Common Pitfalls in JavaFX Tower Defense Game Development
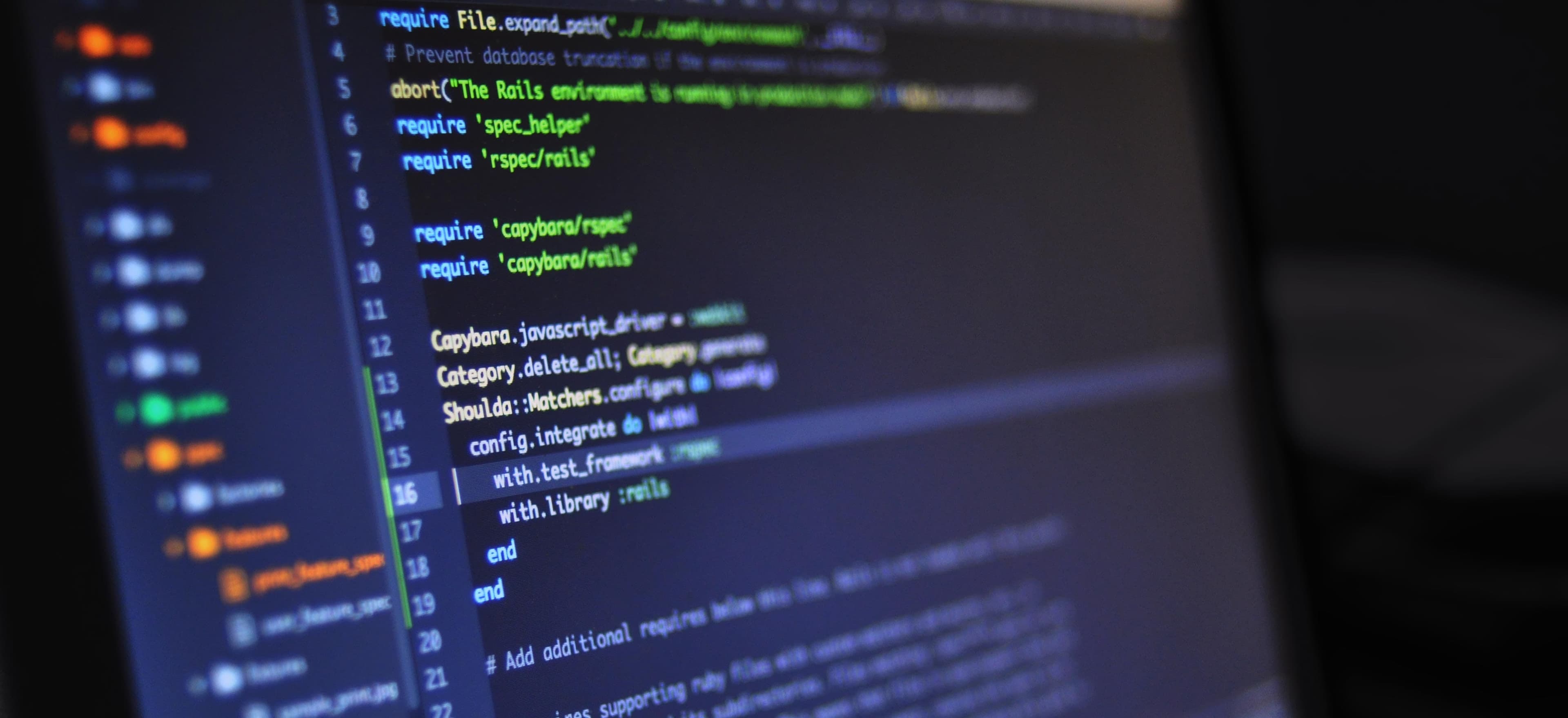
- Published on
Common Pitfalls in JavaFX Tower Defense Game Development
Tower defense games have long enjoyed popularity among gamers due to their strategy-based mechanics and engaging gameplay. Utilizing JavaFX for game development, especially for a tower defense game, can be both rewarding and challenging. In this blog post, we'll discuss common pitfalls in JavaFX tower defense game development and how to avoid them.
1. Performance Issues
Understanding the Challenge
JavaFX is a great framework for creating rich desktop applications, but it can struggle with performance when rendering many game elements, especially in complex scenes.
Solution: Optimize Rendering
One primary optimization tactic is to limit unnecessary re-renders. Ensure that you only redraw elements of the game that have changed instead of the entire scene.
Example Code Snippet:
public void updateGame() {
// Update game logic
if (playerBase.getHealth() <= 0) {
gameOver();
return;
}
// Only redraw the affected areas
playerBase.draw(); // Method only updates the player's health
enemies.forEach(Enemy::draw); // Update enemies only
}
Explanation: In this code, we target specific game elements to update rather than a wholesale scene redraw, improving performance and responsiveness.
2. Inadequate Game State Management
Understanding the Challenge
Game state management is crucial. Failure to effectively manage different game states (menu, playing, paused, game over) can lead to user confusion and bugs.
Solution: Implement a State Pattern
Utilizing the State design pattern can help manage various game states more systematically.
Example Code Snippet:
public interface GameState {
void handleInput(Input input);
void update();
void render(GraphicsContext gc);
}
public class MenuState implements GameState {
@Override
public void handleInput(Input input) {
if (input.isStartGame()) {
game.setCurrentState(new PlayingState());
}
}
// Other method implementations...
}
Explanation: This state management system lets us encapsulate game states within their respective classes, which cleanly separates logic and makes your game easier to maintain.
3. Lack of Resource Management
Understanding the Challenge
Resource management in games is critical. Inadequately loading or freeing resources can lead to memory leaks or performance bottlenecks.
Solution: Use Lazy Loading
Loading resources only when needed will keep your application lightweight and can significantly reduce initial load times.
Example Code Snippet:
public Image loadImage(String path) {
if (imageCache.containsKey(path)) {
return imageCache.get(path);
}
Image img = new Image(path);
imageCache.put(path, img);
return img;
}
Explanation: Here, we utilize a simple caching mechanism to load images only when necessary. This reduces memory usage and speeds up loading times.
4. Poor User Interface Designing
Understanding the Challenge
A cluttered or unresponsive UI can frustrate players. Balancing visual clarity and user interaction is vital.
Solution: Adopt Responsive Design
Make your UI responsive to various resolutions and input methods.
Example Code Snippet:
button.setOnAction(e -> {
// Update UI with new game parameters
updateGameUI();
});
public void updateGameUI() {
// Calculate position based on window size
double centerX = scene.getWidth() / 2;
button.setLayoutX(centerX - (button.getWidth() / 2));
}
Explanation: This code ensures buttons and other UI elements are centered and adjust based on the game's window size, creating a more pleasant user experience.
5. Overcomplicating Game Logic
Understanding the Challenge
Java developers often fall into the trap of over-architecting their game logic, making it hard to manage and debug.
Solution: Keep It Simple
Start with the Minimum Viable Product (MVP) for your game mechanics. This allows you to focus on core functionality first.
Example Code Snippet:
public void createBasicTower(Point position) {
Tower tower = new Tower(position);
towers.add(tower);
}
Explanation: Focus on creating simple tower objects before diving into complex attributes or behavior. This helps in iteratively building your game.
6. Failure to Test Rigorously
Understanding the Challenge
Not testing your game thoroughly can lead to bugs, especially when different elements interact uniquely.
Solution: Implement Unit Testing
Utilize JUnit for JavaFX to ensure your game logic works as expected.
Example Code Snippet:
@Test
public void testTowerDamage() {
Tower tower = new Tower(10);
tower.shoot();
assertEquals(90, enemy.getHealth());
}
Explanation: This unit test checks whether the tower correctly applies damage to enemies. It ensures that your game mechanics are functioning as intended.
7. Ignoring Game Balancing
Understanding the Challenge
In tower defense games, balancing the difficulty is crucial. Ignoring this can either lead to a too-easy game or an excessively frustrating experience.
Solution: Iterative Testing
Playtest your game frequently and adjust parameters based on feedback.
Example Code Snippet:
public void adjustEnemyAttributes(int waveNumber) {
if (waveNumber % 5 == 0) {
// Every fifth wave becomes more challenging
enemy.setHealth(enemy.getHealth() + 10);
enemy.setSpeed(enemy.getSpeed() + 0.5);
}
}
Explanation: With this code, we incrementally make waves tougher, keeping players engaged while also allowing for learning and strategy development.
The Bottom Line
Developing a tower defense game using JavaFX can be an exciting endeavor. By being aware of common pitfalls like performance issues, inadequate state management, memory management, UI design, overcomplicated logic, the need for rigorous testing, and game balancing, you can steer your project on the right course.
For more in-depth guidance, consider diving into JavaFX resources such as Oracle's JavaFX Documentation or exploring communities like Stack Overflow for troubleshooting help. Happy coding, and may your towers always stand strong!
Checkout our other articles