Overcoming Challenges in Serverless Batch Processing Workflows
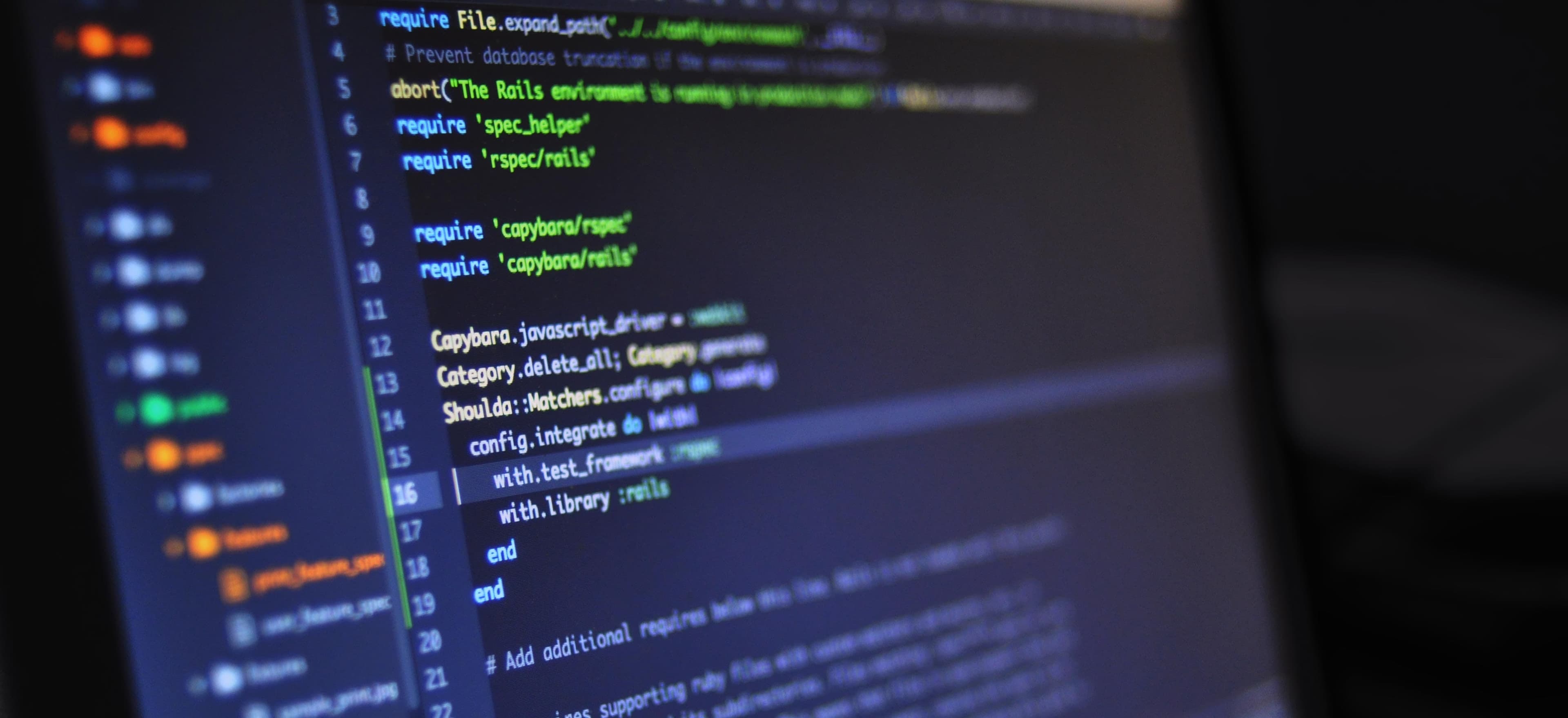
- Published on
Overcoming Challenges in Serverless Batch Processing Workflows
In today’s data-driven world, the demand for efficient batch processing is on the rise. Serverless architecture has emerged as a popular solution, helping organizations minimize costs and improve scalability. However, while serverless computing boasts numerous advantages, it’s not without its challenges. This blog post will explore the pitfalls and potential solutions for overcoming challenges in serverless batch processing workflows.
Understanding Serverless Architecture
Serverless architecture allows developers to focus on code rather than infrastructure management. You write your function, put it in the cloud, and the cloud provider manages the servers. But why is this a boon for batch processing?
- Scalability: Serverless functions can scale automatically depending on demand.
- Cost Efficiency: You only pay for the time your functions run, leading to significant savings.
- Reduced Overhead: No need to manage servers means less operational burden.
However, with these benefits come challenges that can hinder the efficiency of batch processing workflows.
Challenges in Serverless Batch Processing
1. Cold Starts
One major challenge with serverless architectures is the "cold start" issue. When a function is called after being idle for a while, the cloud provider takes some time to spin it up, causing latency. This can be cumbersome when dealing with batch processing where speed matters.
Solution: Use warm-up requests to keep functions alive. Here's an example using AWS Lambda with a CloudWatch Events rule to schedule invocations:
import com.amazonaws.services.lambda.AWSLambda;
import com.amazonaws.services.lambda.AWSLambdaClientBuilder;
import com.amazonaws.services.lambda.model.InvokeRequest;
public class WarmUp {
public void warmUpFunction() {
AWSLambda lambdaClient = AWSLambdaClientBuilder.defaultClient();
InvokeRequest invokeRequest = new InvokeRequest()
.withFunctionName("YourFunctionName")
.withInvocationType("RequestResponse"); // This keeps it warm
lambdaClient.invoke(invokeRequest);
}
}
In the code above, the warmUpFunction
method keeps the Lambda function from timing out due to inactivity. Regular invocation minimizes latency for subsequent requests.
2. Execution Time Limits
Most serverless platforms impose a limit on function execution time (AWS Lambda, for example, limits you to 15 minutes). This can be problematic for long-running batch tasks.
Solution: Break your workloads into smaller chunks. By segmenting your data into manageable batches, you can ensure each function call completes within the allowed limits. Here's an illustrative snippet:
public class BatchProcessor {
public void processLargeDataset(List<Data> dataset) {
final int BATCH_SIZE = 1000;
for (int i = 0; i < dataset.size(); i += BATCH_SIZE) {
List<Data> batch = dataset.subList(i, Math.min(i + BATCH_SIZE, dataset.size()));
processBatch(batch);
}
}
private void processBatch(List<Data> batch) {
// Processing logic here
}
}
By batching your data, you avoid hitting execution time limits and can process large datasets efficiently.
3. Monitoring and Debugging
Serverless applications can be harder to monitor and debug due to their distributed nature. Traditional monitoring tools may not work seamlessly.
Solution: Implement observability tools tailored for serverless. Tools like AWS CloudWatch and Datadog can help you track function performance and errors. Logging can be done using:
import java.util.logging.Logger;
public class MyFunction {
private static final Logger logger = Logger.getLogger(MyFunction.class.getName());
public void handler(String input) {
logger.info("Processing input: " + input);
try {
// Your processing logic
} catch (Exception e) {
logger.severe("Error processing input: " + e.getMessage());
throw e; // Re-throw to allow for further handling
}
}
}
Logging keeps track of operations, making it easier to diagnose issues when they arise.
4. Data Transfer and Network Latency
With serverless compute nodes usually operating outside your local environment, delays from data transfer can lead to performance hits, especially with large datasets.
Solution: Use cloud-native storage solutions. If you're working with AWS, services like S3 can significantly reduce latencies. Instead of transferring data, your functions should directly read from cloud storage.
For example:
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
public class S3DataFetcher {
private AmazonS3 s3Client;
public S3DataFetcher() {
this.s3Client = AmazonS3ClientBuilder.defaultClient();
}
public String fetchData(String bucketName, String key) {
return s3Client.getObjectAsString(bucketName, key);
}
}
This approach minimizes the latencies associated with data transfer and optimizes the speed of your batch processing workflows.
5. Array Dependencies
When processing batches, you may face array dependencies that dictate the order of operations. In conventional environments, this is manageable but can complicate serverless processing.
Solution: Use state management or coordination services like AWS Step Functions. Step Functions manage state and orchestration easily.
Example
Here's how you can use AWS Step Functions to coordinate a batch processing workflow:
{
"Comment": "A simple AWS Step Functions state machine that processes batches.",
"StartAt": "ProcessBatch",
"States": {
"ProcessBatch": {
"Type": "Task",
"Resource": "arn:aws:lambda:region:account-id:function:YourFunctionName",
"Next": "CheckCompletion"
},
"CheckCompletion": {
"Type": "Choice",
"Choices": [
{
"Variable": "$.done",
"BooleanEquals": true,
"Next": "Success"
}
],
"Default": "ProcessBatch"
},
"Success": {
"Type": "Succeed"
}
}
}
By utilizing AWS Step Functions, you gain more control over ensuring each process runs in order and that any dependencies you have are respected.
Final Thoughts
While serverless architectures offer significant advantages for batch processing workflows, they come with their own set of challenges. Understanding and tackling these challenges proactively can lead to efficient, scalable, and cost-effective data processing solutions.
For further reading on serverless architectures and batch processing, check out AWS Serverless Batch Processing and Google Cloud's Serverless Approach.
By employing the strategies discussed, businesses can successfully handle their batch processing needs while enjoying the benefits that serverless computing has to offer.