Mastering API Security: Tips to Protect Your Credentials
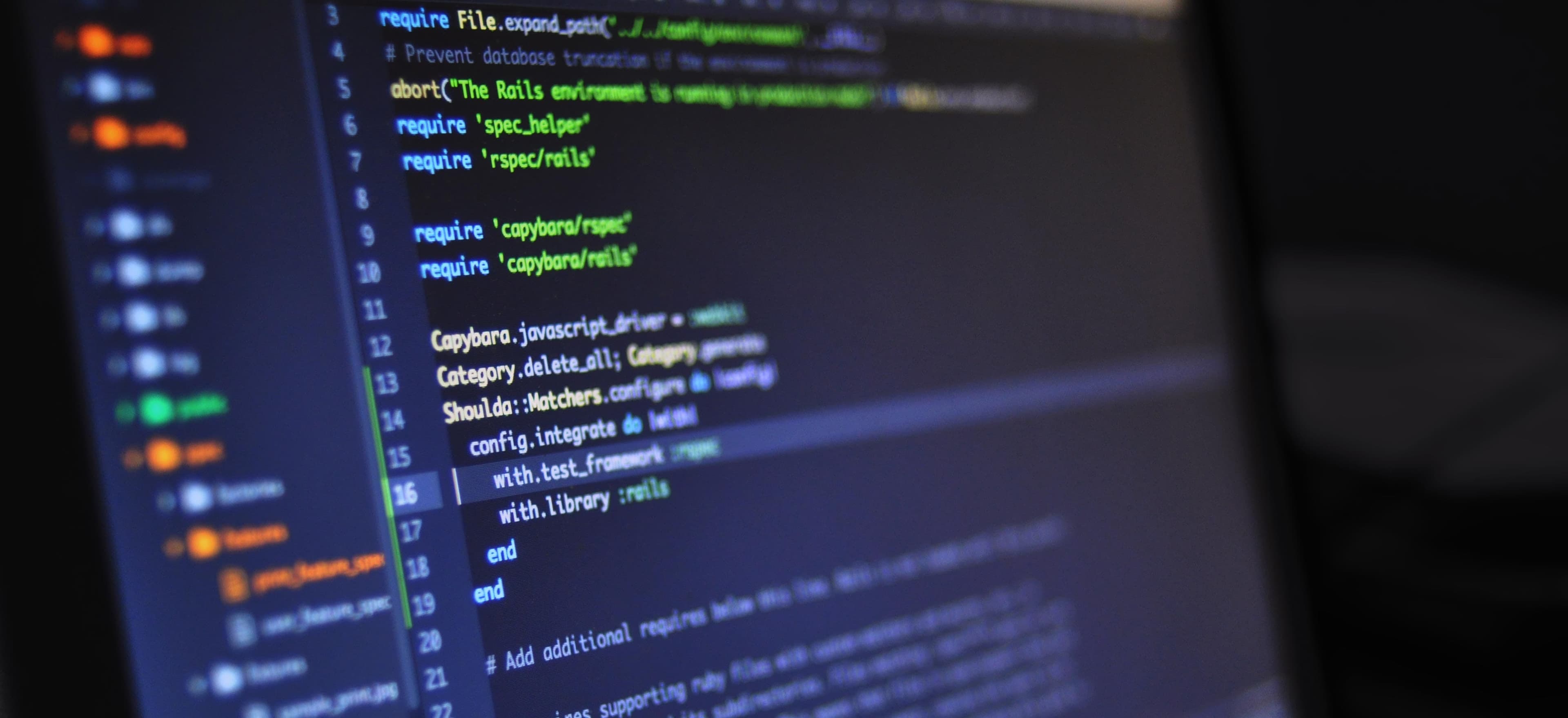
- Published on
Mastering API Security: Tips to Protect Your Credentials
In the digital age, APIs (Application Programming Interfaces) have emerged as the backbone of modern web applications. They connect services and allow seamless data exchange, making them crucial for any development project. However, as the use of APIs increases, so does the risk associated with them. Protecting your API credentials is essential to ensuring the security of your application. This blog post aims to provide actionable tips on mastering API security while highlighting the significance of proper credentials management.
Why API Security Matters
APIs serve as gateways to your application’s data and services. If compromised, attackers can gain unauthorized access, exploit vulnerabilities, and even infiltrate your entire infrastructure. According to a recent report, more than 38% of organizations have experienced a security breach due to improperly secured APIs. Protecting your API and its credentials is not just about avoiding data breaches; it is about safeguarding your organization’s reputation and maintaining user trust.
Best Practices for API Credential Security
1. Use Environment Variables for Storing Credentials
Storing API credentials directly in your code is a bad practice. It makes your credentials visible to anyone who has access to the codebase. Instead, consider using environment variables to manage sensitive information. This method keeps your credentials separate from your application code and makes it easier to manage different environments (development, testing, production).
Example Code Snippet: Using Environment Variables
public class ApiClient {
private String apiKey;
public ApiClient() {
this.apiKey = System.getenv("API_KEY");
}
public void makeApiRequest() {
// Use apiKey securely for authorization
System.out.println("Making request with API key: " + apiKey);
}
}
In this example, the API key is retrieved from an environment variable, preventing it from being hardcoded and exposed.
2. Implement Least Privilege Access
When managing API credentials, always follow the principle of least privilege. Each API user should only have access to the resources they need to perform their function. For example, if your application doesn't need write access to a database, it shouldn’t be granted.
By limiting permissions, you minimize the potential damage in case the API credentials are compromised. This practice is especially important in microservices architectures, where multiple services communicate through APIs.
3. Regularly Rotate Your Credentials
Regularly rotating your API credentials is a vital practice for enhancing security. Frequent changes reduce the chance of prolonged unauthorized access in case of credential leaks. Utilize automated tools for rotating your credentials without downtime.
For many applications, a rotation policy can be established where credentials are changed every three to six months based on risk management strategies. Additionally, implement audit logs to keep track of which credentials have been used and when.
4. Enable Logging and Monitoring
Monitoring API usage can help detect unusual behavior that may indicate a security breach. Set up comprehensive logging to capture every request made using your API credentials. This proactive measure allows you to keep an eye on who is accessing your API and helps identify suspicious activities.
Consider using monitoring services like AWS CloudWatch or Google Cloud Monitoring to analyze and alert you about any anomalies or potential security threats.
5. Use HTTPS Protocols
Transport Layer Security (TLS) encrypts data sent over networks. Always enforce HTTPS for all API communications to protect credentials during transmission. Using HTTPS protects against man-in-the-middle (MITM) attacks, where an attacker could intercept data being transmitted over an unsecured connection.
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class SecureApiClient {
private static final String API_URL = "https://api.yourservice.com/endpoint";
public Response getResponse() throws IOException {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(API_URL)
.build();
return client.newCall(request).execute();
}
}
In this snippet, HTTPS is enforced during the API call to ensure secure data transmission.
6. Implement Rate Limiting and Throttling
Rate limiting restricts the number of requests a client can make within a specific timeframe. This protects your API from abuse, such as denial-of-service (DoS) attacks. By implementing rate limiting and throttling, you can ensure that a single user does not overwhelm your API, thus safeguarding its availability.
Consider using frameworks like Spring WebFlux for implementing these measures efficiently in your application.
7. Secure Your APIs with API Gateways
API gateways serve as a protective layer between your APIs and the outside world. They offer various features, including authentication and authorization, traffic management, caching, and more. Utilizing an API gateway simplifies the management of security policies across multiple APIs.
Gateways like Kong or Amazon API Gateway provide built-in features to enhance API security.
To Wrap Things Up
Securing your API credentials is not just about technology; it’s about establishing a culture of security within your organization. By following the best practices outlined above, you can significantly reduce the risks associated with API usage.
Remember, as threats evolve, so should your security measures. Stay proactive, stay informed, and always prioritize the safety of your API credentials. With the right approach, mastering API security can become a fundamental part of your development strategy, protecting both your application and its users.
Further Reading
- For more in-depth information on API security, check out OWASP's API Security Top 10.
- Consider reading Securing APIs: A Guide for Developers for a comprehensive understanding of potential vulnerabilities.
By implementing these best practices and maintaining a proactive stance on security, you can master the realm of API security and ensure the safety of your credentials in a constantly evolving digital landscape.
Checkout our other articles