Mastering the Balance: I18n vs. L10n Strategies
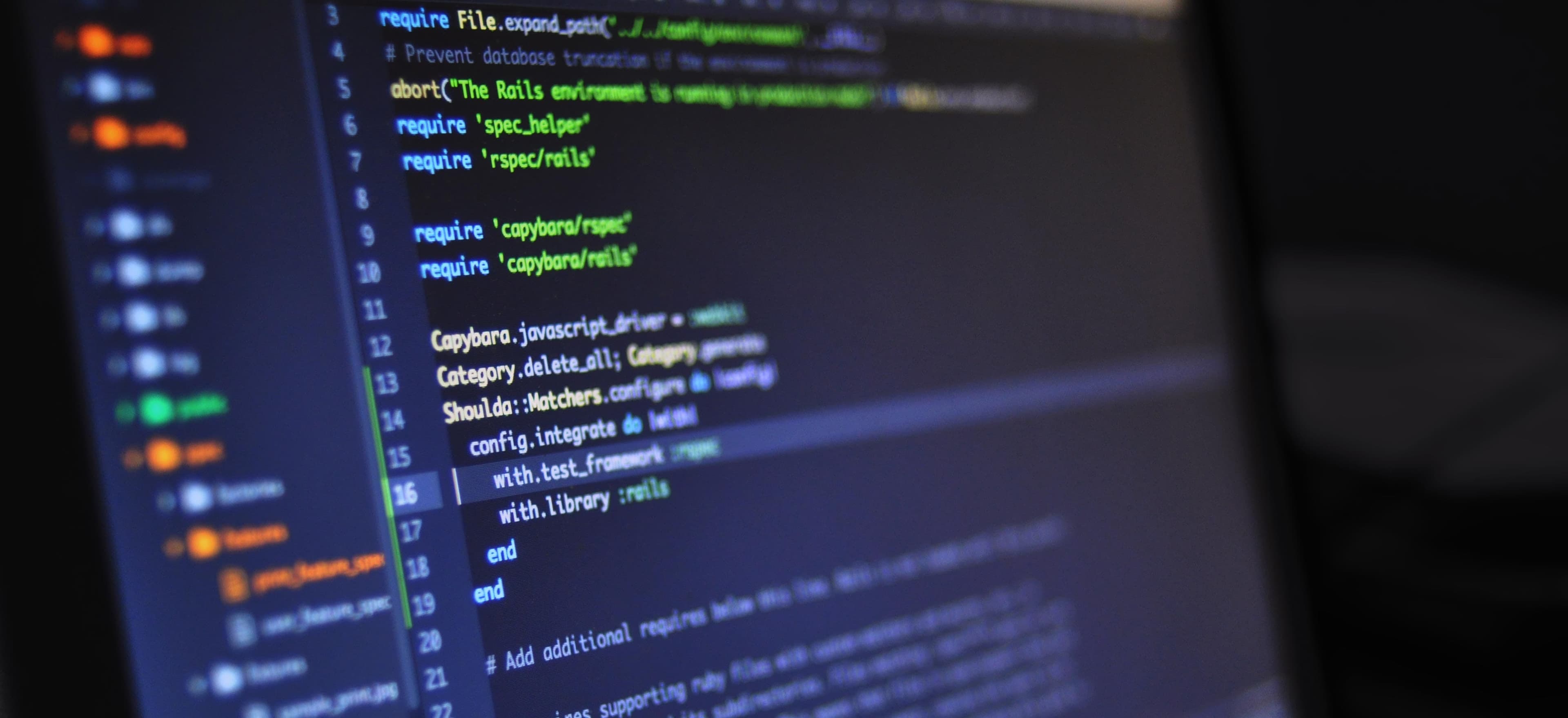
- Published on
Mastering the Balance: I18n vs. L10n Strategies
In the world of software development, especially in the era of global and diverse user bases, understanding Internationalization (I18n) and Localization (L10n) has become a critical component of creating applications that resonate with global audiences. This blog post will cover the key differences between I18n and L10n, their respective strategies, and how to implement them effectively in your projects.
Understanding the Terms: I18n vs. L10n
Before diving deep into the strategies and examples, let's clarify what I18n and L10n mean.
-
Internationalization (I18n) is the process of designing and developing an application so that it can be easily localized for various languages and regions without requiring engineering changes. It involves the infrastructure of your app.
-
Localization (L10n) is the process of adapting your application for a specific market or locale. This includes translating the user interface and adapting the content to meet the cultural expectations of the local audience.
Both terms are crucial in reaching a global audience, but they serve different purposes. I18n lays the groundwork, while L10n is the application of that groundwork in specific contexts.
Strategy: Incorporating I18n in Your Projects
To effectively incorporate I18n in your applications, follow these guidelines:
1. Use Resource Files for Strings
Instead of hardcoding strings directly into the codebase, use resource files. Resource files separate content from code, making it easier to modify for different locales.
Example:
In a Java application, you can create a messages.properties
file for default messages, and additional files like messages_es.properties
for Spanish, messages_fr.properties
for French, etc.
# messages.properties (default)
greeting=Hello
# messages_es.properties (Spanish)
greeting=Hola
# messages_fr.properties (French)
greeting=Bonjour
This organization allows you to add or modify translations without altering the core application code. To load these resources in Java, use the following code snippet:
import java.util.Locale;
import java.util.ResourceBundle;
public class Greeting {
public static void main(String[] args) {
Locale locale = new Locale("es", "ES"); // Spanish Locale
ResourceBundle messages = ResourceBundle.getBundle("messages", locale);
System.out.println(messages.getString("greeting")); // Outputs: Hola
}
}
2. Handle Date and Number Formats
Different countries have different formats for date and numbers. Make sure your app can dynamically change the format based on the user's locale.
Example:
import java.text.NumberFormat;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
public class FormatExample {
public static void main(String[] args) {
Locale locale = new Locale("de", "DE"); // German Locale
NumberFormat numberFormat = NumberFormat.getInstance(locale);
System.out.println(numberFormat.format(1234567.89)); // Outputs: 1.234.567,89
DateFormat dateFormat = DateFormat.getDateInstance(DateFormat.LONG, locale);
System.out.println(dateFormat.format(new Date())); // Outputs date in German format
}
}
In this example, the NumberFormat
and DateFormat
classes adapt to the specified locale to ensure correct representation.
3. Support Bi-Directional Text
When developing for markets where languages are read from right to left (like Arabic or Hebrew), ensure your interface supports bi-directional text. This usually involves adjusting your layout and CSS.
4. Externalize Hardcoded Text
As a best practice, ensure that all user-facing strings in your code are externalized into resource files. This makes it easy for translators to work with your app's content without diving into the source code.
Strategy: Executing L10n with Precision
Now that we have covered the basics of I18n, let’s move to L10n strategies.
1. Employ Professional Translation Services
Don't rely solely on automated translation tools. While they can provide a starting point, professional translation services ensure cultural nuances are considered, making your application feel more natural to the local audience.
Learn more about the vital difference between automated and human translation.
2. Cultural Adaptation
Adapt your content to align with local customs, traditions, and regulations. For instance, images, colors, and even layout can take on different meanings in different cultures.
3. Testing in Local Environments
After localizing your application, testing is crucial. Simulate local environments to identify potential issues with translations, formatting, and cultural relevance.
Example:
Consider a simple feedback form that needs to be localized:
<form action="/submitFeedback" method="post">
<label for="comments">Comments:</label>
<textarea id="comments" name="comments"></textarea>
<button type="submit">Submit</button>
</form>
In Spanish, you’d convert this to:
<form action="/submitFeedback" method="post">
<label for="comments">Comentarios:</label>
<textarea id="comments" name="comments"></textarea>
<button type="submit">Enviar</button>
</form>
Try to get local feedback on such changes to ensure clarity and proper communication.
4. Monitor User Feedback
After localizing your app, closely monitor user feedback and analytics data. This information can provide insights into the effectiveness of your localization efforts and highlight areas in need of improvement.
Bridging I18n and L10n: Best Practices
Achieving the perfect blend of I18n and L10n is essential. Here are some best practices:
-
Involve Local Teams Early: Engage local teams during the design phase for insights into cultural norms and preferences.
-
Iterate and Improve: Language and cultural contexts change. Periodically revisiting your I18n and L10n strategies is essential for staying updated.
-
Test Continually: Make testing a recurring process, especially after any updates or new features in your app.
-
Use Tools: Utilize software solutions like Transifex or Phrase which simplify I18n and L10n processes.
Key Takeaways
In a world where applications can reach users across various geographies, understanding the nuance of I18n and L10n is no longer optional but essential. By incorporating strategic I18n practices into your app development, you prepare the foundation for a seamless L10n process.
Mastering the balance between these two concepts will not only improve the user experience but also broaden your application's appeal. So as you embark on your journey in global markets, keep in mind that a well-crafted strategy is your key to success.
Incorporating these practices will invariably lead to not just better software but also happier users. After all, your goal is to build a product that speaks their language – and resonates with their culture.
Happy coding!
Checkout our other articles