Unlocking Java Streams: Overcoming Performance Pitfalls
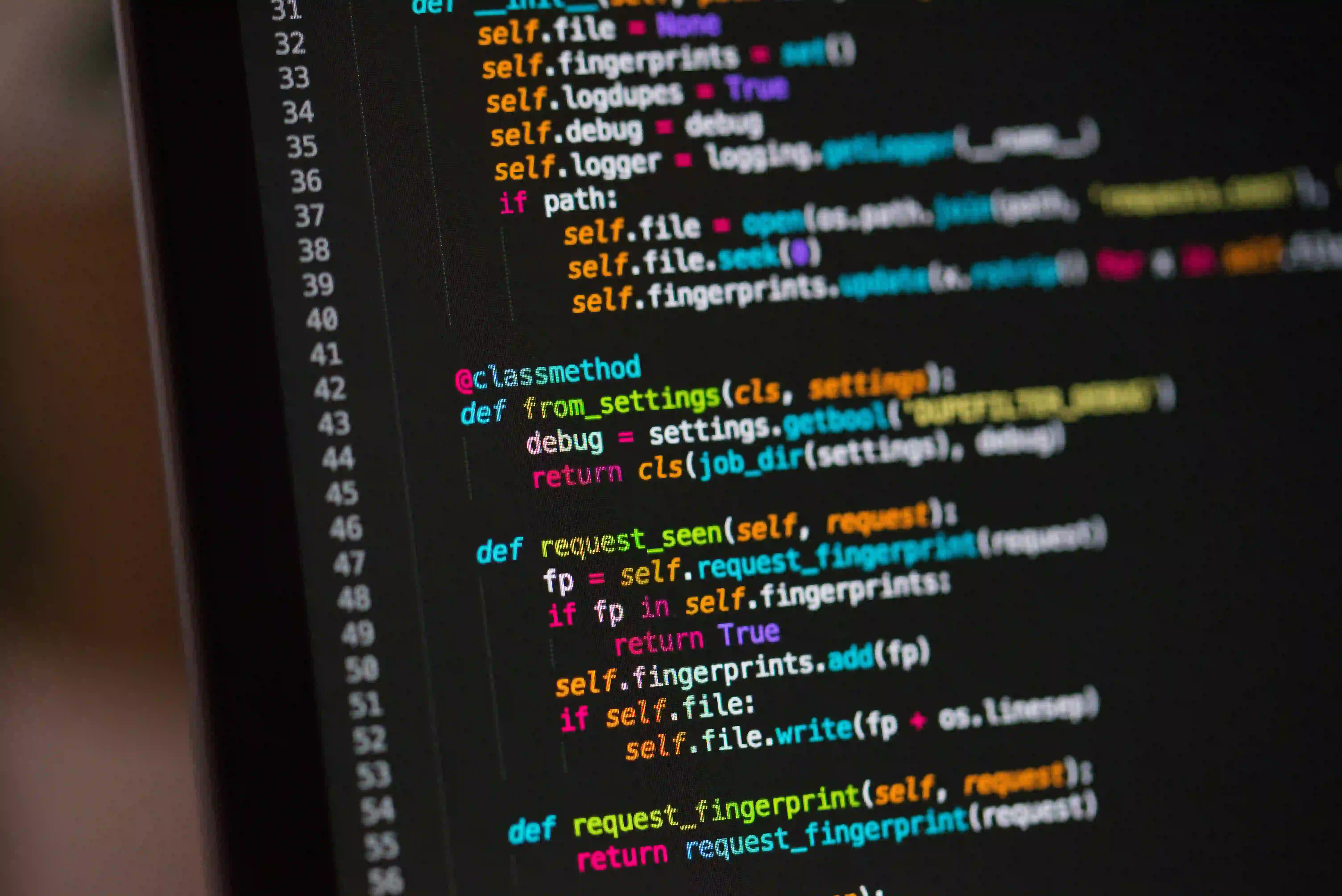
Unlocking Java Streams: Overcoming Performance Pitfalls
Java 8 introduced Streams, a powerful abstraction that enables developers to process sequences of elements seamlessly and expressively. While Streams provide numerous advantages, including parallel processing and a functional programming style, they can also lead to performance pitfalls if misused. In this blog post, we will explore these performance pitfalls and demonstrate how to overcome them, maximizing efficiency while using Java Streams.
Understanding Java Streams
Before diving into performance issues, it's essential to understand what Streams are. A Stream is essentially a sequence of data that you can process in a functional style. They can originate from various data sources, including collections, arrays, or I/O channels. Streams support operations such as filtering, mapping, and reducing.
Example Code Snippet: Basic Stream Operations
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Diana");
// Using Stream to filter and convert names to uppercase
names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.forEach(System.out::println); // Output: ALICE
}
}
In this example, we filter names that start with "A" and convert them to uppercase. This demonstrates the expressive capabilities of Streams, allowing for clean and straightforward code. However, as we will see, achieving optimal performance requires careful consideration.
Common Performance Pitfalls
1. Creating Unnecessary Streams
One of the most common mistakes in using Streams is creating them unnecessarily. Each time you invoke stream()
on a Collection, a new Stream is created. This can lead to performance degradation, especially if the operation is called multiple times.
Example:
import java.util.Arrays;
import java.util.List;
public class UnnecessaryStreams {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Diana");
// Unnecessarily creating a stream multiple times
names.stream().filter(name -> name.startsWith("A")).forEach(System.out::println);
names.stream().filter(name -> name.length() > 3).forEach(System.out::println);
}
}
Here, we created two separate Stream instances for the same names
list. Instead, we can chain the operations into a single Stream to avoid creating additional Streams, improving performance.
Solution: Use a Single Stream
names.stream()
.filter(name -> name.startsWith("A") || name.length() > 3)
.forEach(System.out::println);
2. Not Using Short-Circuiting Operations
Some Stream operations can short-circuit, meaning they can terminate early without having to process all elements. Neglecting these types of operations can make your code inefficient.
Example:
import java.util.List;
public class ShortCircuitExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 3, 5, 7, 12, 15);
// Using filter without short-circuiting
numbers.stream()
.filter(n -> n % 2 == 0)
.findFirst() // Not short-circuiting properly
.ifPresent(System.out::println);
}
}
Though this snippet returns the first even number, it processes all elements unnecessarily. Instead, using findFirst()
directly after a filter allows early termination.
Solution: Combine Filter and FindFirst
numbers.stream()
.filter(n -> n % 2 == 0)
.findFirst() // This line will short-circuit effectively
.ifPresent(System.out::println);
3. Using Parallel Streams Inappropriately
Parallel Streams can be advantageous for CPU-bound tasks since they utilize multiple threads to process data concurrently. However, not all tasks are fit for parallelism, and using parallel streams incorrectly can lead to detrimental performance.
Example:
import java.util.List;
import java.util.stream.Collectors;
public class ParallelStreamExample {
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
// Utilize parallel stream carelessly
List<Integer> squaredNumbers = numbers.parallelStream()
.map(n -> n * n) // Task is trivial; overhead isn't worth it
.collect(Collectors.toList());
}
}
In this case, the overhead of managing multiple threads outweighs the benefits. Trivial tasks should typically remain in a sequential Stream for better performance.
Solution: Assess Task Complexity Before Parallelizing
To decide whether to use a parallel stream, evaluate the complexity and size of the workload. For computationally intensive tasks with larger data sets, parallel Streams are beneficial.
// Only for large datasets or complex calculations
List<Integer> squaredNumbers = numbers.stream() // Use sequential stream for small datasets
.map(n -> n * n)
.collect(Collectors.toList());
4. Collecting Data Inefficiently
When using Streams, choosing the correct collector can have significant performance implications. For instance, utilizing collect(Collectors.toList())
might not always be the best choice.
Example:
import java.util.List;
import java.util.stream.Collectors;
public class CollectorExample {
public static void main(String[] args) {
List<String> names = List.of("Anna", "Bob", "Charlie", "Diana");
// Using list collection without checks
List<String> collectedNames = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toList()); // Memory overhead
}
}
In the above example, if the intention is to only count elements rather than create a list, this can introduce unnecessary overhead.
Solution: Use Appropriate Collector
Instead of collecting into a list when only counting is required, consider:
long count = names.stream()
.filter(name -> name.length() > 3)
.count(); // Returns a count with no list overhead
Closing the Chapter
Java Streams are a powerful tool that, when used correctly, can significantly enhance code expressiveness and efficiency. However, the performance pitfalls discussed in this post can quickly turn advantages into drawbacks if developers are not mindful.
To recap, remember to:
- Avoid creating unnecessary Streams.
- Leverage short-circuiting operations effectively.
- Assess the appropriateness of Parallel Streams.
- Select the right collector based on your intent.
Performance optimization is a journey that requires ongoing learning and adaptation. For more insights on Java programming, check out the official Java documentation and try experimenting with other Stream techniques.
By being aware of these pitfalls and solutions, you can unlock the full potential of Java Streams and create efficient, optimal, and maintainable applications. Happy coding!