing JDK
Symptoms: Build fails with errors indicating that the Java Compiler cannot be found.
Resolution: Check the global tool configuration in Jenkins.
- Navigate to
Manage Jenkins
>Global Tool Configuration
. - Under the JDK section, make sure that the JDK installation is valid and points to the correct JAVA_HOME.
Example:
# Check your JAVA_HOME environment variable
echo $JAVA_HOME
If the environment variable is not set correctly, you may need to set it in the Jenkins configuration or directly in the shell that starts Jenkins.
2. Maven Build Issues
Symptoms: The Maven build is failing due to dependency issues or plugin compatibility.
Resolution:
Make sure that your pom.xml
file is configured correctly.
Here’s a sample excerpt from a pom.xml
:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
- Ensure that the groupId, artifactId, and version are correct.
- Sometimes, switching the version of a dependency can solve issues related to compatibility.
Run Maven with the debug flag to get more details:
mvn clean install -X
This verbose output can help identify exact points of failure.
3. SonarQube Analysis Failing in Jenkins
Symptoms: The SonarQube analysis fails during the Jenkins pipeline with messages indicating issues with your code quality checks.
Resolution: Ensure that SonarQube is configured properly within Jenkins.
- Navigate to your Jenkins job.
- Make sure that the SonarQube plugin is installed. You can see this by going to
Manage Jenkins
>Manage Plugins
.
Here's a snippet on how to configure the SonarQube scanner in a Jenkins Pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('SonarQube analysis') {
steps {
script {
def scannerHome = tool 'SonarQubeScanner'
withSonarQubeEnv('SonarQubeServer') {
sh "${scannerHome}/bin/sonar-scanner"
}
}
}
}
}
}
- Replace
SonarQubeServer
with the name of your SonarQube server configuration in Jenkins. - Ensure that your
sonar-project.properties
file is correctly set up with all required properties.
4. Code Quality Thresholds Not Being Met
Symptoms: SonarQube reports indicate that quality gates are failing.
Resolution: Check the rules defined in SonarQube and compare them with your code.
In the SonarQube UI, navigate to Quality Gates and verify the thresholds set for code duplication, maintainability, security vulnerabilities, and test coverage.
It’s a good practice to:
- Conduct regular code reviews.
- Enforce coding standards across the team.
To improve the coverage, ensure you are writing unit tests. Here’s a simple JUnit test example:
import org.junit.Assert;
import org.junit.Test;
public class MyServiceTest {
@Test
public void testAdd() {
MyService service = new MyService();
Assert.assertEquals(5, service.add(2, 3));
}
}
Writing good tests not only enhances quality but also helps in securing a better score in SonarQube.
5. Incompatible Plugins or Configurations
Symptoms: Jenkins pipeline or SonarQube analysis fails with class not found or version incompatibility errors.
Resolution: Review the installed plugins for both Jenkins and SonarQube. Ensure they are compatible with the versions you’re using.
To check and update plugins in Jenkins:
- Go to
Manage Jenkins
>Manage Plugins
. - Select the
Updates
tab and install any available upgrades.
In the SonarQube system settings, ensure that the plugins installed there are up to date and compatible with the current Sonar version.
6. Connection Issues Between Jenkins and SonarQube
Symptoms: Jenkins fails to connect to SonarQube.
Resolution: Make sure the network configuration allows Jenkins to reach your SonarQube server.
Check the SonarQube server URL in Jenkins:
- Go to
Manage Jenkins
>Configure System
and look for the SonarQube servers section.
Test connection manually:
# You can use curl to see if SonarQube server is accessible
curl -I http://your-sonarqube-server-url
Ensure that firewall settings or proxy configurations are not blocking the traffic.
Final Thoughts
Troubleshooting CI issues in Java 8 with Jenkins and SonarQube requires a methodical approach. By understanding common pitfalls and their solutions, you can significantly reduce downtime and enhance your development cycle. Always keep your configurations aligned and updated, which not only saves time but also boosts team productivity.
If you run into issues that are not covered here, consult the Jenkins Documentation and the SonarQube Documentation.
By following these guidelines, you’ll be well on your way to maintaining a seamless CI process that reinforces robust code quality practices in your Java projects.
Additional Resources:
Checkout our other articles