How to Handle Consumer Rebalance Issues in Apache Kafka
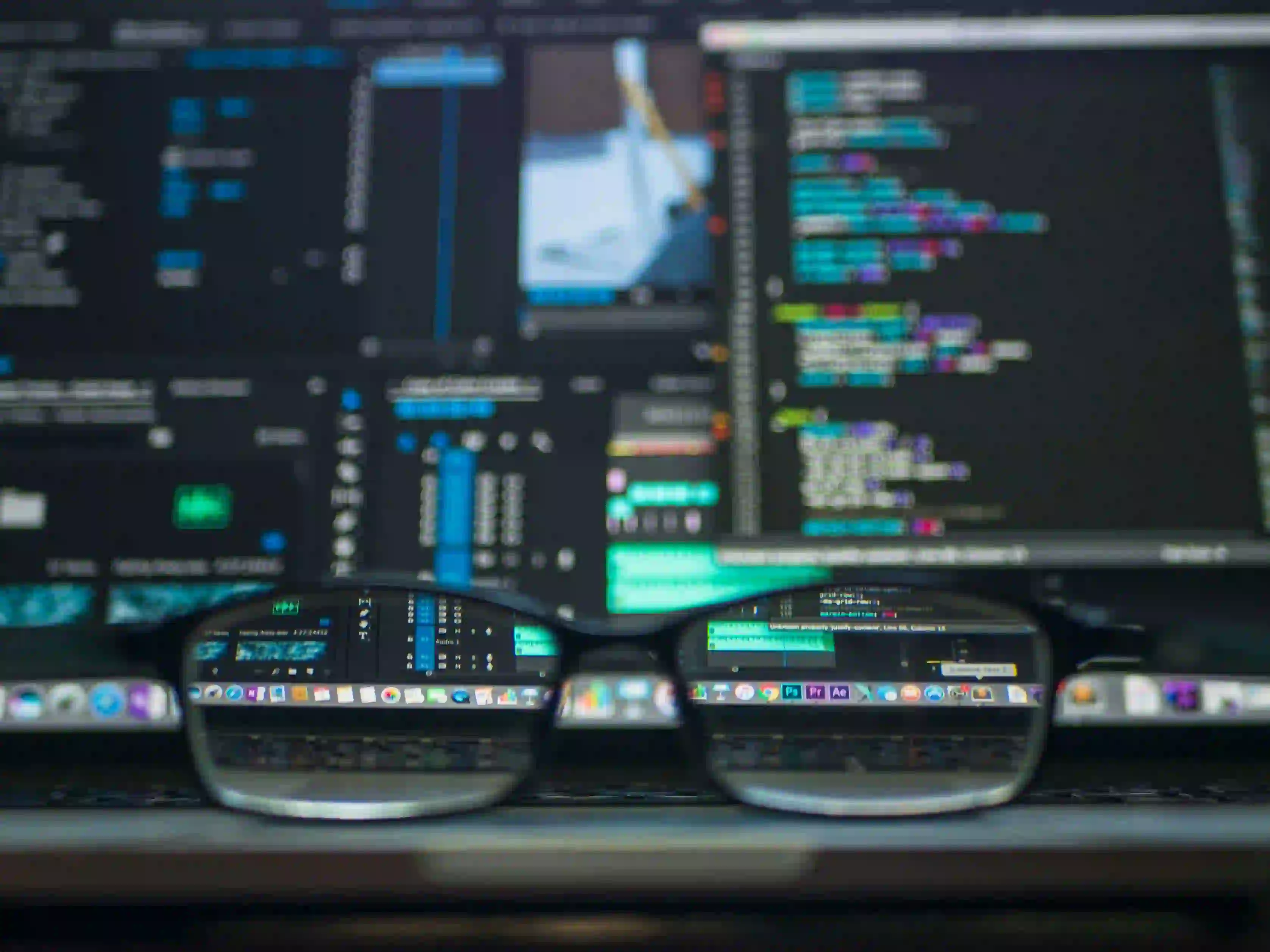
How to Handle Consumer Rebalance Issues in Apache Kafka
When working with Apache Kafka, one must be aware of how consumer groups operate, particularly the challenges that arise during consumer rebalancing. Rebalancing is a crucial aspect of Kafka’s fault-tolerant architecture but can lead to several issues if not handled correctly. In this blog post, we will elucidate what consumer rebalancing entails, the issues it can cause, and the strategies to mitigate these concerns.
Understanding Consumer Rebalancing
In Kafka, consumers are organized into consumer groups. Each consumer within a group reads from one or more partitions assigned to it. As consumers join or leave the group, Kafka automatically redistributes the partitions. This redistribution is known as consumer rebalancing.
Why Rebalancing is Necessary
- Dynamic Scaling: Adding or removing consumers allows systems to scale seamlessly.
- Fault Tolerance: If a consumer crashes, rebalancing ensures that its partitions are reassigned to other consumers.
- Load Distribution: Rebalancing helps distribute the load evenly across active consumers.
While rebalancing is essential, it can also introduce latency and data processing delays. This is where understanding and mitigating its issues become critical.
Common Consumer Rebalance Issues
When a rebalance occurs, the following issues may arise:
- Increased Latency: The time it takes for consumers to reassign partitions can result in message processing delays.
- Duplicate Processing: If not managed correctly, consumers may reprocess messages when they resume after a rebalance.
- Out of Order Processing: Rebalancing can disrupt the order in which messages are processed, especially when multiple consumers are involved.
- State Loss: If a consumer has stateful operations, losing that state due to a rebalance can lead to data inconsistencies.
Strategies to Mitigate Rebalance Issues
1. Understand the Rebalance Protocol
Kafka uses the Cooperative Rebalance protocol to minimize disruption. It ensures that consumers retain their partitions during rebalancing as much as possible.
Implementation Tip: Always use a compatible version of the Kafka client to benefit from the latest rebalancing protocols.
2. Use a Rebalance Listener
Implementing a custom ConsumerRebalanceListener
can help manage tasks before and after the rebalance.
Example Code Snippet
import org.apache.kafka.clients.consumer.Consumer;
import org.apache.kafka.clients.consumer.ConsumerRebalanceListener;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.common.TopicPartition;
import java.util.Collection;
import java.util.Map;
public class CustomRebalanceListener implements ConsumerRebalanceListener {
private final KafkaConsumer<?, ?> consumer;
public CustomRebalanceListener(KafkaConsumer<?, ?> consumer) {
this.consumer = consumer;
}
@Override
public void onPartitionsRevoked(Collection<TopicPartition> partitions) {
// Perform actions before partitions are revoked
System.out.printf("Partitions revoked: %s%n", partitions);
// Commit offsets or checkpoint state here
}
@Override
public void onPartitionsAssigned(Collection<TopicPartition> partitions) {
// Perform actions after partitions are assigned
System.out.printf("Partitions assigned: %s%n", partitions);
// Seek to stored offsets or initialize state here
}
}
Commentary
In the example above, we implement ConsumerRebalanceListener
to handle actions during partition revocation and assignment. By committing offsets or saving the consumer state, we can prevent data loss and duplicate processing. This approach is critical for maintaining stateful and idempotent operations.
3. Minimize Session Timeouts
Adjust the session.timeout.ms
configuration to a higher value. This setting determines how long the consumer can be unreachable before it's considered to be dead. A longer timeout can help avoid unnecessary rebalances.
session.timeout.ms=30000
4. Optimize Heartbeat Intervals
Set the heartbeat.interval.ms
to a lower value than session.timeout.ms
. This allows quicker detection of consumer failures and reduces the chances of unnecessary rebalances.
heartbeat.interval.ms=10000
5. Manage Load Distribution with Partitions
Balancing the number of partitions according to your expected load can help distribute messages more evenly across consumers. A greater number of partitions can facilitate better performance but also increases rebalancing complexities.
You can create partitions using:
kafka-topics.sh --create --topic your-topic --partitions 12 --replication-factor 3 --bootstrap-server localhost:9092
6. Ensure Idempotent Processing
Utilize idempotent consumers where possible. This means structuring your message processing logic so that the same message can be processed multiple times without changing the result.
Example Logic:
void processMessage(String message) {
if (!hasBeenProcessed(message)) {
// Insert message into database
saveToDatabase(message);
}
}
7. Monitor Rebalance Events
Utilize monitoring tools to gain insights into the frequency and duration of rebalances. Tools like Kafka Manager or Kafka Monitor can help identify potential rebalance issues before they escalate.
8. Tune Configuration Settings
Configuration settings such as max.poll.interval.ms
, max.partition.fetch.bytes
, and max.poll.records
can significantly impact consumer performance. Tuning these could reduce rebalance frequency by allowing consumers to poll messages more efficiently.
max.poll.records=500
max.partition.fetch.bytes=1048576
max.poll.interval.ms=300000
Lessons Learned
Handling consumer rebalance issues in Apache Kafka is crucial for maintaining throughput and ensuring data consistency. By understanding the dynamics of rebalancing and utilizing strategies like implementing a ConsumerRebalanceListener
, optimizing configurations, and ensuring idempotent processing, developers can greatly reduce the impact of rebalancing events.
For further reading on Kafka consumer groups and rebalancing strategies, refer to the official Kafka documentation.
Call to Action
Implement these techniques in your Kafka setup and observe the difference in performance and reliability. Share your experiences and challenges with consumer rebalancing in the comments below, so we can continue to improve as a community!
By employing these robust strategies for managing consumer rebalancing in Kafka, you can significantly enhance the resilience and performance of your data processing pipelines. Happy coding!