Mastering LocalDateTime: Overcoming Common Pitfalls
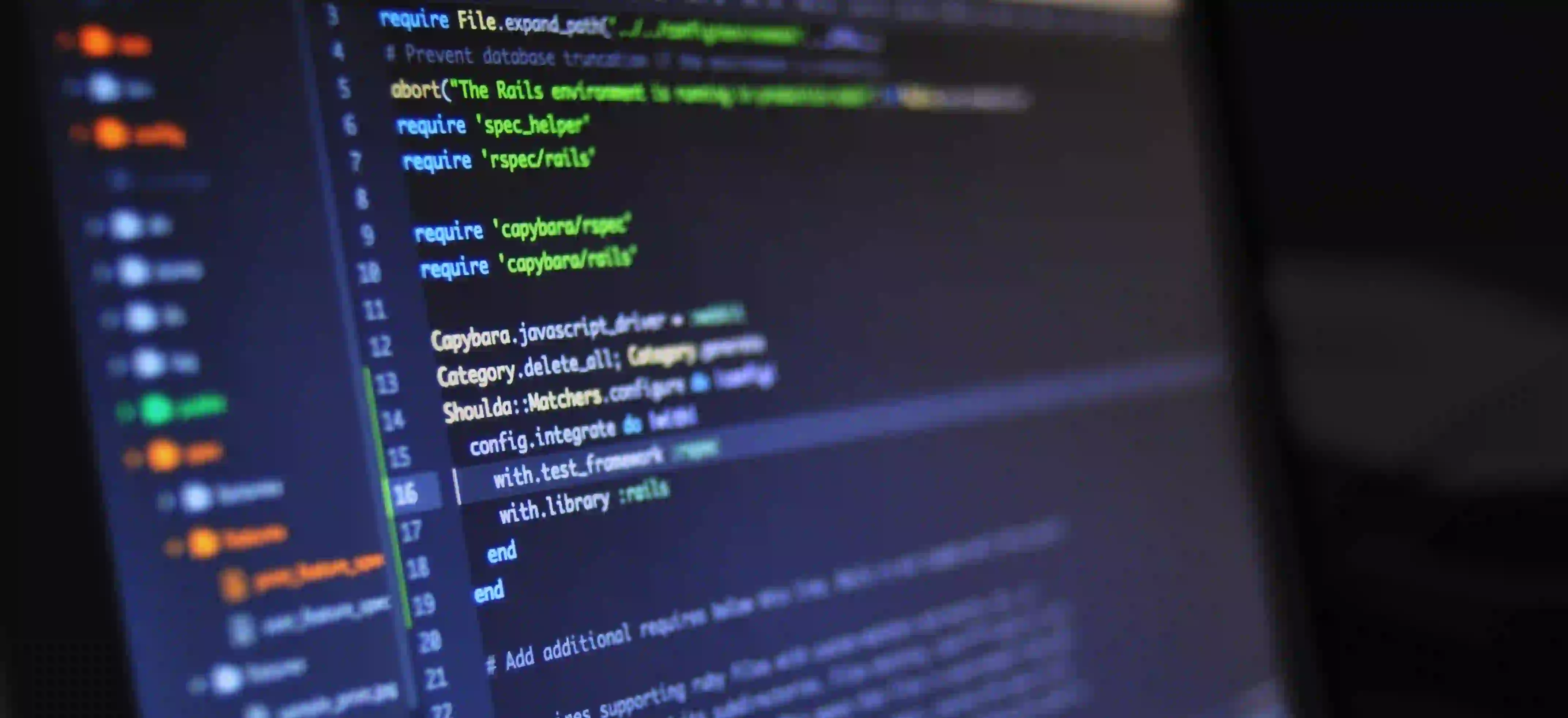
Mastering LocalDateTime: Overcoming Common Pitfalls
Handling dates and times in programming can often be daunting. In Java, the LocalDateTime
class has emerged as a popular choice to manage date-time without timezone information. However, as developers dive deeper into using this class, they frequently encounter pitfalls that lead to erroneous computations or unexpected behavior. This blog post aims to address these common issues, along with providing actionable solutions to master LocalDateTime
in your applications.
What is LocalDateTime?
Before we dive into pitfalls, let’s clarify what LocalDateTime
is. Added in Java 8, LocalDateTime
is a part of the java.time
package and represents a date-time without a timezone in the ISO-8601 calendar system. It’s particularly useful for applications that do not require timezone calculations and need simplicity.
import java.time.LocalDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
System.out.println("Current Date and Time: " + now);
}
}
Why Use LocalDateTime?
You might wonder why to prefer LocalDateTime
over other date-time classes. Here are a few compelling reasons:
-
Immutability:
LocalDateTime
objects are immutable. This design pattern enhances thread safety and reduces bugs related to mutable states. -
Comprehensibility: The API is designed for ease of use and readability, enabling developers to express their intentions clearly.
-
Formatting and Parsing: Built-in support for formatting and parsing makes it convenient for various use cases.
Common Pitfalls with LocalDateTime
Now that we appreciate the benefits of LocalDateTime
, let’s explore some typical problems developers face.
Pitfall 1: Assuming LocalDateTime is Timezone-Aware
One of the most significant misconceptions about LocalDateTime
is that it inherently contains timezone information. In reality, it does not consider time zones at all. This oversight can lead to severe logical bugs in applications linked to different time zones or during day-light saving transitions.
Solution: If your application needs to handle timezone, use ZonedDateTime
instead.
import java.time.ZonedDateTime;
public class ZonedDateTimeExample {
public static void main(String[] args) {
ZonedDateTime zonedNow = ZonedDateTime.now();
System.out.println("Current Date and Time with Time Zone: " + zonedNow);
}
}
Pitfall 2: Misuse of Formatting
When converting a LocalDateTime
to a string, developers often overlook the correct pattern to use in DateTimeFormatter
. Using the wrong format can yield unexpected results or even exceptions.
Solution: Always ensure you’re using the correct pattern for formatting.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeFormatting {
public static void main(String[] args) {
LocalDateTime dt = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = dt.format(formatter);
System.out.println("Formatted Date and Time: " + formattedDateTime);
}
}
Pitfall 3: Calculation Issues
Calculating durations or periods can be tricky. A common error is not accounting for changes like leap seconds or variations in month lengths.
Solution: Utilize the Duration
and Period
classes.
import java.time.LocalDateTime;
import java.time.Duration;
public class DurationExample {
public static void main(String[] args) {
LocalDateTime start = LocalDateTime.now();
LocalDateTime end = start.plusHours(2).plusMinutes(30);
Duration duration = Duration.between(start, end);
System.out.println("Duration in hours and minutes: " + duration.toHours() + " hours " + duration.toMinutesPart() + " minutes");
}
}
Pitfall 4: Limited Support for Dates Beyond 999999999
LocalDateTime
has its limitations; it's only useful in the range from MIN
(0001-01-01T00:00) to MAX
(9999-12-31T23:59:59.999999999). Trying to operate outside this range will throw exceptions.
Solution: If you need to work with historical dates, consider using LocalDate
along with custom handling.
import java.time.LocalDateTime;
import java.time.LocalDate;
public class HistoricalDateExample {
public static void main(String[] args) {
LocalDate historicalDate = LocalDate.of(500, 1, 1); // Supports dates back to year 500
LocalDateTime baseDateTime = historicalDate.atStartOfDay();
System.out.println("Historical Date and Time: " + baseDateTime);
}
}
Best Practices for Using LocalDateTime
To further help you navigate the LocalDateTime
landscape effectively, consider the following best practices:
-
Use Factory Methods: Always use provided factory methods like
LocalDateTime.now()
,LocalDateTime.of()
, andLocalDateTime.parse()
for better clarity and consistency. -
Be Mindful of Serialization: When sending
LocalDateTime
objects over the network or saving to databases, consider how they are serialized and deserialized to avoid loss of information. -
Combine with Java 8 Streams: Use the power of streams for working with collections of
LocalDateTime
values to perform filter and mapping operations. -
Stay Updated with Java's Date-Time API: Always keep an eye on enhancements or updates in the
java.time
package for more efficient functionalities.
Additional Resources
-
For a deeper understanding of Java's date-time API, refer to the official Java documentation.
-
To delve into time zone management, check out this invaluable resource covering timezone-specific date-time management.
Lessons Learned
Mastering LocalDateTime
is not merely about knowing its functionalities but also understanding its limitations and common pitfalls. Being aware of these pitfalls, along with the accompanying solutions and best practices, can help you avoid major headaches in your Java applications. Let LocalDateTime
simplify your date-time handling, but make sure to remain vigilant and informed to use it effectively.
By being proactive in your approach and employing best practices, you will navigate the challenges of date-time management with confidence and efficiency.
Happy coding!