Diagnosing Java Thread Memory Leaks Effectively
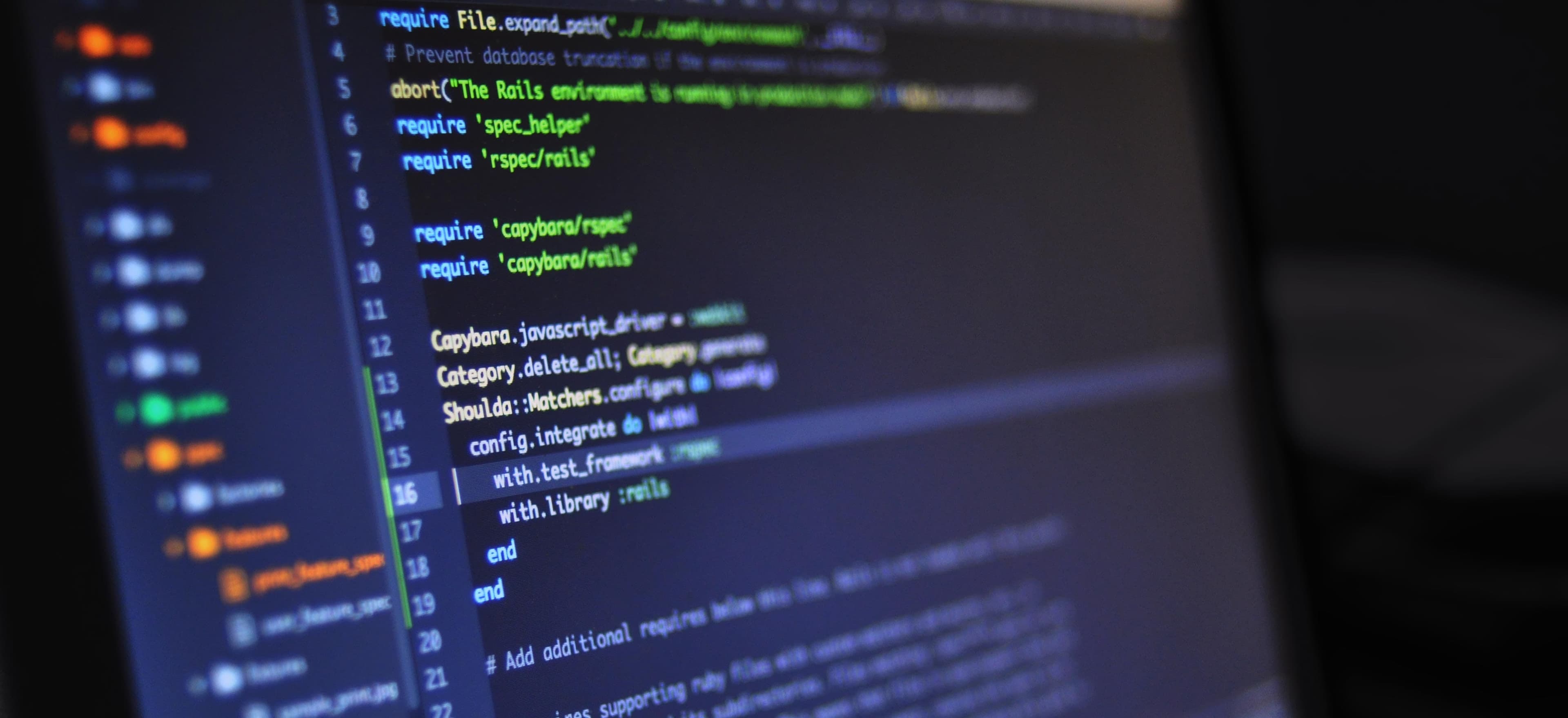
- Published on
Diagnosing Java Thread Memory Leaks Effectively
Memory leaks in Java applications can lead to performance degradation, crashes, and downtime. While Java's automatic garbage collection is designed to manage memory efficiently, developers still have to diagnose and fix memory leaks, especially those associated with threads. In this blog post, we will explore the common sources of thread memory leaks in Java, how to diagnose them, and effective strategies for prevention.
What is a Memory Leak?
A memory leak occurs when an application consumes memory without releasing it back to the system. In Java, this often manifests as objects that are no longer needed but remain referenced, preventing the garbage collector from reclaiming that memory. When it comes to threads, memory leaks can occur due to retained references, listener and callback patterns, or improper use of thread pools.
Common Sources of Thread Memory Leaks
-
Unfinished Threads: When threads are started but not terminated properly, they may continue to hold on to resources.
-
Static References: Static fields holding references to thread-local variables can lead to memory leaks. While static fields live for the duration of the JVM, the objects they point to can grow unexpectedly.
-
Improper Use of Thread Pool Executor: Failing to manage the lifecycle of worker threads can lead to a situation where old threads are not cleaned up.
-
Listener and Callback Mismanagement: If objects that subscribe as listeners or callbacks are not properly deregistered, they might remain in memory longer than intended.
Example of a Memory Leak with Threads
Let's consider a simple example. Below is a Java program that demonstrates a thread memory leak:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private static List<Thread> threads = new ArrayList<>();
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
Thread thread = new Thread(new Task());
thread.start();
threads.add(thread); // Retained reference
}
// Example of an unfinished thread
threads.forEach(thread -> {
try {
thread.join(); // This is where the program waits for threads to finish.
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
static class Task implements Runnable {
@Override
public void run() {
try {
Thread.sleep(10000); // Simulating long-running task
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
Why This Is a Problem
In the above code, we are keeping a reference to each thread in a static list. If this list grows without bounds, it can lead to a memory leak. Once these threads finish their execution, they remain in the list, holding onto resources and impacting the application's memory usage.
Diagnosing Memory Leaks
-
Profiling Tools: Using tools like VisualVM, Eclipse MAT, or JProfiler can help you visualize memory consumption and identify leaks. Profilers can maintain snapshots of the heap memory, enabling you to inspect instances of classes and find stray references.
-
Heap Dump Analysis: Generating a heap dump can be insightful. Use the
jmap
command in Java to create a heap dump:jmap -dump:live,file=heap_dump.hprof <pid>
Analyzing the heap dump file in a tool like Eclipse MAT can reveal which objects are still in memory and what is holding references to them.
-
Thread Dumps: You can generate a thread dump using:
jstack <pid>
Understanding the state of each thread will help you identify which threads are stuck or ongoing unintentionally.
Preventing Thread Memory Leaks
Proper Thread Management
To avoid memory leaks, it's paramount to manage the life cycle of threads correctly.
- Use Executors: Instead of creating threads manually, using the Java Executors framework helps manage a pool of threads efficiently. For example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolingExample {
private static final ExecutorService executor = Executors.newFixedThreadPool(5);
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
executor.execute(new Task());
}
executor.shutdown(); // Properly shut down the executor
}
}
In this example, we use an Executor Service, which helps avoid the need to manage threads directly, thus reducing the chances of memory leaks.
Deregister Listeners
If your classes implement listeners, ensure you have a deregistration mechanism. You can create a method to unregister listeners when they are no longer needed:
class MyEventSource {
private final List<MyEventListener> listeners = new ArrayList<>();
public void registerListener(MyEventListener listener) {
listeners.add(listener);
}
public void unregisterListener(MyEventListener listener) {
listeners.remove(listener); // This one is crucial to prevent memory leaks
}
}
The Bottom Line
Diagnosing and preventing thread memory leaks in Java is critical for maintaining the performance and stability of your applications. By utilizing the right tools and adhering to best practices such as using thread pools and managing listener lifecycles, you can significantly reduce the risk of memory leaks.
For further reading on memory management and debugging in Java, check out these resources:
- Java Memory Management Best Practices
- Understanding Java Garbage Collection
By staying proactive with memory management strategies, you can ensure your Java applications operate smoothly, efficiently, and without leaks. Keep coding wisely!
Checkout our other articles