Common OAuth 2.0 Configuration Mistakes in Spring Boot
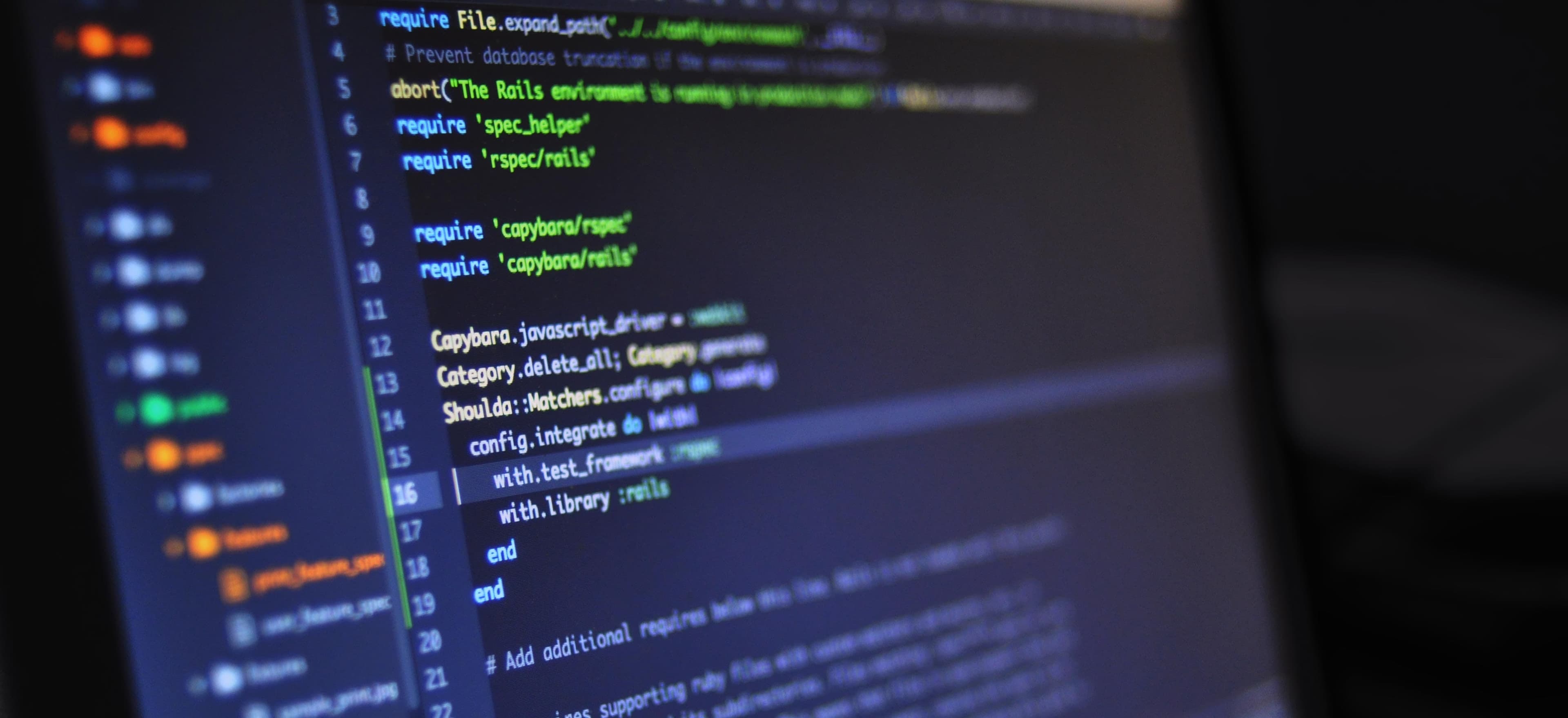
- Published on
Common OAuth 2.0 Configuration Mistakes in Spring Boot
OAuth 2.0 is a widely adopted authorization framework that allows applications to securely access user data without sharing credentials. However, integrating OAuth 2.0 into a Spring Boot application can be tricky, especially for those who are new to the framework or the protocol itself. This blog post explores some common misconfigurations and pitfalls developers encounter when setting up OAuth 2.0 in Spring Boot applications.
Setting the Scene to OAuth 2.0
Before we dive into configuration mistakes, let's take a moment to understand OAuth 2.0. It's important to note that OAuth 2.0 is not an authentication protocol but rather an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service.
For more information, check out the official OAuth 2.0 specification.
Setting Up Spring Boot with OAuth 2.0
Spring Boot simplifies the OAuth 2.0 configuration using Spring Security. Developers typically leverage Spring Security's built-in support to create secure applications quickly. Here's a basic dependency example to get started:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
This dependency brings in the necessary components for OAuth 2.0 client support. However, rushing through the configuration can lead to several common mistakes. Let's discuss some of them.
Common Configuration Mistakes
1. Ignoring HTTPS
Mistake: A frequent oversight is not enforcing HTTPS for OAuth 2.0 applications.
Why it's important: OAuth 2.0 relies on secure channels for transmitting tokens. If your application is using HTTP, access tokens can be intercepted.
How to fix: Ensure that your application is deployed over HTTPS. Additionally, you can set the application to refuse HTTP requests in the Spring Security configuration.
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requiresChannel()
.anyRequest()
.requiresSecure();
}
2. Misconfigured Client Secret
Mistake: Developers often misconfigure the client secret or mistakenly expose it in public repositories.
Why it's important: The client secret is a critical piece of data that authenticates your application. Exposing it can lead to unauthorized access.
How to fix: Use environment variables or a secure vault service to store sensitive data and avoid hardcoding it in your application.
spring:
security:
oauth2:
client:
registration:
my-client:
client-id: ${CLIENT_ID}
client-secret: ${CLIENT_SECRET}
3. Improper Scopes Definition
Mistake: Specifying either too many or too few scopes can lead to security risks or functionality issues.
Why it's important: Scopes define the level of access that the application will obtain. Over-scoping can lead to more data being accessed than necessary.
How to fix: Always use the minimum scopes necessary for your application. Here is how to define scopes succinctly.
spring:
security:
oauth2:
client:
registration:
my-client:
scope: read,write
4. Not Handling Token Expiry
Mistake: Ignoring token expiry and refresh mechanisms is a common error.
Why it's important: OAuth tokens, especially access tokens, often have a limited lifespan. Not managing token expiry can lead to failed requests when the application tries to use an expired token.
How to fix: Implement a token refresh strategy. Spring Security handles this automatically if configured correctly. Verify that your token provider supports refresh tokens and that you have set them up in your configuration.
5. Failing to Validate State Parameter
Mistake: Some developers neglect the state
parameter in OAuth flows.
Why it's important: The state
parameter helps prevent CSRF attacks by maintaining application state during the authorization process.
How to fix: Ensure that a unique state parameter is generated for each request. This can be done by extending the authorization request:
OAuth2AuthorizationRequest authorizationRequest =
OAuth2AuthorizationRequest
.authorizationCode()
.clientId("client-id")
.redirectUri("{baseUrl}/login/oauth2/code/{registrationId}")
.state(UUID.randomUUID().toString())
.build();
Additional Configuration Considerations
6. Inconsistent Redirect URIs
Mistake: Mismatches between registered redirect URIs and the ones used within your application.
Why it's important: An incorrect redirect URI can lead to authorization failures.
How to fix: Double-check that the redirect URI configured in your OAuth provider matches exactly with the one specified in your Spring Boot application.
spring:
security:
oauth2:
client:
registration:
my-client:
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
7. Overlooking User Info Endpoint
Mistake: Developers might forget to configure the User Info endpoint, particularly when using OpenID Connect.
Why it's important: Without the User Info endpoint, your application may not retrieve user profile information necessary for your functionality.
How to fix: Ensure that the User Info endpoint is correctly configured as follows:
spring:
security:
oauth2:
client:
provider:
my-client:
user-info-uri: https://provider.com/userinfo
8. Not Utilizing Spring Security Features
Mistake: Failing to leverage Spring Security's capabilities to manage user sessions and roles.
Why it's important: Properly managing user sessions and roles helps secure your application effectively.
How to fix: Use Spring Security's features to ensure that users with specific roles have appropriate access to resources.
@PreAuthorize("hasRole('ROLE_USER')")
@GetMapping("/user")
public String getUserDetails() {
return "User details here";
}
The Last Word
Configuring OAuth 2.0 in a Spring Boot application doesn't have to be a daunting task. By being aware of common mistakes and following best practices, you can create a secure and reliable application that utilizes OAuth 2.0 for authorization.
Recommended Resources
- Spring Security Reference
- OAuth 2.0 Security Best Practices
- Spring Boot OAuth 2.0 With Authorization Code Flow
By understanding and correcting these common mistakes, you're on your way to mastering OAuth 2.0 configuration in Spring Boot. Happy coding!