Solving Method Call Resolution Issues in Java with JavaSymbolSolver
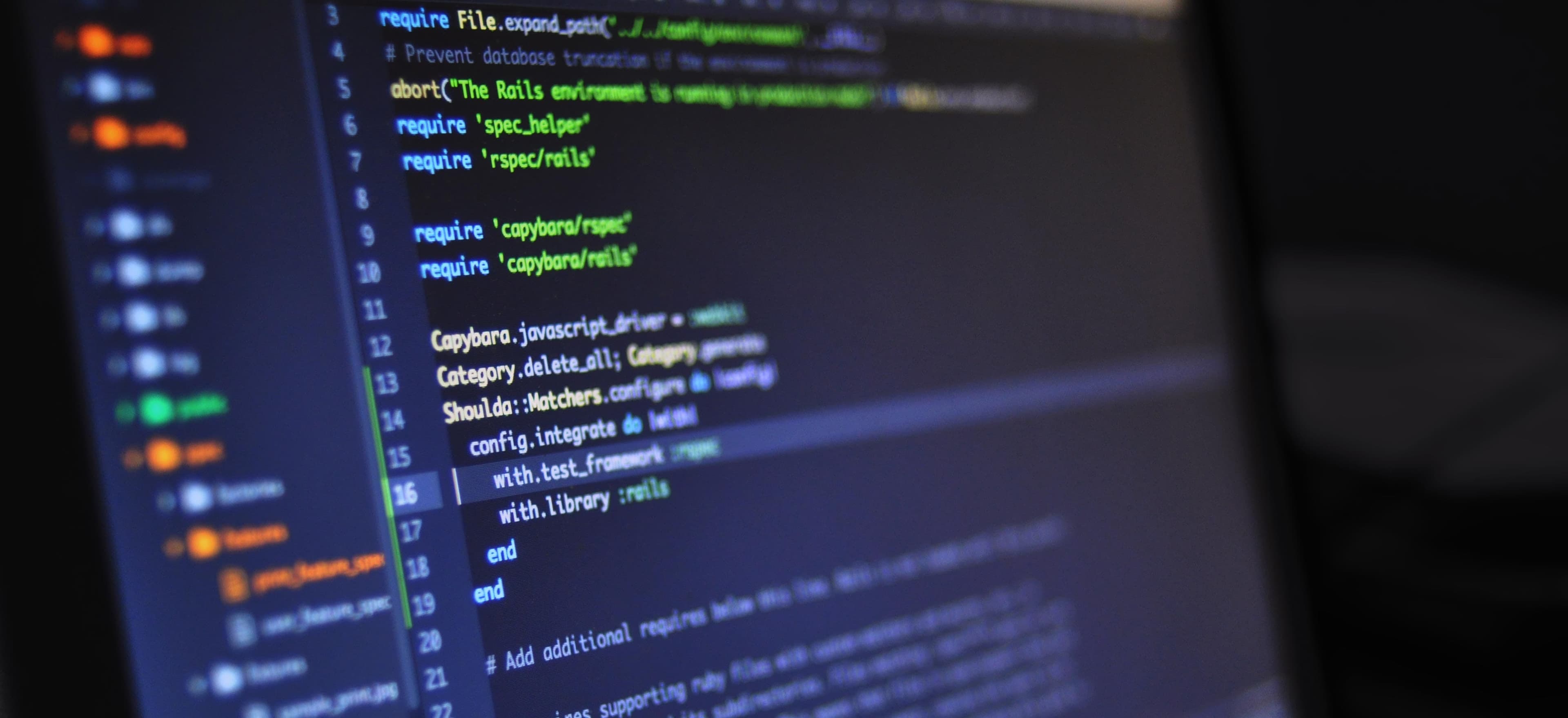
- Published on
Solving Method Call Resolution Issues in Java with JavaSymbolSolver
Java is widely loved for its readability and structure. However, developers often face challenges with method call resolution, especially in complex codebases. Fortunately, tools like JavaSymbolSolver can significantly help address these issues. In this post, we will explore how to resolve method call ambiguities using JavaSymbolSolver and best practices to ensure code clarity and maintainability.
Understanding Method Call Resolution in Java
Method call resolution refers to the process through which the Java compiler determines which method is invoked when a method is called. This process can become complex due to factors like:
- Overloading: Multiple methods with the same name but different signatures.
- Inheritance: Methods in parent classes can be overridden in subclasses.
- Interfaces: Multiple interfaces may declare methods with the same signature.
When these factors collide, Java's compiler can sometimes struggle to resolve the correct method, leading to errors like cannot find symbol
or ambiguous method calls.
Why Use JavaSymbolSolver?
JavaSymbolSolver is a library that interacts with JavaParser, allowing for deeper analysis of Java code. It provides:
- Enhanced symbol resolution capabilities.
- Ability to analyze both source code and compiled code.
This tool is particularly useful in larger projects where class hierarchies can be extensive, and tracking method calls can be tricky. By utilizing JavaSymbolSolver, you can streamline your debugging efforts and enhance your understanding of call resolution.
Setting Up JavaSymbolSolver
Before diving into method resolution with JavaSymbolSolver, ensure you have the necessary dependencies in your project. Add the following Maven dependencies to your pom.xml
:
<dependency>
<groupId>com.github.javaparser</groupId>
<artifactId>javaparser-core</artifactId>
<version>3.23.0</version>
</dependency>
<dependency>
<groupId>com.github.javaparser</groupId>
<artifactId>javaparser-symbol-solver-core</artifactId>
<version>3.23.0</version>
</dependency>
These libraries provide the necessary classes and interfaces to work efficiently.
Basic Implementation
Let's outline a simple implementation of JavaSymbolSolver:
import com.github.javaparser.JavaParser;
import com.github.javaparser.ast.CompilationUnit;
import com.github.javaparser.symbolsolver.JavaSymbolSolver;
import com.github.javaparser.symbolsolver.resolution.ResolveException;
import com.github.javaparser.symbolsolver.resolution.symbols.MethodSymbol;
import java.io.File;
public class MethodResolver {
public static void main(String[] args) {
// Specify the path to your Java file
File sourceFile = new File("path/to/YourJavaFile.java");
// Parse the Java file into a CompilationUnit
CompilationUnit cu = JavaParser.parse(sourceFile);
// Set up the symbol solver
JavaSymbolSolver symbolSolver = new JavaSymbolSolver();
symbolSolver.setRootNode(cu);
try {
// Resolve a specific method call
MethodSymbol methodSymbol = symbolSolver.solveMethod("methodName", new Class<?>[]{String.class}).getCorrespondingMethod();
System.out.println("Method found: " + methodSymbol);
} catch (ResolveException e) {
System.out.println("Method resolution failed: " + e.getMessage());
}
}
}
Code Explanation
In the above code, we:
- Imported necessary classes from JavaParser and JavaSymbolSolver.
- Parsed a Java file to produce a
CompilationUnit
. This is crucial as it transforms your code into an object model for further manipulation. - Set up the symbol solver to operate on the parsed code.
- Attempted to resolve a method using its name and expected parameter types, catching any potential exceptions.
This foundational example can be expanded upon to suit various needs based on the complexity of your project.
Advanced Method Resolution Scenarios
Handling Overloading
Method overloading can introduce additional layers of complexity in method resolution. Consider the following class:
class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
When trying to resolve which add
method to call, you will need to specify the parameter types correctly:
MethodSymbol methodSymbol = symbolSolver.resolveMethod("add", new Class<?>[]{int.class, int.class}).getCorrespondingMethod();
This specificity helps JavaSymbolSolver differentiate between the overloaded methods.
Working with Inherited Methods
Another common challenge is when dealing with classes that extend other classes. If a subclass overrides a superclass's method, you want to ensure you are referencing the right one. Consider the following:
class Animal {
public void sound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
@Override
public void sound() {
System.out.println("Bark");
}
}
If you try to resolve sound
in either class, JavaSymbolSolver will help determine the method you are calling based on the reference type used. For instance:
// Resolving sound in Dog class
MethodSymbol dogSoundSymbol = symbolSolver.resolveMethod("sound", new Class<?>[]{}).getCorrespondingMethod();
This will resolve to Dog's sound
method due to method overriding.
Best Practices for Method Call Resolution
-
Use Clear Naming Conventions: When creating methods, try to be as descriptive as possible with names.
-
Avoid Overloading When Possible: While overloading is a feature of Java, excessive use can lead to confusion. Use clear, distinct method names when you can.
-
Specify Parameter Types: When resolving methods, always provide parameter types to avoid ambiguity.
-
Keep Inheritance Hierarchies Manageable: A deeply nested structure can complicate method resolution significantly. Aim for a flat class hierarchy where applicable.
Closing Remarks
Method call resolution is a critical aspect of working effectively within Java. By leveraging JavaSymbolSolver, developers can take significant steps toward resolving ambiguities in method calls and improving overall code clarity.
Employing the strategies and code snippets we've discussed, combined with the JavaSymbolSolver’s capabilities, can transform your debugging experience and enhance the maintainability of your code. For more information and detailed documentation, visit the JavaSymbolSolver official site.
Happy coding!