Optimizing JPA Performance in GlassFish: Common Pitfalls
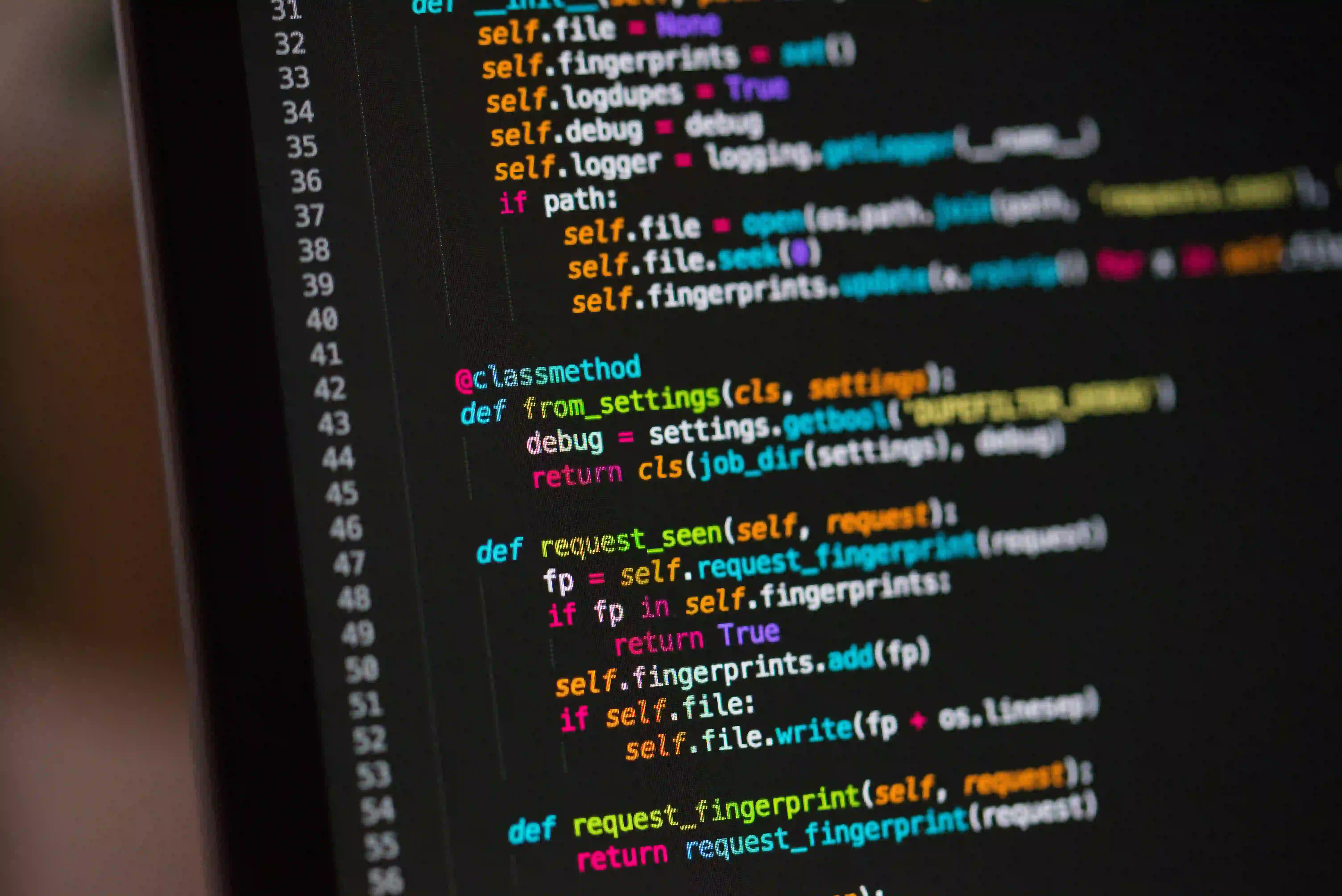
Optimizing JPA Performance in GlassFish: Common Pitfalls
Java Persistence API (JPA) has solidified its place as a fundamental technology in enterprise Java applications for managing relational data. However, as with any technology, performance issues can arise, particularly when deployed in environments like GlassFish. This blog post revisits some common pitfalls in JPA performance and looks into strategies to help you attain optimal performance in GlassFish.
Understanding JPA and GlassFish
Java Persistence API (JPA) provides a set of rules for object-relational mapping (ORM) in Java applications. As an interface, it simplifies database operations by allowing developers to focus on their entities while the underlying framework handles database interactions.
GlassFish, a popular open-source application server, is known for its ease of use and rich set of features. It fully supports JPA, making it a common choice for deploying JPA-based applications.
Common Pitfalls in JPA Performance
1. N+1 Select Problem
One of the most infamous performance pitfalls is the N+1 select problem. This issue arises when querying a collection of parent entities, only to fetch child entities one-by-one in subsequent queries.
List<Order> orders = entityManager.createQuery("SELECT o FROM Order o", Order.class).getResultList();
for (Order order : orders) {
List<Item> items = order.getItems();
}
Why this is a Problem: If there are 10 orders, the above code will execute 11 SQL queries: 1 for orders and 10 for items, leading to inefficient resource usage.
Solution: Use eager fetching to optimize this:
List<Order> orders = entityManager.createQuery("SELECT o FROM Order o JOIN FETCH o.items", Order.class).getResultList();
This single query retrieves both orders and their associated items, reducing the number of round trips to the database.
2. Lack of Indexing
Not using proper database indexing can make entity retrieval slow, especially with large datasets. For example, if you often search for users by email, but the email field isn't indexed:
SELECT * FROM users WHERE email = 'example@example.com';
Why this is a Problem: Without indexing, the database performs a full table scan, severely impacting retrieval times as the data grows.
Solution: Create an index on the email column:
CREATE INDEX idx_email ON users(email);
This allows the database to quickly locate data, increasing query efficiency.
3. Improper Cascade Operations
Cascade operations can simplify data management but may lead to unintended performance overhead when used improperly. For instance, cascading a delete operation through numerous child entities can significantly slow down execution times.
@Entity
public class Category {
@OneToMany(mappedBy = "category", cascade = CascadeType.ALL)
private List<Product> products;
}
Why this is a Problem: If you delete a category with many products, JPA might batch delete each product one by one, resulting in multiple DELETE statements.
Solution: Be explicit with your cascade types. Only use cascading operations when necessary. For example, use CascadeType.PERSIST
only when you create new objects in your application logic.
4. Ignoring Batch Processing
Many developers rely on individual inserts or updates, which can be slow when processing large volumes of data.
for (int i = 0; i < users.size(); i++) {
entityManager.persist(users.get(i));
}
Why this is a Problem: Each persist
call results in a separate transaction, which significantly slows down the insert process.
Solution: Utilize batch processing, which allows multiple operations to be executed in a single transaction.
for (int i = 0; i < users.size(); i++) {
entityManager.persist(users.get(i));
if (i % 50 == 0) { // Flush and clear every 50
entityManager.flush();
entityManager.clear();
}
}
This method reduces the number of transactions, drastically improving performance.
5. Incorrect Fetch Strategy
Choosing the wrong fetch strategy can lead to performance bottlenecks. The default fetch type is set to LAZY
, but developers may inadvertently switch to EAGER
fetching, resulting in over-fetching of data.
@OneToMany(fetch = FetchType.EAGER)
private List<Item> items;
Why this is a Problem: EAGER
fetching retrieves all related entities, which can lead to excessive data being loaded into memory, negatively impacting performance.
Solution: Choose the right strategy. Use LAZY
in cases where fetching related data is optional or when those relationships are not frequently accessed.
6. Unoptimized Queries
Finalizing an application without first optimizing queries can lead to serious performance lags. Developers sometimes use the default JPA-generated queries without reviewing execution plans.
Why this is a Problem: Default JPA queries arenβt always optimal. They might not leverage indexes or might fetch more data than necessary.
Solution: Use named queries or native SQL queries to refine performance.
@NamedQuery(name = "User.findByEmail", query = "SELECT u FROM User u WHERE u.email = :email")
This approach can lead to better performance because you can tailor the query to your needs and add further optimizations.
Monitoring and Profiling
While fixing common pitfalls is essential, it is also crucial to monitor and profile your application for potential performance issues continuously. Tools like Hibernate Profiler, JVisualVM, or Java Mission Control can help detect issues in data fetching and provide insights into memory usage.
Key Takeaways
Optimizing JPA performance in GlassFish requires awareness of common pitfalls and a commitment to adopting best practices. By addressing issues like the N+1 select problem, proper indexing, cascading operations, batch processing, and optimized queries, you can significantly enhance the performance of your applications.
For further reading on JPA performance optimization and best practices, consider exploring the official Java EE documentation or Hibernate ORM documentation.
By implementing these strategies, you will not only improve the performance of your JPA-based applications but also increase their scalability and efficiency in your GlassFish deployment.