How Project Jigsaw Can Break Your Java Code: Key Issues
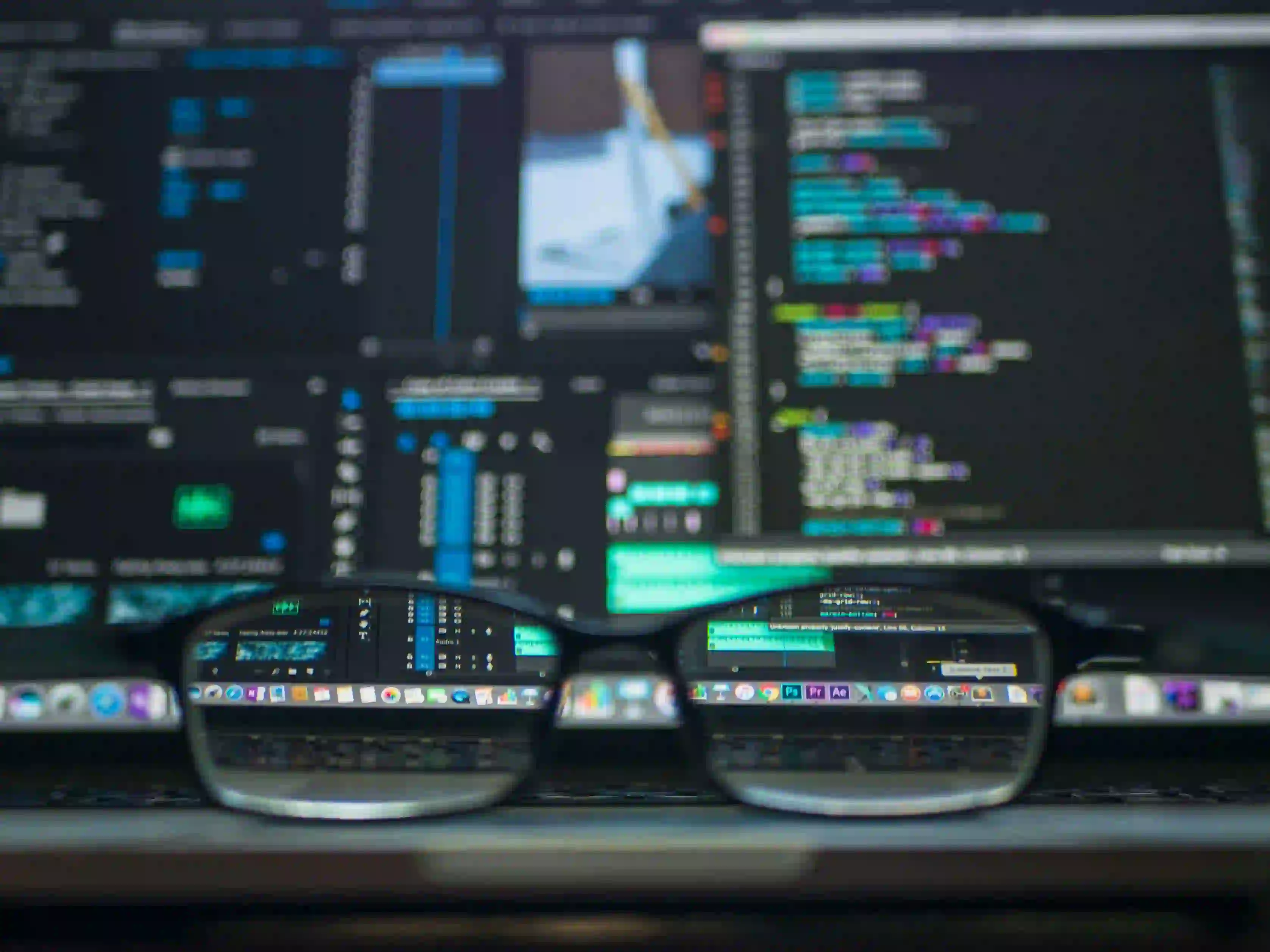
How Project Jigsaw Can Break Your Java Code: Key Issues
Java has been a dominant player in the programming world for over two decades. With its high portability and robust performance, it's no surprise that it is often the first choice for developers. With the release of Java 9, Oracle introduced a transformative feature in the Java ecosystem known as Project Jigsaw, which aimed to modularize the Java platform. While this was an excellent step towards improving modularity, it brought some issues and challenges for existing Java codebases. In this blog post, we will delve into how Project Jigsaw can disrupt your Java code and discuss key issues surrounding this change.
Understanding Project Jigsaw
Before we dive into the complications that Jigsaw can introduce, it’s essential to understand what Project Jigsaw is. At its core, Jigsaw is a modular system that allows developers to define modules and their dependencies clearly. This means you can encapsulate your code better, specify what is publicly exposed, and differentiate between public and internal code.
Why Modularization Matters
Modularization has several advantages:
- Encapsulation: Modules allow you to hide implementation details while exposing only what's necessary.
- Dependency Management: Clear dependencies can lead to better package management and reduce potential conflicts.
- Improved Performance: Smaller modules can improve the startup time and reduce the memory footprint of applications.
However, with these advantages come potential pitfalls.
Key Issues Introduced by Project Jigsaw
1. Code Compatibility Issues
One of the most significant impacts of Project Jigsaw is the potential for incompatibility with existing code. Libraries or applications that were not designed with modularization in mind may experience runtime errors or compilation errors due to module boundaries.
For example, if a library directly accesses classes from the java.base
module but does not adequately declare the dependencies, it can run into issues:
module com.example.module {
requires java.base; // Declare dependency on the base module
}
If you forget to declare a required module, your application won't compile, leading to functionality loss.
2. Class Visibility and Accessibility
In non-modularized Java applications, all classes are generally accessible. However, with Project Jigsaw, you need to declare which packages are exported using the exports
directive. Failure to do so can lead to java.lang.IllegalAccessError
.
Consider the following code snippet:
module com.example.module {
exports com.example.publicapi; // This package is public
//com.example.internalapi - not exported and thus inaccessible outside
}
In the above module definition, com.example.publicapi
is accessible, while com.example.internalapi
is hidden. If another module tries to access com.example.internalapi
, it will face accessibility issues.
3. Reflection and Runtime Access
Reflection is a powerful feature in Java that allows developers to inspect classes, methods, and fields at runtime. Project Jigsaw can complicate things when it comes to reflective access. Modifiers in Jigsaw limit reflection so that you may not be able to access classes or fields as you did before.
For example:
public class ReflectionExample {
public void invokePrivateMethod() throws Exception {
Method privateMethod = MyClass.class.getDeclaredMethod("myPrivateMethod");
privateMethod.setAccessible(true); // Will throw IllegalAccessException if not accessible
privateMethod.invoke(new MyClass());
}
}
If MyClass
isn’t exported, or the module does not allow reflection, an IllegalAccessException
will occur. You'll often need to adjust the module's opens
statement to allow deep reflection:
module com.example.module {
opens com.example.internalapi; // Allow deep reflective access
}
4. Tooling and Build System Compatibility
As compared to earlier versions of Java, many build tools (like Maven and Gradle) had to adapt to accommodate modular systems. If you're using older dependencies that haven’t been modularized, they might conflict with newer Jigsaw modules.
For instance, attempting to include a non-modular JAR in a modular project could lead to issues where classes cannot be resolved. Here is a misleading setup leading to a build failure:
<dependency>
<groupId>com.example</groupId>
<artifactId>non-modular-library</artifactId>
<version>1.0.0</version>
</dependency>
In the above example, if non-modular-library
does not declare its dependencies (be it because it's not modular or improperly bundled), it can lead to a myriad of build issues.
5. Jigsaw’s Impact on Third-Party Libraries
Many libraries in the Java ecosystem are still not modularized. When consuming these libraries in Jigsaw projects, you might run into numerous issues. The lack of modularity can lead to class visibility problems, as those libraries might not properly declare exports or dependencies.
For instance, a non-modular library that relies on internal implementations from the base Java library might face issues when consumed in a modular project. As developers, we need to remain aware of the libraries being utilized and ensure they have been updated or consider alternatives that comply with Jigsaw's requirements.
6. Migration Complexity
Migrating existing codebases to a modular structure can be a daunting task. The amount of existing legacy code you have to refactor quickly escalates, especially if the code was built without modularity in mind.
You might need to:
- Analyze your package structure and understand dependencies thoroughly.
- Abstract and refactor code where needed.
- Configure your module descriptor (module-info.java) correctly.
This migration may prove complicated for very large or heavily intertwined codebases.
Mitigating the Jigsaw Risks
While Project Jigsaw presents challenges, awareness and proper adjustments can significantly ease the transition. Here are some strategies:
-
Audit Your Dependencies: Check the libraries you're using to determine their compatibility with Project Jigsaw. Ensure they have been modularized.
-
Refactor Gradually: Don’t try to modularize everything at once. Start with small sections of your application to identify issues before a full migration.
-
Update Build Tools: Ensure your build tools are up-to-date with the latest version to handle Java 9 and above features effectively.
-
Testing: Rigorously test all code during and after migration to uncover potential issues early.
-
Explore the Java Modules Documentation: Oracle has an extensive guide to modules that provides comprehensive details.
The Last Word
Project Jigsaw introduces a new level of organization and structure in the Java ecosystem that can greatly enhance your development experience. However, the pitfalls and challenges linked to legacy codebases, accessibility, and third-party libraries cannot be overlooked.
Taking a gradual approach, staying informed, and employing the best practices will help you transition smoothly into this new era of Java modularization.
For additional information, you can visit the official Jigsaw overview to better understand how these systems work together.
Remember, awareness is the first step to ensuring your Java projects remain stable and adaptable in an ever-changing landscape.