Boosting ActiveMQ Performance: Common Pitfalls to Avoid
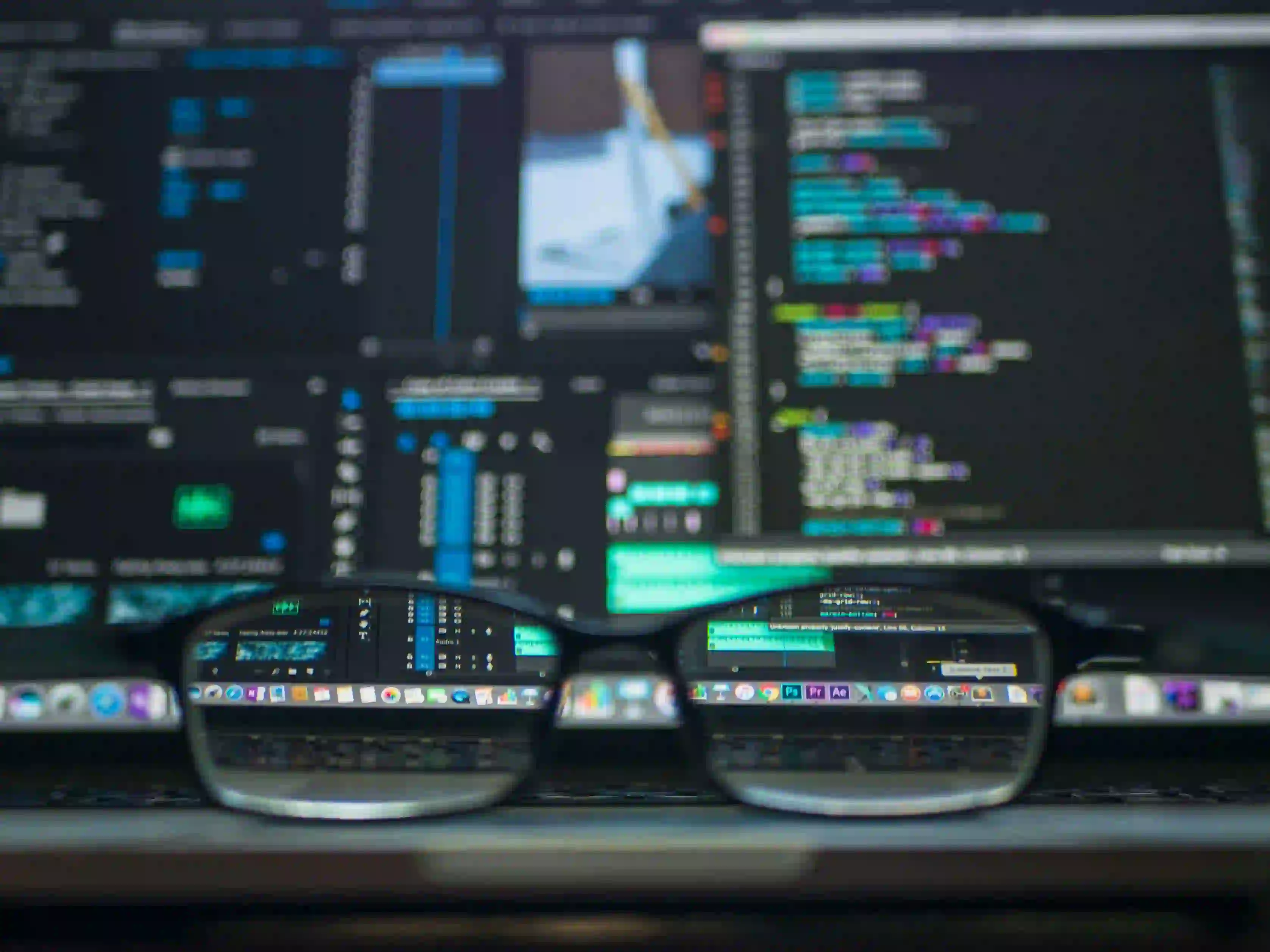
Boosting ActiveMQ Performance: Common Pitfalls to Avoid
ActiveMQ is a robust open-source messaging system that facilitates communication between different applications. While it boasts many features and benefits, ensuring optimal performance requires understanding and addressing common pitfalls. In this blog post, we will discuss ways to boost ActiveMQ performance by avoiding these pitfalls, offering practical code snippets, and providing links for further reading.
Understanding ActiveMQ Architecture
Before diving into common pitfalls, let's grasp the architecture of ActiveMQ. The broker, which routes messages, plays a crucial role. Clients can connect to the broker via different protocols, including OpenWire, AMQP, MQTT, and STOMP. Their interactions directly impact performance.
To understand how to improve performance, we must first identify common issues that can lead to poor performance.
Common Pitfalls in ActiveMQ Performance
1. Improper Configuration
One of the most frequent mistakes is using the default configuration. While it may work for initial testing, it often leads to poor performance under load.
Solution: Always tune ActiveMQ's configuration based on your use case. Assign appropriate values to parameters like maxConnections
, maxActive
, and memoryLimit
.
<broker xmlns="http://activemq.apache.org/schema/core" brokerName="localhost" dataDirectory="${activemq.data}">
<systemUsage>
<systemUsage>
<memoryUsage>
<memoryUsage limit="64mb"/>
</memoryUsage>
<storeUsage>
<storeUsage limit="1gb"/>
</storeUsage>
<tempUsage>
<tempUsage limit="1gb"/>
</tempUsage>
</systemUsage>
</systemUsage>
</broker>
By adjusting these parameters, you can ensure that your broker has sufficient memory and storage, improving message handling capacity.
2. Ignoring Persistent Messages
Another pitfall is neglecting to define the persistence strategy of your messages. Using non-persistent messages for critical transactions can lead to data loss.
Solution: Use persistent delivery for important messages, ensuring they are logged to disk. This is achieved by setting the delivery mode when sending messages.
import javax.jms.*;
ConnectionFactory connectionFactory = new ActiveMQConnectionFactory("tcp://localhost:61616");
Connection connection = connectionFactory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Destination destination = session.createQueue("TestQueue");
MessageProducer producer = session.createProducer(destination);
// Set delivery mode to persistent
producer.setDeliveryMode(DeliveryMode.PERSISTENT);
// Create a message
TextMessage message = session.createTextMessage("Hello, ActiveMQ!");
producer.send(message);
Persistent messages assure that even if there's a broker failure, the message will be delivered once the system recovers.
3. Inefficient Message Serialization
Serialization impacts message size and processing time. If a message takes longer to serialize, it can lead to bottlenecks.
Solution: Use efficient serialization strategies, such as Protocol Buffers or Avro, instead of Java's default serialization.
Here is an example using Protocol Buffers:
- Define your message format in a
.proto
file:
syntax = "proto3";
message User {
string name = 1;
int32 id = 2;
}
-
Compile this file using the Protocol Buffers compiler to generate Java classes.
-
Use the generated classes to serialize your messages:
User user = User.newBuilder().setName("John Doe").setId(123).build();
byte[] serializedUser = user.toByteArray();
Utilizing efficient serialization technologies can help reduce overhead and improve overall throughput.
4. Not Using Connection Pooling
Creating multiple connections to the broker can lead to unnecessary overhead. Each connection consumes resources, leading to decreased performance.
Solution: Implement connection pooling. Libraries like Apache Commons Pool can help manage resource allocation efficiently.
Here is an example of implementing a simple connection pool:
import org.apache.commons.pool2.impl.GenericObjectPool;
import org.apache.commons.pool2.PooledObjectFactory;
// Define a factory that generates connections
class ConnectionFactory implements PooledObjectFactory<Connection> {
public Connection create() throws Exception {
// Create and return a new connection
return new ActiveMQConnectionFactory("tcp://localhost:61616").createConnection();
}
// Implement other required methods...
}
GenericObjectPool<Connection> pool = new GenericObjectPool<>(new ConnectionFactory());
Connection connection = pool.borrowObject();
// Use the connection...
pool.returnObject(connection);
Connection pooling reuses existing connections, enhancing performance and reducing resource consumption.
5. Ignoring Message Acknowledgment Modes
Understanding acknowledgment modes can tremendously affect the system's performance. The wrong acknowledgment mode can lead to delayed message delivery.
Solution: Choose the appropriate acknowledgment mode based on your application's requirements.
Session session = connection.createSession(false, Session.CLIENT_ACKNOWLEDGE);
// Or use Session.AUTO_ACKNOWLEDGE for automatic handling
By choosing Session.CLIENT_ACKNOWLEDGE
, the receiver has control over when to acknowledge message processing, which can be more efficient in certain scenarios.
6. Not Monitoring ActiveMQ Metrics
ActiveMQ provides various metrics and monitoring options, including JMX beans, to help track performance. Ignoring these can lead to performance issues that remain unnoticed until it's too late.
Solution: Enable monitoring and regularly check metrics such as message rates, queue sizes, and broker load. Tools like JConsole or VisualVM can visualize these metrics.
By monitoring the metrics, you can identify bottlenecks and adjust configurations accordingly.
Additional Resources
- ActiveMQ Documentation
- Best Practices for Using ActiveMQ
- Java Connection Pooling with Apache Commons Pool
To Wrap Things Up
Improving ActiveMQ performance involves avoiding common pitfalls through thoughtful configuration, serialization, acknowledgment handling, and resource management. By applying the solutions outlined in this post, users can harness the true potential of ActiveMQ, ensuring efficient communication between applications. Remember, performance monitoring is essential for ongoing optimization.
Happy coding!