Groovy 2.1 Slows Down Java 7: Key Performance Issues Explained
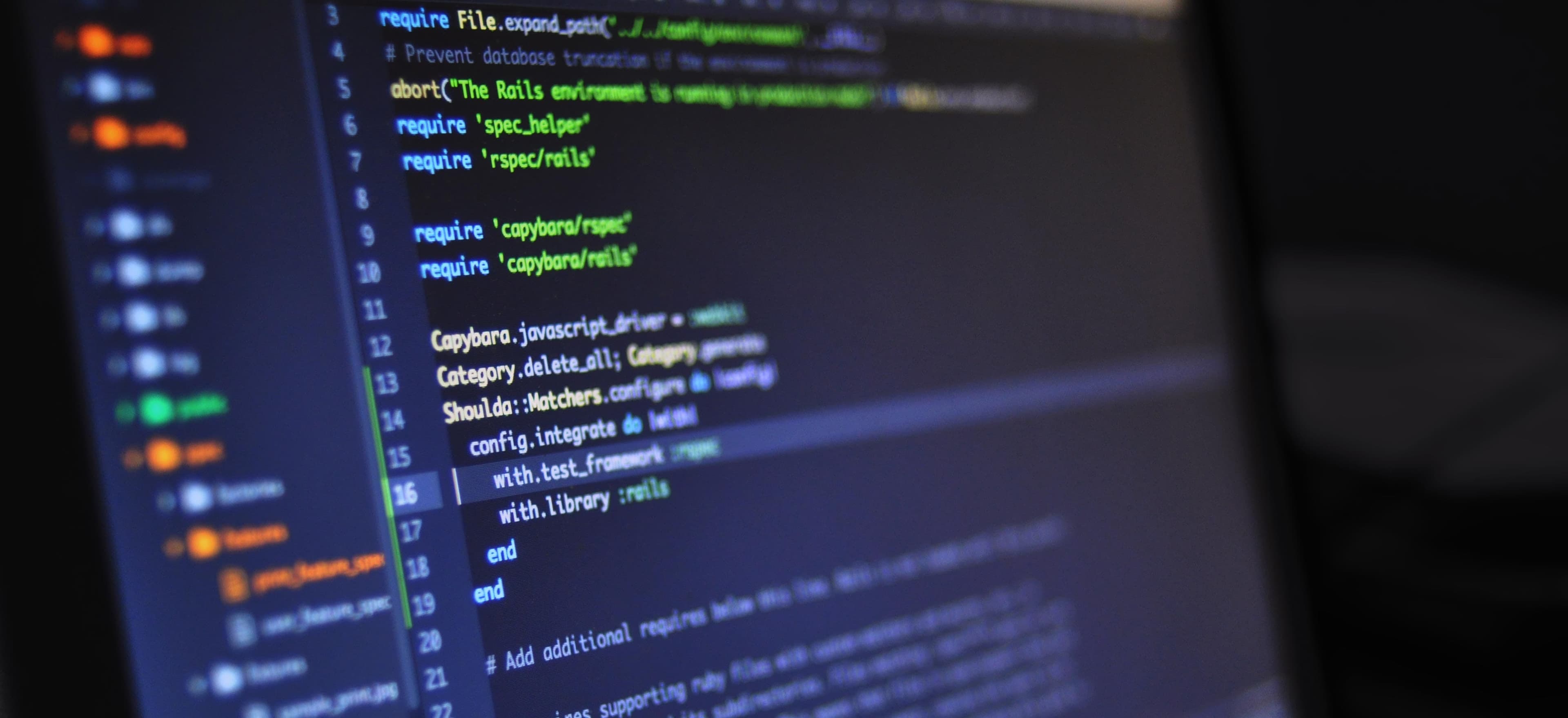
- Published on
Groovy 2.1 Slows Down Java 7: Key Performance Issues Explained
In the fast-paced world of software development, maintaining high performance is crucial, especially when working with languages that run on the Java Virtual Machine (JVM) like Groovy. As the evolution of Groovy continues, performance can vary across different versions. In this blog post, we'll delve into the key performance issues associated with Groovy 2.1 in the context of Java 7.
Understanding Groovy and Java
Before we jump into performance analysis, let’s recap what Groovy and Java are.
- Java is a widely-used programming language known for its robustness, portability, and performance.
- Groovy, on the other hand, is a dynamic language built on the Java platform. It integrates smoothly with existing Java code and offers syntax enhancements.
The flexibility of Groovy comes at a cost: performance. As Groovy evolves, it sometimes introduces inefficiencies which can be exacerbated when running alongside Java versions like Java 7.
Key Factors Contributing to Performance Issues
With Groovy 2.1, several factors contribute to its performance issues when running on Java 7:
1. Dynamic Nature of Groovy
Groovy's dynamic typing and run-time metaprogramming capabilities make it easy to write concise code. However, this flexibility can lead to overhead that degrades performance compared to statically typed Java.
Example
Consider the difference in handling method calls in Java and Groovy:
Java:
public class Example {
public int sum(int a, int b) {
return a + b;
}
}
Example example = new Example();
int result = example.sum(5, 10);
Groovy:
class Example {
int sum(a, b) {
return a + b
}
}
def example = new Example()
def result = example.sum(5, 10)
Commentary: While Groovy reduces boilerplate and improves readability, it incurs a performance hit from runtime method resolution. The Groovy interpreter must constantly interpret types, leading to slower execution.
2. Bytecode Generation Overhead
Groovy utilizes a more complex bytecode generation process compared to Java. Each Groovy class is converted into bytecode dynamically, and this adds layers of abstraction that can slow down execution.
Example
When defining a simple class in Groovy, the underlying bytecode contains additional instructions and logic to facilitate dynamic behavior.
Groovy:
class Person {
String name
int age
String greet() {
return "Hello, my name is $name and I am $age years old."
}
}
Commentary: The Groovy compiler introduces extra bytecode instructions to handle properties and method invocation. While it adds powerful features, it also increases compile time and runtime overhead.
3. Collection Manipulation Performance
Groovy collections often outshine their Java counterparts in terms of functionality but can lag in performance. The dynamic nature and fluent API often lead to chains of method calls that can slow down execution speed.
Example
Consider this Groovy closure that filters a list:
def numbers = [1, 2, 3, 4, 5]
def evenNumbers = numbers.findAll { it % 2 == 0 }
Commentary: This code is brief and easy to read. However, every time the closure is called, Groovy incurs overhead for additional context and evaluation, which can impact performance when processing large collections.
Benchmarking Groovy 2.1 vs. Java 7
To illustrate the performance discrepancies between Java and Groovy, let's look at a simple benchmarking scenario.
import java.util.List;
import java.util.ArrayList;
public class PerformanceTest {
public static void main(String[] args) {
long startTime = System.nanoTime();
List<Integer> list = new ArrayList<>();
for(int i = 0; i < 1000000; i++) {
list.add(i);
}
long endTime = System.nanoTime();
System.out.println("Java execution time: " + (endTime - startTime) + "ns");
}
}
In comparison, here's a Groovy equivalent:
def start = System.nanoTime()
def list = (0..<1000000).collect { it }
def end = System.nanoTime()
println "Groovy execution time: ${end - start}ns"
Commentary: When you run both snippets, you typically find that the Java version runs significantly faster in heavy computational scenarios. This stark contrast underscores the impact of Groovy's abstraction.
Best Practices to Improve Performance with Groovy 2.1
While Groovy 2.1 may have its pitfalls, developers can employ several best practices to mitigate performance issues:
1. Use Static Type Checking
Static type checking helps minimize the overhead introduced by dynamically typed code. By using the @TypeChecked
annotation, you can catch errors at compile time rather than runtime.
import groovy.transform.TypeChecked
@TypeChecked
class Example {
int add(int a, int b) {
return a + b
}
}
2. Opt for Compile-Time Static Type Inference
Compile-time type checking is essential for boosting performance. Groovy allows you to use @CompileStatic
, which leads to performance that can closely resemble Java.
import groovy.transform.CompileStatic
@CompileStatic
class Statistics {
static int sum(List<Integer> numbers) {
return numbers.sum()
}
}
3. Limit Use of Closures in Performance-Critical Code
While closures are a powerful feature in Groovy, excessive use can lead to performance degradation, especially in loops. Aim to minimize closures in performance-critical sections.
Instead of:
def numbers = (1..1000000)
numbers.each { println it }
Use:
def numbers = (1..1000000)
for (number in numbers) {
println number
}
4. Profile Your Code
Utilize profiling tools to identify bottlenecks in your Groovy applications. Tools like VisualVM or YourKit can help pinpoint areas where optimization is needed.
To Wrap Things Up
While Groovy 2.1 offers many advantages, developers working with Java 7 should be cognitive of its performance implications. By understanding the nuances and employing best practices, you can still harness the power of Groovy while mitigating its inherent slowdowns.
If you’d like to explore more advanced topics or stay updated on Groovy and Java, consider checking out the Groovy documentation or the Java Performance Tuning Guide.
By being proactive and informed, you can navigate the complexities of language interdependencies, ensuring that your applications remain performant. Happy coding!
This post provides an insight into Groovy 2.1's impact on Java 7 performance, supported by code examples and best practices to overcome the challenges. By following these guidelines, developers can make informed decisions when using Groovy alongside Java.